个人 博客链接:博客系统 | 登录
测试总结:测试用例涉及到的范围有界面、功能、兼容性、性能、安全性测试这五大方面,由于设计和测试环境受限的原因,本篇文章测试主要以功能部分测试为主,而BUG最容易出现的地方就是功能,界面测试结果与需求一致,未发现BUG,兼容性测试在主流的浏览器Chrome和Firefox运行时无异常。由于项目在安全性方面只做了一个密码的加密与解密,并且注册之后在数据库中是采用加密方式存储的,所以与设计需求的安全性一致。
? ? ? ? 通过手工测试分别测试了注册功能的测试用例一共20个,发现?BUG2个,登录部分测试了11个用例,发现BUG2个,主页部分测试了7个用例,发现1个BUG,发表博客部分测试了7个用例,发现BUG3个。
????????自动化测试采用了unittest框架分别测试了共13个用例,注册功能部分代码6个用例,登录部分代码6个用例,写博客部分代码1个用例。测试脚本都成功运行,发现BUG7个。
一、测试用例
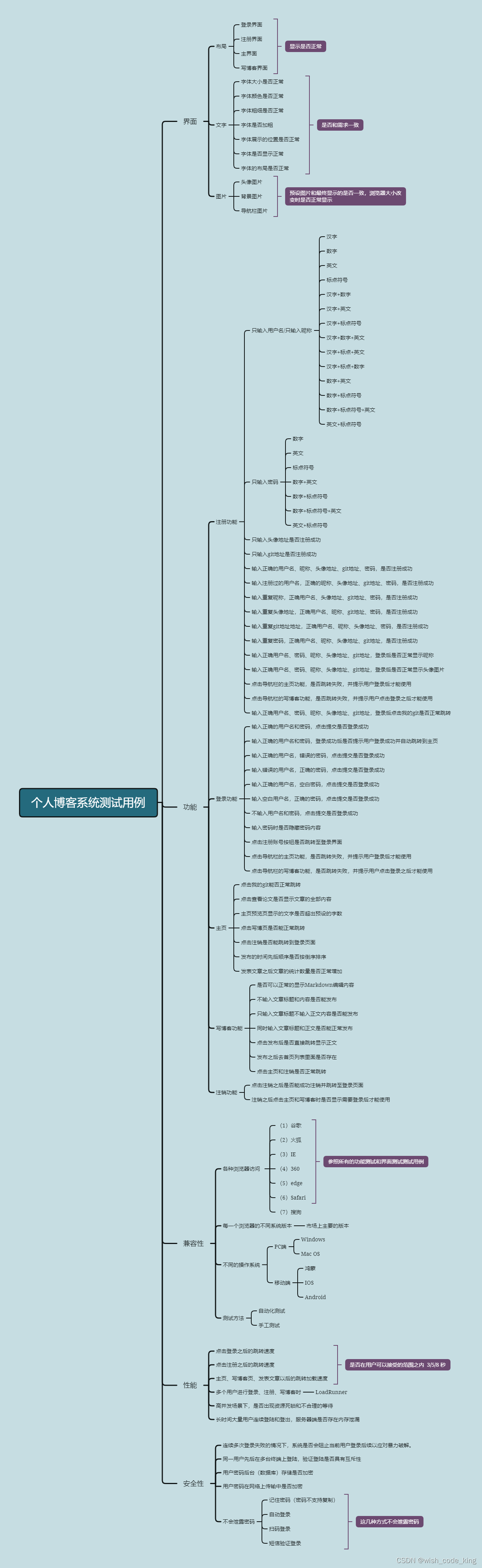
二、BUG描述
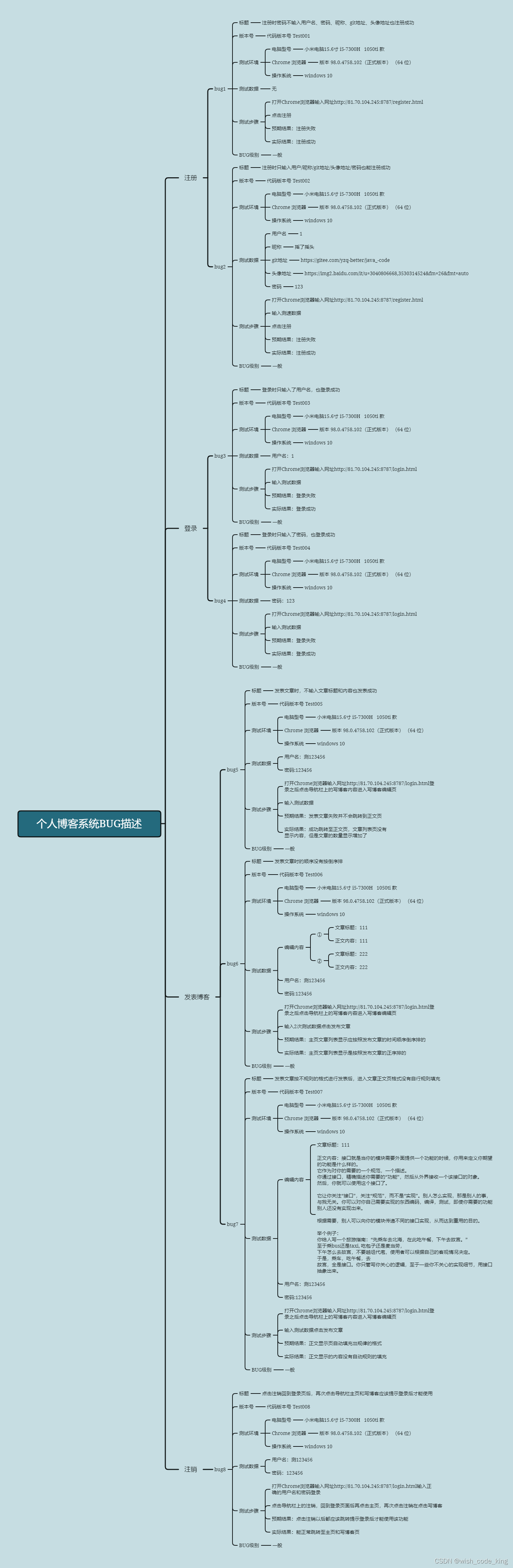
三、自动化测试(selenium)
自动化测试采用Unittest框架
(1)注册
from selenium import webdriver
import unittest
import time
class Register(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.implicitly_wait(10)
self.base_url = "http://81.70.104.245:8787/register.html"
self.driver.maximize_window()
self.verificationErrors = []
self.accept_next_alert = True
# 测试注册时只输入用户名的情况
def test_01_register_username(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("测110110110")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 注册成功之后会显示注册成功,并显示点击跳转 这里是点击是否能跳转到首页
# 测试注册时只输入昵称的情况
def test_02_register_nickname(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("nickname").send_keys("测试NICKNAME110")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 测试注册时只输入头像地址的情况
def test_03_register_avatar(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("avatar").send_keys(
"https://img1.baidu.com/it/u=2716398045,2043787292&fm=253&fmt=auto&app=120&f=JPEG?w=800&h=800")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 测试注册时只输入git地址的情况
def test_04_register_git(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("git").send_keys("https://gitee.com/yzq-better/java_-code.git")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 测试注册时只输入密码的情况
def test_05_register_git(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("password").send_keys("1234567890")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 注册时全部输入的情况
def test_06_register_git(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("测120120120")
driver.find_element_by_id("nickname").send_keys("测试name")
driver.find_element_by_id("avatar").send_keys(
"https://img1.baidu.com/it/u=2716398045,2043787292&fm=253&fmt=auto&app=120&f=JPEG?w=800&h=800")
driver.find_element_by_id("git").send_keys("https://gitee.com/yzq-better/java_-code.git")
driver.find_element_by_id("password").send_keys("120120120")
driver.find_element_by_id("submit").click()
time.sleep(3)
def tearDown(self):
self.driver.quit()
self.assertEqual([], self.verificationErrors)
if __name__ == "__main__":
unittest.main(verbosity=2)
(2)登录
from selenium import webdriver
import unittest
