GlidedSky 爬虫-基础1 使用selenium练手
1、题目描述
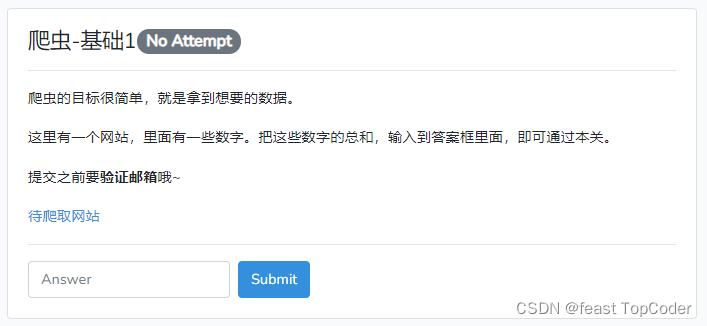
2、解题思路与代码实现
2.1、用selenium模拟登录(输入账号密码并点击登录按钮)
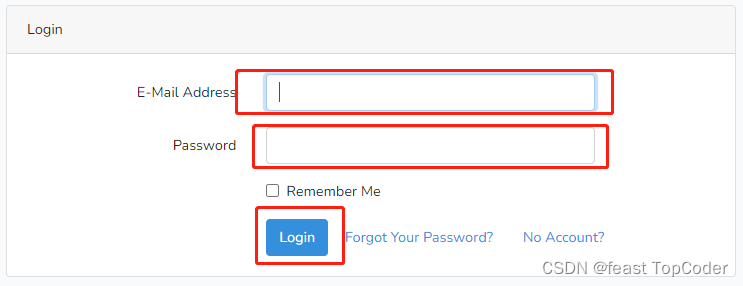
import json
import time
from selenium import webdriver
# 加载驱动
driver = webdriver.Chrome(executable_path='./chromedriver.exe')
# 跳转到目标网址
driver.get("http://www.glidedsky.com/")
time.sleep(1)
# 输入邮箱地址(用户名)
email_address = driver.find_elements_by_xpath('//*[@id="email"]')[0]
email_address.send_keys("你的邮箱")
time.sleep(1)
