package io.renren.common.utils;
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.*;
import io.renren.modules.util.StringUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.util.HashMap;
import java.util.Map;
@Component
public class PdfUtils {
public static String downloadFilePath;
@Value("${downloadFilePath}")
public void setDownloadFilePath(String downloadFilePath) {
PdfUtils.downloadFilePath = downloadFilePath;
}
public static String fillDataToTemplate(String templateName, String newPdfPath, Map<String, Object> dataMap) throws Exception {
PdfReader reader;
FileOutputStream out;
ByteArrayOutputStream bos;
PdfStamper stamper;
try {
out = new FileOutputStream(newPdfPath);
reader = new PdfReader(getTemplatePath(templateName));
int pageCount = reader.getNumberOfPages();
bos = new ByteArrayOutputStream();
stamper = new PdfStamper(reader, bos);
AcroFields form = stamper.getAcroFields();
String fontPath = getFontPath("");
BaseFont bfChinese = BaseFont.createFont(fontPath + ",1", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
form.addSubstitutionFont(bfChinese);
for (String key : dataMap.keySet()) {
String value = StringUtils.checkObj2Str(dataMap.get(key));
form.setField(key, value);
}
stamper.setFormFlattening(true);
stamper.close();
Document doc = new Document();
PdfCopy copy = new PdfCopy(doc, out);
doc.open();
PdfImportedPage importPage = null;
for (int j = 1; j <= pageCount; j++) {
importPage = copy.getImportedPage(new PdfReader(bos.toByteArray()), j);
copy.addPage(importPage);
}
doc.close();
} catch (Exception e) {
throw e;
}
return newPdfPath;
}
