1、Maven依赖
<!-- json转换依赖 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.78</version>
</dependency>
<!-- 发送http请求依赖 -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
2、HttpClientUtils工具类
public class HttpClientUtils {
private static final String ENCODING = "UTF-8";
private static final Integer CONNECT_TIMEOUT = 6000;
private static final Integer CONNECTION_REQUEST_TIMEOUT = 6000;
private static final Integer SOCKET_TIMEOUT = 6000;
public static String doGet(String url) throws Exception {
return doGet(url, null, null);
}
public static String doGet(String url, Map<String, String> params) {
return doGet(url, null, params);
}
public static String doGet(String url, Map<String, String> headers, Map<String, String> params) {
CloseableHttpClient client = HttpClients.createDefault();
CloseableHttpResponse response = null;
String result = null;
try {
URIBuilder uriBuilder = handleParam(url, params);
HttpGet httpGet = new HttpGet(uriBuilder.build());
handleConfig(httpGet);
handleHeader(headers, httpGet);
response = client.execute(httpGet);
result = EntityUtils.toString(response.getEntity(), ENCODING);
} catch (Exception e) {
e.getMessage();
} finally {
release(client, response);
}
return result;
}
public static String doPost(String url, Map<String, String> headers) {
return doPost(url, headers, null, null);
}
public static String doPost(String url, Map<String, String> headers, Map<String, String> params) {
return doPost(url, headers, params, null);
}
public static String doPost(String url, Map<String, String> headers, String body) {
return doPost(url, headers, null, body);
}
public static String doPost(String url, Map<String, String> headers, Map<String, String> params, String body) {
CloseableHttpClient client = HttpClients.createDefault();
CloseableHttpResponse response = null;
String result = null;
try {
