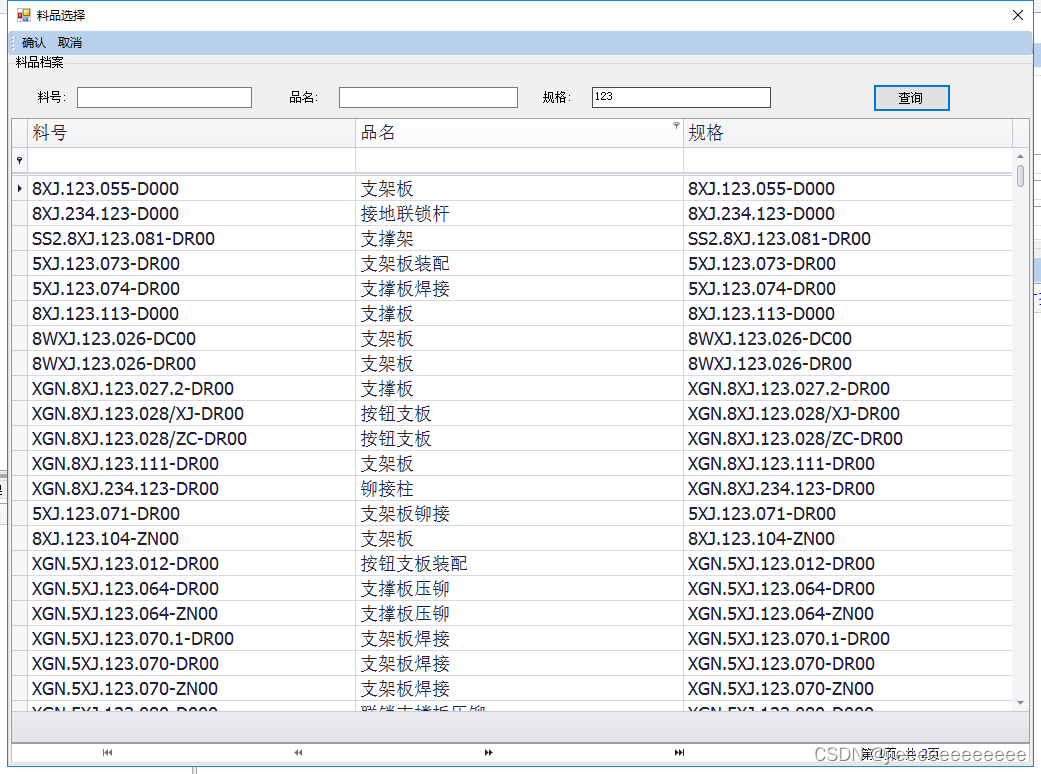
? /// <summary> ? ? ? ? /// 查询方法 ? ? ? ? /// </summary> ? ? ? ? /// <param name="sender"></param> ? ? ? ? /// <param name="e"></param> ? ? ? ? private void btnSearch_Click(object sender, EventArgs e) ? ? ? ? { ? ? ? ? ? ? WaitDialogForm wdf = new WaitDialogForm("提示", "正在从服务器读取数据。。。"); ? ? ? ? ? ? try ? ? ? ? ? ? { ? ? ? ? ? ? ? ? _root.type = "GetRoutingList"; ? ? ? ? ? ? ? ? _root.paramList = new List<ParamDTOData>(); ? ? ? ? ? ? ? ? ParamDTOData pa = new ParamDTOData(); ? ? ? ? ? ? ? ? pa.DocNo = GetWhereSql(); ? ? ? ? ? ? ? ? pa.Prv1 = pageIndex + ""; ? ? ? ? ? ? ? ? pa.Prv2 = pagesize + "";
? ? ? ? ? ? ? ? _root.paramList.Add(pa);
? ? ? ? ? ? ? ? wdf.SetCaption(string.Format("加载数据中..."));
? ? ? ? ? ? ? ? var jsonSetting = new JsonSerializerSettings { NullValueHandling = NullValueHandling.Ignore }; ? ? ? ? ? ? ? ? string json = JsonConvert.SerializeObject(_root, Formatting.None, jsonSetting); ? ? ? ? ? ? ? ? string result = PostPara.HttpPost(_loginContext.WebServiceISV, json);
? ? ? ? ? ? ? ? wdf.Close();
? ? ? ? ? ? ? ? ResultJson.Root root = PostPara.FromJSON<ResultJson.Root>(result); ? ? ? ? ? ? ? ? if (root == null) ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? XtraMessageBox.Show("服务器返回数据无效,请检查服务器接口是否正常!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Error); ? ? ? ? ? ? ? ? ? ? return; ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? ResultJson.RootD rootd = PostPara.FromJSON<ResultJson.RootD>(root.d);
? ? ? ? ? ? ? ? if (rootd != null && rootd.state != null) ? ? ? ? ? ? ? ? {
? ? ? ? ? ? ? ? ? ? if (!string.IsNullOrEmpty(rootd.state) && rootd.state.ToLower() == "success") ? ? ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? ? ? DataSet qc = PostPara.FromJSON<DataSet>(rootd.data); ? ? ? ? ? ? ? ? ? ? ? ? RecordCount = JsonToDataTable.ToInt(rootd.msg, 0); ? ? ? ? ? ? ? ? ? ? ? ? _dtList = qc.Tables[0]; ? ? ? ? ? ? ? ? ? ? ? ? BindPageGridList();
? ? ? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? ? ? else ? ? ? ? ? ? ? ? ? ? {
? ? ? ? ? ? ? ? ? ? ? ? MessageBox.Show(string.Format("查询失败,{0}!", rootd.msg)); ? ? ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? } ? ? ? ? ? ? ? ? else ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? MessageBox.Show("查询失败,服务器没有返回结果!");