import time
class Login(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.implicitly_wait(10)
self.base_url = "http://81.70.104.245:8787/login.html"
self.driver.maximize_window()
self.verificationErrors = []
self.accept_next_alert = True
# 登录时只输入用户名的情况
def test_07_login_username(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("测120120120")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 登录时只输入不存在的用户名的情况
def test_08_login_username(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("不存在123456")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 登录时只输入密码的情况
def test_09_register_password(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("password").send_keys("120120120")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 登录时只输入不存在密码的情况
def test_10_register_password(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("password").send_keys("1357910")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 登录时输入正确的用户名和密码的情况
def test_11_register_avatar(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("测120120120")
driver.find_element_by_id("password").send_keys("120120120")
driver.find_element_by_id("submit").click()
time.sleep(3)
# 登录时输入不存在的用户名和密码的情况
def test_12_register_avatar(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("不存在123456789")
driver.find_element_by_id("password").send_keys("789456123")
driver.find_element_by_id("submit").click()
time.sleep(3)
def tearDown(self):
self.driver.quit()
self.assertEqual([], self.verificationErrors)
if __name__ == "__main__":
unittest.main(verbosity=2)
(3)写博客
from selenium import webdriver
import unittest
import time
class Login(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
self.driver.implicitly_wait(10)
self.base_url = "http://81.70.104.245:8787/login.html"
self.driver.maximize_window()
self.verificationErrors = []
self.accept_next_alert = True
# 登录
def test_13_Write(self):
driver = self.driver
driver.get(self.base_url)
driver.find_element_by_id("username").send_keys("测120120120")
driver.find_element_by_id("password").send_keys("120120120")
driver.find_element_by_id("submit").click()
time.sleep(3)
#跳转到写博客页
driver.find_element_by_xpath("/html/body/div[1]/a[2]").click()
time.sleep(5)
#添加文章标题
driver.find_element_by_id("title").click()
driver.find_element_by_id("title").send_keys("这是一个测试")
driver.find_element_by_id("submit").click()
time.sleep(3)
#返回主页查看文章
driver.find_element_by_xpath("/html/body/div[1]/a[1]").click()
time.sleep(3)
#注销
driver.find_element_by_xpath("/html/body/div[1]/a[3]").click()
time.sleep(3)
def tearDown(self):
self.driver.quit()
self.assertEqual([], self.verificationErrors)