private static String getFontPath(String font) {
font = StringUtils.isNotEmpty(font) ? font : "simsun.ttc";
String path = Thread.currentThread().getContextClassLoader().getResource("").getPath();
File file = new File(path);
String absolutePath = file.getAbsolutePath().replace("\\", "/");
String fontPath = absolutePath + "/font/" + font;
return fontPath;
}
private static String getTemplatePath(String filePath) {
String path = Thread.currentThread().getContextClassLoader().getResource("").getPath();
File file = new File(path);
String absolutePath = file.getAbsolutePath().replace("\\", "/");
String templatePath = absolutePath + "/public/template/" + filePath;
return templatePath;
}
public static void main(String[] args) {
Map<String, Object> dataMap = new HashMap<String, Object>();
dataMap.put("xue_hao", "2009232425009abcdefg");
dataMap.put("xing_ming", "小三a");
dataMap.put("xing_bie", "男");
dataMap.put("chu_sheng_ri_qi", "1989年9月5日");
dataMap.put("shen_fen_zheng_hao", "130525198909051234");
dataMap.put("jia_ting_zhu_zhi", "河北省邢台市");
try {
String path = Thread.currentThread().getContextClassLoader().getResource("").getPath();
File file = new File(path);
String absolutePath = file.getAbsolutePath().replace("\\", "/");
String templatePath = absolutePath + "/public/template/student.pdf";
String newPdfPath = "D:/student.pdf";
fillDataToTemplate(templatePath, newPdfPath, dataMap);
System.out.println("生成文件成功!");
} catch (Exception e) {
System.err.println("生成文件出错!");
e.printStackTrace();
}
}
}
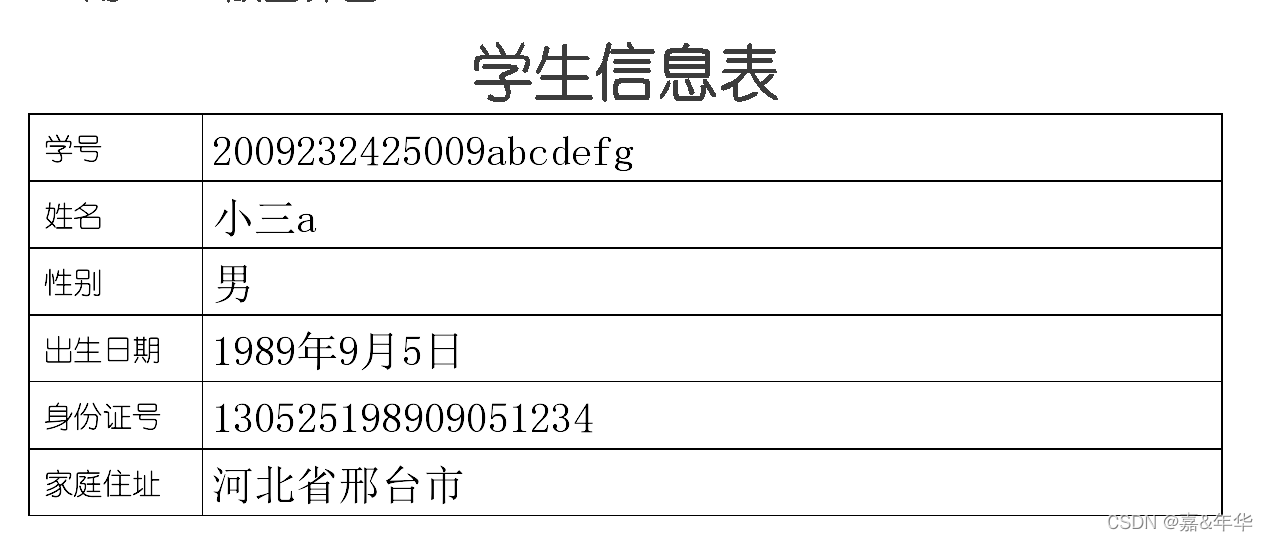 制作模板过程: 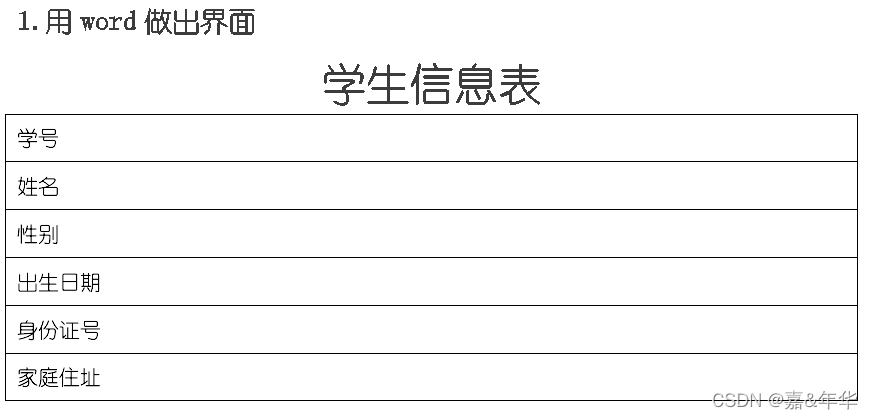  