startup里
public void ConfigureServices(IServiceCollection services)
{
try
{
string stsDiscoveryEndpoint = Configuration["AzureAd:Instance"] + "/common/v2.0/.well-known/openid-configuration";
IConfigurationManager<OpenIdConnectConfiguration> configManager = new ConfigurationManager<OpenIdConnectConfiguration>(stsDiscoveryEndpoint, new OpenIdConnectConfigurationRetriever());
OpenIdConnectConfiguration openidconfig = configManager.GetConfigurationAsync(CancellationToken.None).Result;
services.AddAuthentication(sharedoptions =>
{
sharedoptions.DefaultScheme = JwtBearerDefaults.AuthenticationScheme;
})
.AddJwtBearer(options =>
{
options.Authority = Configuration["AzureAd:Instance"] + "/" + Configuration["AzureAd:TenantId"];
options.TokenValidationParameters = new TokenValidationParameters
{
ValidAudience = Configuration["AzureAd:ClientId"],
ValidIssuer = Configuration["AzureAd:Instance"] + Configuration["AzureAd:TenantId"] + "/v2.0",
IssuerSigningKeys = openidconfig.SigningKeys,
ValidateLifetime = true
};
options.Events = new JwtBearerEvents()
{
//收到请求会进来
OnMessageReceived = async c =>
{
var a = c.Request.Headers;
await Task.FromResult(0);
},
//token合法会进来
OnTokenValidated = async c =>
{
var a = c.Request.Headers;
await Task.FromResult(0);
},
//token过期会进来
OnAuthenticationFailed = async c =>
{
var ex1 = c.Exception.Message;
var ex2 = c.Exception.StackTrace;
//Log.Error("JWT Auth failed: " + c.Exception.Message + "\n" + c.Exception.StackTrace);
await Task.FromResult(0);
}
};
});
//services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
//.AddMicrosoftIdentityWebApi(Configuration, "AzureAd");
//;
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "webapi", Version = "v1" });
c.DocInclusionPredicate((docName, description) => true);
c.AddSecurityDefinition("Bearer", new OpenApiSecurityScheme
{
Description = "JWT授权(数据将在请求头中进行传输) 在下方输入Bearer {token} 即可,注意两者之间有空格",
Name = "Authorization",//jwt默认的参数名称
In = ParameterLocation.Header,//jwt默认存放Authorization信息的位置(请求头中)
Type = SecuritySchemeType.ApiKey
});
//认证方式,此方式为全局添加
c.AddSecurityRequirement(new OpenApiSecurityRequirement {
{
new OpenApiSecurityScheme {
Reference = new OpenApiReference() {
Id = "Bearer",
Type = ReferenceType.SecurityScheme
}
}, Array.Empty<string>() }
});
});
}
catch (Exception ex)
{
var ex1 = ex.ToString();
throw ex;
}
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
//这里注意launchSettings.json配置如果启动方式不是IIS Express需要把对应的环境变量调整一下,改成"ASPNETCORE_ENVIRONMENT": "Development",swagger才会出现在项目启动
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "webapi v1"));
}
app.UseHttpsRedirection();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
}
launchSettings.json
{
"$schema": "http://json.schemastore.org/launchsettings.json",
"iisSettings": {
"windowsAuthentication": false,
"anonymousAuthentication": true,
"iisExpress": {
"applicationUrl": "http://localhost:62974",
"sslPort": 44393
}
},
"profiles": {
"IIS Express": {
"commandName": "IISExpress",
"launchBrowser": true,
"launchUrl": "swagger",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"webapi": {
"commandName": "Project",
"dotnetRunMessages": "true",
"launchBrowser": true,
"launchUrl": "swagger",
"applicationUrl": "https://localhost:52484/;http://localhost:5000",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
}
appsetting.json
"AzureAd": {
//"IsEnabled": "true",
"Instance": "https://login.microsoftonline.com/",
"Host": "https://localhost:52484/",
"CallbackPath": "signin-oidc",
"Domain": "...",
"ClientId": "...",
"TenantId": "...",
"ClientSecret": "..."
