MyBatis
十.复杂查询环境搭建
1.多对一处理
例如:多个学生对应一个老师 对于学生这边而言:关联 多个学生关联一个老师 (多对一) 对于老师这边而言:集合,一个老师有很多学时(一对多)
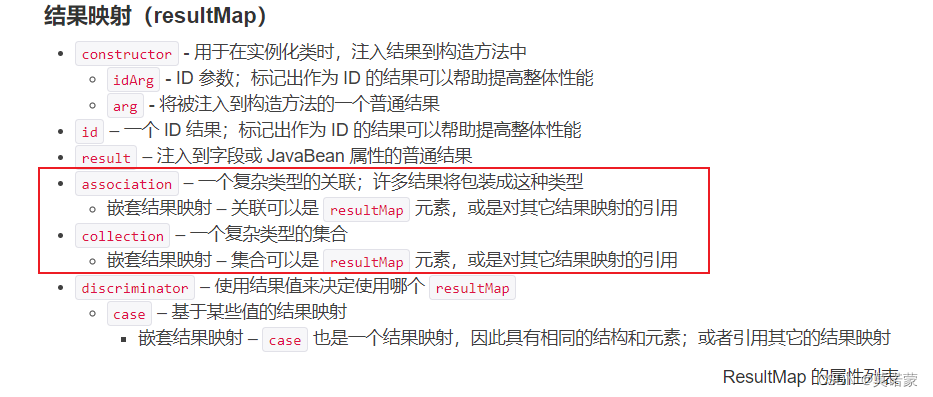 创建数据库:
CREATE TABLE `teacher` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8;
INSERT INTO teacher(`id`, `name`) VALUES (1, '秦老师');
CREATE TABLE `student` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
`tid` INT(10) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `fktid` (`tid`),
CONSTRAINT `fktid` FOREIGN KEY (`tid`) REFERENCES `teacher` (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8;
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('1', '小明', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('2', '小红', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('3', '小张', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('4', '小李', '1');
INSERT INTO `student` (`id`, `name`, `tid`) VALUES ('5', '小王', '1');
测试环境搭建: 结构: 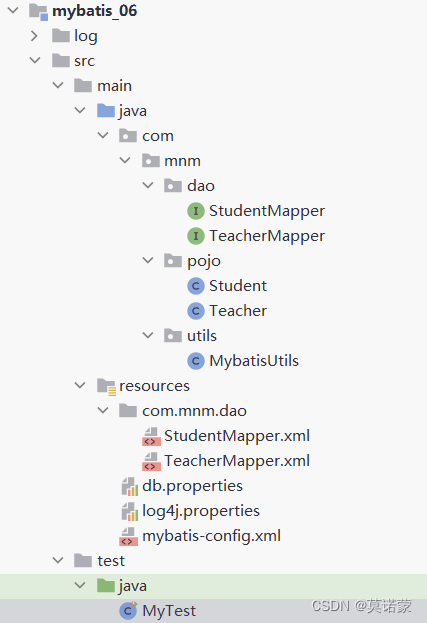
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.24</version>
<scope>provided</scope>
</dependency>
import lombok.Data;
@Data
public class Teacher {
private int id;
private String name;
}
import lombok.Data;
@Data
public class Student {
private int id;
private String name;
private Teacher teacher;
}
以下为:按照查询嵌套处理
import com.mnm.pojo.Teacher;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
public interface TeacherMapper {
@Select("select * from teacher where id = #{tid}")
Teacher getTeacher(@Param("tid") int id);
}
import com.mnm.pojo.Student;
import java.util.List;
public interface StudentMapper {
List<Student> getStudent();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.TeacherMapper">
</mapper>
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.StudentMapper">
<select id="getStudent" resultMap="StudentTeacher">
select * from student;
</select>
<resultMap id="StudentTeacher" type="Student">
<result property="id" column="id"/>
<result property="name" column="name"/>
<association property="teacher" column="tid" javaType="Teacher" select="getTeacher"/>
</resultMap>
<select id="getTeacher" resultType="Teacher">
select * from teacher where id = #{id};
</select>
</mapper>
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="db.properties"/>
<settings>
<setting name="logImpl" value="LOG4J"/>
</settings>
<typeAliases>
<package name="com.mnm.pojo"/>
</typeAliases>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<mappers>
<package name="com.mnm.dao"/>
</mappers>
</configuration>
import com.mnm.dao.StudentMapper;
import com.mnm.dao.TeacherMapper;
import com.mnm.pojo.Student;
import com.mnm.pojo.Teacher;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
import java.util.List;
public class MyTest {
@Test
public void test(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
TeacherMapper mapper = sqlSession.getMapper(TeacherMapper.class);
Teacher teacher = mapper.getTeacher();
System.out.println(teacher);
sqlSession.close();
}
@Test
public void getStudent(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
List<Student> studentList = mapper.getStudent();
