此文章为本人开发项目中的学习笔记,如有侵权请联系我删除 目录 一、创建步骤: 1、创建类GetReadDataLogInterface继承CtrlServiceAdapter,重写doCtrlService()方法。 2、接收HTTP请求的参数 3、调用后台服务获取数据库连接,处理业务 (1)在/server/package30.xml中注册后台服务 (2)创建ReadDataPluginService类,编写callReadData()方法,在方法中获取数据库连接,写业务。 (3)doCtrlService()方法中调用后台 4、返回一个json对象 5、在/server/package30.xml中注册 6、PostMan调用 二、实现实例
使用HTTP请求调用接口
一、创建步骤: 1、创建类GetReadDataLogInterface继承CtrlServiceAdapter,重写doCtrlService()方法。 2、接收HTTP请求的参数
public static String getPostData(HttpServletRequest request) {
StringBuilder data = new StringBuilder();
String line;
BufferedReader reader;
try {
reader = request.getReader();
while (null != (line = reader.readLine())) {
data.append(line);
}
} catch (IOException e) {
return null;
}
return data.toString();
}
3、调用后台服务获取数据库连接,处理业务 (1)在/server/package30.xml中注册后台服务
<CLSID guid="00000000-2021-0309-1111077000000003" id="ReadDataPluginService" name="报表数据读取服务ctrlService" class="com.pansoft.form.io.newstyle.dal.ReadDataPluginService" ver="1.0.0" type="CLSID_AbstractDataActiveObjectCategory" />
(2)创建ReadDataPluginService类,编写callReadData()方法,在方法中获取数据库连接,写业务。
public class ReadDataPluginService extends JActiveObject{
public JResponseObject getLogById(Object Param, Object o2, Object o3, Object o4) throws Exception{
JParamObject po = (JParamObject)Param;
JConnection conn = null;
Map<String, String> logMap = null;
JResponseObject RO = null;
try{
conn = (JConnection)EAI.DAL.IOM("DBManagerObject", "GetDBConnection", po);
logMap = JReadDataLogService.getLogbyGuIdAndTime(conn,po);
if (null==logMap || logMap.size() <= 0) {
RO = new JResponseObject(logMap, -1);
}else {
RO = new JResponseObject(logMap, 0);
}
}catch (Exception e) {
e.printStackTrace();
return new JResponseObject(e.getMessage(), -1);
} finally {
if(conn!=null){
conn.close();
}
}
return RO;
}
}
(3)doCtrlService()方法中调用后台
JResponseObject ro=(JResponseObject) EAI.DAL.IOM("ReadDataPluginService", "getLogById",PO);
4、返回一个json对象
public static void setResposeData(HttpServletResponse response,JSONObject retJson) {
PrintWriter out;
try {
response.setContentType("application/json;charset=utf-8");
out = response.getWriter();
out.print(retJson);
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
5、在/server/package30.xml中注册
<CtrlService id="CtrlService" caption="">
<ws id="ReadDataLogPluginService" caption="ReadDataLogPluginService" clazz="com.pansoft.form.io.newstyle.dal.GetReadDataLogInterface" action="getIndexReportExecuteStatus"/>
