EasyExcel
EasyExcel相比于EasyPoi性能好一点,特别是在大数据量时候,EasyExcel的性能相较于更好一些。对于普通低数据量的导入导出EasyExcel占用资源的数量相对少一些。
1. 初体验
1.1 依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>3.0.5</version>
</dependency>
1.2 注解
@ExcelProperty
- 作用: 用于指定实体类属性和表格列名称的映射关系
- 举个栗子:
@ExcelProperty("编号")
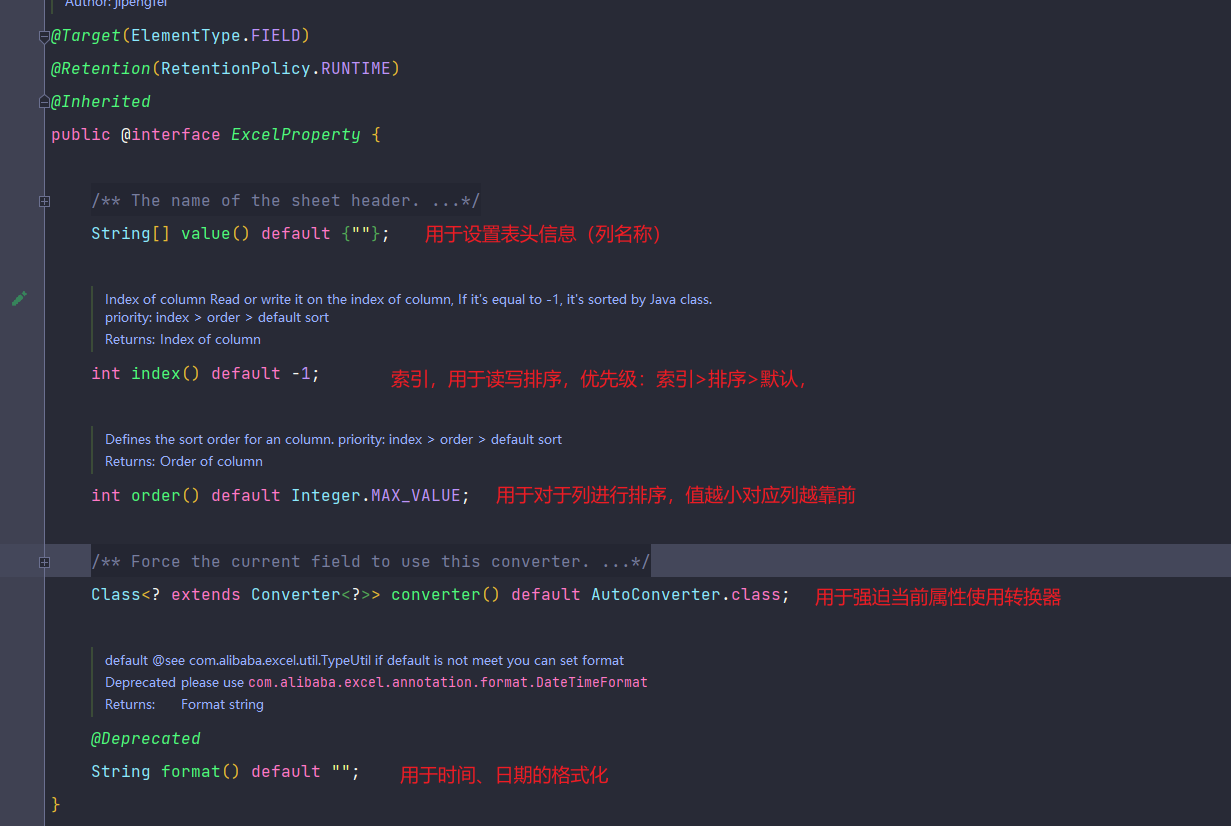
@ColumnWidth
- 作用: 用于指定某个属性对应的表格列的宽度。
- 举个栗子:
@ExcelProperty(value = "编号")
@ColumnWidth(20)
private Long id;
@HeadRowHeight
@HeadRowHeight()
@ContentRowHeight()
public class User implements Serializable {
...