for (Student student : studentList) {
System.out.println(student);
}
sqlSession.close();
}
}
以下为按照结果嵌套处理:
<select id="getStudent2" resultMap="StudentTeacher2">
select s.id sid,
s.name sname,
t.id tid,
t.name tname
from student s left join teacher as t on s.tid=t.id
</select>
<resultMap id="StudentTeacher2" type="Student">
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<association property="teacher" javaType="Teacher">
<result property="id" column="tid"/>
<result property="name" column="tname"/>
</association>
</resultMap>
回顾Mysql多对一查询方式:
2.一对多处理
比如:一个老师拥有多个学生 对于老师而言,就是一对多的关系
修改实体类:
import lombok.Data;
@Data
public class Student {
private int id;
private String name;
private int tid;
}
import lombok.Data;
import java.util.List;
@Data
public class Teacher {
private int id;
private String name;
private List<Student> students;
}
按照结果嵌套处理 按照查询嵌套处理
建立mapper接口
import com.mnm.pojo.Teacher;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import java.util.List;
public interface TeacherMapper {
List<Teacher> getTeacher();
Teacher getTeacherStudent(@Param("tid") int id);
Teacher getTeacherStudent2(@Param("tid") int id);
}
创建mapper.xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.TeacherMapper">
<select id="getTeacher" resultType="Teacher">
select * from teacher;
</select>
<select id="getTeacherStudent" resultMap="TeacherStudent">
select t.id tid,
t.name tname,
s.id sid,
s.name sname
from teacher t left join student as s on t.id = s.tid
where t.id = #{tid};
</select>
<resultMap id="TeacherStudent" type="Teacher">
<result property="id" column="tid"/>
<result property="name" column="tname"/>
<collection property="students" ofType="Student">
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<result property="tid" column="tid"/>
</collection>
</resultMap>
<select id="getTeacherStudent2" resultMap="TeacherStudent2">
select * from teacher where id = #{tid}
</select>
<resultMap id="TeacherStudent2" type="Teacher">
<result property="id" column="id"/>
<result property="name" column="name"/>
<collection property="students" javaType="ArrayList" ofType="Student" select="getStudentByTeacherId" column="id"/>
</resultMap>
<select id="getStudentByTeacherId" resultType="Student">
select * from student where tid = #{tid}
</select>
</mapper>
测试:
import com.mnm.dao.StudentMapper;
import com.mnm.dao.TeacherMapper;
import com.mnm.pojo.Student;
import com.mnm.pojo.Teacher;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
import java.util.List;
public class MyTest {
@Test
public void test(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
TeacherMapper mapper = sqlSession.getMapper(TeacherMapper.class);
List<Teacher> teacherList = mapper.getTeacher();
for (Teacher teacher : teacherList) {
System.out.println(teacher);
}
sqlSession.close();
}
@Test
public void getTeacherStudent(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
TeacherMapper mapper = sqlSession.getMapper(TeacherMapper.class);
Teacher teacherStudent = mapper.getTeacherStudent(1);
