springboot 单元测试
? ? ? ? ? ? ? ? ? ? ? ? ??
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?
*********************
相关类与接口
? ? ? ? ? ? ? ? ? ? ?
MockMvcBuilders
public final class MockMvcBuilders {
private MockMvcBuilders() {
}
public static DefaultMockMvcBuilder webAppContextSetup(WebApplicationContext context) {
return new DefaultMockMvcBuilder(context);
}
public static StandaloneMockMvcBuilder standaloneSetup(Object... controllers) {
return new StandaloneMockMvcBuilder(controllers);
}
}
? ? ? ? ? ? ? ? ? ? ? ? ?
DefaultMockMvcBuilder
public class DefaultMockMvcBuilder extends AbstractMockMvcBuilder<DefaultMockMvcBuilder> {
private final WebApplicationContext webAppContext;
protected DefaultMockMvcBuilder(WebApplicationContext webAppContext) {
Assert.notNull(webAppContext, "WebApplicationContext is required");
Assert.notNull(webAppContext.getServletContext(), "WebApplicationContext must have a ServletContext");
this.webAppContext = webAppContext;
}
protected WebApplicationContext initWebAppContext() {
? ? ? ? ? ? ? ? ? ? ? ?
AbstractMockMvcBuilder
public abstract class AbstractMockMvcBuilder<B extends AbstractMockMvcBuilder<B>> extends MockMvcBuilderSupport implements ConfigurableMockMvcBuilder<B> {
private List<Filter> filters = new ArrayList();
@Nullable
private RequestBuilder defaultRequestBuilder;
@Nullable
private Charset defaultResponseCharacterEncoding;
private final List<ResultMatcher> globalResultMatchers = new ArrayList();
private final List<ResultHandler> globalResultHandlers = new ArrayList();
private final List<DispatcherServletCustomizer> dispatcherServletCustomizers = new ArrayList();
private final List<MockMvcConfigurer> configurers = new ArrayList(4);
public AbstractMockMvcBuilder() {
}
public final <T extends B> T addFilters(Filter... filters) {
public final <T extends B> T addFilter(Filter filter, String... urlPatterns) {
public final <T extends B> T defaultRequest(RequestBuilder requestBuilder) {
public final <T extends B> T defaultResponseCharacterEncoding(Charset defaultResponseCharacterEncoding) {
public final <T extends B> T alwaysExpect(ResultMatcher resultMatcher) {
public final <T extends B> T alwaysDo(ResultHandler resultHandler) {
public final <T extends B> T addDispatcherServletCustomizer(DispatcherServletCustomizer customizer) {
public final <T extends B> T dispatchOptions(boolean dispatchOptions) {
public final <T extends B> T apply(MockMvcConfigurer configurer) {
public final MockMvc build() {
protected <T extends B> T self() {
protected abstract WebApplicationContext initWebAppContext();
? ? ? ? ? ? ? ? ? ? ? ? ?
MockMvcBuilderSupport
public abstract class MockMvcBuilderSupport {
public MockMvcBuilderSupport() {
}
protected final MockMvc createMockMvc(Filter[] filters, MockServletConfig servletConfig, WebApplicationContext webAppContext, @Nullable RequestBuilder defaultRequestBuilder, @Nullable Charset defaultResponseCharacterEncoding, List<ResultMatcher> globalResultMatchers, List<ResultHandler> globalResultHandlers, @Nullable List<DispatcherServletCustomizer> dispatcherServletCustomizers) {
protected final MockMvc createMockMvc(Filter[] filters, MockServletConfig servletConfig, WebApplicationContext webAppContext, @Nullable RequestBuilder defaultRequestBuilder, List<ResultMatcher> globalResultMatchers, List<ResultHandler> globalResultHandlers, @Nullable List<DispatcherServletCustomizer> dispatcherServletCustomizers) {
************
静态内部类:MockMvcBuildException
private static class MockMvcBuildException extends NestedRuntimeException {
public MockMvcBuildException(String msg, Throwable cause) {
super(msg, cause);
}
}
}
? ? ? ? ? ? ? ? ? ? ? ? ? ? ??