</CtrlService>
id和caption保证唯一性。clazz中配置第一步中创建的类ReadDataInterface的全限定名。
6、PostMan调用 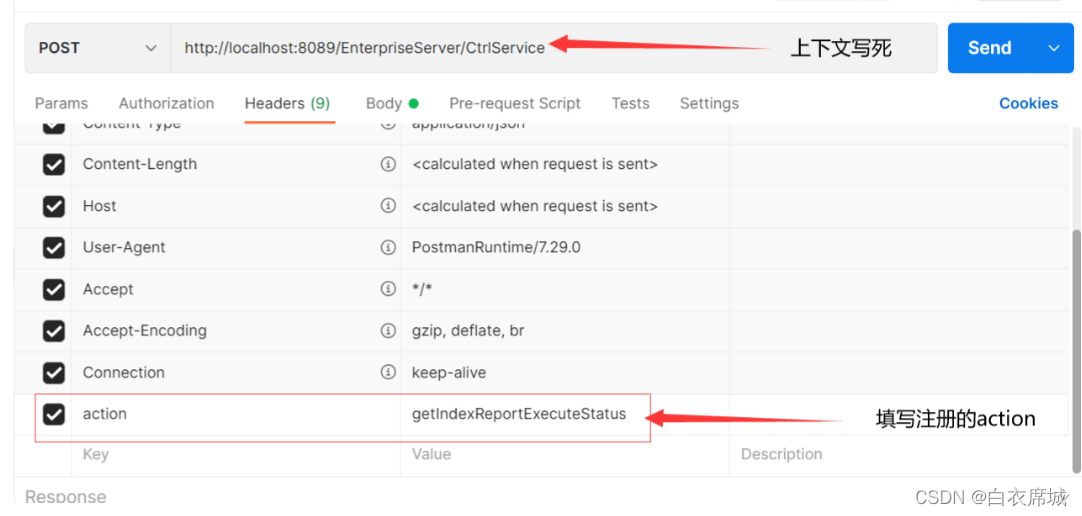 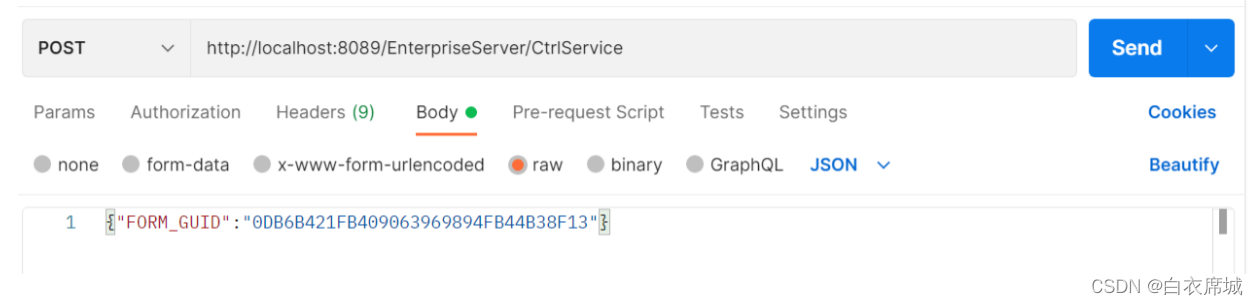
二、实现实例
package com.pansoft.form.io.newstyle.dal;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.lang.StringUtils;
import net.sf.json.JSONObject;
import com.core.xml.StubObject;
import com.efounder.eai.EAI;
import com.efounder.eai.data.JParamObject;
import com.efounder.eai.data.JResponseObject;
import com.efounder.service.ctrl.CtrlServiceAdapter;
import com.efounder.sql.JConnection;
public class GetReadDataLogInterface extends CtrlServiceAdapter{
@Override
public Object doCtrlService(StubObject context, JParamObject PO,
HttpServletRequest request, HttpServletResponse response) throws Exception {
JSONObject retJson = new JSONObject();
if (null == PO) {
PO = JParamObject.Create();
PO.setEAIServer("JJHDFX");
}
try {
String postData=getPostData(request);
if (StringUtils.isNotEmpty(postData)) {
PO.SetValueByParamName("PostParam", postData);
JSONObject jsonObject=JSONObject.fromObject(postData);
PO.SetValueByParamName("FORM_GUID", jsonObject.get("FORM_GUID").toString());
JResponseObject ro=(JResponseObject) EAI.DAL.IOM("ReadDataPluginService", "getLogById",PO);
if(ro==null){
retJson.put("code","-1");
retJson.put("data", jsonObject.get("FORM_GUID").toString());
retJson.put("message","查询失败,无法获取日志信息!");
}else if(ro.getErrorCode()!=0){
retJson.put("code","-1");
retJson.put("data", jsonObject.get("FORM_GUID").toString());
retJson.put("message","查询失败,原因:"+ro.getErrorString());
}else{
retJson.put("code","0");
retJson.put("data", ro.getResponseObject());
retJson.put("message","查询成功!");
}
setResposeData(response,retJson);
}else {
retJson.put("code", "-1");
retJson.put("data", "请输入正确的参数");
retJson.put("message", "查询失败!");
setResposeData(response,retJson);
}
return null;
} catch (Exception ex) {
ex.printStackTrace();
retJson.put("code", "-1");
retJson.put("data", ex.getMessage());
retJson.put("message", "查询失败!");
setResposeData(response,retJson);
return null;
}
}
public static void setResposeData(HttpServletResponse response,JSONObject retJson) {
PrintWriter out;
try {
response.setContentType("application/json;charset=utf-8");
out = response.getWriter();
out.print(retJson);
out.flush();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static String getPostData(HttpServletRequest request) {
