1 单元测试(白盒测试)
-
测试类 -
独立运行(返回值void 、参数void) -
方法添加@Test -
被测试的类
package com.xhh.calc.calcClass;
public class Calc {
public int addNum(int a, int b){
return a + b;
}
public int subNum(int a, int b){
return a - b;
}
}
package com.xhh.calc.test;
import com.xhh.calc.calcClass.Calc;
import org.junit.After;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
public class CalcTest {
@Before
public void init(){
System.out.println("init 申请资源,如IO等等");
}
@After
public void close(){
System.out.println("close .. 释放资源");
}
@Test
public void testAdd(){
Calc calc = new Calc();
int ret = calc.addNum(1, 2);
Assert.assertEquals(ret, 3);
}
@Test
public void testSub(){
Calc calc = new Calc();
int ret = calc.subNum(1, 2);
Assert.assertEquals(ret, -1);
}
}
2 反射
类对象
- 成员变量:Field[] fields
- 构造方法:Constructor[] cons
- 成员方法:Method[] methods
2.1 获取Class对象的方式
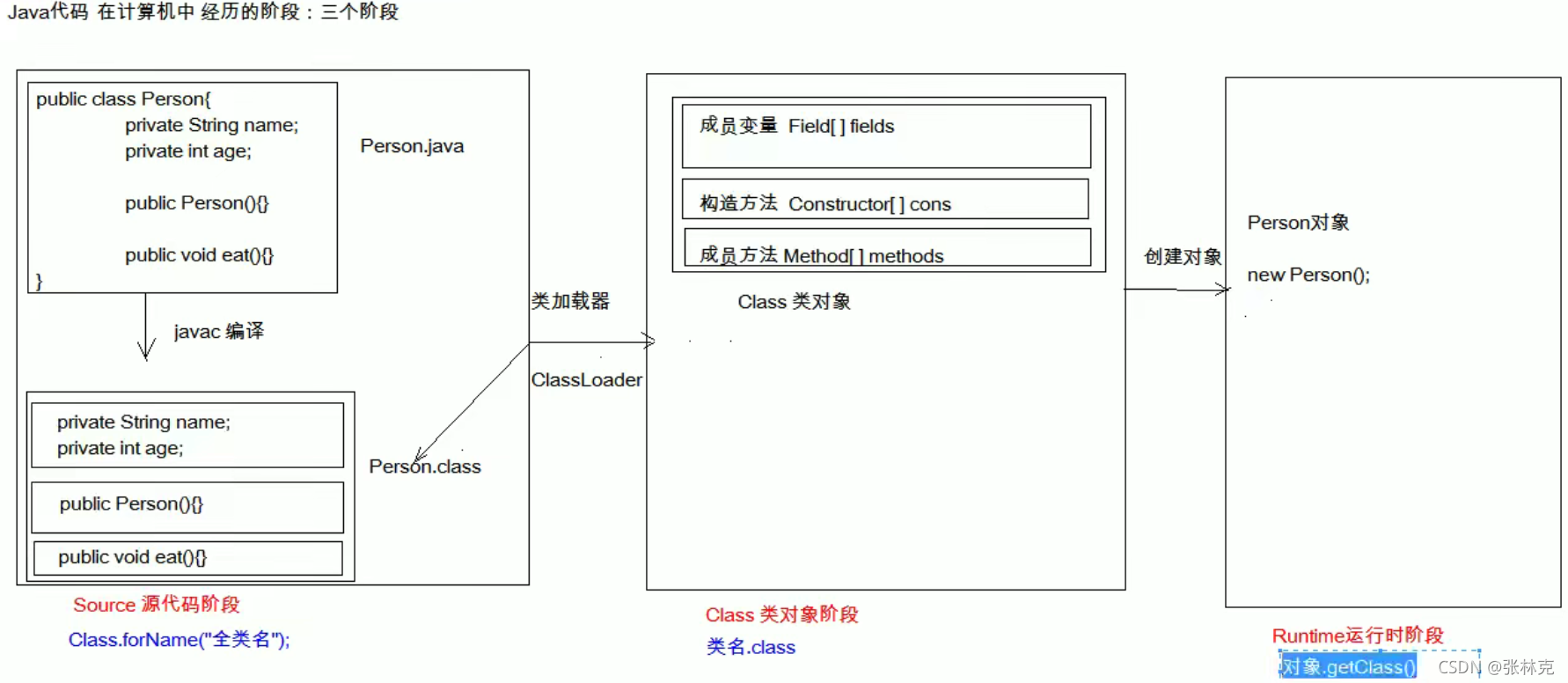
Class.forName("类名");
类名.class
对象.getClass()
package com.xhh.reflection;
import com.xhh.reflection.domain.Person;
public class GetClass {
public static void main(String[] args) throws Exception {
Class<?> cls1 = Class.forName("com.xhh.reflection.domain.Person");
System.out.println(cls1);
Class<Person> cls2 = Person.class;
Person xhh = new Person("xhh", 18);
Class<? extends Person> cls3 = xhh.getClass();
System.out.println(cls1 == cls2);
System.out.println(cls1 == cls3);
}
}
2.2 使用Class
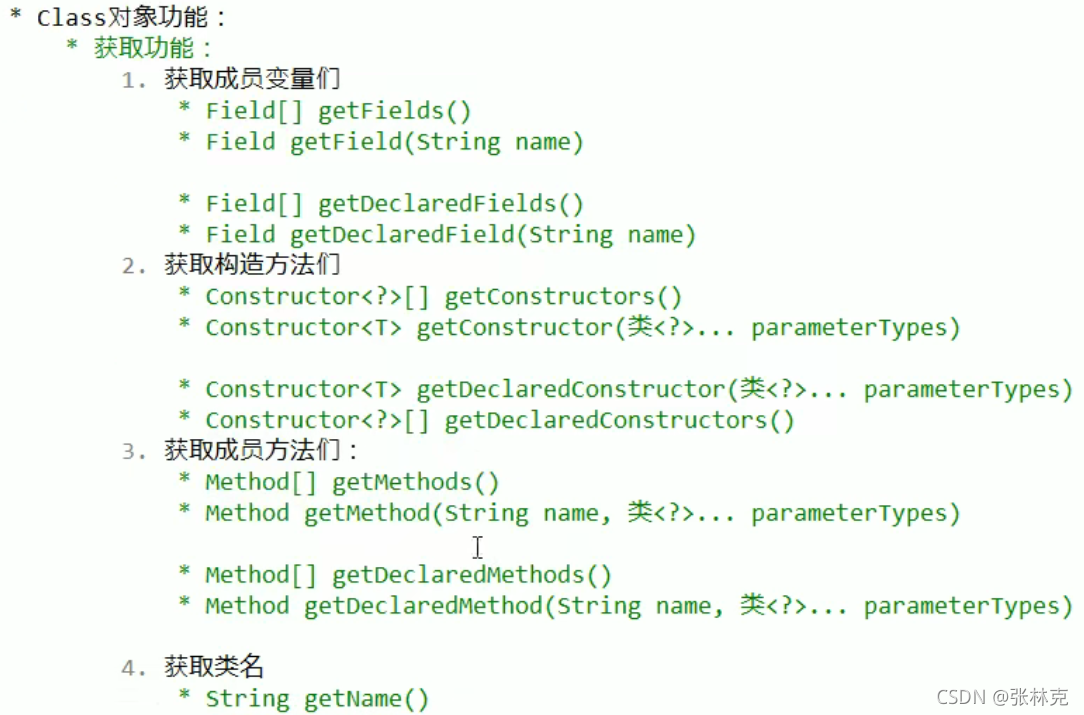
package com.xhh.reflection.domain;
public class Person {
public Person(){}