ConfigurableMockMvcBuilder
public interface ConfigurableMockMvcBuilder<B extends ConfigurableMockMvcBuilder<B>> extends MockMvcBuilder {
<T extends B> T addFilters(Filter... filters);
<T extends B> T addFilter(Filter filter, String... urlPatterns);
<T extends B> T defaultRequest(RequestBuilder requestBuilder);
default <T extends B> T defaultResponseCharacterEncoding(Charset defaultResponseCharacterEncoding) {
throw new UnsupportedOperationException("defaultResponseCharacterEncoding is not supported by this MockMvcBuilder");
}
<T extends B> T alwaysExpect(ResultMatcher resultMatcher);
<T extends B> T alwaysDo(ResultHandler resultHandler);
<T extends B> T dispatchOptions(boolean dispatchOptions);
<T extends B> T addDispatcherServletCustomizer(DispatcherServletCustomizer customizer);
<T extends B> T apply(MockMvcConfigurer configurer);
}
? ? ? ? ? ? ? ? ? ? ? ? ?
MockMvcBuilder
public interface MockMvcBuilder {
MockMvc build();
}
? ? ? ? ? ? ? ? ? ? ? ? ? ? ??
MockMvc
public final class MockMvc {
static final String MVC_RESULT_ATTRIBUTE = MockMvc.class.getName().concat(".MVC_RESULT_ATTRIBUTE");
private final TestDispatcherServlet servlet;
private final Filter[] filters;
private final ServletContext servletContext;
@Nullable
private RequestBuilder defaultRequestBuilder;
@Nullable
private Charset defaultResponseCharacterEncoding;
private List<ResultMatcher> defaultResultMatchers = new ArrayList();
private List<ResultHandler> defaultResultHandlers = new ArrayList();
MockMvc(TestDispatcherServlet servlet, Filter... filters) {
public DispatcherServlet getDispatcherServlet() {
public ResultActions perform(RequestBuilder requestBuilder) throws Exception {
void setDefaultRequest(@Nullable RequestBuilder requestBuilder) {
void setDefaultResponseCharacterEncoding(@Nullable Charset defaultResponseCharacterEncoding) {
void setGlobalResultMatchers(List<ResultMatcher> resultMatchers) {
void setGlobalResultHandlers(List<ResultHandler> resultHandlers) {
private MockHttpServletResponse unwrapResponseIfNecessary(ServletResponse servletResponse) {
private void applyDefaultResultActions(MvcResult mvcResult) throws Exception {
? ? ? ? ? ? ? ? ? ? ? ? ??
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ??
MockMvcRequestBuilders
public abstract class MockMvcRequestBuilders {
public MockMvcRequestBuilders() {
}
public static MockHttpServletRequestBuilder get(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder get(URI uri) {
public static MockHttpServletRequestBuilder post(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder post(URI uri) {
public static MockHttpServletRequestBuilder put(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder put(URI uri) {
public static MockHttpServletRequestBuilder patch(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder patch(URI uri) {
public static MockHttpServletRequestBuilder delete(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder delete(URI uri) {
public static MockHttpServletRequestBuilder options(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder options(URI uri) {
public static MockHttpServletRequestBuilder head(String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder head(URI uri) {
public static MockHttpServletRequestBuilder request(HttpMethod method, String urlTemplate, Object... uriVars) {
public static MockHttpServletRequestBuilder request(HttpMethod httpMethod, URI uri) {
public static MockHttpServletRequestBuilder request(String httpMethod, URI uri) {
public static MockMultipartHttpServletRequestBuilder multipart(String urlTemplate, Object... uriVars) {
public static MockMultipartHttpServletRequestBuilder multipart(URI uri) {
public static RequestBuilder asyncDispatch(MvcResult mvcResult) {
? ? ? ? ? ? ? ? ? ? ? ? ? ? ??
MockHttpServletRequestBuilder
public class MockHttpServletRequestBuilder implements ConfigurableSmartRequestBuilder<MockHttpServletRequestBuilder>, Mergeable {
private final String method;
private final URI url;
private String contextPath;
private String servletPath;
@Nullable
private String pathInfo;
@Nullable
private Boolean secure;
@Nullable
private Principal principal;
@Nullable
private MockHttpSession session;
@Nullable
private String characterEncoding;
@Nullable
private byte[] content;
@Nullable
private String contentType;
private final MultiValueMap<String, Object> headers;
private final MultiValueMap<String, String> parameters;
private final MultiValueMap<String, String> queryParams;
private final List<Cookie> cookies;
private final List<Locale> locales;
private final Map<String, Object> requestAttributes;
private final Map<String, Object> sessionAttributes;
private final Map<String, Object> flashAttributes;
private final List<RequestPostProcessor> postProcessors;
MockHttpServletRequestBuilder(HttpMethod httpMethod, String url, Object... vars) {
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?
