一,测试调用类
1.调用类
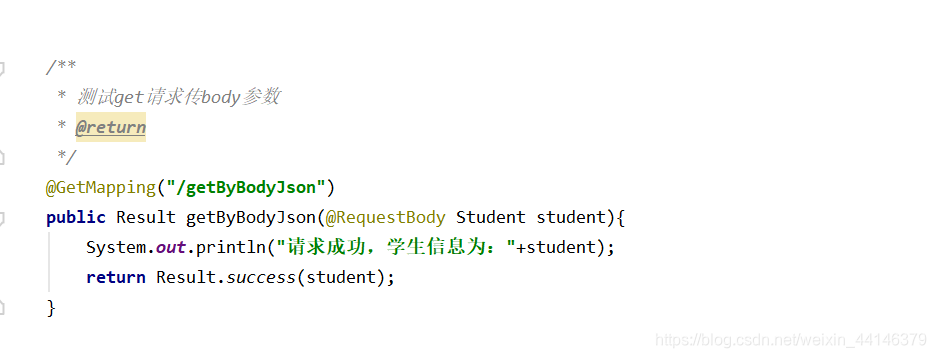
2.postman调用
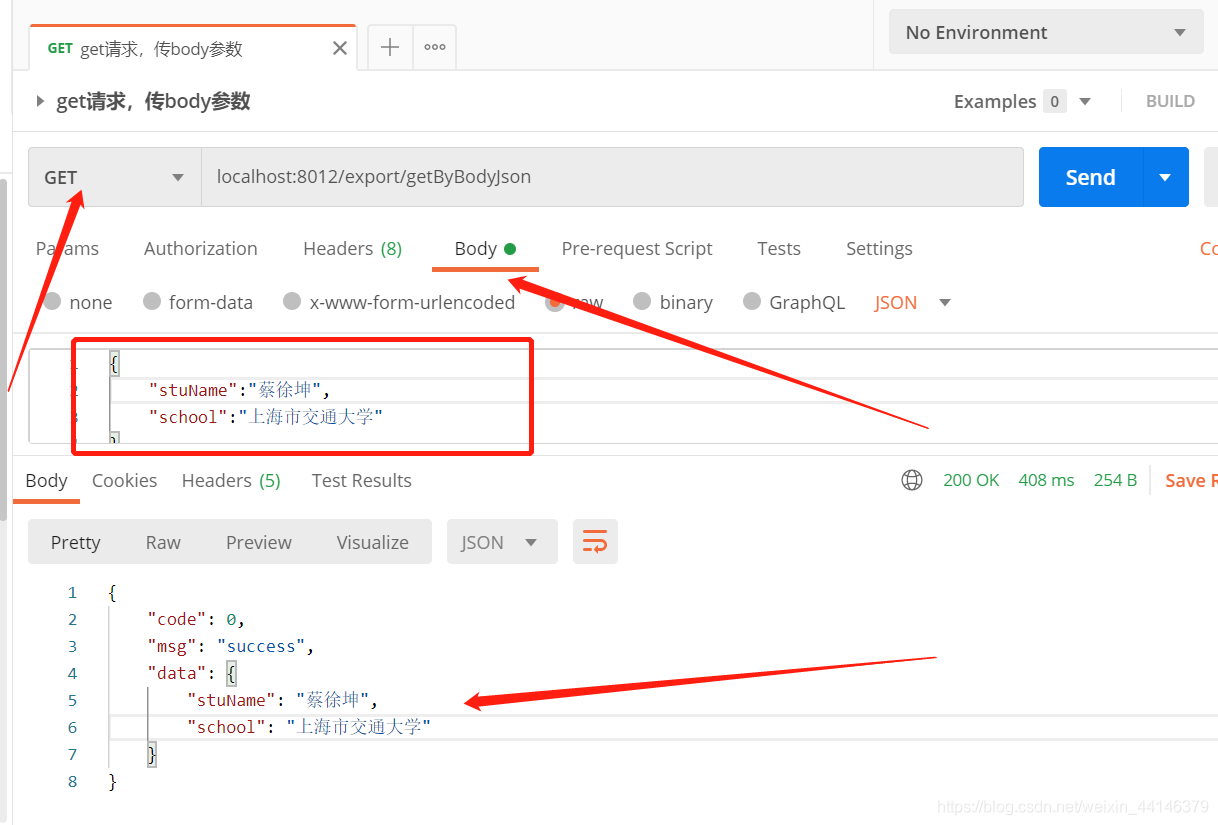
get请求,入参是json格式,可以请求成功,具体解决方式如下:
?二.使用Http工具类调用Get请求(json参数)
1.引入httpClient依赖
? ? ?
?<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
? ? ? ? <dependency>
? ? ? ? ? ? <groupId>org.apache.httpcomponents</groupId>
? ? ? ? ? ? <artifactId>httpclient</artifactId>
? ? ? ? ? ? <version>4.5.6</version>
? ? ? ? </dependency>
2.定义一个HttpGet实体类
import org.apache.http.client.methods.HttpEntityEnclosingRequestBase;
import java.net.URI;
/**
?* @author wdy
?* @version 1.0.0
?* @ClassName HttpGetWithEntity
?* @Description TODO 定义一个带body的GET请求 继承 HttpEntityEnclosingRequestBase
?* @createTime 2020.11.18 13:51
?*/
public class HttpGetWithEntity extends HttpEntityEnclosingRequestBase {
? ? private final static String METHOD_NAME = "GET";
? ? @Override
? ? public String getMethod() {
? ? ? ? return METHOD_NAME;
? ? }
? ? public HttpGetWithEntity() {
? ? ? ? super();
? ? }
? ? public HttpGetWithEntity(final URI uri) {
? ? ? ? super();
? ? ? ? setURI(uri);
? ? }
? ? HttpGetWithEntity(final String uri) {
? ? ? ? super();
? ? ? ? setURI(URI.create(uri));
? ? }
}
3.HttpGet请求公共方法
? ?