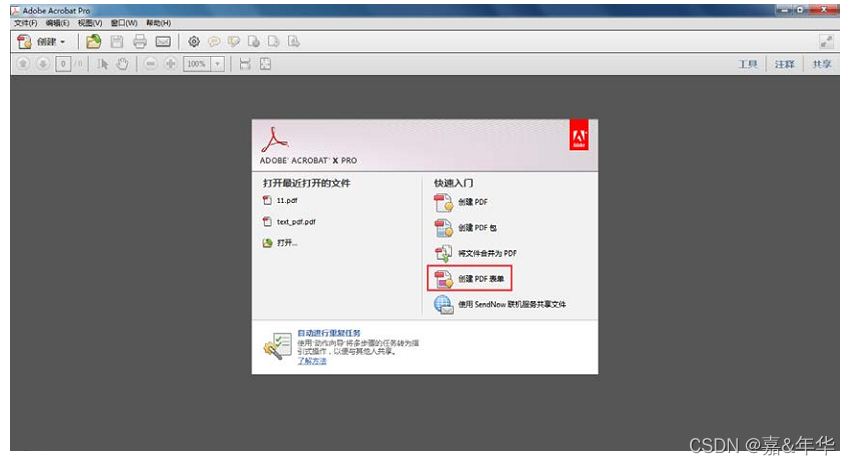 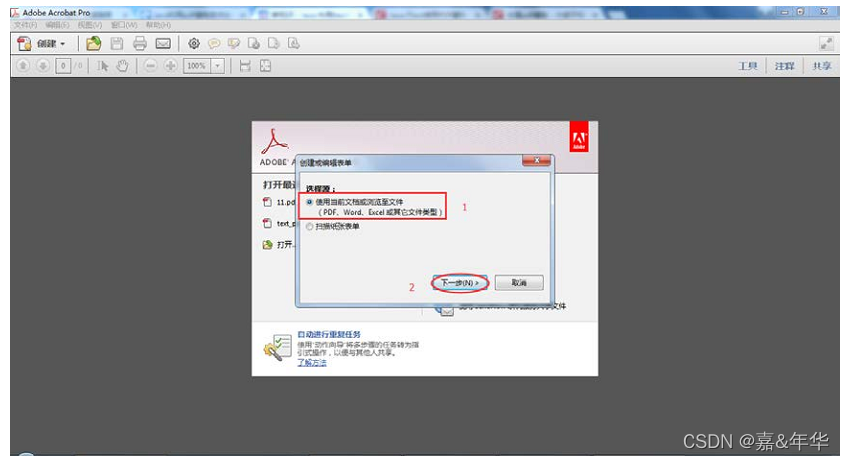 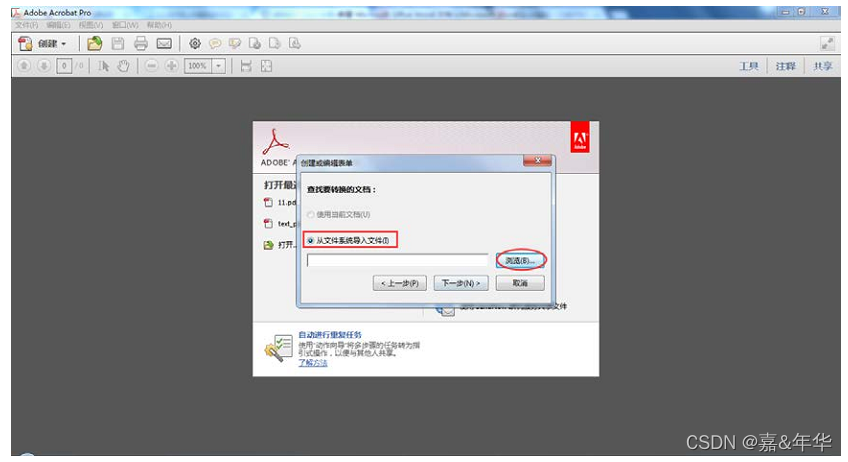 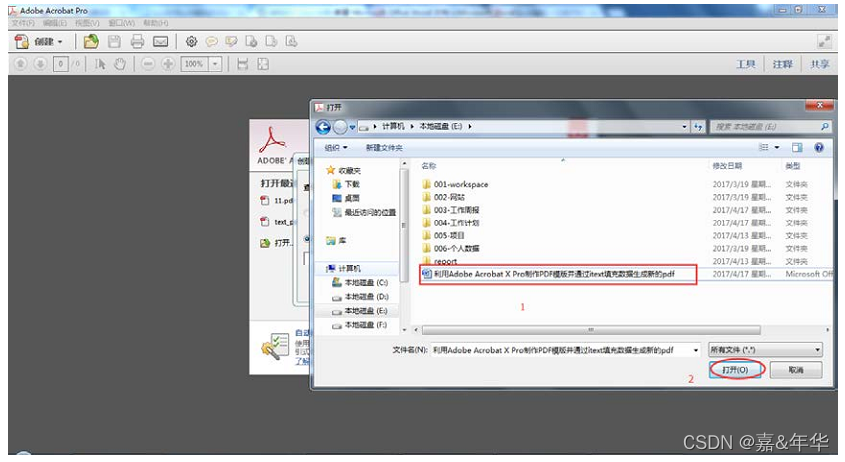 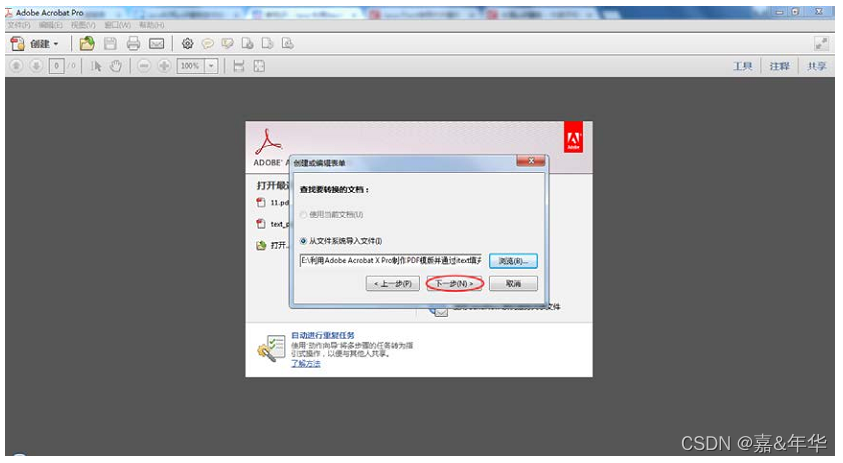 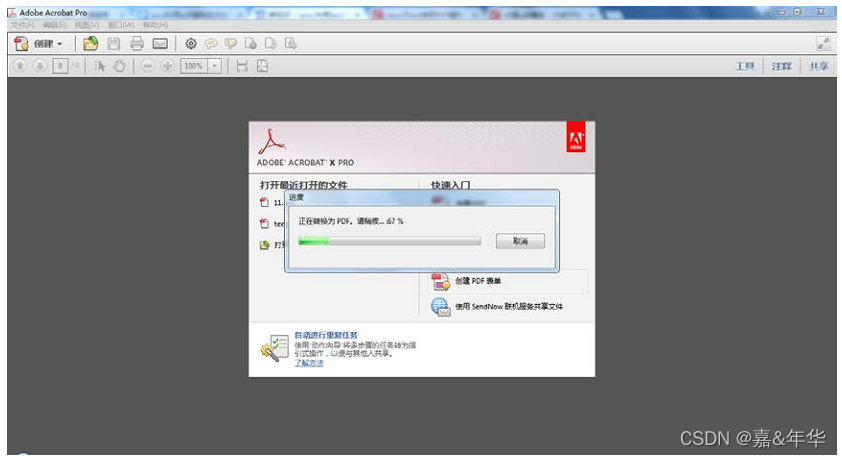 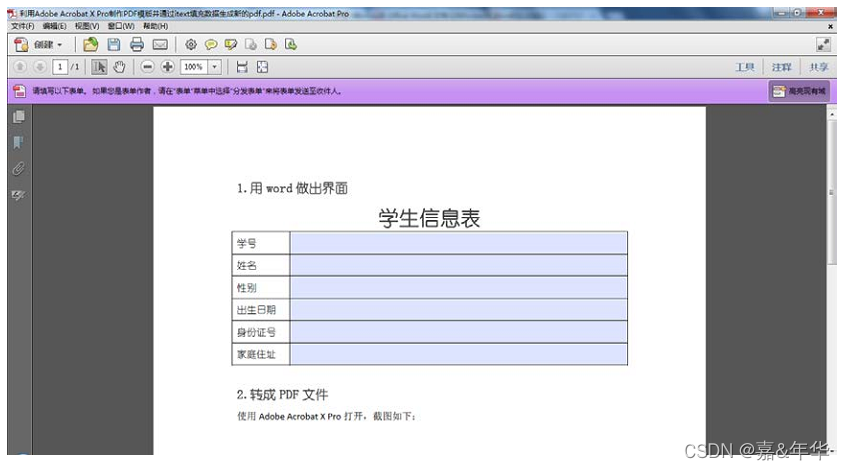 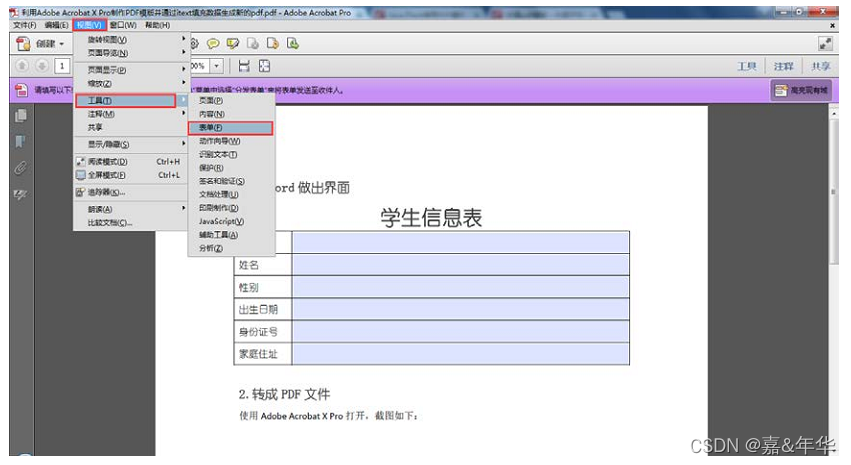 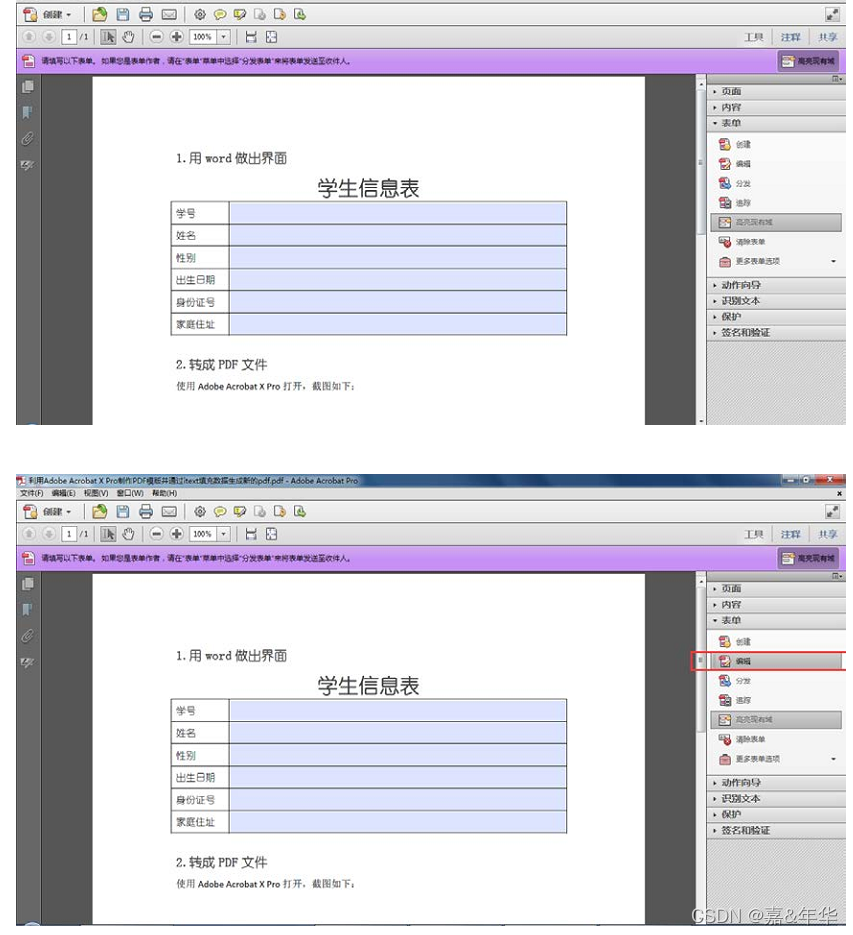 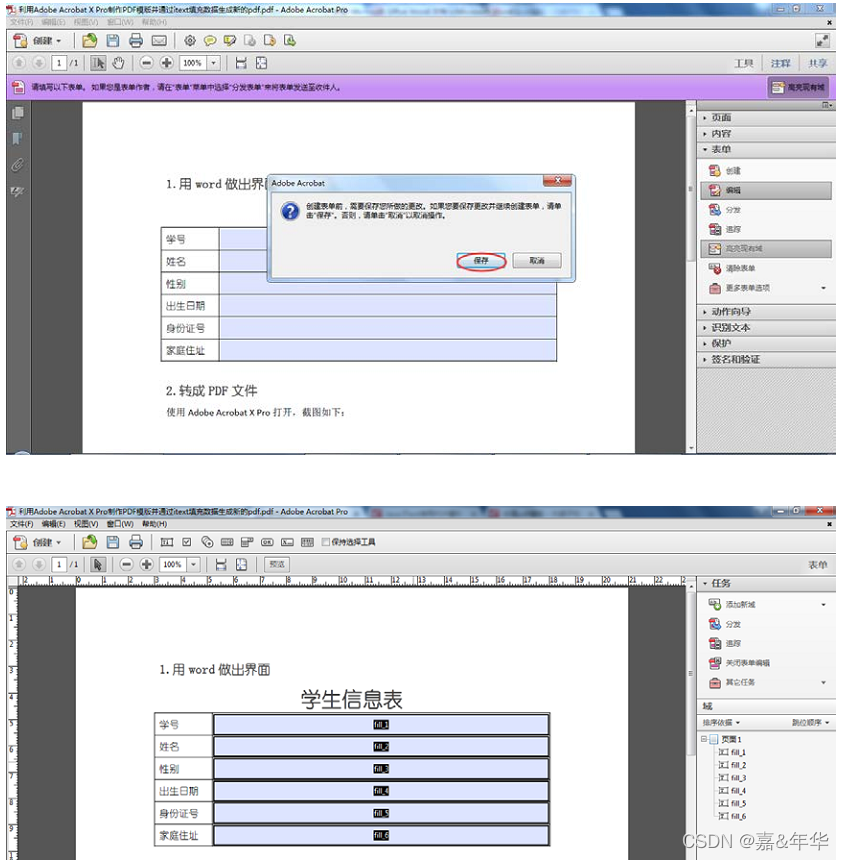 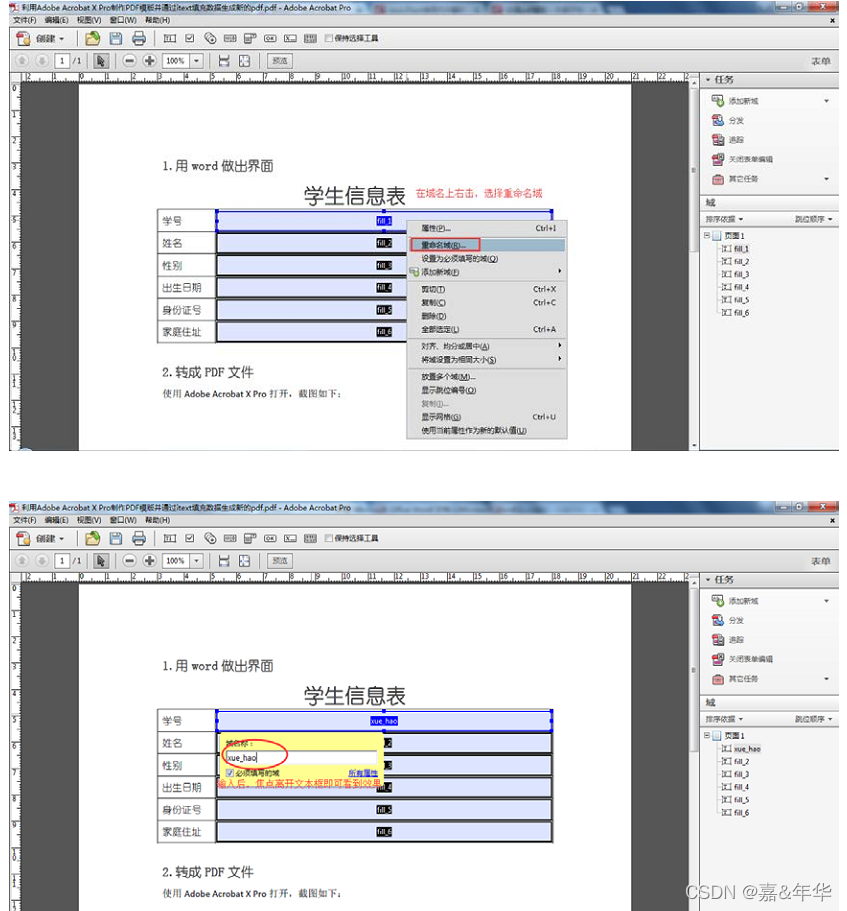 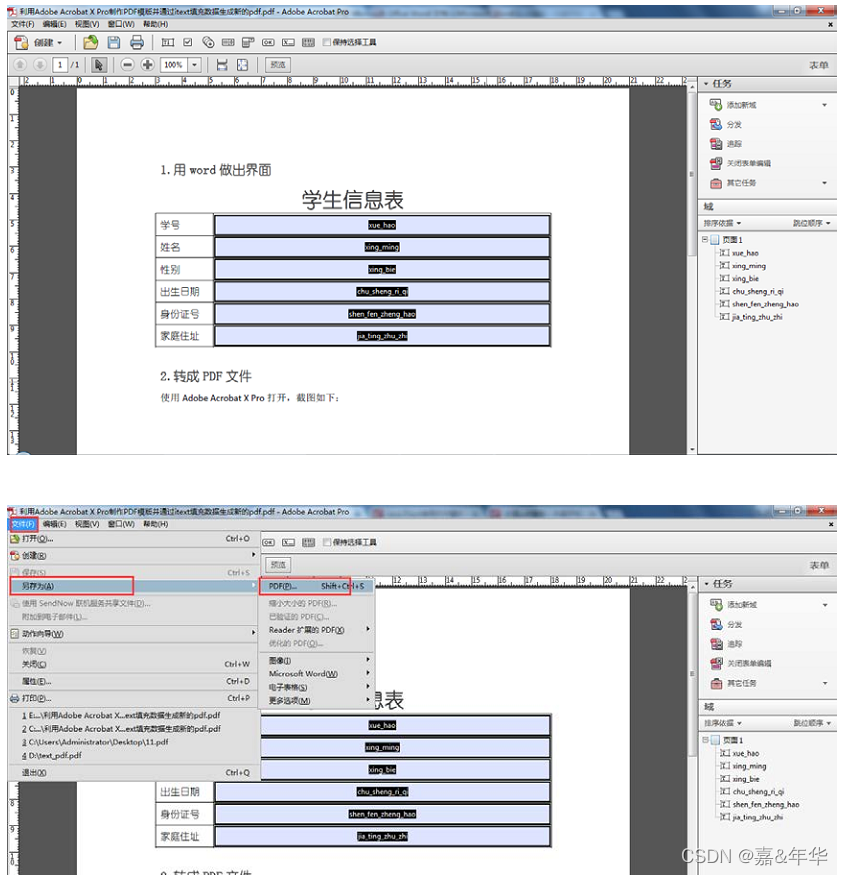 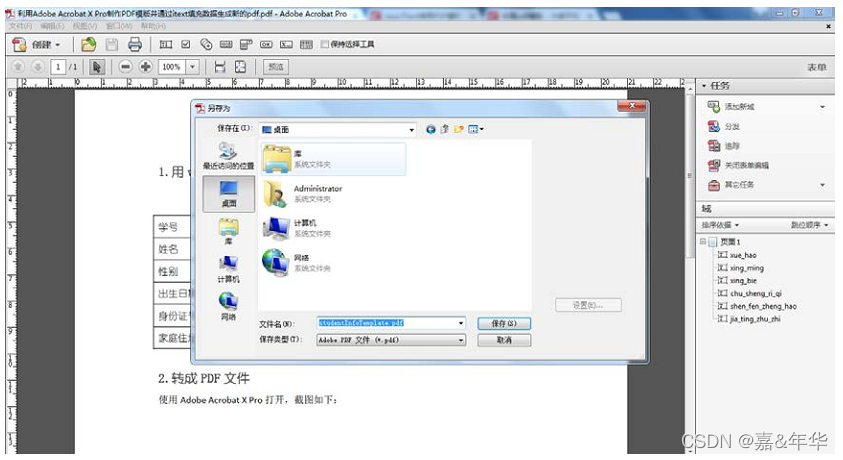
|