首先需要导入对应的jar包
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
然后去springmvc.xml里编写相关的配置
<!-- 给上传做配置(解析器) -->
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!--编码格式-->
<property name="defaultEncoding" value="utf-8"></property>
<!--上传的最大限制(字节k) -->
<property name="maxUploadSize" value="102400000"></property>
</bean>
案例:
<form action="controller/upload" method="post" enctype="multipart/form-data">
上传1 :<input type="file" value="file"><br>
上传2 :<input type="file" value="file"><br>
上传3 :<input type="file" value="file"><br>
<input type="submit" value="确定">
<a href="download/xxx.zip">下载1</a>
<a href="download/ci.png">下载2</a>
<a href="controller/download?fileName=ci.png">下载3</a>
</form>
接下来要在webapp文件夹下新建上传的文件夹upload和下载的文件夹download
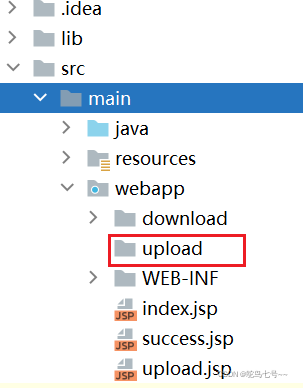
package org.zhx.controller;
import org.apache.commons.io.FileUtils;
import org.aspectj.util.FileUtil;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.Date;
import java.util.Random;
@Controller
@RequestMapping("/controller")
public class UploadController {
@RequestMapping("/upload")
public String upLoad(MultipartFile[] file,HttpServletRequest request) {
for (int i = 0; i < file.length; i++) {
//获得上传的路径 路径要与你自己所建的文件夹的名字保持一致
String path = request.getServletContext().getRealPath("/upload/");
//拿到从页面上传过来的文件
String name = file[i].getOriginalFilename();
//将拿到的文件重命名
String newName = new Date().getTime()+new Random(100000).nextInt()+name;
//上传的路径
File f = new File(path+newName);
//上传
try {
file[i].transferTo(f);
} catch (IOException e) {
e.printStackTrace();
}
}
return "success";
}
@RequestMapping("/download")
public ResponseEntity<byte[]> download(@RequestParam("fileName")String fileName, HttpServletRequest request) throws IOException {
//1.获得下载的路径
String path=request.getServletContext().getRealPath("/download/");
System.out.println(path);
//下载的完整路径
File f = new File(path+fileName);
System.out.println("path+fileName:"+path+fileName);
//转换格式
String name = new String(fileName.getBytes("utf-8"),"iso8859-1");
//头部信息对象,转流
HttpHeaders hh = new HttpHeaders();
hh.setContentDispositionFormData("attachment",name);
hh.setContentType(MediaType.APPLICATION_OCTET_STREAM);
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(f),hh,HttpStatus.CREATED);
}
}
|