? ? ? ? ? ? ? ? }
? ? ? ? ? ? } ? ? ? ? ? ? catch (Exception ex) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? wdf.Close(); ? ? ? ? ? ? ? ? MessageBox.Show(ex.Message); ? ? ? ? ? ? }
? ? ? ? } ?
? /// <summary> ? ? ? ? /// 分页事件处理 ? ? ? ? /// </summary> ? ? ? ? /// <param name="eventString">事件名称</param> ? ? ? ? /// <param name="button">按钮控件</param> ? ? ? ? /// <time>2013-11-5 14:25:59</time> ? ? ? ? void ShowEvent(string eventString, NavigatorButtonBase button) ? ? ? ? { ? ? ? ? ? ? try ? ? ? ? ? ? { ? ? ? ? ? ? ? ? //不使用系统自带的按钮上下页 ? ? ? ? ? ? ? ? string type = button.ButtonType.ToString(); ? ? ? ? ? ? ? ? //DevExpress.XtraEditors.NavigatorButton btn = (DevExpress.XtraEditors.NavigatorButton)button; ? ? ? ? ? ? ? ? //string type = btn.ButtonType.ToString();
? ? ? ? ? ? ? ? //if (type == "First") ? ? ? ? ? ? ? ? //{ ? ? ? ? ? ? ? ? // ? ?pageIndex = 1; ? ? ? ? ? ? ? ? //}
? ? ? ? ? ? ? ? //if (type == "NextPage") ? ? ? ? ? ? ? ? //{ ? ? ? ? ? ? ? ? // ? ?pageIndex++; ? ? ? ? ? ? ? ? //}
? ? ? ? ? ? ? ? //if (type == "Last") ? ? ? ? ? ? ? ? //{ ? ? ? ? ? ? ? ? // ? ?pageIndex = pageCount; ? ? ? ? ? ? ? ? //}
? ? ? ? ? ? ? ? //if (type == "PrevPage") ? ? ? ? ? ? ? ? //{ ? ? ? ? ? ? ? ? // ? ?pageIndex--; ? ? ? ? ? ? ? ? //}
? ? ? ? ? ? ? ? NavigatorCustomButton btn = (NavigatorCustomButton)button; ? ? ? ? ? ? ? ? string type = btn.Tag.ToString(); ? ? ? ? ? ? ? ? if (type == "首页") ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? pageIndex = 1; ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? if (type == "下一页") ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? pageIndex++; ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? if (type == "末页") ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? pageIndex = pageCount; ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? if (type == "上一页") ? ? ? ? ? ? ? ? { ? ? ? ? ? ? ? ? ? ? pageIndex--; ? ? ? ? ? ? ? ? }
? ? ? ? ? ? ? ? //绑定分页控件和GridControl数据 ? ? ? ? ? ? ? ? btnSearch_Click(null, null); ? ? ? ? ? ? ? ? // BindPageGridList(); ? ? ? ? ? ? } ? ? ? ? ? ? catch (Exception e) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? this.Close(); ? ? ? ? ? ? }
? ? ? ? }
