基本信息介绍
*编程语言:python3.7
使用的包:selenium,ddt,unittest,yaml等*
项目结构:
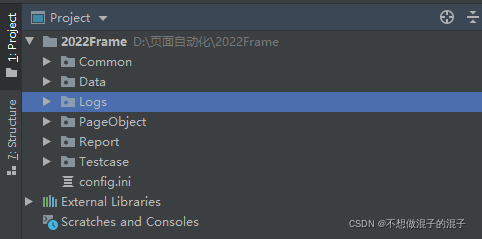
详细代码
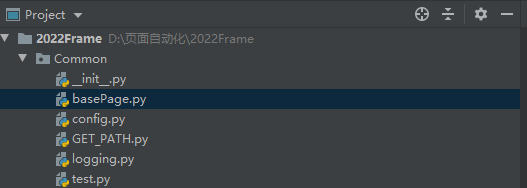
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.wait import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
class BasePage(object):
def __init__(self, driver):
self.driver = driver
self.driver.maximize_window()
def driver_close(self):
self.driver.close()
def visit(self,url):
self.driver.get(url)
"""
定位元素的方法+显示等待
selecotr是元祖类型,放入两个值,一个是定位方式,一个是具体的值
x是定位方式,y是值
__selector = selector.lower()
"""
def get_element(self, selector):
__selector = selector
x = __selector[0].lower()
y = __selector[1]
__aaa = ['id', 'name', 'class_name', 'link_text', 'xpath', 'tag_name', 'css_selector']
if x in __aaa:
if x == 'id':
element = WebDriverWait(self.driver, 10, 1).until(EC.visibility_of(self.driver.find_element(By.ID, y)))
return element
elif x == 'name':
element = self.driver.find_element(By.NAME, y)
return element
elif x == 'class_name':
element = self.driver.find_element(By.CLASS_NAME, y)
return element
elif x == 'link_text':
element = self.driver.find_element(By.LINK_TEXT, y)
return element
elif x == 'xpath':
element = WebDriverWait(self.driver, 10, 1).until(EC.visibility_of(self.driver.find_element(By.XPATH, y)))
element = self.driver.find_element(By.XPATH, y)
return element
elif x == 'tag_name':
element = self.driver.find_element(By.TAG_NAME, y)
return element
elif x == 'css_selector':
element = self.driver.find_element(By.CSS_SELECTOR, y)
return element
else:
print('错误的定位方式:{}'.format(x) + ',请输入 id name class_name link_text xpath tag_name css_selector 等')
"""获取当前窗口的url"""
def get_current_url(self):
current_url = self.driver.current_url
return current_url
"""获取所有窗口句柄"""
def get_all_windows(self):
windows = self.driver.window_handles
print("windows:" + windows)
return windows
"""获取当前窗口句柄"""
def get_current_window(self):
current_window = self.driver.current_window_handle
return current_window
"""获取当前标题"""
def get_title(self):
title = self.driver.title
return title
"""切换窗口"""
def switch_window(self):
all_window_handles = self.driver.window_handles
if len(all_window_handles) > 0:
self.driver.switch_to.window(all_window_handles[-1])
"""点击操作"""
def click(self, selector):
item = selector[1]
if selector[0].upper() =='XPATH':
if WebDriverWait(self.driver, 10, 1).until(EC.element_to_be_clickable((By.XPATH, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='ID':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.ID, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='CLASS_NAME':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.CLASS_NAME, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='TAG_NAME':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.TAG_NAME, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='LINK_TEXT':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.LINK_TEXT, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='NAME':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.NAME, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='CSS_SELECTOR':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.CSS_SELECTOR, item))).click():
self.get_element(selector).click()
elif selector[0].upper() =='PARTIAL_LINK_TEXT':