# 输入密码
password = driver.find_elements_by_xpath('//*[@id="password"]')[0]
password.send_keys("你的密码")
time.sleep(1)
# 点击登录按钮
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/div/div[2]/form/div[4]/div/button')[0].click()
2.2、点击爬虫-基础1进入题目详情
# 点击 爬虫-基础1 进入题目详情
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/table/tbody/tr/td[1]/a')[0].click()
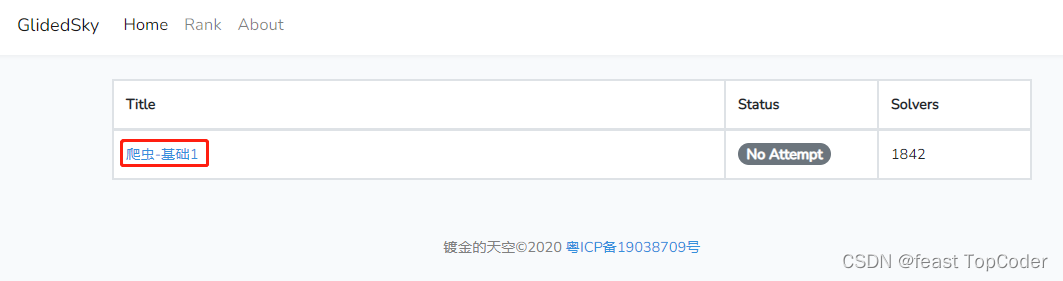
2.3、点击待爬取网站进入待爬取内容的网页。
# 点击待爬取网站进入待爬取内容的网页。
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/div/div/a')[0].click()