},
效果图:
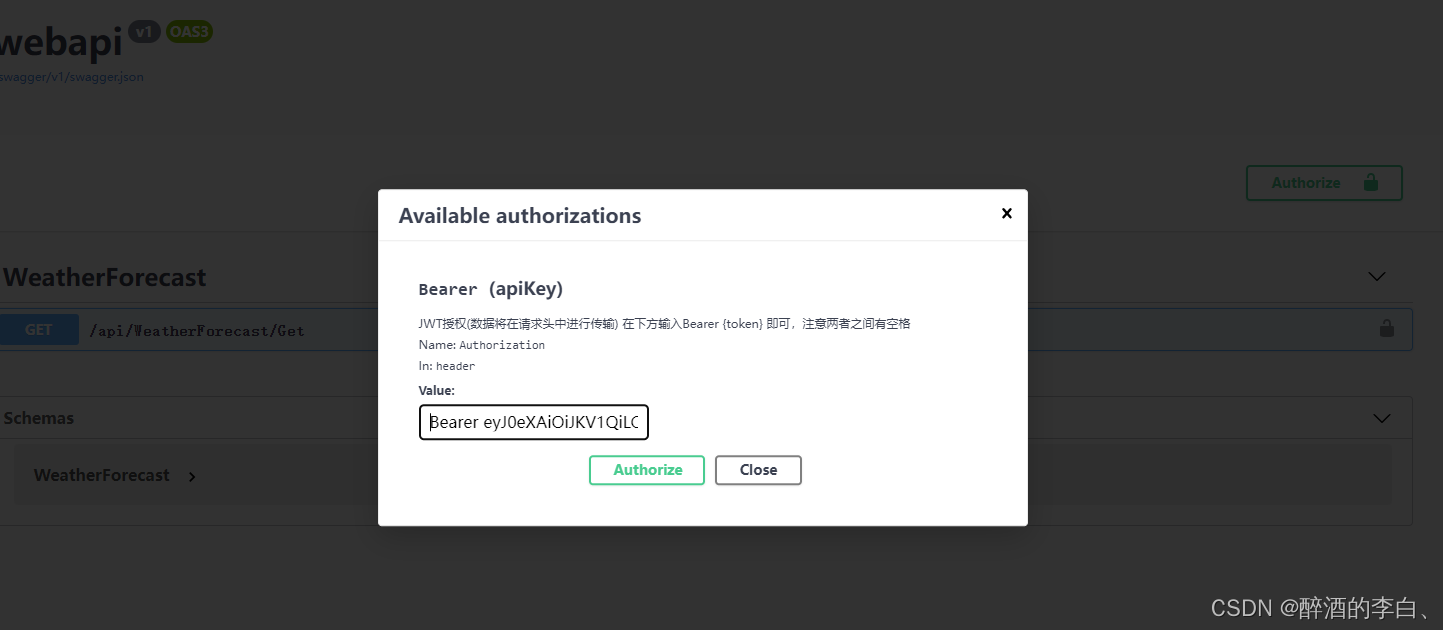
controller测试方法
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Claims;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
using Microsoft.Identity.Web;
using Microsoft.Identity.Web.Resource;
namespace webapi.Controllers
{
//[Authorize(Roles = "HR")]
//[Authorize(Roles = "Admin_hr")]
//[Authorize(Roles = "Hello World!")]
[ApiController]
//[Route("[controller]")]
[Route("api/[controller]/[action]")]
//[ApiController]
[Authorize]
public class WeatherForecastController : ControllerBase
{
private readonly ITokenAcquisition tokenAcquisition;
private static readonly string[] Summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
private readonly ILogger<WeatherForecastController> _logger;
// The Web API will only accept tokens 1) for users, and 2) having the "access_as_user" scope for this API
//static readonly string[] scopeRequiredByApi = new string[] { "access_as_user" };
static readonly string[] scopeRequiredByApi = new string[] { "user_impersonation" };
