使用懒汉式实现单例模式应用时存在线程的安全性,如果不使用同步锁测试一下是否会创建多个实例,代码如下:
public class Singleton2 {
private static Singleton2 instance = null;
private Singleton2() {
}
public static Singleton2 getInstance() {
if (instance == null) {
instance = new Singleton2();
}
return instance;
}
}
创建一个使用该单例模式的线程,代码如下:
public class MyThread extends Thread {
public void run(){
try {
Singleton2 ss = Singleton2.getInstance();
System.out.println(ss.hashCode());
}catch (Exception ex){
System.out.println(ex.getMessage());
}
}
}
一次创建10个线程,执行这10个线程,测试代码如下:
public class App
{
public static void main( String[] args )
{
Thread[] threads= new Thread[10];
for (int i = 0; i <threads.length ; i++) {
threads[i]= new MyThread();
}
for (int j = 0; j <threads.length ; j++) {
threads[j].start();
}
}
多运行几次,发现实例对象有好几个。 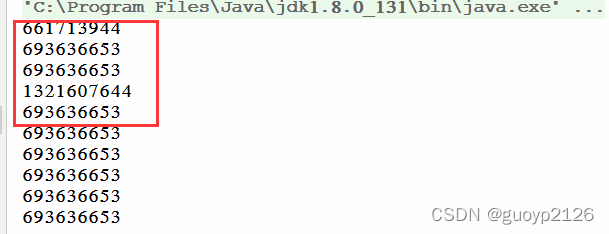 加同步锁单例模式
public class Singleton2 {
private static Singleton2 instance = null;
private Singleton2() {
}
public static synchronized Singleton2 getInstance() {
if (instance == null) {
instance = new Singleton2();
}
return instance;
}
}
双重校验锁单例模式,示例代码如下:
public class SingleTon3 {
private SingleTon3(){};
private static volatile SingleTon3 singleTon=null;
public static SingleTon3 getInstance(){
if(singleTon==null){
synchronized(SingleTon3.class){
if(singleTon==null){
singleTon=new SingleTon3();
}
}
}
return singleTon;
}
|