MockHttpServletRequest
public class MockHttpServletRequest implements HttpServletRequest {
private static final String HTTP = "http";
private static final String HTTPS = "https";
private static final String CHARSET_PREFIX = "charset=";
private static final TimeZone GMT = TimeZone.getTimeZone("GMT");
private static final ServletInputStream EMPTY_SERVLET_INPUT_STREAM = new DelegatingServletInputStream(StreamUtils.emptyInput());
private static final BufferedReader EMPTY_BUFFERED_READER = new BufferedReader(new StringReader(""));
private static final String[] DATE_FORMATS = new String[]{"EEE, dd MMM yyyy HH:mm:ss zzz", "EEE, dd-MMM-yy HH:mm:ss zzz", "EEE MMM dd HH:mm:ss yyyy"};
public static final String DEFAULT_PROTOCOL = "HTTP/1.1";
public static final String DEFAULT_SCHEME = "http";
public static final String DEFAULT_SERVER_ADDR = "127.0.0.1";
public static final String DEFAULT_SERVER_NAME = "localhost";
public static final int DEFAULT_SERVER_PORT = 80;
public static final String DEFAULT_REMOTE_ADDR = "127.0.0.1";
public static final String DEFAULT_REMOTE_HOST = "localhost";
private final ServletContext servletContext;
private boolean active;
private final Map<String, Object> attributes;
@Nullable
private String characterEncoding;
@Nullable
private byte[] content;
@Nullable
private String contentType;
@Nullable
private ServletInputStream inputStream;
@Nullable
private BufferedReader reader;
private final Map<String, String[]> parameters;
private String protocol;
private String scheme;
private String serverName;
private int serverPort;
private String remoteAddr;
private String remoteHost;
private final LinkedList<Locale> locales;
private boolean secure;
private int remotePort;
private String localName;
private String localAddr;
private int localPort;
private boolean asyncStarted;
private boolean asyncSupported;
@Nullable
private MockAsyncContext asyncContext;
private DispatcherType dispatcherType;
@Nullable
private String authType;
@Nullable
private Cookie[] cookies;
private final Map<String, HeaderValueHolder> headers;
@Nullable
private String method;
@Nullable
private String pathInfo;
private String contextPath;
@Nullable
private String queryString;
@Nullable
private String remoteUser;
private final Set<String> userRoles;
@Nullable
private Principal userPrincipal;
@Nullable
private String requestedSessionId;
@Nullable
private String requestURI;
private String servletPath;
@Nullable
private HttpSession session;
private boolean requestedSessionIdValid;
private boolean requestedSessionIdFromCookie;
private boolean requestedSessionIdFromURL;
private final MultiValueMap<String, Part> parts;
public MockHttpServletRequest() {
public MockHttpServletRequest(@Nullable String method, @Nullable String requestURI) {
public MockHttpServletRequest(@Nullable ServletContext servletContext) {
public MockHttpServletRequest(@Nullable ServletContext servletContext, @Nullable String method, @Nullable String requestURI) {
? ? ? ? ? ? ? ? ? ? ??
MockHttpSession
public class MockHttpSession implements HttpSession {
public static final String SESSION_COOKIE_NAME = "JSESSION";
private static int nextId = 1;
private String id;
private final long creationTime;
private int maxInactiveInterval;
private long lastAccessedTime;
private final ServletContext servletContext;
private final Map<String, Object> attributes;
private boolean invalid;
private boolean isNew;
public MockHttpSession() {
public MockHttpSession(@Nullable ServletContext servletContext) {
public MockHttpSession(@Nullable ServletContext servletContext, @Nullable String id) {
? ? ? ? ? ? ? ? ? ? ? ? ? ? ??
? ? ? ? ? ? ? ? ? ? ? ??