time.sleep(1)
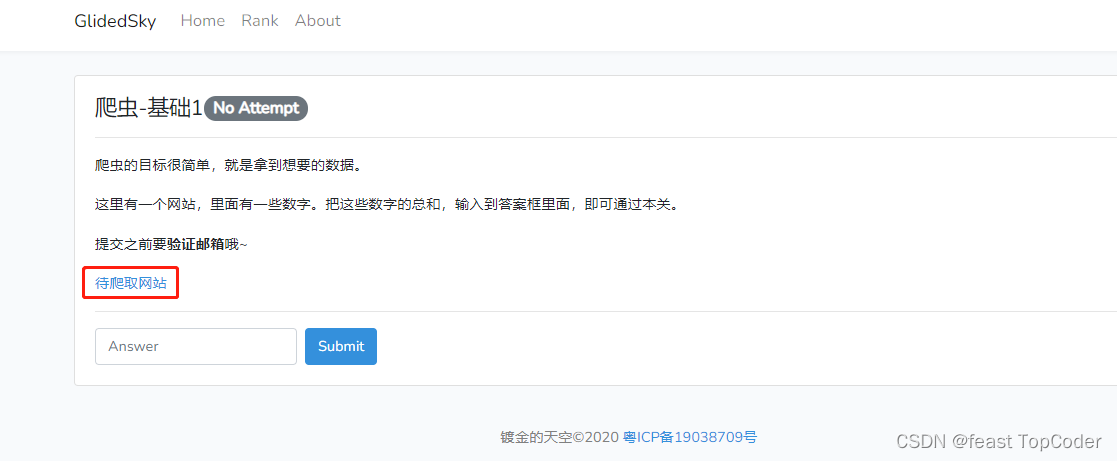
2.4、获取本文本框中所有的数据并相加。
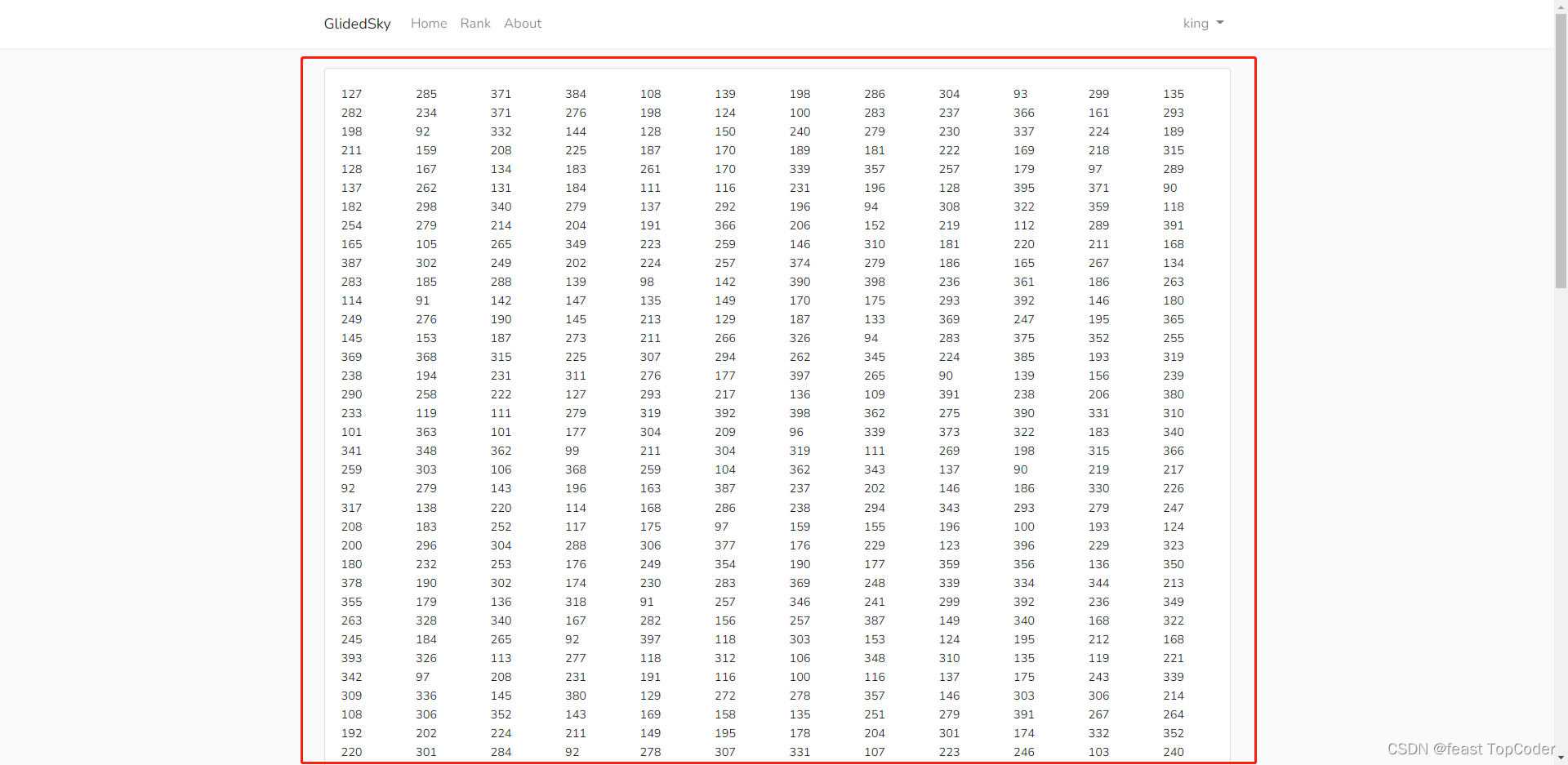
driver.switch_to.window(driver.window_handles[1])
time.sleep(1)
text_num = driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div')[0].text
list_num = text_num.split('\n')
total = 0
for i in range(len(list_num)):
per = int(list_num[i])
total = total + per
2.5、关闭当前网页,返回待输入结果的上一个页面。
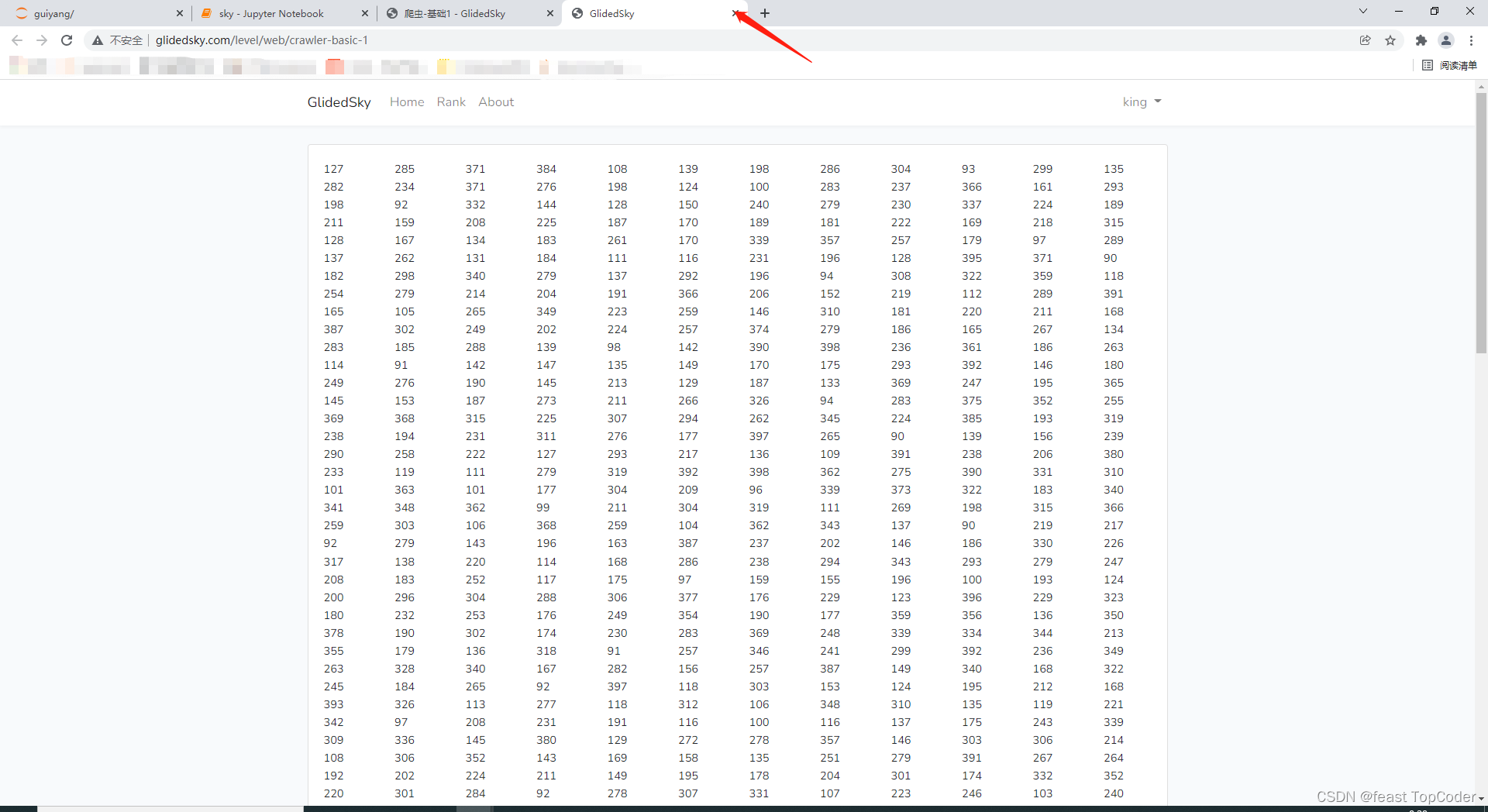
# 关闭当前网页,返回待输入结果的上一个页面。
driver.close()
