下载并安装Gin
下载并安装gin
go get -u github.com/gin-gonic/gin
将gin引入代码中
import "github.com/gin-gonic/gin"
HTML渲染
创建一个存放模板的templates文件夹,然后在内部写入一个index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>
账号是:{{.name}}
</h1>
<h1>
密码是:{{.pwd}}
</h1>
</body>
</html>
在gin框架中使用LoadHTMLLGlob() 或者LoadHTMLFiles() 来渲染HTML模板
func main() {
r := gin.Default()
r.LoadHTMLGlob("./templates/*")
r.GET("/demo",func(c *gin.Context) {
c.HTML(http.StatusOK,"index.html",gin.H{
"name":"admin",
"pwd":"123456",
})
})
r.Run()
}
获取参数
获取Query参数
通过Query来获取URL中? 后面所携带的参数。例如/?name=admin&pwd=123456
func main() {
r := gin.Default()
r.GET("/", func(c *gin.Context) {
name := c.Query("name")
pwd := c.Query("pwd")
c.JSON(http.StatusOK, gin.H{
"name": name,
"pwd": pwd,
})
})
r.Run()
}
发个get请求到http://localhost:8080/?name=admin&pwd=123456 看下效果
获取form参数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form method="post" action="/">
用户名:<input type="text" name="username"><br/>
密码:<input type="password" name="password"><br/>
<input type="submit" value="提交">
</form>
</body>
</html>
func main() {
r := gin.Default()
r.LoadHTMLFiles("./login.html", "./index.html")
r.GET("/", func(c *gin.Context) {
c.HTML(http.StatusOK, "login.html", nil)
})
r.POST("/", func(c *gin.Context) {
username := c.PostForm("username")
password := c.PostForm("password")