StringBuilder data = new StringBuilder();
String line;
BufferedReader reader;
try {
reader = request.getReader();
while (null != (line = reader.readLine())) {
data.append(line);
}
} catch (IOException e) {
return null;
}
return data.toString();
}
}
package com.pansoft.form.io.newstyle.dal;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import net.sf.json.JSONObject;
import com.core.xml.StubObject;
import com.efounder.dbservice.data.AccountStub;
import com.efounder.dbservice.data.DataStorageStub;
import com.efounder.eai.EAI;
import com.efounder.eai.data.JParamObject;
import com.efounder.eai.data.JResponseObject;
import com.efounder.eai.framework.JActiveObject;
import com.efounder.eai.service.dal.JDALDBManagerObject;
import com.efounder.form.convert.ConvertContext;
import com.efounder.service.config.ConfigManager;
import com.efounder.sql.JConnection;
import com.pansoft.form.io.rpt.convert.view.panel.thread.WaitExecuteReadReportDataAPI;
import com.pansoft.modeldefine.dof.dbcon.sybase.JDOFSybaseDBObject;
import com.pansoft.pub.util.UUIDCreator;
public class ReadDataPluginService extends JActiveObject{
public JResponseObject getLogById(Object Param, Object o2, Object o3, Object o4) throws Exception{
JParamObject po = (JParamObject)Param;
JConnection conn = null;
Map<String, String> logMap = null;
JResponseObject RO = null;
try{
conn = (JConnection)EAI.DAL.IOM("DBManagerObject", "GetDBConnection", po);
logMap = JReadDataLogService.getLogbyGuIdAndTime(conn,po);
if (null==logMap || logMap.size() <= 0) {
RO = new JResponseObject(logMap, -1);
}else {
RO = new JResponseObject(logMap, 0);
}
}catch (Exception e) {
e.printStackTrace();
return new JResponseObject(e.getMessage(), -1);
} finally {
if(conn!=null){
conn.close();
}
}
return RO;
}
}
<?xml version="1.0" encoding="gb2312"?>
<package id="" caption="装配清单">
<CLASSES id="" caption="可以在此注册对象,无需修改注册表文件">
<CLSID guid="00000000-2021-0309-1111077000000003" id="ReadDataPluginService" name="报表数据读取服务ctrlService" class="com.pansoft.form.io.newstyle.dal.ReadDataPluginService" ver="1.0.0" type="CLSID_AbstractDataActiveObjectCategory" />
</CLASSES>
<CtrlService id="CtrlService" caption="">
<ws id="ReadDataLogPluginService" caption="ReadDataLogPluginService" clazz="com.pansoft.form.io.newstyle.dal.GetReadDataLogInterface" action="getIndexReportExecuteStatus"/>
</CtrlService>
</package>
|