if WebDriverWait(self.driver, 10).until(EC.element_to_be_clickable((By.PARTIAL_LINK_TEXT, item))).click():
self.get_element(selector).click()
else:
print('错误的定位方式:{}'.format(selector[0].upper()))
raise ValueError
"""输入操作"""
def type(self, selector, value):
element = self.get_element(selector)
element.clear()
try:
element.send_keys(value)
except BaseException:
print("输入内容错误")
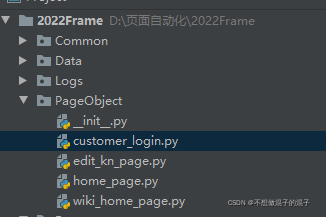
class CustomerLogin(BasePage):
username = ('xpath', '/html/body/div[1]/div/div/div[1]/input')
password = ('xpath', '/html/body/div[1]/div/div/div[2]/input')
login_button = ('xpath', '/html/body/div[1]/div/div/div[4]/button')
def customerLogin(self):
self.type(self.username, "user20220105")
self.type(self.password, "123456abc")
self.click(self.login_button)
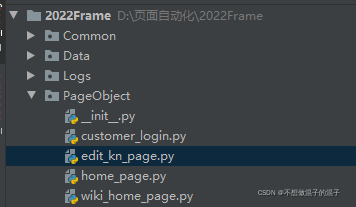
from time import sleep
from selenium.webdriver.common.keys import Keys
from Common.basePage import BasePage
import datetime
class EditKnPage(BasePage):
title = ("XPATH", '//*[@id="app"]/div/div[2]/div[2]/div[2]/div[1]/input')
class_icon = ("XPATH", '//*[@id="app"]/div/div[2]/div[2]/div[1]/div[1]/div[2]/i')
class_1 = ("XPATH", "//*[@id='tree']/li/ul/li[3]/span[2]/span")
class_ensure = ("XPATH", '/html/body/div[last()]/div/div[2]/div/div[2]/div[3]/div/button[2]')
apply_site_baike = ("XPATH", "//*[@id='app']/div/div[2]/div[2]/div[1]/div[2]/div[2]/label[1]/span[2]/span")
apply_site_bot = ("XPATH", "//*[@id='app']/div/div[2]/div[2]/div[1]/div[2]/div[2]/label[2]/span[2]/span")
field_icon = ("XPATH", "//*[@id='app']/div/div[2]/div[2]/div[1]/div[3]/div[2]/i")
field_option = ("XPATH", "//*[@id='tree']/li/ul/li[2]/span[2]/span")
field_ensure = ("XPATH", "/html/body/div[last()]/div/div[2]/div/div[2]/div[3]/div/button[2]")
open_to_all_fields = ("XPATH", '//*[@id="app"]/div/div[2]/div[2]/div[1]/div[4]/label/span[2]')
expire_to_look_opt1 = ("XPATH", "//*[@id='app']/div/div[2]/div[2]/div[1]/div[5]/div[2]/div/label[2]/span[2]")
add_answer_button = ("xpath", "//*[@id='app']/div/div[2]/div[2]/div[1]/div[6]/button")
belong_entry_button = ("xpath", "//*[@id='app']/div/div[2]/div[2]/div[2]/div[4]/div/div[2]/div/div/div[1]/div/div[2]/i")
belong_entry_first = ("xpath", "/html/body/div[4]/div/div[2]/div/div[2]/div[2]/div/div/div/div/ul/li[2]/span[2]/span/span")
belong_entry_close = ("xpath", "/html/body/div[4]/div/div[2]/div/div[2]/div[3]/div/button[2]")
submit_button = ('xpath', "//*[@id='app']/div/div[2]/div[3]/div[3]/div[2]/button[3]")
def type_kn_title(self):
txt = str(datetime.datetime.today())[:-7]
self.type(self.title, txt)
def choose_classification(self):
sleep(1)
self.click(self.class_icon)
self.click(self.class_1)
print('选择待删除分类done')
self.click(self.class_ensure)
print('关闭分类弹框done')
def choose_apply(self, value=1):
if value == 0:
self.click(self.apply_site_baike)
self.click(self.apply_site_bot)
print('应用端选择为【门户bot+百科】')
elif value == 1:
self.click(self.apply_site_bot)
print('应用端选择为【百科】')
elif value == 2:
self.click(self.apply_site_baike)
print('应用端选择为【门户bot】')
else:
print('value值不正确,请输入0 1 2')
def choose_field(self):
self.click(self.field_icon)
self.click(self.field_option)
self.click(self.field_ensure)
print('勾选领域完成')
def open_to_fields(self):
self.click(self.open_to_all_fields)
print('开放领域全选')
