1.作用
给已知的运算符赋予新的功能 以往所学习的运算符只能进行基本的数据类型运算符,无法实现 两个字符串的相加 , 两个类的相加,两个结构体的相加。 这时候我们就可以利用 c++ 新增的运算符重载的方法赋予运算符新的功能实现这些数据的运算。
2.语法
返回类型说明符 operator 运算符符号(<参数表>)
例如:重载两个 base 类相加的运算符
base operator+(base a)
{
}
- 例子:重置base的加法运算符
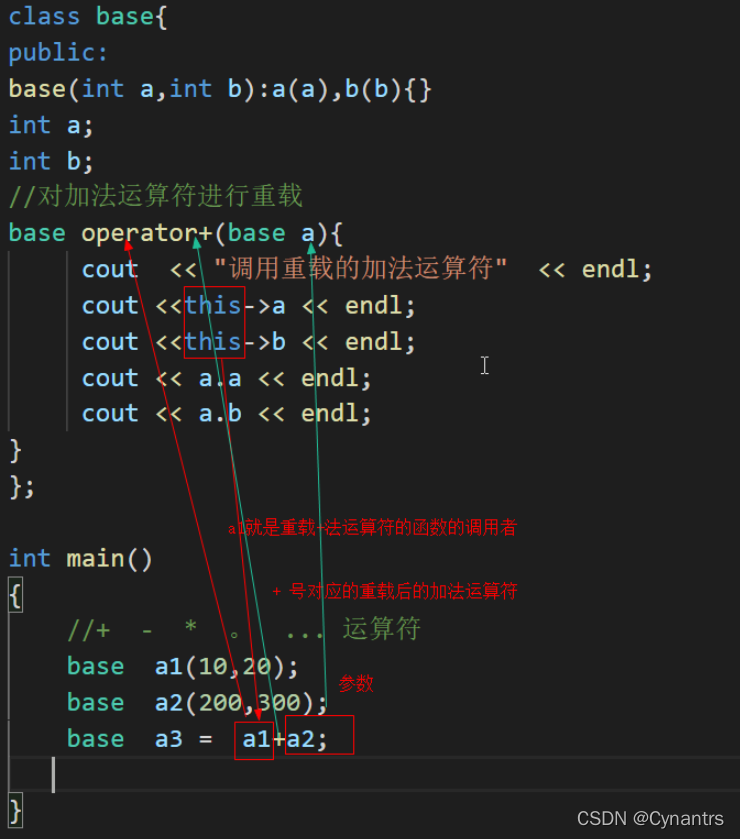 (结果如下) 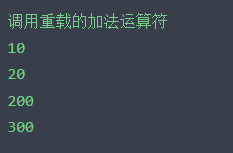
3.可重载运算符与不可重载运算符
1,哪些运算能重载
双目运算符 (+,-,*,/, %) operator+
关系运算符 (==, !=, <, >, <=, >=)
逻辑运算符 (||, &&, !)
单目运算符 (*, &, ++, --)
位运算符 (|, &, ~, ^, <<, >>)
赋值运算符 (=, +=, -=, .....)
空间申请运算符 (new , delete)
其他运算符 ((), ->, [])
2,哪些运算符不能重载
.(成员访问运算符)
.*(成员指针访问运算符)
::(域运算符)
sizeof(数据类型长度运算符)
?:(条件运算符, 三目运算符)
4. 运算符重载的两种方式
类内重载方式:
base operator+(参数列表)
调用规律:
base c = a+b; -> a.operator+(b) 等价于这种调用方式。
特点: a是调用者, b是参数。 因为是在类的内部重载所以有 this 指针
类外重载方式:
base operator+(base a,base b)
调用规律:
base c=a+b; -> operator+(a,b) 等价于这种调用方式。单纯的函数调用
特点: a 和 b 都是 operator+ 函数里面的参数,所以不可以使用 this
5. 不同运算符重载的方式
5.1.关系运算符
class base
{
public:
base(int a):a(a){}
private:
int a;
friend bool operator>(base a,base b);
};
bool operator>(base a,base b)
{
if(a.a > b.a)
{
return true;
}else{
return false;
}
}
int main()
{
base a(100);
base b(20);
if(a > b)
{
cout << "a > b" << endl;
}else{
cout << "a<b" << endl;
}
}
(效果如下) 
5.2. 逻辑运算符
class base
{
public:
base(int a):a(a){}
bool operator&&(base b)
{
if(this->a && b.a)
{
return true;
}else
{
return false;
}
}
private:
int a;
};
5.3. 单目运算符
class base
{
public:
int a;
base(int a):a(a){}
base operator++()
{
cout << "调用++a" << endl;
this->a++;
return *this;
}
base operator++(int)
{
cout << "调用a++" << endl;
base tmp=*this;
this->a++;
return tmp;
}
};
int main()
{
base a(0);
base b=a++;
cout << b.a << endl;
cout << a.a << endl;
}
(结果如下) 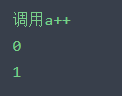
5.4.输出运算符的重载
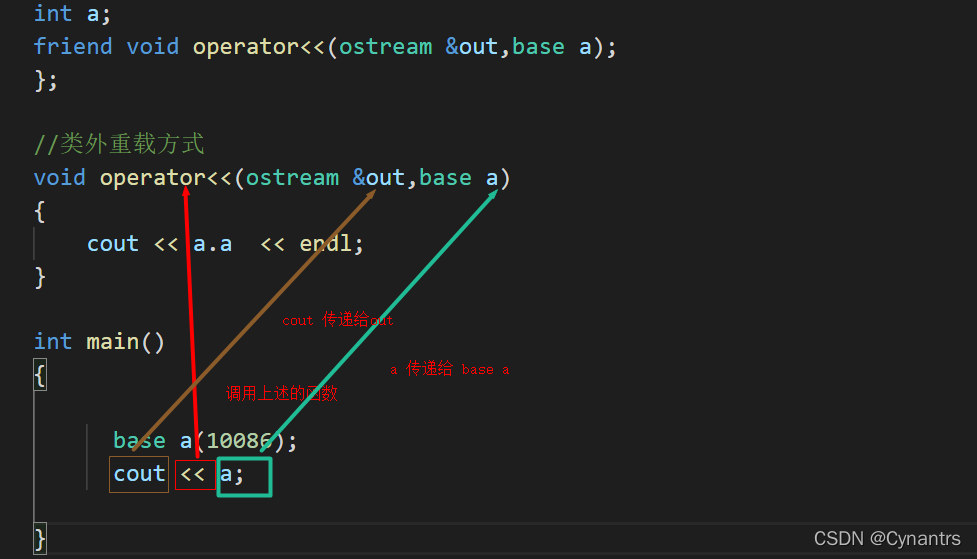
完美重载输出运算符的 demo :
class base
{
public:
base(int a):a(a){}
private:
int a;
friend ostream &operator<<(ostream &out,base a);
};
ostream &operator<<(ostream &out,base a)
{
cout << a.a;
return out;
}
int main()
{
base a(10086);
base b(10010);
cout << a << b << endl;
}
(结果如下) 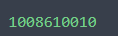
5.5.输入运算符的重载
class base{
public:
base(){}
base(int a):a(a){}
int a;
};
istream & operator>>(istream &in,base &a)
{
cin >> a.a;
return in;
}
int main()
{
base a;
base b;
cin >> a >> b;
cout << a.a << endl;
cout << b.a << endl;
}
|