driver.switch_to.window(driver.window_handles[0])
2.6、输入答案,点击提交。
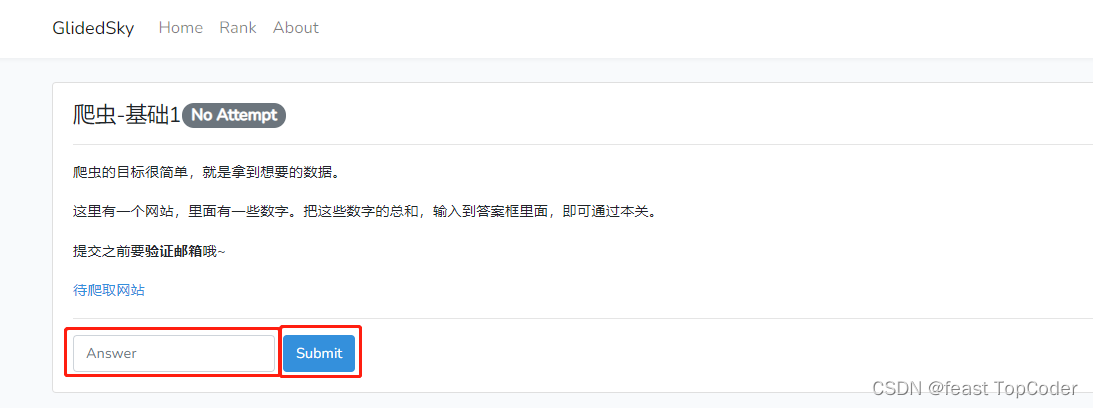
# 输入答案,点击提交。
answer = driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/form/div/input[1]')[0]
answer.send_keys(total)
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/form/button')[0].click()
3、难点解析
上面的代码中,所有需要点击的地方我都是用xpath来定位的,而且获取的方法也很暴力,比如一开始输入邮箱的那个文本框是怎么定位的?按照下面这个操作去获取即可: ①首先打开开发者模式: 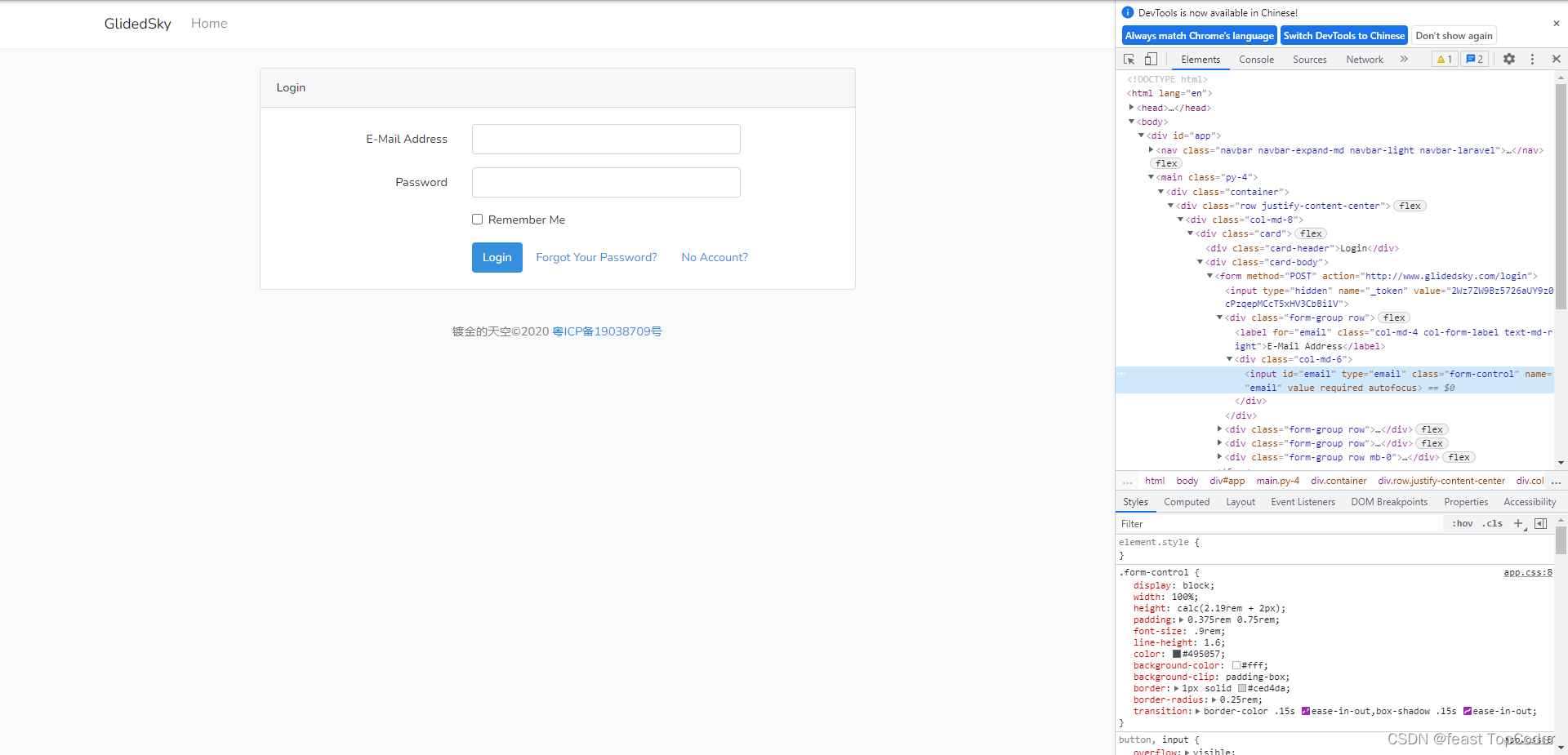 ②然后在输入邮箱地址那个文本框那右键,选择检查,然后开发者模式那边会自动定义到那个代码。 