使用Easyexcel读取excel文件
EasyExcel是一个基于Java的简单、省内存的读写Excel的开源项目。 官网:https://www.yuque.com/easyexcel/doc/easyexcel
1.maven导入
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>3.0.5</version>
</dependency>
2.创建实体类
注解中的名称应与excel表头的名称一致 excel表 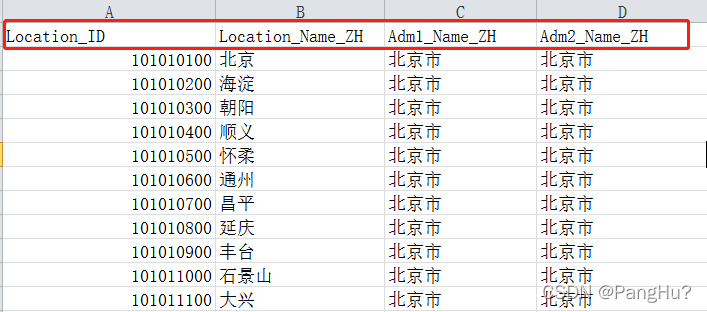 3.创建实体类
@Data
public class WeatherExcelData {
@ExcelProperty("Location_ID")
private String Location_ID;
@ExcelProperty("Location_Name_ZH")
private String locationName;
@ExcelProperty("Adm1_Name_ZH")
private String primaryArea;
@ExcelProperty("Adm2_Name_ZH")
private String SecondArea;
}
4.使用easyexcel读取excel文件后,存入缓存。使用Collator类,重写compare比较方法。使用stream流根据一级地区名,二级地区名分组排序。 此处使用easyexcel最简单的读,更多使用方式可查询官网
public class ResourceController {
private static Map<String, WeatherExcelData> locationMap = new HashMap<>();
private static List<WeatherExcelData> locationList = new ArrayList<>();
private static TreeMap<String, Map<String, List<WeatherExcelData>>> treeMap = new TreeMap();
public void getExcel(){
try{
ClassPathResource resource = new ClassPathResource("China-City-List-latest.xlsx");
EasyExcel.read(resource.getInputStream(), WeatherExcelData.class, new PageReadListener<WeatherExcelData>(dataList -> {
for (WeatherExcelData data : dataList) {
locationMap.put(data.getLocationName() + "", data);
}
locationList.addAll(dataList);
})).sheet().doRead();
putLocationCache(locationList);
}catch (Exception e){
log.error("读取地区文件错误");
}
}
public void putLocationCache(List<WeatherExcelData> locationList) {
if(locationList.size() == 0){
return;
}
TreeMap<String, Map<String, List<WeatherExcelData>>> newLocation = new TreeMap<>(new Comparator<Object>() {
Collator collator = Collator.getInstance(Locale.CHINA);
@Override
public int compare(Object object1, Object object2) {
if (object1 == null || object2 == null){
return 0;
}
CollationKey keyOne = collator.getCollationKey(String.valueOf(object1));
CollationKey keyTwo = collator.getCollationKey(String.valueOf(object2));
return keyOne.compareTo(keyTwo);
}
});
Map<String, Map<String, List<WeatherExcelData>>> location = locationList.stream()
.collect(Collectors.groupingBy(WeatherExcelData::getPrimaryArea,
Collectors.groupingBy(WeatherExcelData::getSecondArea, Collectors.toList())));
for (Map.Entry<String, Map<String, List<WeatherExcelData>>> entry : location.entrySet()) {
newLocation.put(entry.getKey(), entry.getValue());
}
treeMap = newLocation;
System.out.println(treeMap);
}
}
|