System.out.println(teacherStudent);
sqlSession.close();
}
@Test
public void getTeacherStudent2(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
TeacherMapper mapper = sqlSession.getMapper(TeacherMapper.class);
Teacher teacherStudent = mapper.getTeacherStudent2(1);
System.out.println(teacherStudent);
sqlSession.close();
}
}
3.小结:
关联: accociation 多对一 集合: collection 一对多
javaType & ofType
- javaType 用来指定实体类中属性的类型
- ofType 用来指定映射到集合中的pojo类型,泛型中的约束类型
注意点:
- 保证sql的可读性 ,尽量保证通俗易懂
- 注意一对多和多对一中,属性名和字段的问题
- 如果问题不好排除错误,可以使用日志
面试高频:
十一.动态SQL
什么是动态SQL:动态SQL就是指,根据不同的条件生成的不同的SQL语句 动态 SQL 是 MyBatis 的强大特性之一。 如果你之前用过 JSTL 或任何基于类 XML 语言的文本处理器,你对动态 SQL 元素可能会感觉似曾相识。在 MyBatis 之前的版本中,需要花时间了解大量的元素。借助功能强大的基于 OGNL 的表达式,MyBatis 3 替换了之前的大部分元素,大大精简了元素种类,现在要学习的元素种类比原来的一半还要少。
- if
- choose (when, otherwise)
- trim (where, set)
- foreach
1.环境搭建
创建数据库:
CREATE TABLE `blog`(
`id` VARCHAR(50) NOT NULL COMMENT '博客id',
`title` VARCHAR(100) NOT NULL COMMENT '博客标题',
`author` VARCHAR(30) NOT NULL COMMENT '博客作者',
`create_time` DATETIME NOT NULL COMMENT '创建时间',
`views` INT(30) NOT NULL COMMENT '浏览量'
)ENGINE=INNODB DEFAULT CHARSET=utf8;
创建基础工程:
import lombok.Data;
import java.util.Date;
@Data
public class Blog {
private int id;
private String title;
private String author;
private Date createTime;
private int views;
}
我们要用到这个配置  项目结构: 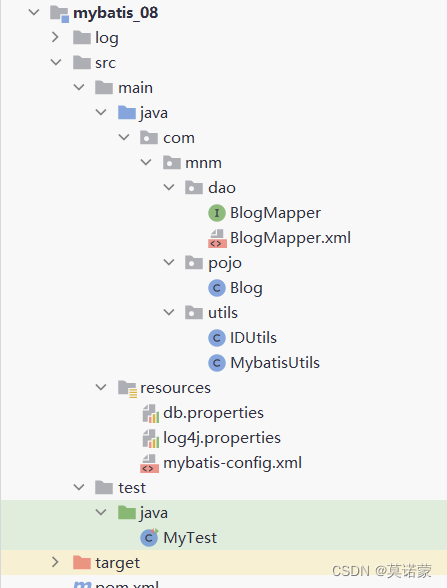
创建构造随机id的类
import java.util.UUID;
public class IDUtils {
public static String getId(){
return UUID.randomUUID().toString().replaceAll("-","");
}
}
创建mapper接口
import com.mnm.pojo.Blog;
public interface BlogMapper {
int addBlog(Blog blog);
}
mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.BlogMapper">
<insert id="addBlog" parameterType="blog">
insert into blog (id, title, author, create_time, views)
VALUES (#{id},#{title},#{author},#{createTime},#{views});
</insert>
</mapper>
mybatis-config.xml配置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="db.properties"/>
<settings>
<setting name="logImpl" value="LOG4J"/>
<setting name="mapUnderscoreToCamelCase" value="true"/>
</settings>
<typeAliases>
<package name="com.mnm.pojo"/>
</typeAliases>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<mappers>
<package name="com.mnm.dao"/>
</mappers>
</configuration>
添加:
import com.mnm.dao.BlogMapper;
import com.mnm.pojo.Blog;
import com.mnm.utils.IDUtils;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.jupiter.api.Test;
import java.util.Date;
public class MyTest {
@Test
public void addInitBlog(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
Blog blog = new Blog();
blog.setId(IDUtils.getId());
blog.setTitle("MyBatis(一)");
blog.setAuthor("莫诺蒙");
blog.setCreateTime(new Date());
blog.setViews(999);
mapper.addBlog(blog);
blog.setId(IDUtils.getId());
blog.setTitle("MyBatis(二)");
mapper.addBlog(blog);
blog.setId(IDUtils.getId());
blog.setTitle("MyBatis(三)");
mapper.addBlog(blog);
blog.setId(IDUtils.getId());
blog.setTitle("MyBatis(四)");
mapper.addBlog(blog);
sqlSession.close();
}
}
2.if语句
mapper接口
import com.mnm.pojo.Blog;
import java.util.List;