if __name__ == "__main__":
unittest.main(verbosity=2)
(4)HTMLTestRunner
import HTMLTestRunner
import os
import sys
import time
import unittest
def createsuite():
discovers = unittest.defaultTestLoader.discover("../selenium_blog_2022_03_07", pattern="unittest*.py", top_level_dir=None)
print(discovers)
return discovers
if __name__=="__main__":
curpath = sys.path[0]
print(sys.path)
print(sys.path[0])
if not os.path.exists(curpath+'/resultreport'):
os.makedirs(curpath+'/resultreport')
now = time.strftime("%Y-%m-%d-%H %M %S", time.localtime(time.time()))
print(now)
print(time.time())
print(time.localtime(time.time()))
# 文件名
filename = curpath + '/resultreport/'+ now + 'resultreport.html'
with open(filename, 'wb') as fp:
runner = HTMLTestRunner.HTMLTestRunner(stream=fp, title=u"测试报告",description=u"用例执行情况", verbosity=2)
suite = createsuite()
runner.run(suite)
自动生成的测试报告
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>测试报告</title>
<meta name="generator" content="HTMLTestRunner 0.8.2"/>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/>
<style type="text/css" media="screen">
body { font-family: verdana, arial, helvetica, sans-serif; font-size: 80%; }
table { font-size: 100%; }
pre { }
/* -- heading ---------------------------------------------------------------------- */
h1 {
font-size: 16pt;
color: gray;
}
.heading {
margin-top: 0ex;
margin-bottom: 1ex;
}
.heading .attribute {
margin-top: 1ex;
margin-bottom: 0;
}
.heading .description {
margin-top: 4ex;
margin-bottom: 6ex;
}
/* -- css div popup ------------------------------------------------------------------------ */
a.popup_link {
}
a.popup_link:hover {
color: red;
}
.popup_window {
display: none;
position: relative;
left: 0px;
top: 0px;
/*border: solid #627173 1px; */
padding: 10px;
background-color: #E6E6D6;
font-family: "Lucida Console", "Courier New", Courier, monospace;
text-align: left;
font-size: 8pt;
width: 500px;
}
}
/* -- report ------------------------------------------------------------------------ */
#show_detail_line {
margin-top: 3ex;
margin-bottom: 1ex;
}
#result_table {
width: 80%;
border-collapse: collapse;
border: 1px solid #777;
}
#header_row {
font-weight: bold;
color: white;
background-color: #777;
}
#result_table td {
border: 1px solid #777;
padding: 2px;
}
#total_row { font-weight: bold; }
.passClass { background-color: #6c6; }
.failClass { background-color: #c60; }
.errorClass { background-color: #c00; }
.passCase { color: #6c6; }
.failCase { color: #c60; font-weight: bold; }
.errorCase { color: #c00; font-weight: bold; }
.hiddenRow { display: none; }
.testcase { margin-left: 2em; }