c.JSON(http.StatusOK, gin.H{
"name": username,
"pwd": password,
})
})
r.Run()
}
直接访问http://localhost:8080/ 然后提交表单即可
获取path参数
func main() {
r := gin.Default()
r.GET("/user/:username", func(c *gin.Context) {
username := c.Param("username")
c.JSON(http.StatusOK, gin.H{
"username": username,
})
})
r.Run()
}
访问http://localhost:8080/user/admin
这时,username就是admin
获取json参数
func main() {
r := gin.Default()
r.POST("/json", func(c *gin.Context) {
b, _ := c.GetRawData()
var m map[string]interface{}
_ = json.Unmarshal(b, &m)
c.JSON(http.StatusOK, m)
})
r.Run()
}
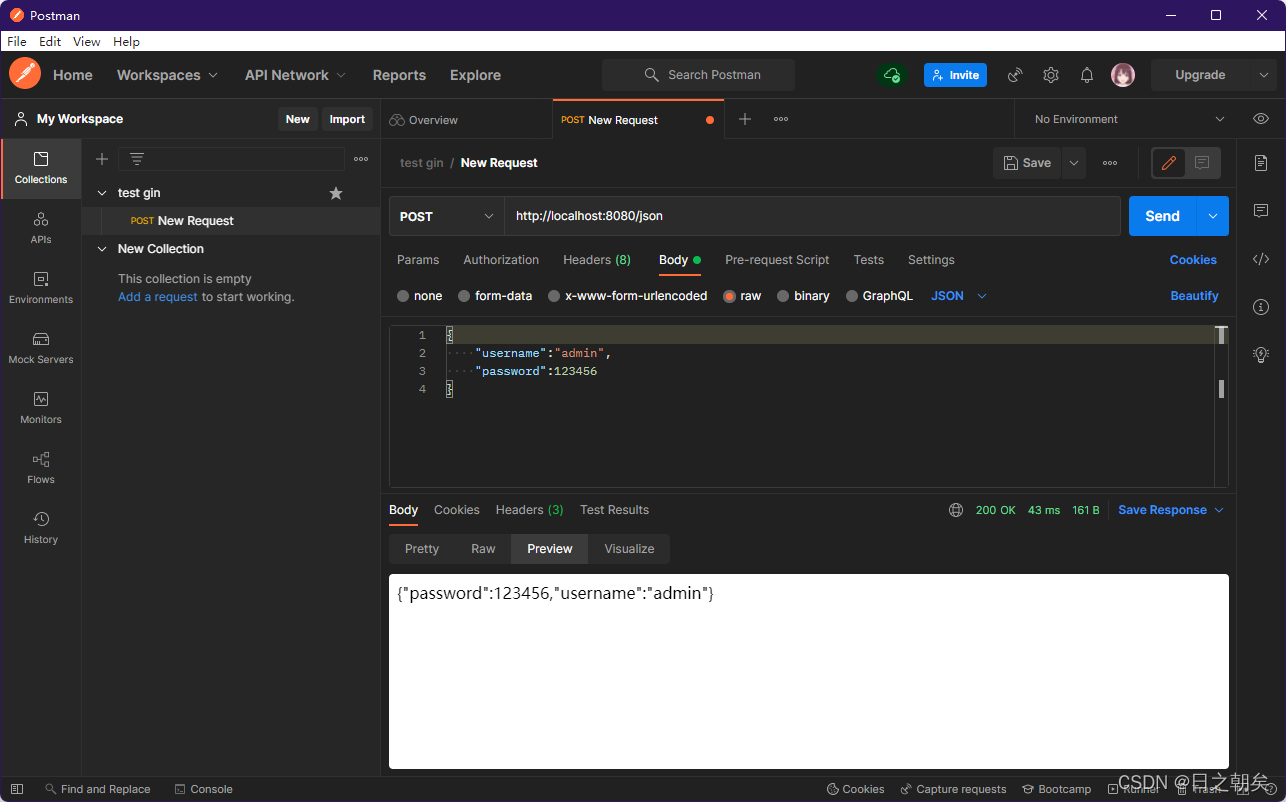
路由
普通路由
r.GET("/get",func(c *gin.Context){})
r.POST("/post",func(c *gin.Context){})
以及匹配所有请求方法的Any方法
r.Any("/test",func(c *gin.Context){})
还有没有匹配到路由的跳转界面
r.NoRoute(func(c *gin.Context){
c.HTML(http.StatusNotFound,"templates/404.html",nil)
})
路由组
将相同前缀URL的路由划分为一个路由组
func main() {
r := gin.Default()
user := r.Group("/user")
user.GET("/index", func(c *gin.Context) {})
user.POST("/login", func(c *gin.Context) {})
r.Run()
}
以及,可以嵌套
func main() {
r := gin.Default()
user := r.Group("/user")
user.GET("/index", func(c *gin.Context) {})
user.POST("/login", func(c *gin.Context) {})
pwd:=user.Group("/pwd")
pwd.GET("/pwd",func(c *gin.Context) {})
r.Run()
}
中间件
func m1(c *gin.Context) {
fmt.Println("m1 in...")