URIBuilder uriBuilder = handleParam(url, params);
HttpPost httpPost = new HttpPost(uriBuilder.build());
handleConfig(httpPost);
handleHeader(headers, httpPost);
handleBody(body, httpPost);
response = client.execute(httpPost);
result = EntityUtils.toString(response.getEntity(), ENCODING);
} catch (Exception e) {
e.printStackTrace();
} finally {
release(client, response);
}
return result;
}
public static String doDelete(String url) throws IOException {
return doDelete(url, null, null);
}
public static String doDelete(String url, Map<String, String> headers, Map<String, String> params) {
CloseableHttpClient client = HttpClients.createDefault();
CloseableHttpResponse response = null;
String result = null;
try {
URIBuilder uriBuilder = handleParam(url, params);
HttpDelete httpDelete = new HttpDelete(uriBuilder.build());
handleConfig(httpDelete);
handleHeader(headers, httpDelete);
response = client.execute(httpDelete);
result = EntityUtils.toString(response.getEntity(), ENCODING);
} catch (Exception e) {
e.printStackTrace();
} finally {
release(client, response);
}
return result;
}
private static URIBuilder handleParam(String url, Map<String, String> params) throws URISyntaxException {
URIBuilder uriBuilder = new URIBuilder(url);
if (params != null) {
Set<Map.Entry<String, String>> entrySet = params.entrySet();
for (Map.Entry<String, String> entry : entrySet) {
uriBuilder.setParameter(entry.getKey(), entry.getValue());
}
}
return uriBuilder;
}
private static void handleConfig(HttpRequestBase httpMethod) {
if (httpMethod != null) {
RequestConfig requestConfig = RequestConfig.custom().setConnectTimeout(CONNECT_TIMEOUT).setConnectionRequestTimeout(CONNECTION_REQUEST_TIMEOUT).setSocketTimeout(SOCKET_TIMEOUT).build();
httpMethod.setConfig(requestConfig);
}
}
private static void handleHeader(Map<String, String> params, HttpRequestBase httpMethod) {