import java.util.Map;
public interface BlogMapper {
List<Blog> queryBlogIf(Map map);
}
mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.BlogMapper">
<select id="queryBlogIf" parameterType="map" resultType="blog">
select * from blog where 1=1
<if test="title != null">
and title=#{title}
</if>
<if test="author != null">
and author = #{author}
</if>
</select>
</mapper>
测试
import com.mnm.dao.BlogMapper;
import com.mnm.pojo.Blog;
import com.mnm.utils.IDUtils;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.jupiter.api.Test;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
public class MyTest {
@Test
public void queryBlogIf(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
HashMap map = new HashMap();
map.put("title","MyBatis(一)");
map.put("author","莫诺蒙");
List<Blog> blogs = mapper.queryBlogIf(map);
for (Blog blog : blogs) {
System.out.println(blog);
}
sqlSession.close();
}
}
当我们写多个if 的时候会遇到where的问题,这时候我们需要用到where标签
where 元素只会在子元素返回任何内容的情况下才插入 “WHERE” 子句。而且,若子句的开头为 “AND” 或 “OR”,where 元素也会将它们去除。
如:
<select id="findActiveBlogLike"
resultType="Blog">
SELECT * FROM BLOG
<where>
<if test="state != null">
state = #{state}
</if>
<if test="title != null">
AND title like #{title}
</if>
<if test="author != null and author.name != null">
AND author_name like #{author.name}
</if>
</where>
</select>
就不用再写 where 1=1了
3. choose(when,otherwise)
有时候,我们不想使用所有的条件,而只是想从多个条件中选择一个使用。针对这种情况,MyBatis 提供了 choose 元素
import com.mnm.pojo.Blog;
import java.util.List;
import java.util.Map;
public interface BlogMapper {
List<Blog> queryBlogChoose(Map map);
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.BlogMapper">
<select id="queryBlogChoose" parameterType="map" resultType="blog">
select * from blog
<where>
<choose>
<when test="title!=null">
title = #{title}
</when>
<when test="author != null">
and author = #{author}
</when>
<otherwise>
and views = #{views}
</otherwise>
</choose>
</where>
</select>
</mapper>
@Test
public void queryBlogIf(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
HashMap map = new HashMap();
map.put("title","MyBatis(一)");
map.put("author","莫诺蒙");
map.put("views",999);
List<Blog> blogs = mapper.queryBlogChoose(map);
for (Blog blog : blogs) {
System.out.println(blog);
}
sqlSession.close();
}
4. trim(where、set)
如果 where 元素与你期望的不太一样,你也可以通过自定义 trim 元素来定制 where 元素的功能。
import com.mnm.pojo.Blog;
import java.util.List;
import java.util.Map;
public interface BlogMapper {
int updateBlog(Map map);
int updateBlog2(Map map);
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.BlogMapper">
<update id="updateBlog" parameterType="map">
update blog
<set>
<if test="title != null">
title = #{title},
</if>
<if test="author != null">
author = #{author},
</if>
<if test="views != null">
views = #{views}
</if>
</set>
where id = #{id}
</update>
<update id="updateBlog2" parameterType="map">
update blog
<trim prefix="SET" prefixOverrides=",">
<if test="title != null">
title = #{title},
</if>
<if test="author != null">
author = #{author},
</if>
<if test="views != null">
views = #{views}