public WeatherForecastController(ILogger<WeatherForecastController> logger
)
{
_logger = logger;
//this.tokenAcquisition = tokenAcquisition;
}
[HttpGet]
public IEnumerable<WeatherForecast> Get()
{
//HttpContext.VerifyUserHasAnyAcceptedScope(scopeRequiredByApi);
//string[] scopes = new string[] { "user.read" };
//string accessToken = tokenAcquisition.GetAccessTokenForUserAsync(scopes).ToString();
var userId = User.FindFirst(ClaimTypes.Role).Value;
var userName = User.FindFirst("enterpriseID").Value;
var userName1 = User.FindAll("enterpriseID").FirstOrDefault().Value;
var rolelist = User.FindAll(ClaimTypes.Role);
HttpContext.Response.WriteAsync($"测试结果 {userId}---{userName}--{rolelist}");
var rng = new Random();
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = rng.Next(-20, 55),
Summary = Summaries[rng.Next(Summaries.Length)]
})
.ToArray();
}
}
}
?返回结果:
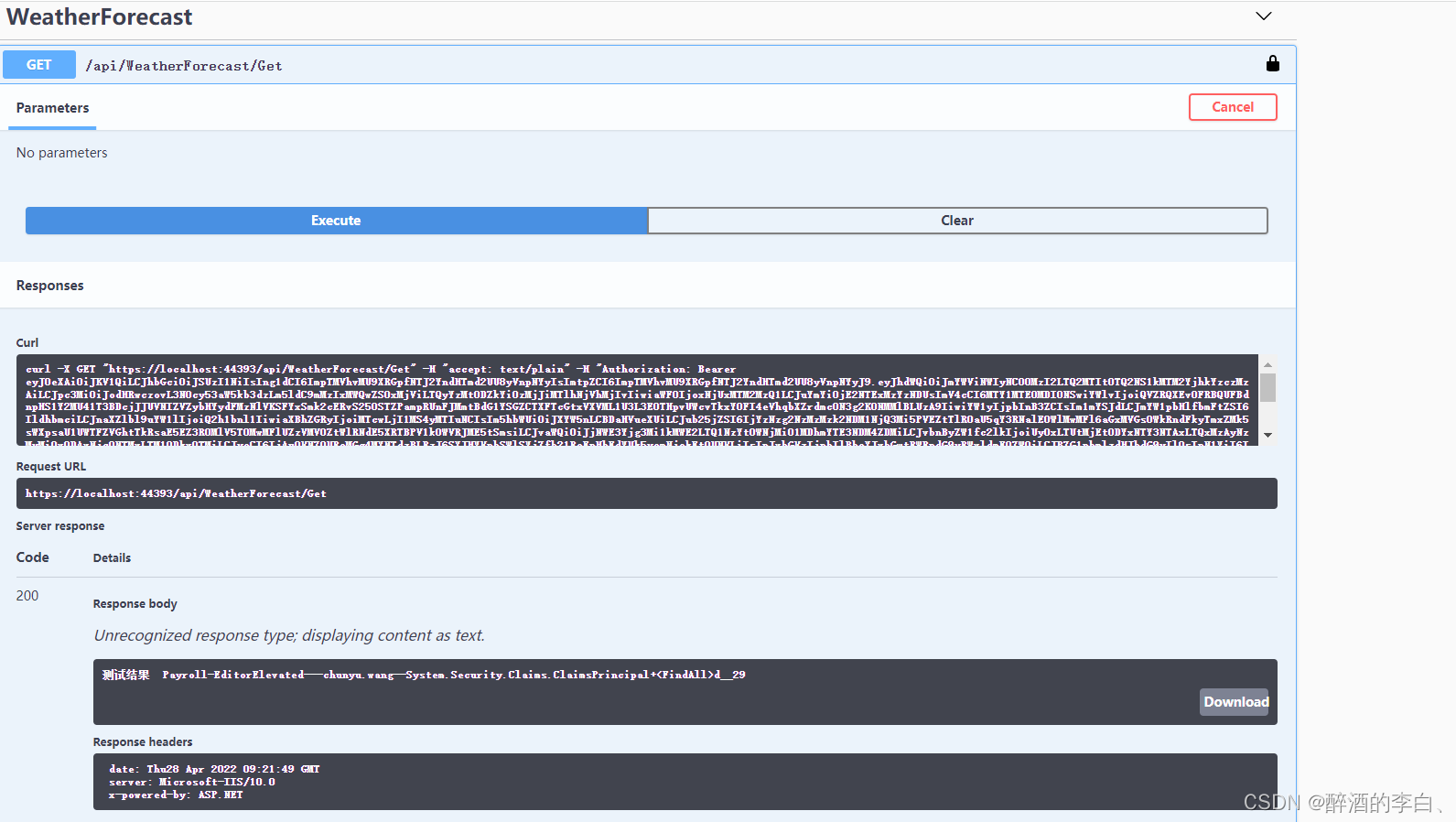
引用包:
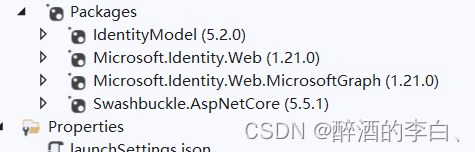
此一套虽然比官方文档麻烦些,但能最快速搭建一个架构并且快速调试出token遇到的问题,因为有事件监听。
仅供学习参考,如有侵权联系我删除?
|