def expire_to_look(self):
self.click(self.expire_to_look_opt1)
print('失效可看')
def add_answers(self):
try:
self.click(self.add_answer_button)
except ValueError:
print('edit_kn_page: 新增答案错误')
def belong_entry(self):
try:
self.click(self.belong_entry_button)
self.click(self.belong_entry_first)
self.click(self.belong_entry_close)
except ValueError:
print('edit_kn_page: 所属词条箭头点击失败')
def submit_kn(self):
try:
self.click(self.submit_button)
except ValueError:
print('edit_kn_page: 提交知识点成功')
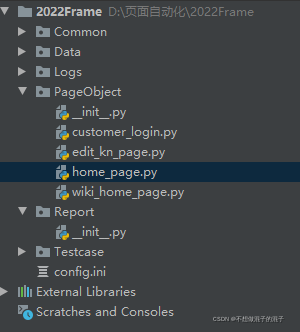
from Common.basePage import BasePage
class HomePage(BasePage):
wiki_module = ('xpath', '/html/body/div[1]/header/div[2]/div/div[2]/div')
doc_module = ('xpath', '//*[@id="app"]/header/div[2]/div/div[3]/div')
school_module = ('xpath', '//*[@id="app"]/header/div[2]/div/div[4]/div')
community_module = ('xpath', '//*[@id="app"]/header/div[2]/div/div[5]/div')
def jump_wiki(self):
self.click(self.wiki_module)
def jump_doc(self):
self.click(self.doc_module)
def jump_school(self):
self.click(self.school_module)
def jump_community(self):
self.click(self.community_module)
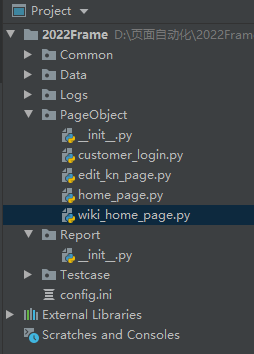
from Common.basePage import BasePage
class WikiHomePage(BasePage):
new_wiki_button = ('XPATH', "//div[@class='create-btn']")
new_entry_button = ('XPATH', '//*[@id="app"]/div[1]/div[2]/div/div/div[1]/div[2]/div[1]/div[2]/div[2]')
feedback_button = ('XPATH', '//*[@id="app"]/div[1]/div[2]/div/div/div[1]/div[2]/div[1]/div[2]/div[3]')
def click_new_knowledge(self):
self.click(self.new_wiki_button)
def click_new_entry(self):
self.click(self.new_entry_button)
def click_feedback(self):
self.click(self.feedback_button)
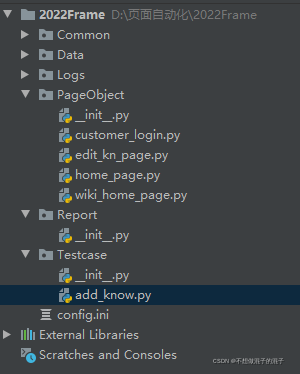
"""百科模块"""
import unittest
from time import sleep
from selenium import webdriver
from Common.basePage import BasePage
from PageObject import wiki_home_page
from PageObject.customer_login import CustomerLogin
from PageObject.edit_kn_page import EditKnPage
from PageObject.home_page import HomePage
from PageObject.wiki_home_page import WikiHomePage
class Login1(unittest.TestCase):
@classmethod
def setUpClass(cls) -> None:
cls.driver =webdriver.Chrome()
cls.lp = CustomerLogin(cls.driver)
cls.hp = HomePage(cls.driver)
cls.wkp = WikiHomePage(cls.driver)
cls.knp =EditKnPage(cls.driver)
@classmethod
def tearDownClass(cls) -> None:
cls.driver.quit()
pass
def test_01_goto_wiki(self):
self.lp.visit('https://v5-test.faqrobot.cn/webaikn/#/login')
self.lp.customerLogin()
self.hp.jump_wiki()
print('跳转到百科首页成功')
def test_02_new_wiki_point(self):
self.wkp.click_new_knowledge()
self.wkp.switch_window()
sleep(3)
self.knp.type_kn_title()
self.knp.choose_classification()
self.knp.choose_apply()
self.knp.open_to_fields()
self.knp.expire_to_look()
self.knp.add_answers()
self.driver.execute_script("window.scrollTo(0,0)")
self.knp.belong_entry()
self.driver.switch_to.frame("ueditor_0")
self.knp.type(('xpath', "/html/body"), "自动化测试:python")
self.driver.switch_to.default_content()
self.driver.execute_script("window.scrollTo(0,600)")
self.driver.switch_to.frame("ueditor_1")
self.knp.type(('xpath', "/html/body"), "自动化测试:java")
self.driver.switch_to.default_content()
self.driver.execute_script("window.scrollTo(0,800)")
sleep(5)
self.knp.submit_kn()
|