/**
? ? ?* 发送get请求,参数为json
? ? ?* @param url
? ? ?* @param param
? ? ?* @param encoding
? ? ?* @return
? ? ?* @throws Exception
? ? ?*/
? ? public static String sendJsonByGetReq(String url, String param, String encoding) throws Exception {
? ? ? ? String body = "";
? ? ? ? //创建httpclient对象
? ? ? ? CloseableHttpClient client = HttpClients.createDefault();
? ? ? ? HttpGetWithEntity httpGetWithEntity = new HttpGetWithEntity(url);
? ? ? ? HttpEntity httpEntity = new StringEntity(param, ContentType.APPLICATION_JSON);
? ? ? ? httpGetWithEntity.setEntity(httpEntity);
? ? ? ? //执行请求操作,并拿到结果(同步阻塞)
? ? ? ? CloseableHttpResponse response = client.execute(httpGetWithEntity);
? ? ? ? //获取结果实体
? ? ? ? HttpEntity entity = response.getEntity();
? ? ? ? if (entity != null) {
? ? ? ? ? ? //按指定编码转换结果实体为String类型
? ? ? ? ? ? body = EntityUtils.toString(entity, encoding);
? ? ? ? }
? ? ? ? //释放链接
? ? ? ? response.close();
? ? ? ? return body;
? ? }
4.运行服务,本地测试调用一下该接口
?
? /**
? ? ?* 测试 Get 请求
? ? ?*/
? ? @Test
? ? public void test(){
? ? ? ? String url = "http://127.0.0.1:8012/export/getByBodyJson";
? ? ? ? Map<String, Object> map = new HashMap<>();
? ? ? ? map.put("stuName","张一山");
? ? ? ? map.put("school","北京戏剧学院");
? ? ? ? String reqParams = JSONArray.toJSON(map).toString();
? ? ? ? try {
? ? ? ? ? ? String s = sendJsonByGetReq(url, reqParams, "UTF-8");
? ? ? ? ? ? System.out.println("请求Get请求返回结果:"+s);
? ? ? ? } catch (Exception e) {
? ? ? ? ? ? e.printStackTrace();
? ? ? ? }
? ? }
调用成功
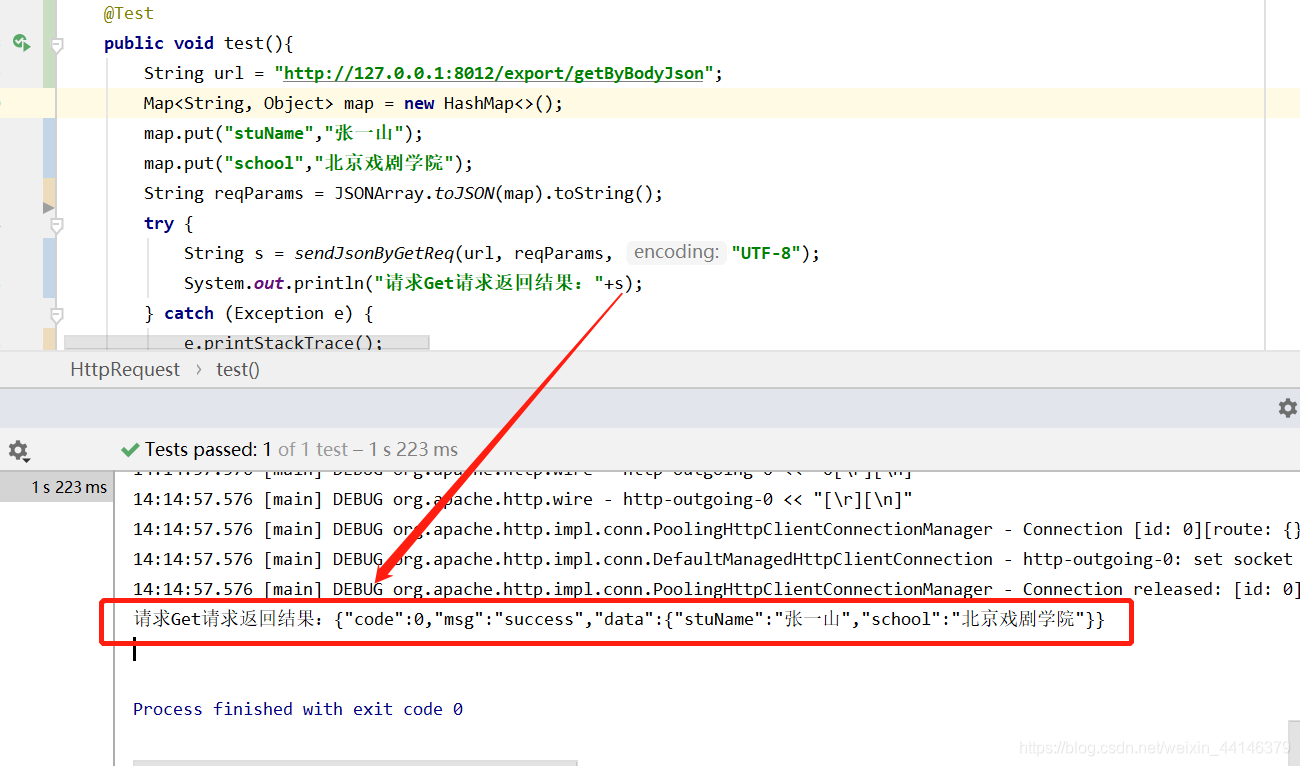
|