public Person(String name, Integer age) {
this.name = name;
this.age = age;
}
public void methodInfo(){
System.out.println("methodInfo ...");
}
private String name;
private Integer age;
public String info = "xhh123456";
}
package com.xhh.reflection;
import com.xhh.reflection.domain.Person;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
public class GetClass {
public static void main(String[] args) throws Exception {
Class<?> cls1 = Class.forName("com.xhh.reflection.domain.Person");
System.out.println(cls1);
Constructor<?> cons1 = cls1.getConstructor();
Constructor<?> cons2 = cls1.getConstructor(String.class, Integer.class);
Person mcy = (Person)cons2.newInstance("mcy", 123);
System.out.println(mcy);
Method methodInfo = cls1.getMethod("methodInfo");
methodInfo.invoke(mcy);
Field info = cls1.getField("info");
info.setAccessible(true);
info.set(mcy, "mcy----newValue");
Object o = info.get(mcy);
System.out.println(o);
}
}
2.3 案例
package com.xhh.reflection;
public class Cat {
public void catMethod(){
System.out.println("miao miao miao ...");
}
}
package com.xhh.reflection;
public class Dog {
public void dogMethod(){
System.out.println("wang wang wang ...");
}
}
className=com.xhh.reflection.Dog
methodName=dogMethod
package com.xhh.reflection;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStreamReader;
import java.lang.reflect.Method;
import java.util.Properties;
public class TestPro {
public static void main(String[] args) throws Exception {
Properties properties = new Properties();