}
@ContentRowHeight
@ExcelIgnore
- 作用: 只作为一个属性标记,用于标记该属性不被导出到excel。
@DateTimeFormat
@NumberFormat
- 标注在成员变量上,数字转换,代码中用String类型的成员变量去接收excel数字格式的数据会调用这个注解。里边的value参照java.text.DecimalFormat
@ExcelIgnoreUnannotated
不标注该注解时,默认类中所有的成员变量都会参与读写,无论是否在成员变量上加了@ExcelProperty的注解。
标注该注解后,类中的成员变量如果没有标注@ExcelProperty注解将不会参与读写。
1.3 写操作
3.1 简单写
String filePath = "C:\\Users\\ArchieSean\\Desktop\\a.xlsx";
EasyExcel.write(filePath, User.class)
.sheet(0, "用户信息")
.doWrite(users);
String filePath = "C:\\Users\\ArchieSean\\Desktop\\b.xlsx";
ExcelWriter excelWriter = EasyExcel.write(filePath, User.class).build();
WriteSheet writeSheet = EasyExcel.writerSheet("模板").build();
excelWriter.write(users, writeSheet);
excelWriter.finish();
3.2 指定写
EasyExcel.write(filePath, User.class)
.includeColumnFiledNames()
.excludeColumnFiledNames()
3.3 分页写
String filePath = "C:\\Users\\ArchieSean\\Desktop\\b.xlsx";
ExcelWriter excelWriter = EasyExcel.write(filePath, User.class).build();
WriteSheet writeSheet = EasyExcel.writerSheet("模板").build();
excelWriter.write(users, writeSheet);
excelWriter.finish();
3.4 图片导出
private File file;
private InputStream inputStream;
@ExcelProperty(converter = StringImageConverter.class)
private String string;
private byte[] byteArray;
private URL url;
3.5 自定义样式
样式策略: WriteCellSty类
WriteCellStyle headWriteCellStyle = new WriteCellStyle();
WriteCellStyle contentWriteCellStyle = new WriteCellStyle();
private DataFormatData dataFormatData;
private WriteFont writeFont;
private Boolean hidden;
private Boolean locked;
private Boolean quotePrefix;
private HorizontalAlignment horizontalAlignment;
private Boolean wrapped;
private VerticalAlignment verticalAlignment;
private Short rotation;
private Short indent;
private BorderStyle borderLeft;
private BorderStyle borderRight;
private BorderStyle borderTop;
private BorderStyle borderBottom;
private Short leftBorderColor;
private Short rightBorderColor;
private Short topBorderColor;
private Short bottomBorderColor;
private FillPatternType fillPatternType;
private Short fillBackgroundColor;
private Short fillForegroundColor;
private Boolean shrinkToFit;
样式设置:HorizontalCellStyleStrategy
- HorizontalCellStyleStrategy的家族
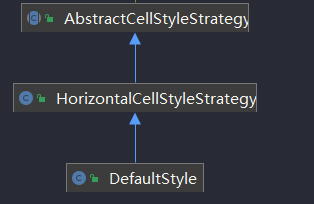
public HorizontalCellStyleStrategy(WriteCellStyle headWriteCellStyle, WriteCellStyle contentWriteCellStyle) {
this.headWriteCellStyle = headWriteCellStyle;
if (contentWriteCellStyle != null) {
this.contentWriteCellStyleList = ListUtils.newArrayList(contentWriteCellStyle);
}
}
HorizontalCellStyleStrategy strategy = new HorizontalCellStyleStrategy(头部样式, 内容样式);
3.6 模板写出
- 将模板放在项目当中,写出时设置模板,通过withTemplate()方法设置模板。
- 举个例子
@Test
public void templateWrite() {
String templateFileName = TestFileUtil.getPath() + "demo" + File.separator + "demo.xlsx";
String fileName = TestFileUtil.getPath() + "templateWrite" + System.currentTimeMillis() + ".xlsx";
EasyExcel.write(fileName, DemoData.class).withTemplate(templateFileName).sheet().doWrite(data());
}
3.7 合并单元格
- 使用的类:LoopMergeStrategy
- 源码:
public class LoopMergeStrategy implements RowWriteHandler {
private int eachRow;
private int columnExtend;
private int columnIndex;
}
LoopMergeStrategy loopMergeStrategy = new LoopMergeStrategy(2, 0);
EasyExcel.write(fileName, DemoData.class).registerWriteHandler(loopMergeStrategy).sheet("模板")
.doWrite(data());
1.4 读操作
4.1 简单读
String filePath = "C:\\Users\\ArchieSean\\Desktop\\b.xlsx";
EasyExcel.read(filePath, User.class, new AnalysisEventListener<User>() {
@Override
public void invoke(User data, AnalysisContext context) {
System.out.println(data.toString());
}
@Override
public void doAfterAllAnalysed(AnalysisContext context) {
System.out.println("=======解析完成===");
}
}).sheet(0).doRead();
4.2 读多个sheet
EasyExcel.read(fileName, DemoData.class, new DemoDataListener()).doReadAll();
ExcelReader excelReader = EasyExcel.read(fileName).build();
ReadSheet readSheet1 =
EasyExcel.readSheet(0).head(DemoData.class).registerReadListener(new DemoDataListener()).build();
ReadSheet readSheet2 =
EasyExcel.readSheet(1).head(DemoData.class).registerReadListener(new DemoDataListener()).build();
excelReader.read(readSheet1, readSheet2);
excelReader.finish();
4.3 日期、数字或者自定义格式转换
@DateTimeFormat("yyyy年MM月dd日HH时mm分ss秒")
@NumberFormat("#.##%")
|