</if>
</trim>
<trim prefix="WHERE" prefixOverrides="AND |OR ">
<if test="id != null">
id = #{id}
</if>
</trim>
</update>
</mapper>
import com.mnm.dao.BlogMapper;
import com.mnm.pojo.Blog;
import com.mnm.utils.IDUtils;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.jupiter.api.Test;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
public class MyTest {
@Test
public void updateBlog(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
HashMap map = new HashMap();
map.put("author","莫诺蒙2");
map.put("views",9999);
map.put("id","f78bf04d51d04f14b9674055a2e5c7c8");
mapper.updateBlog(map);
sqlSession.close();
}
@Test
public void updateBlog2(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
HashMap map = new HashMap();
map.put("author","张三");
map.put("views",9999);
map.put("id","42230e11f52448a7b909fc6239809d64");
mapper.updateBlog2(map);
sqlSession.close();
}
}
所谓的动态SQL,本质还是SQL语句,只是我们可以在SQL层面,去执行一个逻辑代码
5.SQL片段
有的时候,我们可能会将一些功能的部分抽取出来,方便复用
- 使用SQL标签提取公共部分
- 在需要使用的地方使用include标签引用即可
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!--core configuration file-->
<mapper namespace="com.mnm.dao.BlogMapper">
<sql id="if-tov">
<if test="title != null">
title = #{title},
</if>
<if test="author != null">
author = #{author},
</if>
<if test="views != null">
views = #{views}
</if>
</sql>
<update id="updateBlog" parameterType="map">
update blog
<set>
<include refid="if-tov"/>
</set>
where id = #{id}
</update>
</mapper>
注意事项:
- 最好基于单表来定义SQL片段
- 不要存在Where标签
6.Foreach
动态 SQL 的另一个常见使用场景是对集合进行遍历(尤其是在构建 IN 条件语句的时候)
foreach 元素的功能非常强大,它允许你指定一个集合,声明可以在元素体内使用的集合项(item)和索引(index)变量。它也允许你指定开头与结尾的字符串以及集合项迭代之间的分隔符。这个元素也不会错误地添加多余的分隔符
为了测试需要,将数据库中作者名修改为莫诺蒙1-4号 
import com.mnm.pojo.Blog;
import java.util.List;
import java.util.Map;
public interface BlogMapper {
List<Blog> queryBlogForeach(Map map);
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.BlogMapper">
<select id="queryBlogForeach" parameterType="map" resultType="Blog">
select * from blog
<where>
<foreach collection="authors" item="author"
open="(" close=")" separator=" or ">
author = #{author}
</foreach>
</where>
</select>
</mapper>
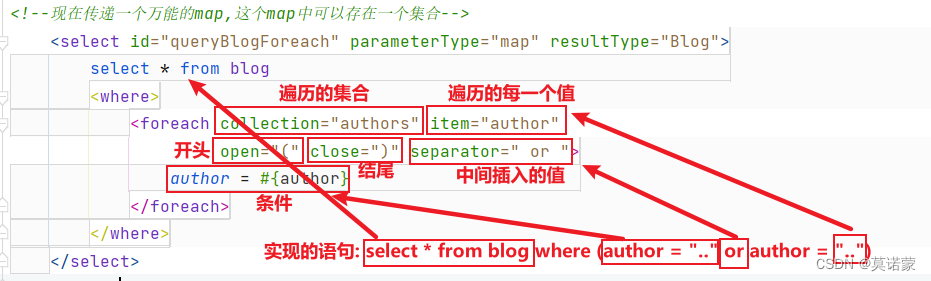
import com.mnm.dao.BlogMapper;
import com.mnm.pojo.Blog;
import com.mnm.utils.IDUtils;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.jupiter.api.Test;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
public class MyTest {
@Test
public void queryBlogForeach(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
HashMap map = new HashMap();
ArrayList<String> authors = new ArrayList<>();
authors.add("莫诺蒙1");
authors.add("莫诺蒙2");
map.put("authors",authors);
List<Blog> blogs = mapper.queryBlogForeach(map);
for (Blog blog : blogs) {
System.out.println(blog);
}
sqlSession.close();
}
}
动态SQL就是在拼接SQL语句,我们只要保证SQL的正确性,按照SQL的格式去排列组合就可以了 建议:
- 先在Mysql中写出完整的SQL,再对应的去修改成为我们的动态SQL,实现通用即可.
十二.缓存
1.简介
1.什么是缓存[Cache]?
- 存在内存中的临时数据。
- 将用户经常查询的数据放在缓存(内存)中,用户去查询数据就不用从磁盘上(关系型数据库数据文件)查询,从缓存中查询,从而提高查询效率,解决了高并发系统的性能问题。
2.为什么使用缓存?
- 减少和数据库的交互次数,减少系统开销,提高系统效率。
3.什么样的数据能使用缓存?