/* -- ending ---------------------------------------------------------------------- */
#ending {
}
</style>
</head>
<body>
<script language="javascript" type="text/javascript"><!--
output_list = Array();
/* level - 0:Summary; 1:Failed; 2:All */
function showCase(level) {
trs = document.getElementsByTagName("tr");
for (var i = 0; i < trs.length; i++) {
tr = trs[i];
id = tr.id;
if (id.substr(0,2) == 'ft') {
if (level < 1) {
tr.className = 'hiddenRow';
}
else {
tr.className = '';
}
}
if (id.substr(0,2) == 'pt') {
if (level > 1) {
tr.className = '';
}
else {
tr.className = 'hiddenRow';
}
}
}
}
function showClassDetail(cid, count) {
var id_list = Array(count);
var toHide = 1;
for (var i = 0; i < count; i++) {
tid0 = 't' + cid.substr(1) + '.' + (i+1);
tid = 'f' + tid0;
tr = document.getElementById(tid);
if (!tr) {
tid = 'p' + tid0;
tr = document.getElementById(tid);
}
id_list[i] = tid;
if (tr.className) {
toHide = 0;
}
}
for (var i = 0; i < count; i++) {
tid = id_list[i];
if (toHide) {
document.getElementById('div_'+tid).style.display = 'none'
document.getElementById(tid).className = 'hiddenRow';
}
else {
document.getElementById(tid).className = '';
}
}
}
function showTestDetail(div_id){
var details_div = document.getElementById(div_id)
var displayState = details_div.style.display
// alert(displayState)
if (displayState != 'block' ) {
displayState = 'block'
details_div.style.display = 'block'
}
else {
details_div.style.display = 'none'
}
}
function html_escape(s) {
s = s.replace(/&/g,'&');
s = s.replace(/</g,'<');
s = s.replace(/>/g,'>');
return s;
}
/* obsoleted by detail in <div>
function showOutput(id, name) {
var w = window.open("", //url
name,
"resizable,scrollbars,status,width=800,height=450");
d = w.document;
d.write("<pre>");
d.write(html_escape(output_list[id]));
d.write("\n");
d.write("<a href='javascript:window.close()'>close</a>\n");
d.write("</pre>\n");
d.close();
}
*/
--></script>
<div class='heading'>
<h1>测试报告</h1>
<p class='attribute'><strong>Start Time:</strong> 2022-03-07 22:09:08</p>
<p class='attribute'><strong>Duration:</strong> 0:01:33.613236</p>
<p class='attribute'><strong>Status:</strong> Pass 13</p>
<p class='description'>用例执行情况</p>
</div>
<p id='show_detail_line'>Show
<a href='javascript:showCase(0)'>Summary</a>
<a href='javascript:showCase(1)'>Failed</a>
<a href='javascript:showCase(2)'>All</a>
</p>
<table id='result_table'>
<colgroup>
<col align='left' />
<col align='right' />
<col align='right' />
<col align='right' />
<col align='right' />
<col align='right' />
</colgroup>
<tr id='header_row'>
<td>Test Group/Test case</td>
<td>Count</td>
<td>Pass</td>
<td>Fail</td>