start := time.Now()
c.Abort()
cost := time.Since(start)
fmt.Printf("cost:%v\n", cost)
}
func index(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{
"msg": "ok",
})
}
func main() {
r := gin.Default()
r.GET("/", m1, index)
r.Run()
}
在中间件中使用Goroutine
注意不能使用原始上下文,必须拷贝一份用
func main(){
r := gin.Default()
r.GET("/test1", func(c *gin.Context) {
temp := c.Copy()
go func() {
time.Sleep(5 * time.Second)
log.Println("Done! in path " + temp.Request.URL.Path)
}()
})
r.Run()
}
参数绑定
参数绑定主要使用了函数ShouldBind()
type UserInfo struct{
Username string `form:"username"`
Password string `form:"password"`
}
func main(){
r := gin.Default()
r.GET("/user",func(c *gin.Context){
var u UserInfo
err := c.ShouldBind(&u)
if err != nil{
c.JSON(http.StatusBadGateway, gin.H{
"error":err.Error(),
})
}else{
c.JSON(http.StatusOk, gin.H{
"message":"ok"
})
}
fmt.Println(u)
})
r.Run()
}
ShouldBind会按照以下顺序解析请求中的数据并完成绑定:
- 如果是GET请求,只使用Form绑定引擎(Query)
- 如果是POST请求,首先检查content-type是否为JSON或XML,然后再使用Form(form-data)
文件上传
单文件上传
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<title>上传文件示例</title>
</head>
<body>
<form action="http://localhost:8080/upload" method="post" enctype="multipart/form-data">
<input type="file" name="upload" multiple>
<input type="submit" value="上传">
</form>
</body>
</html>
func main(){
r := gin.Default()
r.POST("/upload",func(c *gin.Context){
file, err := c.FormFile("upload")
if err != nil{
c.JSON(http.StatusInternalServerError, gin.H{
"message":err.Error(),
})
return
}
log.Println(file.Filename)
dst := fmt.Sprintf("D:/testFiles/%s",file.Filename)
c.SaveUploadedFile(file,dst)
c.JSON(http.StatusOk,gin.H{
"message": fmt.Sprintf("'%s' uploaded!",file.Filename),
})
})
r.Run()
}
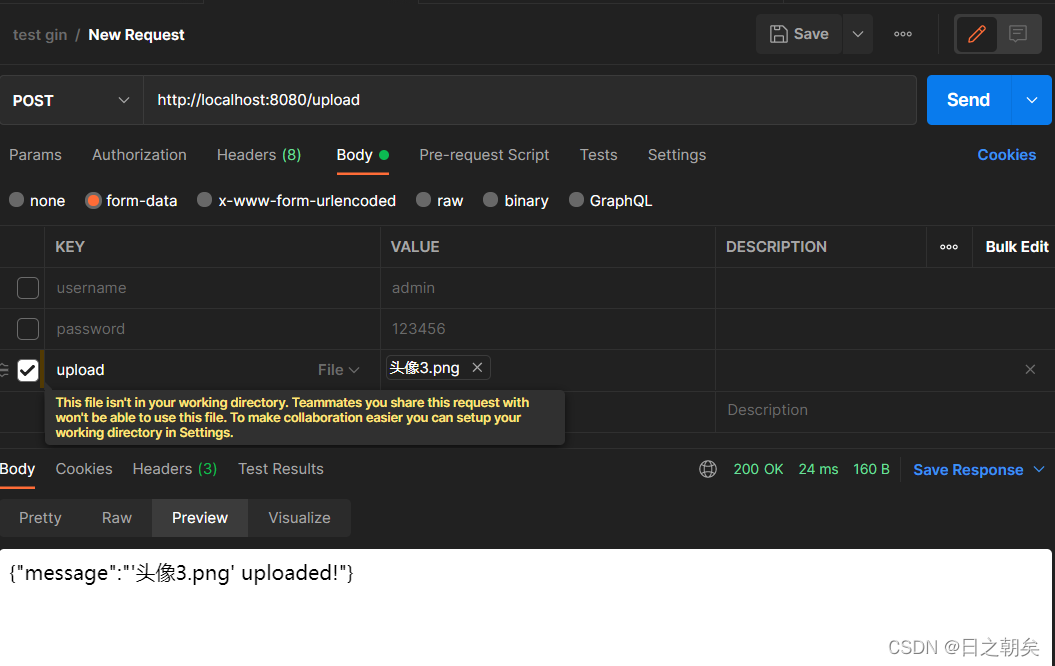
多文件上传
func main(){
r := gin.Default()