2.MyBatis缓存
MyBatis包含一个非常强大的查询缓存特性,它可以非常方便地定制和配置缓存。缓存可以极大的提升查询效率。
MyBatis系统中默认定义了两级缓存:一级缓存和二级缓存
- 默认情况下,只有一级缓存开启。(SqlSession级别的缓存,也称为本地缓存)
- 二级缓存需要手动开启和配置,他是基于namespace级别的缓存。
- 为了提高扩展性,MyBatis定义了缓存接口Cache。我们可以通过实现Cache接口来自定义二级缓存
3.一级缓存
·一级缓存也叫本地缓存: SqlSession
- 数据库同一次会话期间查询到的数据会放在本地缓存中。
- 以后如果需要获取相同的数据,直接从缓存中拿,没必须再去查询数据库;
新建一个项目,内容大致不变 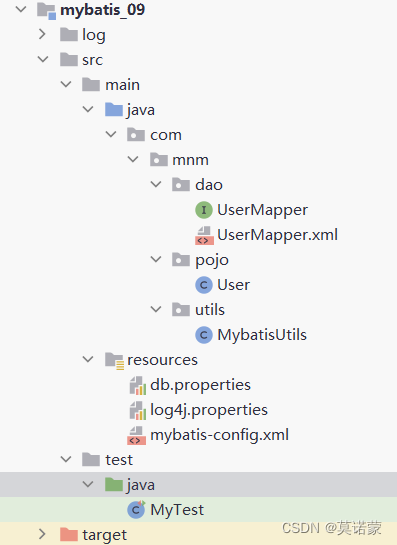
import com.mnm.pojo.User;
import org.apache.ibatis.annotations.Param;
public interface UserMapper {
User queryUserById(@Param("id") int id);
}
Mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.UserMapper">
<select id="queryUserById" parameterType="_int" resultType="user">
select * from user where id = #{id}
</select>
</mapper>
测试在一个Session中查询两次相同记录,查看日志输出
import com.mnm.dao.UserMapper;
import com.mnm.pojo.User;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
public class MyTest {
@Test
public void getUserById(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User user = mapper.queryUserById(2);
System.out.println(user);
User user2 = mapper.queryUserById(2);
System.out.println(user2);
System.out.println(user == user2);
sqlSession.close();
}
}
结果: 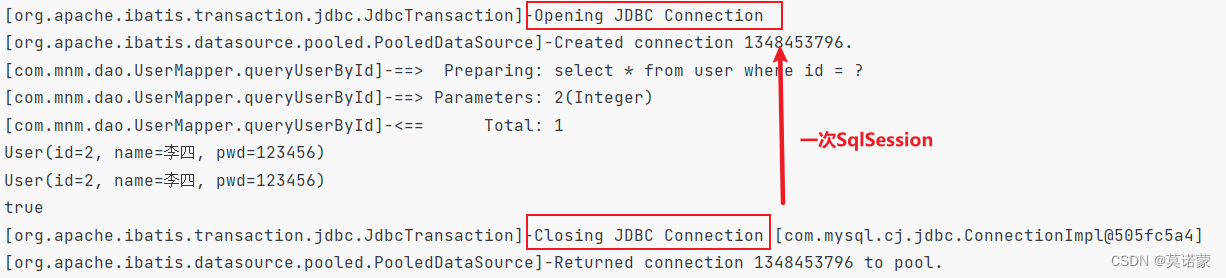  现在新增一个修改
import com.mnm.pojo.User;
import org.apache.ibatis.annotations.Param;
public interface UserMapper {
User queryUserById(@Param("id") int id);
int updateUser(User user);
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.UserMapper">
<select id="queryUserById" parameterType="_int" resultType="user">
select * from user where id = #{id}
</select>
<update id="updateUser" parameterType="user">
update user set name = #{name},pwd = #{pwd} where id = #{id}
</update>
</mapper>
import com.mnm.dao.UserMapper;
import com.mnm.pojo.User;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