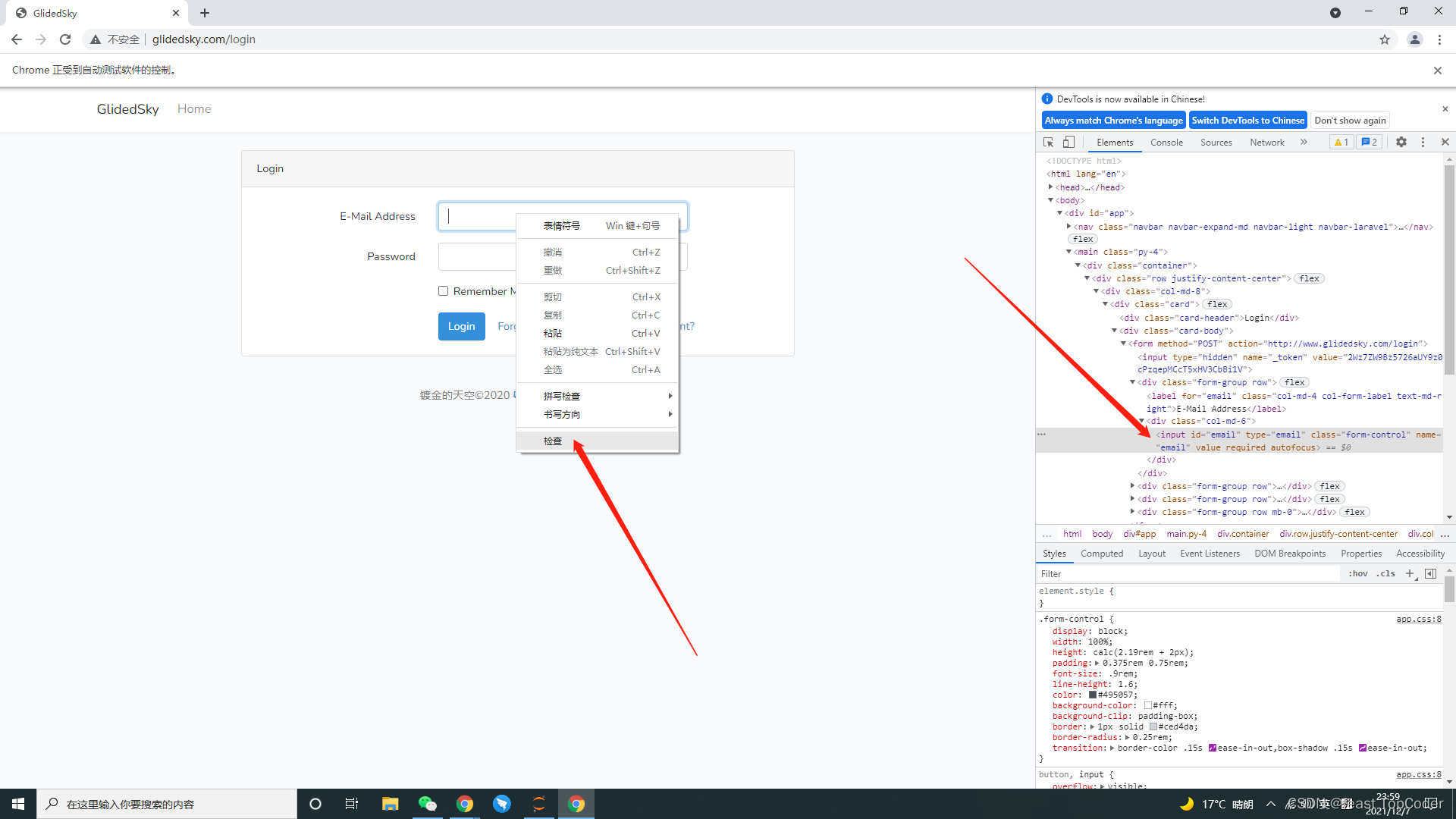 ③然后右键,选择copy,copy path,即可获取到其xpath://*[@id=“email”]
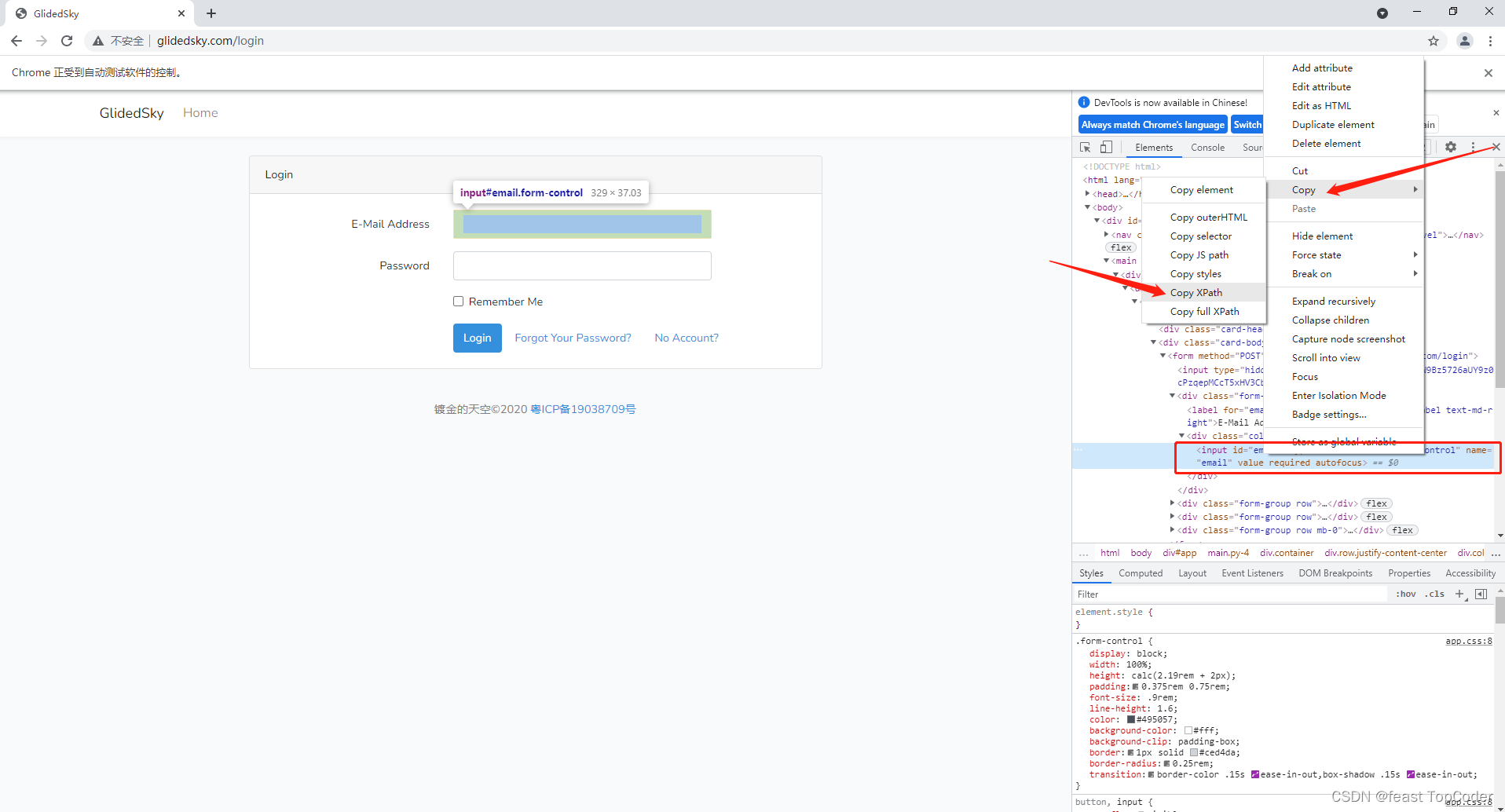
4、完整代码
只需要填写上自己的Glidesky的账号密码即可。所有的步骤都写在一个函数里面了。
import json
import time
from selenium import webdriver
def Round_one(user,pwd):
driver = webdriver.Chrome(executable_path='./chromedriver.exe')
driver.get("http://www.glidedsky.com/")
time.sleep(3)
email_address = driver.find_elements_by_xpath('//*[@id="email"]')[0]
email_address.send_keys(user)
time.sleep(1)
password = driver.find_elements_by_xpath('//*[@id="password"]')[0]
password.send_keys(pwd)
time.sleep(1)
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/div/div[2]/form/div[4]/div/button')[0].click()
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/table/tbody/tr/td[1]/a')[0].click()
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/div/div/a')[0].click()
time.sleep(1)
driver.switch_to.window(driver.window_handles[1])
time.sleep(1)
text_num = driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div')[0].text
list_num = text_num.split('\n')
total = 0
for i in range(len(list_num)):
per = int(list_num[i])
total = total + per
driver.close()
driver.switch_to.window(driver.window_handles[0])
answer = driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/form/div/input[1]')[0]
answer.send_keys(total)
driver.find_elements_by_xpath('//*[@id="app"]/main/div[1]/div/div/form/button')[0].click()
user = "你的邮箱地址"
pwd = "你的密码"
Round_one(user,pwd)
觉得有帮助的朋友,可以一键三连再走哦。
|