ResultActions
public interface ResultActions {
ResultActions andExpect(ResultMatcher matcher) throws Exception;
default ResultActions andExpectAll(ResultMatcher... matchers) throws Exception {
ResultActions andDo(ResultHandler handler) throws Exception;
MvcResult andReturn();
}
? ? ? ? ? ? ? ? ? ? ??
ResultMatcher
@FunctionalInterface
public interface ResultMatcher {
void match(MvcResult result) throws Exception;
}
? ? ? ? ? ? ? ? ? ? ?
MvcResult
public interface MvcResult {
MockHttpServletRequest getRequest();
MockHttpServletResponse getResponse();
@Nullable
Object getHandler();
@Nullable
HandlerInterceptor[] getInterceptors();
@Nullable
ModelAndView getModelAndView();
@Nullable
Exception getResolvedException();
FlashMap getFlashMap();
Object getAsyncResult();
Object getAsyncResult(long timeToWait);
}
? ? ? ? ? ? ? ? ? ? ? ?
ResultHandler
@FunctionalInterface
public interface ResultHandler {
void handle(MvcResult result) throws Exception;
}
? ? ? ? ? ? ??
MockMvcResultMatchers
public abstract class MockMvcResultMatchers {
private static final AntPathMatcher pathMatcher = new AntPathMatcher();
public MockMvcResultMatchers() {
}
public static RequestResultMatchers request() {
public static HandlerResultMatchers handler() {
public static StatusResultMatchers status() {
public static HeaderResultMatchers header() {
public static ContentResultMatchers content() {
public static ModelResultMatchers model() {
public static ViewResultMatchers view() {
public static FlashAttributeResultMatchers flash() {
public static ResultMatcher forwardedUrl(@Nullable String expectedUrl) {
public static ResultMatcher forwardedUrlTemplate(String urlTemplate, Object... uriVars) {
public static ResultMatcher forwardedUrlPattern(String urlPattern) {
public static ResultMatcher redirectedUrl(String expectedUrl) {
public static ResultMatcher redirectedUrlTemplate(String urlTemplate, Object... uriVars) {
public static ResultMatcher redirectedUrlPattern(String urlPattern) {
public static JsonPathResultMatchers jsonPath(String expression, Object... args) {
public static <T> ResultMatcher jsonPath(String expression, Matcher<? super T> matcher) {
public static <T> ResultMatcher jsonPath(String expression, Matcher<? super T> matcher, Class<T> targetType) {
public static XpathResultMatchers xpath(String expression, Object... args) throws XPathExpressionException {
public static XpathResultMatchers xpath(String expression, Map<String, String> namespaces, Object... args) throws XPathExpressionException {
public static CookieResultMatchers cookie() {
? ? ? ? ? ? ? ? ? ? ? ??
? ? ? ? ? ? ? ? ? ? ? ? ? ?
*********************
示例
? ? ? ? ? ?
***************
pojo 层
? ? ? ? ? ? ??
Person
@Data
public class Person {
private String name;
private Integer age;
}
? ? ? ? ? ? ? ? ? ? ? ? ? ??
***************
service 层
? ? ? ? ? ?
HelloService
public interface HelloService {
Person getPerson();
}
? ? ? ? ? ? ? ? ? ?
***************
service.impl 层
? ? ? ? ? ?
HelloServiceImpl
@Service
public class HelloServiceImpl implements HelloService {
@Override
public Person getPerson() {
Person person = new Person();
person.setName("瓜田李下");
person.setAge(20);
return person;
}
}
? ? ? ? ? ? ? ? ?
***************
controller 层
? ? ? ? ? ?
HelloController
@RestController
public class HelloController {
@Resource
private HelloService helloService;
@RequestMapping(value = "/hello",produces = "application/json;charset=utf-8")
public Person hello(){
Person person= helloService.getPerson();
System.out.println(person);
return person;
}
@RequestMapping("/get")
public Map<String,Object> get(HttpSession session){
Enumeration<String> names=session.getAttributeNames();
while (names.hasMoreElements()){
String name= names.nextElement();
System.out.println(name+" "+session.getAttribute(name));
}
Map<String,Object> result=new HashMap<>();
result.put("status","success");
result.put("name","瓜田李下");
result.put("age",20);
return result;
}
@RequestMapping("/get2")
public String get2(String name, Integer age){
System.out.println(name+" "+age);
return "success";
}
@RequestMapping("/get3")
public String get3(String name, Integer age){
System.out.println(name+" "+age);
return "success";
}
@RequestMapping("/get4/{name}/{age}")
public String get4(@PathVariable("name") String name,
@PathVariable("age") Integer age){
System.out.println(name+" "+age);
return "success";
}
@RequestMapping("/get5")
public String get5(@RequestBody Person person){
System.out.println(person);
return "success";
}
}
? ? ? ? ? ? ? ? ? ? ? ?