<td>Error</td>
<td>View</td>
</tr>
<tr class='passClass'>
<td>unittest_01_register.Register</td>
<td>6</td>
<td>6</td>
<td>0</td>
<td>0</td>
<td><a href="javascript:showClassDetail('c1',6)">Detail</a></td>
</tr>
<tr id='pt1.1' class='hiddenRow'>
<td class='none'><div class='testcase'>test_01_register_username</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt1.2' class='hiddenRow'>
<td class='none'><div class='testcase'>test_02_register_nickname</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt1.3' class='hiddenRow'>
<td class='none'><div class='testcase'>test_03_register_avatar</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt1.4' class='hiddenRow'>
<td class='none'><div class='testcase'>test_04_register_git</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt1.5' class='hiddenRow'>
<td class='none'><div class='testcase'>test_05_register_git</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt1.6' class='hiddenRow'>
<td class='none'><div class='testcase'>test_06_register_git</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr class='passClass'>
<td>unittest_02_login.Login</td>
<td>6</td>
<td>6</td>
<td>0</td>
<td>0</td>
<td><a href="javascript:showClassDetail('c2',6)">Detail</a></td>
</tr>
<tr id='pt2.1' class='hiddenRow'>
<td class='none'><div class='testcase'>test_07_login_username</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt2.2' class='hiddenRow'>
<td class='none'><div class='testcase'>test_08_login_username</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt2.3' class='hiddenRow'>
<td class='none'><div class='testcase'>test_09_register_password</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt2.4' class='hiddenRow'>
<td class='none'><div class='testcase'>test_10_register_password</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt2.5' class='hiddenRow'>
<td class='none'><div class='testcase'>test_11_register_avatar</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='pt2.6' class='hiddenRow'>
<td class='none'><div class='testcase'>test_12_register_avatar</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr class='passClass'>
<td>unittest_03_WriteBlog.Login</td>
<td>1</td>
<td>1</td>
<td>0</td>
<td>0</td>
<td><a href="javascript:showClassDetail('c3',1)">Detail</a></td>
</tr>
<tr id='pt3.1' class='hiddenRow'>
<td class='none'><div class='testcase'>test_13_Write</div></td>
<td colspan='5' align='center'>pass</td>
</tr>
<tr id='total_row'>
<td>Total</td>
<td>13</td>
<td>13</td>
<td>0</td>
<td>0</td>
<td> </td>
</tr>
</table>
<div id='ending'> </div>
</body>
</html>
?自动化测试报告截图
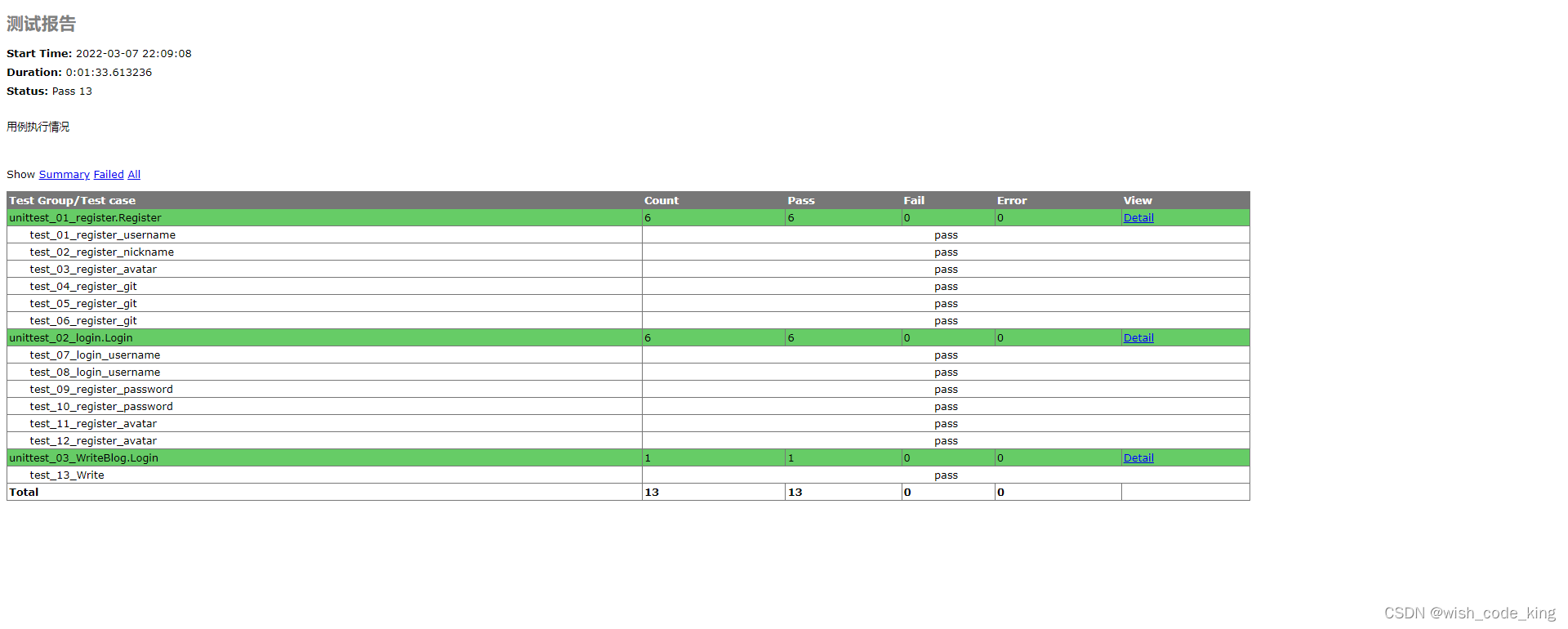
|