properties.load(new FileInputStream("./src/pro.properties"));
String className = properties.getProperty("className");
String methodName = properties.getProperty("methodName");
Class<?> cls = Class.forName(className);
Object obj = cls.newInstance();
Method method = cls.getMethod(methodName);
method.invoke(obj);
}
}
3 注解
3.1 内置三种注解
package com.xhh.reflection;
@SuppressWarnings("all")
public class Annotation_ {
@Override
public String toString(){
return super.toString();
}
@Deprecated
public void showVersion1(){
}
public void showVersion2(){
}
}
3.2 自定义注解
public @interface XhhAnno{
}
- 元注解
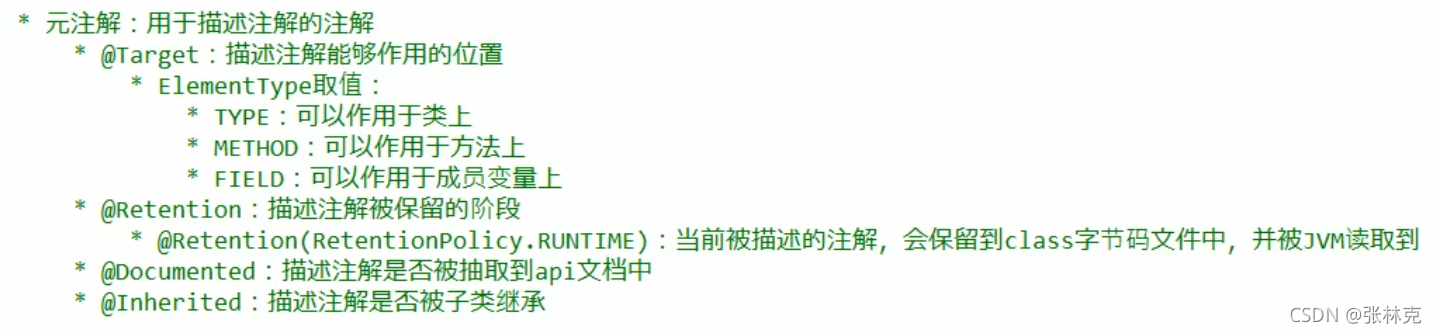
package com.xhh.reflection;
import java.lang.annotation.*;
@Target(value = {ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
public @interface Anno_ {
}
注解
package com.xhh.reflection;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
public @interface LoadPro {
String className();
String methodName();
}
使用
package com.xhh.reflection;
import java.lang.reflect.Method;
@LoadPro(className = "com.xhh.reflection.Dog", methodName = "dogMethod")
public class LoadProTest {
public static void main(String[] args) throws Exception {
Class<LoadProTest> lpcls = LoadProTest.class;
LoadPro anno = lpcls.getAnnotation(LoadPro.class);
String className = anno.className();
String methodName = anno.methodName();
Class<?> cls = Class.forName(className);
Object obj = cls.newInstance();
Method method = cls.getMethod(methodName);
method.invoke(obj);
}
}
|