***************
生成测试类
? ? ? ? ? ??
ll类所在页面右键 ==> go to ==> test
? ? ? ? ? ? ? ? ? ? ? ? ? ? ?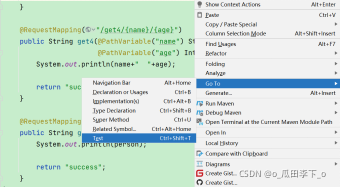
? ? ? ? ? ? ? ? ? ? ? ? ? ? ?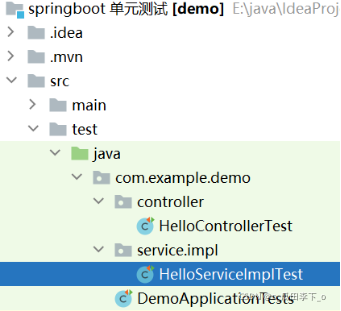
? ? ? ? ? ? ? ? ? ? ? ?
HelloServiceImplTest
@SpringBootTest
class HelloServiceImplTest {
@Resource
private HelloService helloService;
@Test
void getPerson() {
System.out.println(helloService.getPerson());
}
}
? ? ? ? ? ? ? ? ? ? ?
HelloControllerTest
@SpringBootTest
class HelloControllerTest {
@Resource
private WebApplicationContext applicationContext;
private MockMvc mockMvc;
private MockHttpSession session;
@BeforeEach
public void before(){
mockMvc = MockMvcBuilders.webAppContextSetup(applicationContext).build();
session = new MockHttpSession();
Person person=new Person();
person.setName("瓜田李下");
person.setAge(20);
session.setAttribute("person",person);
}
@Test
void hello() throws Exception {
mockMvc.perform(MockMvcRequestBuilders.get("/hello"))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(result -> System.out.println(result.getResponse()))
.andDo(MockMvcResultHandlers.print());
}
@Test
void get() throws Exception{
mockMvc.perform(MockMvcRequestBuilders.get("/get")
.session(session))
.andExpect(MockMvcResultMatchers.status().isOk())
.andExpect(MockMvcResultMatchers.jsonPath("$.status").value("success"))
.andExpect(MockMvcResultMatchers.jsonPath("$.status").exists())
.andExpect(MockMvcResultMatchers.jsonPath("$.name").value("瓜田李下"))
.andExpect(MockMvcResultMatchers.jsonPath("$.age").value(20))
.andDo(result -> System.out.println("content: "+result.getResponse().getContentAsString()));
}
@Test
void get2() throws Exception{
mockMvc.perform(MockMvcRequestBuilders.get("/get2")
.param("name","瓜田李下")
.param("age","20"))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(result -> System.out.println("content: "+result.getResponse().getContentAsString()));
}
@Test
void get3() throws Exception{
MultiValueMap<String,String> map=new LinkedMultiValueMap<>();
map.add("name","瓜田李下");
map.add("age","20");
mockMvc.perform(MockMvcRequestBuilders.post("/get3")
.params(map))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(result -> System.out.println("content: "+result.getResponse().getContentAsString()));
}
@Test
void get4() throws Exception{
mockMvc.perform(MockMvcRequestBuilders.get("/get4/瓜田李下/20")
.characterEncoding("utf-8"))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(result -> System.out.println("content:"+result.getResponse().getContentAsString()));
}
@Test
void get5() throws Exception{
Person person=new Person();
person.setName("瓜田李下");
person.setAge(20);
mockMvc.perform(MockMvcRequestBuilders.get("/get5")
.content(JSON.toJSONBytes(person))
.contentType("application/json"))
.andExpect(MockMvcResultMatchers.status().isOk())
.andDo(result -> System.out.println("content:"+result.getResponse().getContentAsString()));
}
}
? ? ? ? ? ? ? ? ??
? ? ? ? ? ? ? ? ? ? ? ??
|