单元测试方法(IDEA)
1.创建和src同级的test文件夹 2.ctrl+shift+t创建对应的测试类 3.用generate声明test方法,权限是public并且没有返回值,没有形参 4.test方法上面一定有一个@test注解。 5.run就完事了
包装类的使用(Wrapper)
- 包装类又称封装类。
- 包装类的目的是把基本数据类型封装成类,这样可以把基本数据类型当做对象来调用,这样面向对象的思想才在JAVA里得到了统一,JAVA才是真正的面向对象。
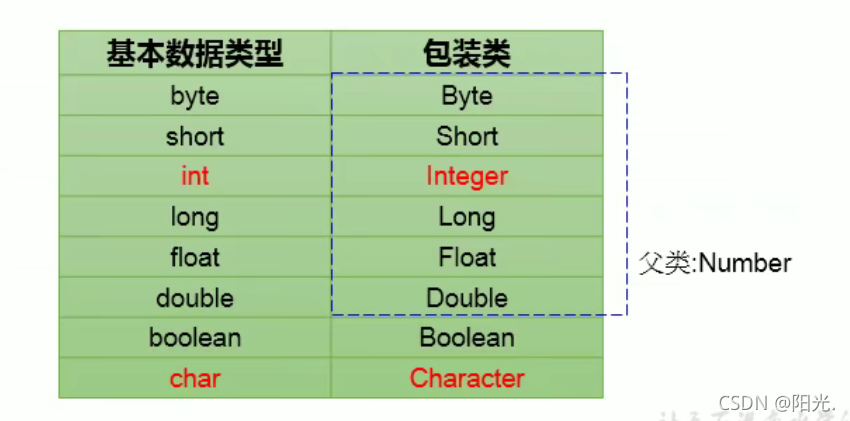 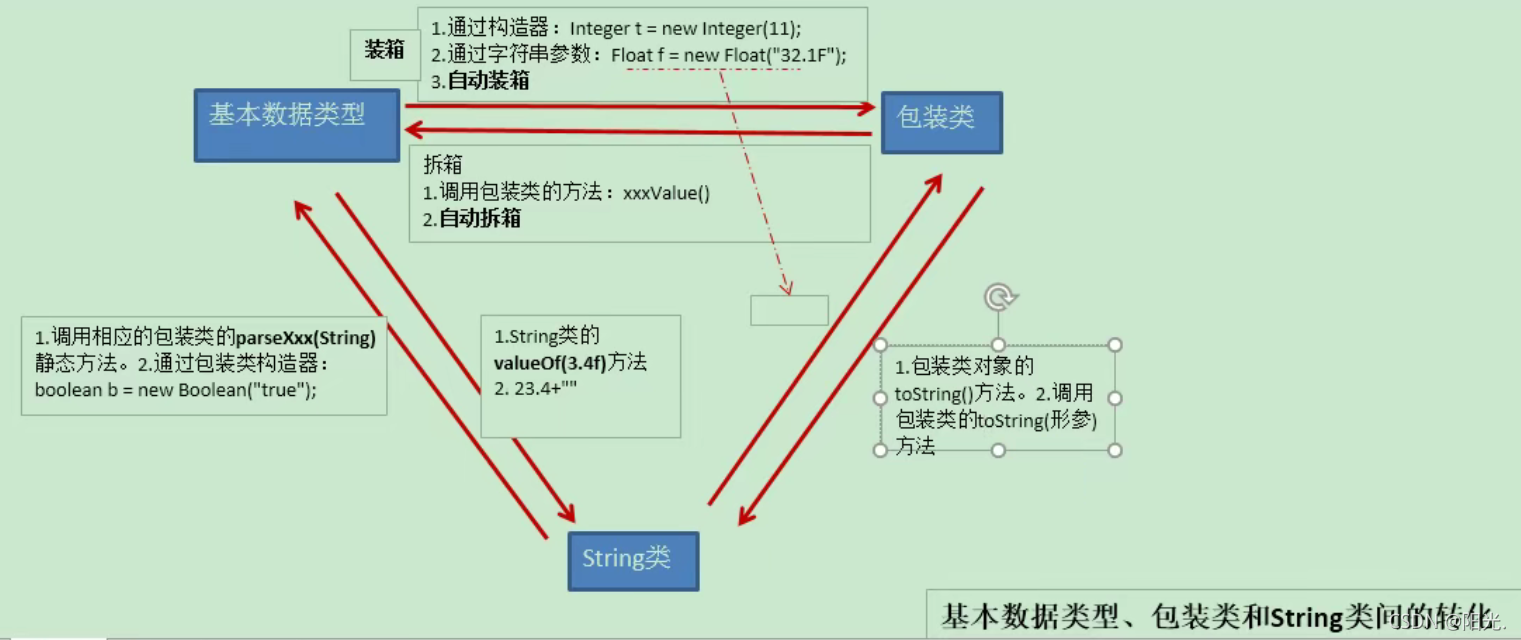 包装类的使用:
- java提供了8种基本数据类型对应的包装类,使得基本数据类型的变量具有类的特征
- 需要掌握:基本数据类型、包装类、String三者之间的相互转换。
- JDK5.0新特性:自动装箱与自动拆箱。有了这个,基本数据类型和包装类的相互转换不再是问题,我们只需要关心基本数据类型、包装类如何转换成String类型就可以了。
自动装箱与自动拆箱 自动装箱:
int num2 = 10;
Integer in1 = num2;
这是合法的。有了自动拆箱,我们把基本数据类型转化为包装类,就不用new了。
自动拆箱:
int num3=in1;
直接把包装类赋给一个基本数据类型。
包装类面试题
public void test1() {
Object o1 = true ? new Integer(1) : new Double(2.0);
System.out.println(o1);
}
输出结果为1.0,因为编译时,要求new Integer(1) : new Double(2.0) 的类型必须一致,所以会转化为double类型。
public void test2() {
Object o2;
if (true)
o2 = new Integer(1);
else
o2 = new Double(2.0);
System.out.println(o2);
}
输出1。
public void test3() {
Integer i = new Integer(1);
Integer j = new Integer(1);
System.out.println(i == j);
Integer m = 1;
Integer n = 1;
System.out.println(m == n);
Integer x = 128;
Integer y = 128;
System.out.println(x == y);
}
输出:
false
true
false
解释: 三者都是比较地址,第二个为true的原因是Integer里定义了IntegerCache结构,IntegerCache中定义了Integer格式的cache数组,长度为-128-127,如果自动装箱时在这个范围内,就不额外new了,否则就要额外new。
|