一、测试工具安装
postman安装地址:Download Postman
测试结果:
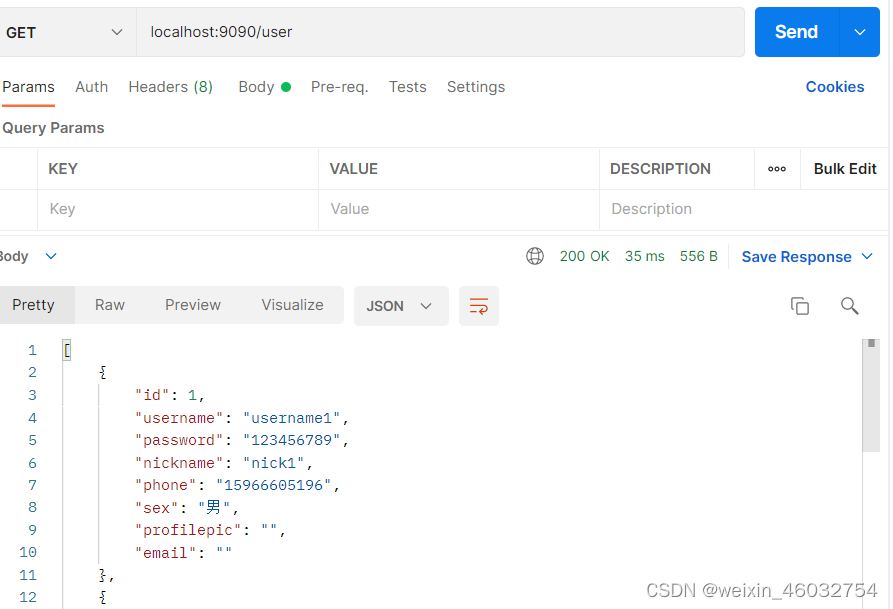
二、完善结构
增加service层,UserService.java,编写save方法根据user的id是否为空执行新增或者修改
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public int save(User user) {
if (user.getId() == null) { // user没有id,则表示是新增
return userMapper.insert(user);
} else { // 否则为更新
return userMapper.update(user);
}
}
}
UserMapper.java,新增插入用户数据的方法,定义修改用户数据方法并在xml中编写sql
@Mapper
public interface UserMapper {
@Select("SELECT * from user")
List<User> findAll();
@Insert("INSERT into user(username, password,nickname,email,phone,sex,profilepic) VALUES (#{username}, #{password}," +
" #{nickname}, #{email},#{phone}, #{sex},#{profilepic})")
int insert(User user);
int update(User user);
@Delete("delete from user where id = #{id}")
Integer deleteById(@Param("id") Integer id);
}
在resources文件夹下新建mapper文件夹,新建User.xml,进行动态sql编写;安装mybatisx插件,方便在mapper文件与xml文件对应方法之间进行跳转
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.springboot.mapper.UserMapper">
<update id="update">
update user
<set>
<if test="username != null">
username = #{username},
</if>
<if test="nickname != null">
nickname = #{nickname},
</if>
<if test="email != null">
email = #{email},
</if>
<if test="phone != null">
phone = #{phone},
</if>
<if test="address != null">
address = #{address}
</if>
<if test="sex != null">
sex = #{sex}
</if>
</set>
<where>
id = #{id}
</where>
</update>
</mapper>
UserController.java,新增接口:传入user对象,将其插入用户表或修改用户表
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserMapper userMapper;
@Autowired
private UserService userService;
// 新增和修改
@PostMapping
public Integer save(@RequestBody User user) {
return userService.save(user);
}
// 查询所有数据
@GetMapping
public List<User> index() {
List<User> all = userMapper.findAll();
return all;
}
@DeleteMapping("/{id}")
public Integer delete(@PathVariable Integer id) {
return userMapper.deleteById(id);
}
}
其中测试新接口时报错:Invalid bound statement (not found): com.example.springboot.mapper.UserMapper.update
错因:未在配置文件中扫描新建的xml
将配置文件application.properties重命名为application.yml,格式更加美观:
server:
port: 9090
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/creative_tool?serverTimezone=GMT%2b8
username: root
password: 123456
mybatis:
mapper-locations: classpath:mapper/*.xml #扫描所有mybatis的xml文件
|