? ? ? ? /// <summary> ? ? ? ? /// 绑定分页控件和GridControl数据 ? ? ? ? /// </summary> ? ? ? ? /// <param name="strWhere">查询条件</param> ? ? ? ? public void BindPageGridList() ? ? ? ? { ? ? ? ? ? ? // SystemOperateLog objSOL = new BLL.SystemOperateLog();
? ? ? ? ? ? //nvgtDataPager.Buttons.Last.Enabled = true; ? ? ? ? ? ? //nvgtDataPager.Buttons.NextPage.Enabled = true; ? ? ? ? ? ? //nvgtDataPager.Buttons.PrevPage.Enabled = true; ? ? ? ? ? ? //nvgtDataPager.Buttons.First.Enabled = true; ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[0].Enabled = true; ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[1].Enabled = true; ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[2].Enabled = true; ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[3].Enabled = true; ? ? ? ? ? ? //记录获取开始数 ? ? ? ? ? ? int startIndex = (pageIndex - 1) * pagesize + 1; ? ? ? ? ? ? //结束数 ? ? ? ? ? ? int endIndex = pageIndex * pagesize;
? ? ? ? ? ? //总行数 ? ? ? ? ? ? int row = RecordCount;
? ? ? ? ? ? //获取总页数 ? ? ? ? ? ? ? if (row % pagesize > 0) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? pageCount = row / pagesize + 1; ? ? ? ? ? ? } ? ? ? ? ? ? else ? ? ? ? ? ? { ? ? ? ? ? ? ? ? pageCount = row / pagesize; ? ? ? ? ? ? }
? ? ? ? ? ? if (pageIndex == 1) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? //nvgtDataPager.Buttons.First.Enabled = false; ? ? ? ? ? ? ? ? //nvgtDataPager.Buttons.PrevPage.Enabled = false; ? ? ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[0].Enabled = false; ? ? ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[1].Enabled = false; ;
? ? ? ? ? ? }
? ? ? ? ? ? //最后页时获取真实记录数 ? ? ? ? ? ? if (pageCount == pageIndex) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? //endIndex = row; ? ? ? ? ? ? ? ? //nvgtDataPager.Buttons.Last.Enabled = false; ? ? ? ? ? ? ? ? //nvgtDataPager.Buttons.NextPage.Enabled = false;
? ? ? ? ? ? ? ? endIndex = row; ? ? ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[2].Enabled = false; ? ? ? ? ? ? ? ? nvgtDataPager.Buttons.CustomButtons[3].Enabled = false;
? ? ? ? ? ? }
? ? ? ? ? ? //分页获取数据列表 ? ? ? ? ? ? // ? DataTable dt = null;
? ? ? ? ? ? gcList.DataSource = _dtList; ? ? ? ? ? ? Caption_CN.RoutingList(gvList);
? ? ? ? ? ? nvgtDataPager.DataSource = _dtList; ? ? ? ? ? ? nvgtDataPager.TextStringFormat = string.Format("第 {0}页, 共 {1}页", pageIndex, pageCount); ? ? ? ? }
?
|