if (params != null) {
Set<Map.Entry<String, String>> entrySet = params.entrySet();
for (Map.Entry<String, String> entry : entrySet) {
httpMethod.setHeader(entry.getKey(), entry.getValue());
}
}
}
private static void handleBody(String body, HttpPost httpPost) {
if (body != null) {
httpPost.setEntity(new StringEntity(body, ENCODING));
}
}
private static void release(CloseableHttpClient client, CloseableHttpResponse response) {
if (client != null) {
try {
client.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (response != null) {
try {
response.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
3、Get请求方式举例
代码:
public class Test {
public static void main(String[] args) throws Exception {
System.out.println(HttpClientUtils.doGet("http://www.baidu.com"));
}
}
结果:
<!DOCTYPE html><!--STATUS OK--><html> <head><meta http-equiv=content-type content=text/html;charset=utf-8><meta http-equiv=X-UA-Compatible content=IE=Edge><meta content=always name=referrer><link rel=stylesheet type=text/css href=http://s1.bdstatic.com/r/www/cache/bdorz/baidu.min.css><title>百度一下,你就知道</title></head> <body link=#0000cc> <div id=wrapper> <div id=head> <div class=head_wrapper> <div class=s_form> <div class=s_form_wrapper> <div id=lg> <img hidefocus=true src=
4、POST请求方式举例
例1:获取token
代码
public class Test {
public static void main(String[] args) {
System.out.println("token:" + doPost("接口地址", "root", "123456", "default"));
}
private static String doPost(String apiUrl, String username, String password, String tenantUrl) {
String idToken = null;
Map<String, String> headers = new HashMap<>();
headers.put("Content-Type", "application/json;charset=utf-8");
headers.put("Accept", "application/json;charset=utf-8");
JSONObject json = new JSONObject();
json.put("username", username);
json.put("password", DigestUtils.md5Hex(password));
