记录下项目用到的小功能。
private Sprite ShowCircle()
{
if (selectedList.Count <= 0)
{
return null;
}
int radius = 50;
Texture2D texture2D = new Texture2D(100, 100);
Vector2 center = new Vector2(50, 50);
for (int i = 0; i < texture2D.width; i++)
{
for (int j = 0; j < texture2D.height; j++)
{
texture2D.SetPixel(i,j,new Color(0,0,0,0));
}
}
int unitAngle = 360 / selectedList.Count;
int count = selectedList.Count;
int index = 0;
Color color = Color.white;
for (float i = 0; i <= 360; i += 0.2f)
{
for (int k = 1; k <= count; k++)
{
if (i <= k * unitAngle)
{
index = k - 1;
float change = (float)Math.Round((float)(i - (index * unitAngle)) / unitAngle, 2);
if (index < selectedList.Count - 1)
{
if (selectedList[index].isLerpColor)
{
color = Color.Lerp(selectedList[index].colorImage, selectedList[index + 1].colorImage, change);
}
else
{
color = selectedList[index].colorImage;
}
}
else
{
if (selectedList[index].isLerpColor)
{
color = Color.Lerp(selectedList[index].colorImage, selectedList[0].colorImage, change);
}
else
{
color = selectedList[index].colorImage;
}
}
break;
}
}
for (int j = 0; j < radius; j++)
{
float xx = center.x + j * Mathf.Cos(i * Mathf.Deg2Rad);
float yy = center.y + j * Mathf.Sin(i * Mathf.Deg2Rad);
texture2D.SetPixel((int)xx, (int)yy, color);
}
}
texture2D.Apply();
return Sprite.Create(texture2D, new Rect(0, 0, texture2D.width, texture2D.height), Vector2.zero);
}
private Sprite ShowAnnulus()
{
if (selectedList.Count<=1)
{
return null;
}
int radius = 50;
Texture2D texture2D = new Texture2D(100, 100);
Vector2 center = new Vector2(50, 50);
for (int i = 0; i < texture2D.width; i++)
{
for (int j = 0; j < texture2D.height; j++)
{
texture2D.SetPixel(i, j, new Color(0, 0, 0, 0));
}
}
int count = selectedList.Count;
int index = 0;
Color color = Color.white;
for (float i = 0; i <= 360; i += 0.2f)
{
for (int j = 0; j < radius; j++)
{
float xx = center.x + j * Mathf.Cos(i * Mathf.Deg2Rad);
float yy = center.y + j * Mathf.Sin(i * Mathf.Deg2Rad);
int unitCount = radius / (count-1);
index = Mathf.Clamp((int)(j / unitCount), 0, count - 1);
if (selectedList[index].isLerpColor)
{
color= Color.Lerp(selectedList[index].colorImage, selectedList[Mathf.Min(index + 1,count-1)].colorImage,(float)(j - (index * unitCount)) / unitCount);
}
else
{
color = selectedList[index].colorImage;
}
texture2D.SetPixel((int)xx, (int)yy, color);
}
}
texture2D.Apply();
return Sprite.Create(texture2D, new Rect(0, 0, texture2D.width, texture2D.height), Vector2.zero);
}
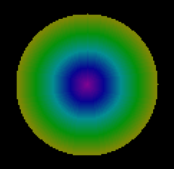 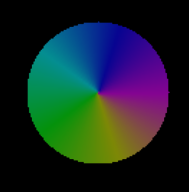
|