r.POST("/upload",func(c *gin.Context){
form, _ := c.MultipartForm()
files := form.File["file"]
for index, file := range files{
log.Println(file.Filename)
dst := fmt.Sprintf("")
c.SaveUploadedFile(file,dst)
}
c.JSON(200,gin.H{
"message": "files uploaded! "
})
})
r.Run()
}
重定向
func main() {
r := gin.Default()
r.GET("/test", func(c *gin.Context) {
c.Redirect(http.StatusMovedPermanently, "https://www.baidu.com/")
})
r.Run()
}
或路由重定向
func main() {
r := gin.Default()
r.GET("/test1", func(c *gin.Context) {
c.Request.URL.Path = "/test2"
r.HandleContext(c)
})
r.GET("/test2", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"hello": "world"})
})
r.Run()
}
cookie
func main() {
r := gin.Default()
r.GET("/cookie", func(c *gin.Context) {
cookie, err := c.Cookie("gin_cookie")
if err != nil {
cookie = "NotSet"
c.SetCookie("gin_cookie", "test", 3600, "/", "localhost", false, true)
}
})
r.Run()
}
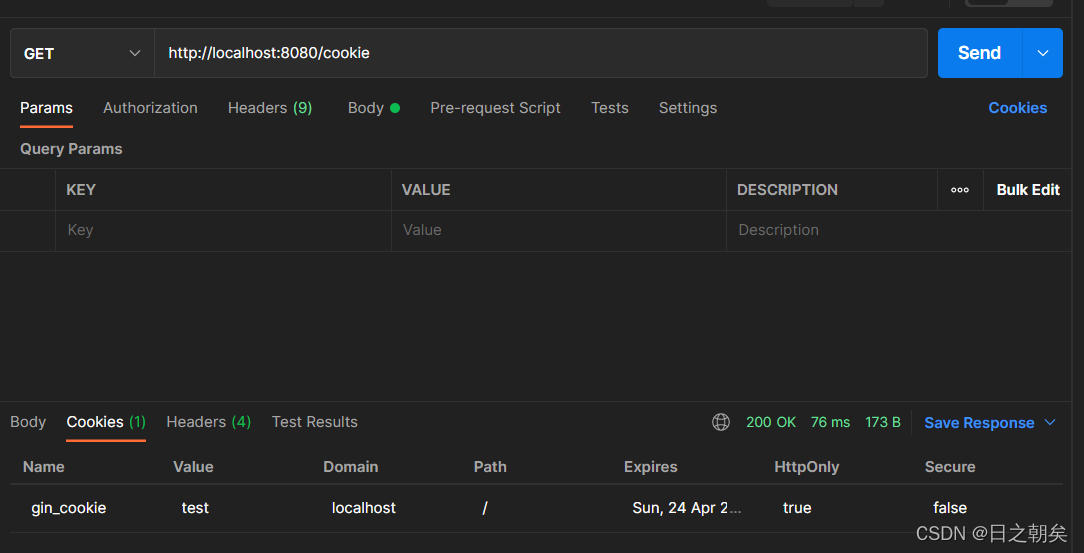
绑定html复选框
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="/" method="POST">
<p>Check some colors</p>
<label for="red">Red</label>
<input type="checkbox" name="colors[]" value="red" id="red" />
<label for="green">Green</label>
<input type="checkbox" name="colors[]" value="green" id="green" />
<label for="blue">Blue</label>
<input type="checkbox" name="colors[]" value="blue" id="blue" />
<input type="submit" />
</form>
</body>
</html>
type myForm struct {
Colors []string `form:"colors[]"`
}
func main() {
r := gin.Default()
r.LoadHTMLGlob("views/*")
r.GET("/", indexHandler)
r.POST("/", formHandler)
r.Run(":8080")
}
func indexHandler(c *gin.Context) {
c.HTML(200, "form.html", nil)
}
func formHandler(c *gin.Context) {
var fakeForm myForm
c.Bind(&fakeForm)
c.JSON(200, gin.H{"color": fakeForm.Colors})
}
|