json.put("tenantUrl", tenantUrl);
String result = HttpClientUtils.doPost(apiUrl, headers, json.toJSONString());
if (result != null && !"".equals(result.trim())) {
JSONObject rjo = JSONObject.parseObject(result, Feature.OrderedField);
if (rjo != null && rjo.containsKey("retCode")) {
int retCode = rjo.getInteger("retCode");
if (retCode == 0 && rjo.containsKey("data")) {
JSONObject data = rjo.getJSONObject("data");
if (null != data && data.containsKey("idToken")) {
idToken = data.getString("idToken");
}
}
}
}
return idToken;
}
}
结果
token:853b7c87-c16f-4e3b-96c4-9701fe3448ab
接口文档
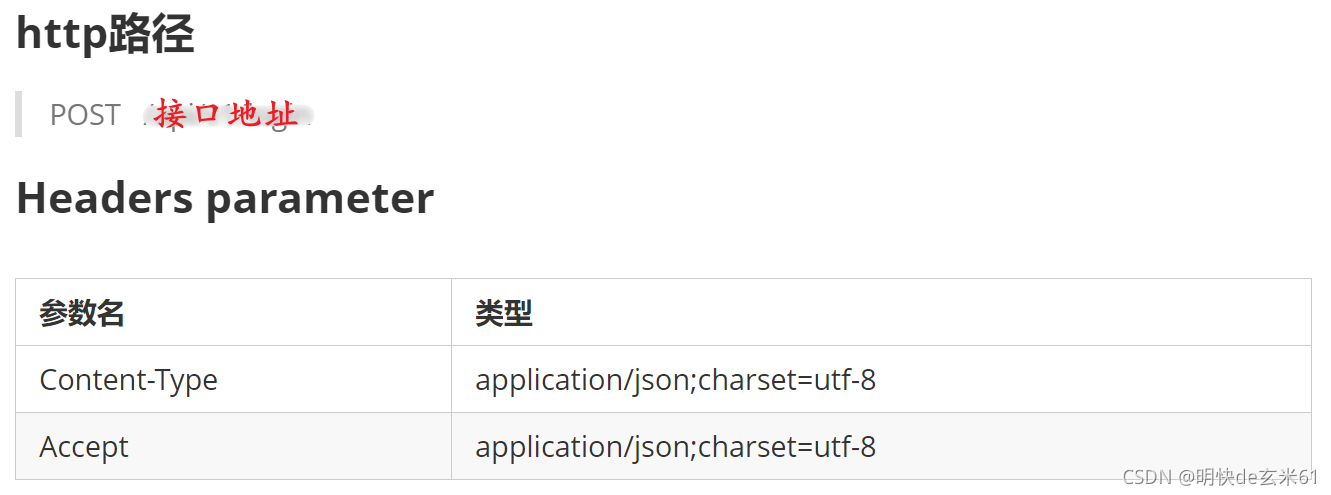 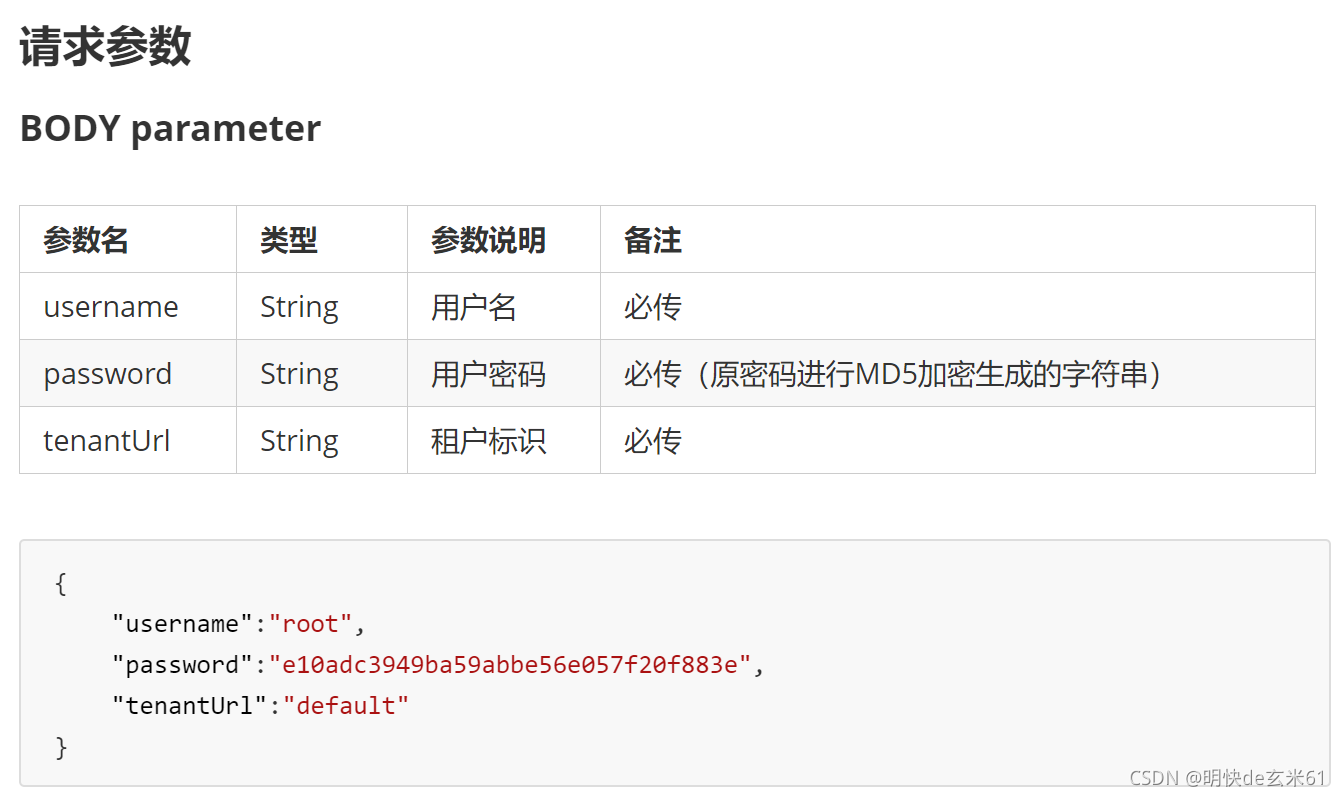 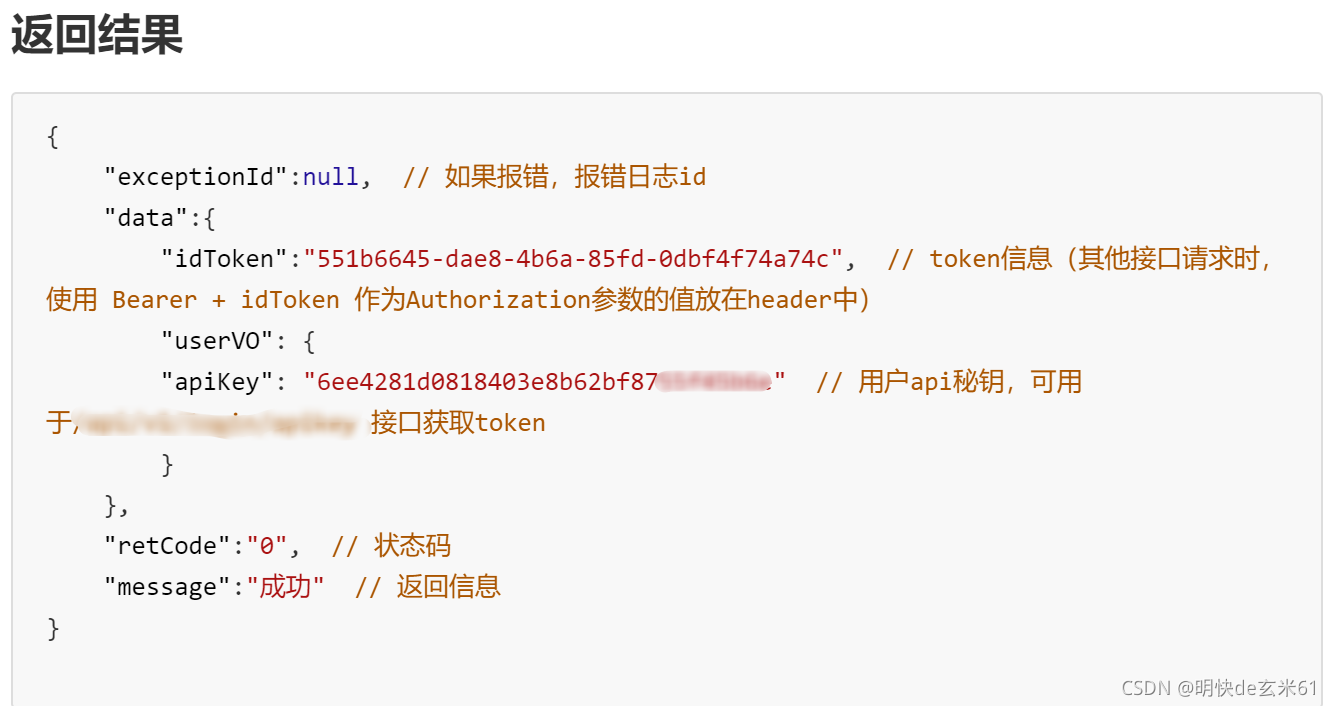
Postman访问示例
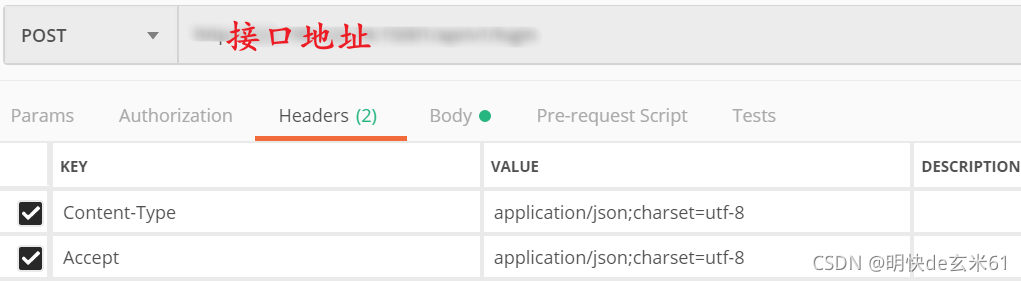 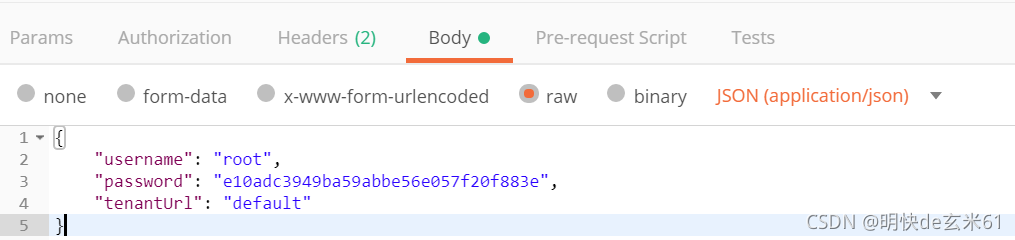
5、拓展
1、http协议切换为https协议(视情况使用,也不是所有https协议都要用这种方式)
如果http协议变成了https协议,我们需要更换HttpCloseableHttpClient的获取方式,我们先创建一个DefaultSSLUtils工具类,如下:
public class DefaultSSLUtils extends DefaultHttpClient {
public DefaultSSLUtils() throws Exception {
super();
SSLContext ctx = SSLContext.getInstance("TLS");
X509TrustManager tm = new X509TrustManager() {
@Override
public void checkClientTrusted(X509Certificate[] chain,
String authType) throws CertificateException {
}
@Override
public void checkServerTrusted(X509Certificate[] chain,
String authType) throws CertificateException {
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return null;
}
};
ctx.init(null, new TrustManager[]{tm}, null);
SSLSocketFactory ssf = new SSLSocketFactory(ctx, SSLSocketFactory.ALLOW_ALL_HOSTNAME_VERIFIER);
ClientConnectionManager ccm = this.getConnectionManager();
SchemeRegistry sr = ccm.getSchemeRegistry();
sr.register(new Scheme("https", 443, ssf));
}
}
在获取HttpCloseableHttpClient对象的时候直接使用如下代码:
CloseableHttpClient httpclient = new DefaultSSLUtils();
|