1 安装 Input System
Unity 推出的控制诸如玩家移动,UI 界面选择按钮的新的选择。首先在 Package Manager 中,点击 Unity Registry,然后搜索找到 Input System,下载导入。出现报错不必理会,重启 Unity,查看是否安装成功。
2 配置 Input Actions 文件
在 Setting 文件夹右键 create,往下找,点击 Input Actions,新建出来一个配置所有输入动作的文件,命名为 InputActions 即可。双击打开,设置 Action Maps(动作表),可分为玩家,UI 等。然后在第二列进行设置动作并绑定按键。 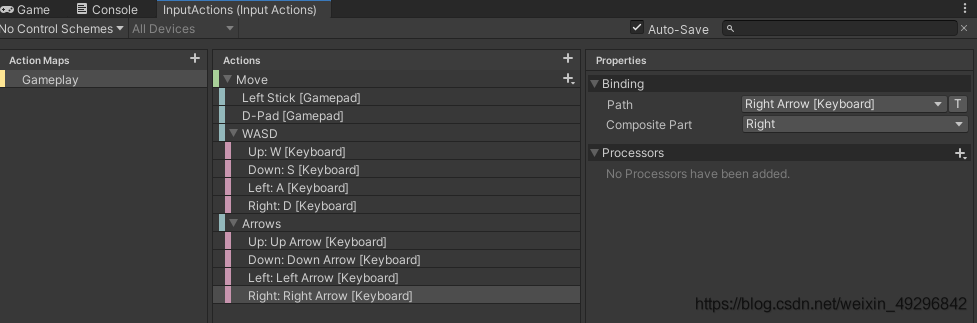
图 1 Input Actions 配置界面
3 使用 Input System 进行按键绑定
直接在 Actions 的右侧点击加号,添加完成后命名动作,进行设置,Action type 设置为 Value,Control type 设置为 Vector 2。再点击该动作右侧的加号。此时出现两种选择,如果是手柄,可以直接点击 Add Binding,如果是键盘输入,则点击 Add 2D Vector Composite。接下来就是对其进行命名和选择了。**切记:设置好以后点击 Save Asset 按钮或者勾选 Auto-Save 保存配置文件。
4 使用 Input System 自动生成 C# 类
点击之前创建好的 InputActions 配置文件,在 Inspector 面板勾选 Generate C# class,默认就好,点击 Apply。打开生成好的脚本,在最后有 IGameplay 接口,那是开始配置好的 Gameplay。里面还有 OnMove 函数,也是我们创建好的 Move 动作。
5 创建输入管理器脚本 PlayerInput
自行创建。代码如下。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
using UnityEngine.InputSystem;
[CreateAssetMenu(menuName = "Player Input")]
public class PlayerInput : ScriptableObject, InputActions.IGameplayActions
{
public event UnityAction<Vector2> onMove = delegate {};
public event UnityAction onStopMove = delegate {};
InputActions inputActions;
void OnEnable()
{
inputActions = new InputActions();
inputActions.Gameplay.SetCallbacks(this);
}
void OnDisable()
{
DisableAllInput();
}
public void DisableAllInput()
{
inputActions.Gameplay.Disable();
}
public void EnableGameplayInput()
{
inputActions.Gameplay.Enable();
Cursor.visible = false;
Cursor.lockState = CursorLockMode.Locked;
}
public void OnMove(InputAction.CallbackContext context)
{
if (context.phase == InputActionPhase.Performed)
{
onMove.Invoke(context.ReadValue<Vector2>());
}
if (context.phase == InputActionPhase.Canceled)
{
onStopMove.Invoke();
}
}
}
写好后,等待 Unity 编译器完成,然后右键 create 出 Player Input,这就是上述配置文件,Unity 给其一个特殊的图标,以后可以直接拿来用的东西。
6 创建玩家移动的脚本
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
[SerializeField] PlayerInput input;
new Rigidbody2D rigidbody;
[SerializeField] float moveSpeed = 10.0f;
private void Awake()
{
rigidbody = GetComponent<Rigidbody2D>();
}
private void OnEnable()
{
input.onMove += Move;
input.onStopMove += StopMove;
}
private void OnDisable()
{
input.onMove -= Move;
input.onStopMove -= StopMove;
}
void Start()
{
rigidbody.gravityScale = 0.0f;
input.EnableGameplayInput();
}
void Update()
{
}
void Move(Vector2 moveInput)
{
rigidbody.velocity = moveInput * moveSpeed;
}
void StopMove()
{
rigidbody.velocity = Vector2.zero;
}
}
|