1. 简介
jackson1.x版本已经停止维护 jackson2.x版本仍在在维护 2.x不兼容1.x jackson老版本的包路径:org.codehaus.jackson jackson新版本的包路径是:com.fasterxml.jackson
jackson新版本主要包含3个模块:
- jackson-core
- jackson-annotations
- jackson-databind databind 依赖 jackson-core, jack-annotations
maven仓库的jar包如下: 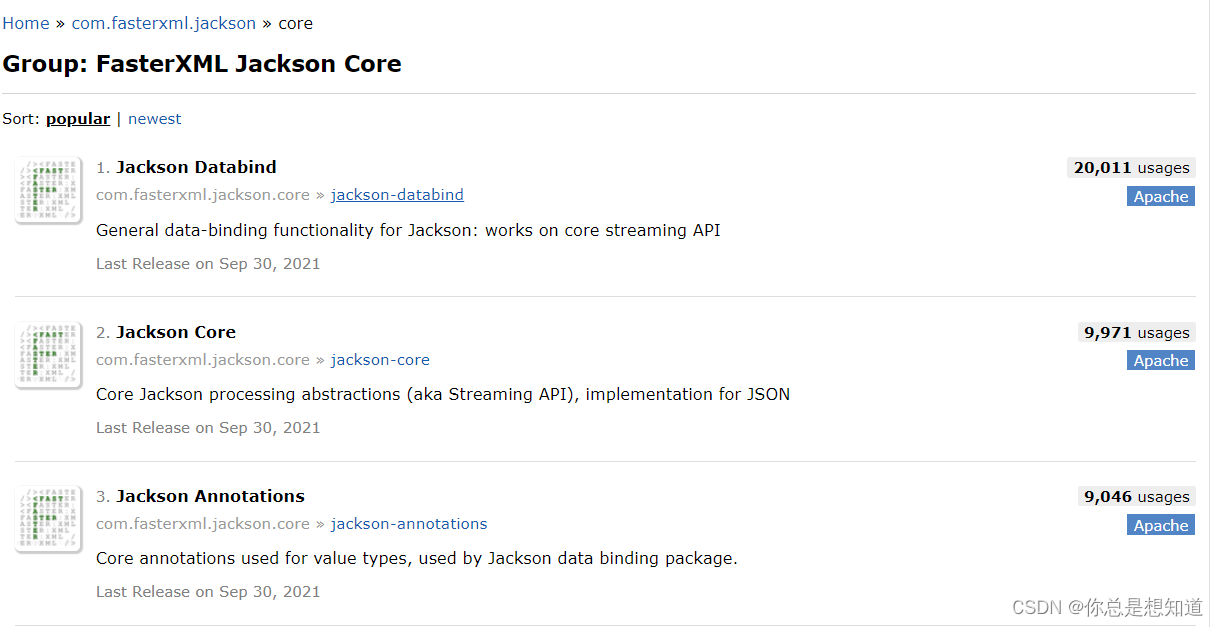
2.基本使用
2.1 jar包引入
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.13.0</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
</dependency>
2.2 封装工具类
public class JacksonUtil {
private static final ObjectMapper objectMapper;
static {
objectMapper = new ObjectMapper();
}
public static <T> T json2Object(String json, Class<T> cls) {
T t = null;
try {
t = objectMapper.readValue(json, cls);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
return t;
}
public static String object2Json(Object obj) {
String json = null;
try {
json = objectMapper.writeValueAsString(obj);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
return json;
}
}
2.3 测试
@Data
@Accessors(chain = true)
public class TestUserGroup {
private Integer id;
private String name;
private List<TestUser> users;
}
@Data
@Accessors(chain = true)
public class TestUser {
private Integer id;
private String name;
private Integer age;
private Date createTime;
}
public class TestJackson {
public static void main(String[] args) {
TestUser testUser01 = new TestUser().setId(1).setAge(23).setName("用户一").setCreateTime(new Date());
TestUser testUser02 = new TestUser().setId(2).setAge(34).setName("用户二");
List<TestUser> testUsers = new ArrayList<>();
testUsers.add(testUser01);
testUsers.add(testUser02);
TestUserGroup testUserGroup = new TestUserGroup().setId(1).setName("用户组一").setUsers(testUsers);
String objJsonStr = JacksonUtil.object2Json(testUserGroup);
System.out.println("objectToJson >>>: " + objJsonStr);
TestUserGroup restTestUserGroup = JacksonUtil.json2Object(objJsonStr, TestUserGroup.class);
System.out.println("json2Object >>>: " + restTestUserGroup.toString());
}
}
|