public class MyTest {
@Test
public void getUserById(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User user = mapper.queryUserById(1);
System.out.println(user);
mapper.updateUser(new User(2,"孙七","6666"));
User user2 = mapper.queryUserById(1);
System.out.println(user2);
System.out.println(user == user2);
sqlSession.close();
}
}
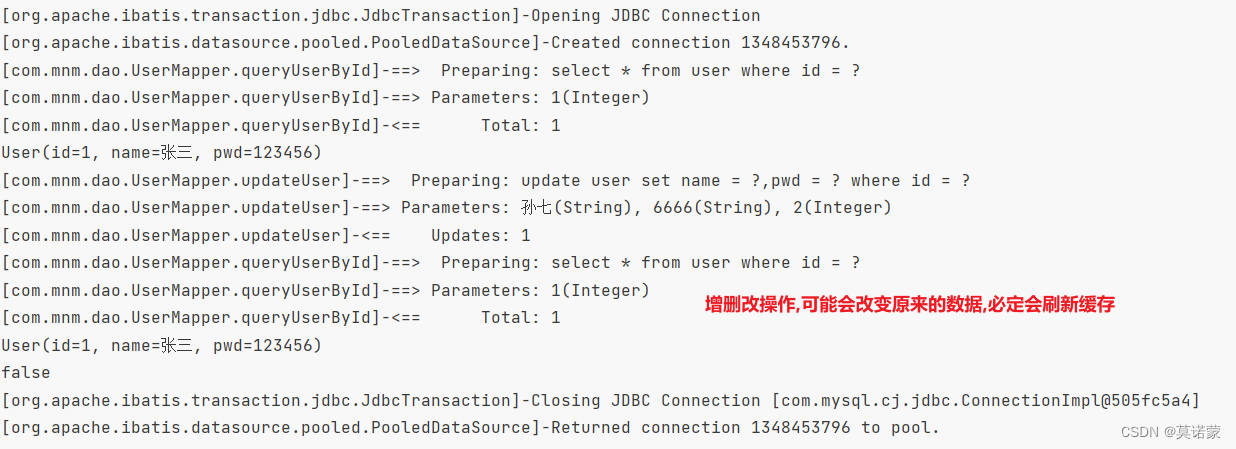 缓存失效的情况:
- 查询不同的东西
- 增删改操作,可能会改变原来的数据,所以必定会刷新缓存
- 查询不同的的mapper.xml
- 手动清理缓存
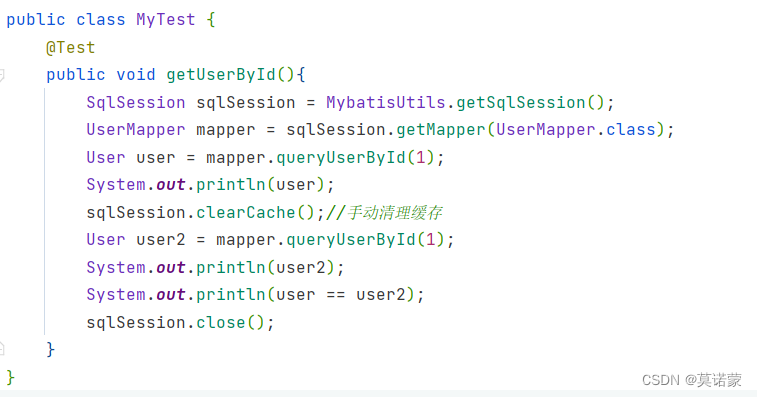
小结:一级缓存默认开启,只在一次SqlSession中有效,也就是拿到连接到关闭连接这个区间,一级缓存就是一个Map
4.二级缓存
二级缓存也叫全局缓存,一级缓存作用域太低了,所以诞生了二级缓存
- 基于namespace级别的缓存,一个名称空间,对应一个二级缓存;
工作机制
- 一个会话查询一条数据,这个数据就会被放在当前会话的一级缓存中;
- 如果当前会话关闭了,这个会话对应的一级缓存就没了;但是我们想要的是,会话关闭了,一级缓存中的数据被保存到二级缓存中;
- 新的会话查询信息,就可以从二级缓存中获取内容;
- 不同的mapper查出的数据会放在自己对应的缓存(map)中:
也可以修改cache的一些属性,比如: 
可用的清除策略有:
- LRU – 最近最少使用:移除最长时间不被使用的对象。
- FIFO – 先进先出:按对象进入缓存的顺序来移除它们。
- SOFT – 软引用:基于垃圾回收器状态和软引用规则移除对象。
- WEAK – 弱引用:更积极地基于垃圾收集器状态和弱引用规则移除对象。
步骤: 1.开启全局缓存(默认开启,但是还是会手动设置)  mapper.xml
<settings>
<setting name="logImpl" value="LOG4J"/>
<setting name="mapUnderscoreToCamelCase" value="true"/>
<setting name="cacheEnabled" value="true"/>
</settings>
2.手动设置二级缓存
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.UserMapper">
<cache
eviction="FIFO"
flushInterval="60000"
size="512"
readOnly="true"/>
<select id="queryUserById" parameterType="_int" resultType="user">
select * from user where id = #{id}
</select>
<update id="updateUser" parameterType="user">
update user set name = #{name},pwd = #{pwd} where id = #{id}
</update>
</mapper>
3.在
import com.mnm.dao.UserMapper;
import com.mnm.pojo.User;
import com.mnm.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.Test;
public class MyTest {
@Test
public void getUserById(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
