1.创建一个JSONHelper类。
public class JSONHelper
{
/// <summary>
/// 实体对象转换成JSON字符串
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="x"></param>
/// <returns></returns>
public static string EntityToJSON<T>(T x)
{
string result = string.Empty;
try
{
result = JsonConvert.SerializeObject(x);
}
catch (Exception)
{
result = string.Empty;
}
return result;
}
/// <summary>
/// JSON字符串转换成实体类
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="json"></param>
/// <returns></returns>
public static T JSONToEntity<T>(string json)
{
T t = default(T);
try
{
t = (T)JsonConvert.DeserializeObject(json, typeof(T));
}
catch (Exception)
{
t = default(T);
}
return t;
}
}
2.创建实体类用于保存数据
public class Student
{
public int StudentId { get; set; }
public string StudentName { get; set; }
}
3.创建winform 通过集合条用Model 和 JSONHelper类
private void btn_Save_Click(object sender, EventArgs e)
{
List<Student> stuList = new List<Student>()
{
new Student(){StudentId=1,StudentName="小明"},
new Student(){StudentId=2,StudentName="小红"},
new Student(){StudentId=3,StudentName="小丽"}
};
if (SaveConfig(JSONHelper.EntityToJSON(stuList), Application.StartupPath+"\\1.txt"))
{
MessageBox.Show("导出配置文件成功!", "导出配置");
}
else
{
MessageBox.Show("导出配置文件失败!", "导出配置");
}
}
private bool SaveConfig(string info, string path)
{
FileStream fs;
StreamWriter sw;
try
{
//保存协议信息
fs = new FileStream(path, FileMode.Create);
sw = new StreamWriter(fs, Encoding.Default);
sw.WriteLine(info);
sw.Close();
fs.Close();
}
catch (Exception)
{
return false;
}
return true;
}
private List<Student> ConfigList = new List<Student>();
private void btn_Read_Click(object sender, EventArgs e)
{
using (OpenFileDialog fileDialog = new OpenFileDialog())
{
fileDialog.Multiselect = false;
fileDialog.Filter = "Text文件|*.txt";
if (fileDialog.ShowDialog() == DialogResult.OK)
{
ConfigList = GetConfigInfo(fileDialog.FileName);
dataGridView1.DataSource = null;
dataGridView1.DataSource = ConfigList;
}
}
}
public List<Student> GetConfigInfo(string filename)
{
FileStream fs = new FileStream(filename, FileMode.Open);
StreamReader sr = new StreamReader(fs, Encoding.Default);
string str = sr.ReadToEnd();
sr.Close();
fs.Close();
return JSONHelper.JSONToEntity<List<Student>>(str);
}
4.结果
读取.
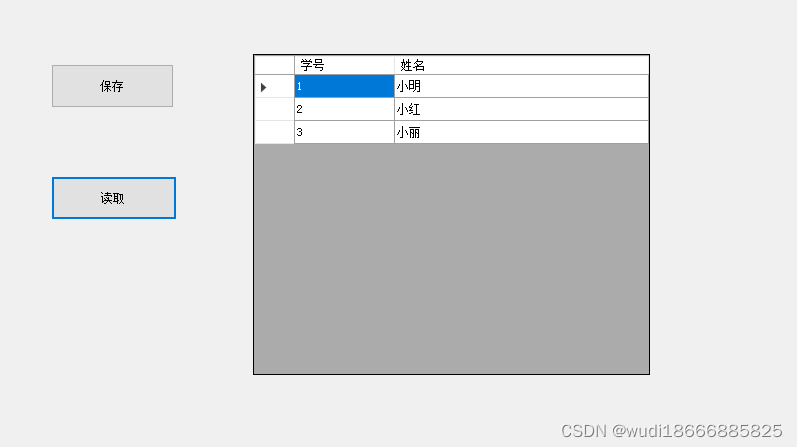
保存。
?
?注意:写入是把类以集合的形式序列化,读取是把字符串反序列化成为类。
|