SqlSession sqlSession2 = MybatisUtils.getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
User user = mapper.queryUserById(1);
System.out.println(user);
sqlSession.close();
UserMapper mapper2 = sqlSession2.getMapper(UserMapper.class);
User user2 = mapper2.queryUserById(1);
System.out.println(user2);
System.out.println(user == user2);
sqlSession2.close();
}
}
只要开启了二级缓存,在同一个Mapper下就有效 所有的数据都会先放在一级缓存中 只有当会话提交,或者关闭的时候,才会提交到二级缓存中
遇到的问题:序列化异常 Cause: java.io.NotSerializableException 解决方法: 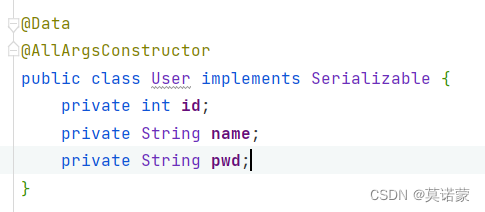
5.缓存原理
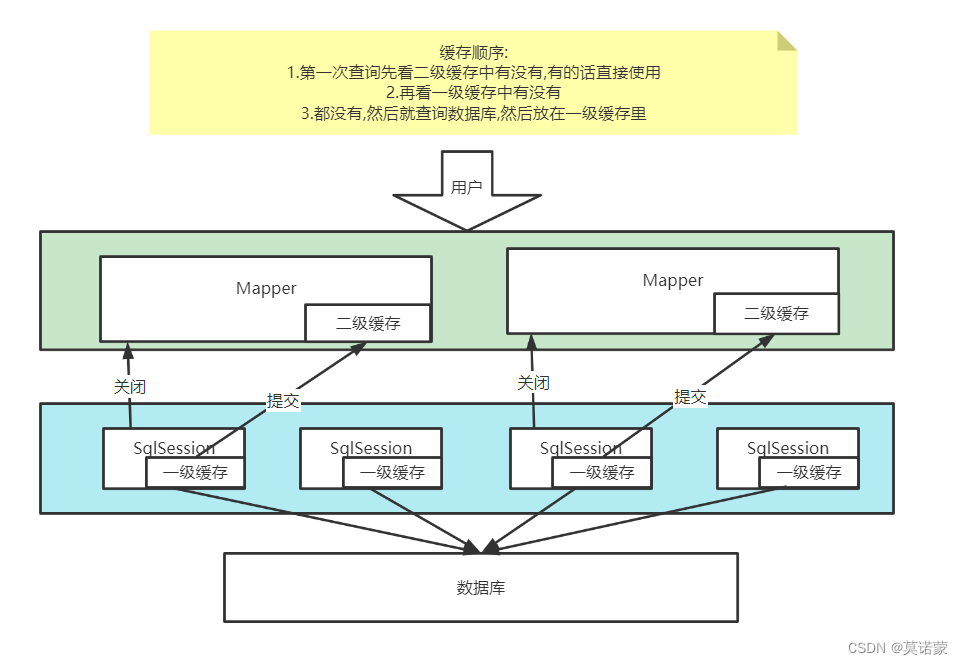
6.自定义缓存
Ehcache是一种广泛使用的开源java分布式缓存,主要面向通用缓存
要在程序中使用Ehcache,要先导包
<dependency>
<groupId>org.mybatis.caches</groupId>
<artifactId>mybatis-ehcache</artifactId>
<version>1.2.2</version>
</dependency>
 新建一个ehcache.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://ehcache.org/ehcache.xsd"
updateCheck="false">
<diskStore path="./tmpdir/Tmp_EhCache"/>
<defaultCache
eternal="false"
maxElementsInMemory="10000"
overflowToDisk="false"
diskPersistent="false"
timeToIdleSeconds="1800"
timeToLiveSeconds="259200"
memoryStoreEvictionPolicy="LRU"/>
<cache
name="cloud_user"
eternal="false"
maxElementsInMemory="5000"
overflowToDisk="false"
diskPersistent="false"
timeToIdleSeconds="1800"
timeToLiveSeconds="1800"
memoryStoreEvictionPolicy="LRU"/>
</ehcache>
结果和原本没有什么区别
在mapper中指定我们的ehcache缓存
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.mnm.dao.UserMapper">
<cache type="org.mybatis.caches.ehcache.EhcacheCache"/>
<select id="queryUserById" parameterType="_int" resultType="user">
select * from user where id = #{id}
</select>
<update id="updateUser" parameterType="user">
update user set name = #{name},pwd = #{pwd} where id = #{id}
</update>
</mapper>
|