目录
1.游戏规则
?2.注册登录
?3.创建方块对象
4.创建食物和炸弹对象
5.各种碰撞
6.发射子弹
7.碰到边缘结束游戏
以下就是贪吃方块小游戏的页面效果
1.游戏规则
如果没有注册会弹出提示框
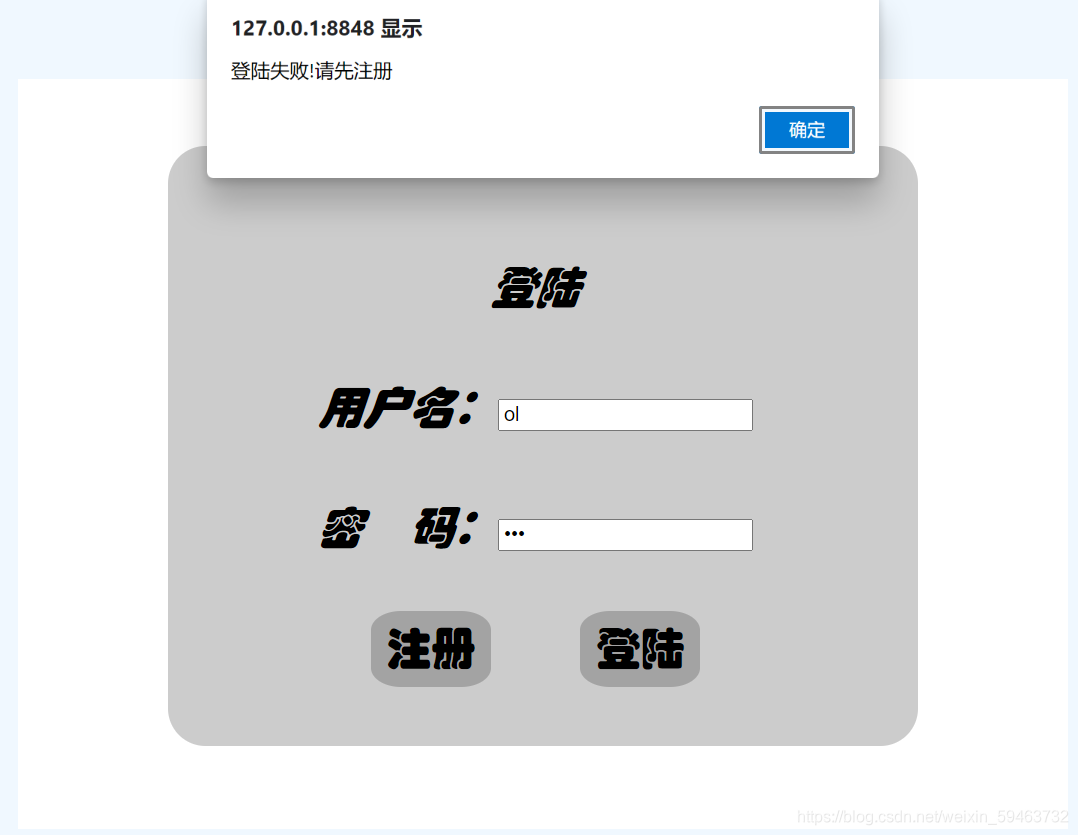
注册后可以直接进入游戏,点击开始游戏,可以体验这款游戏(如果密码错误,不会有反应)
?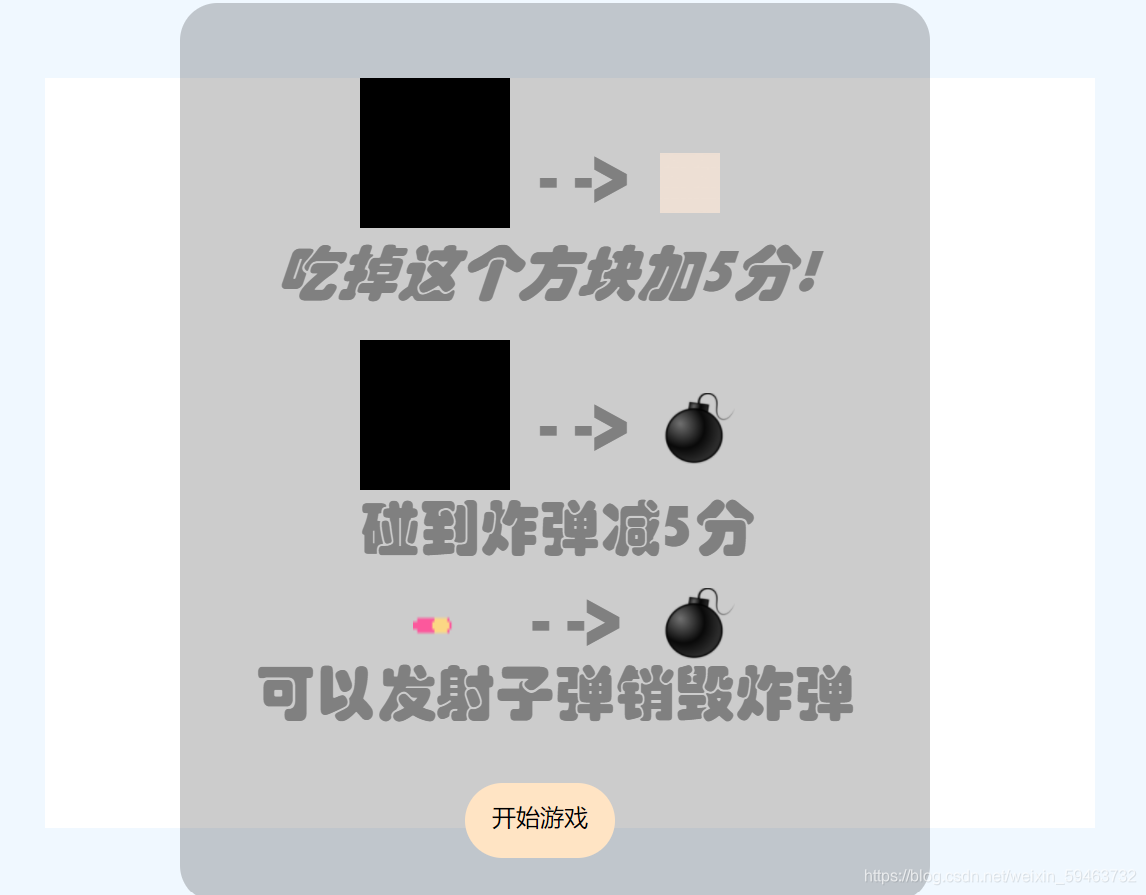
?利用上下左右键可以移动方块,触碰边缘会直接结束游戏,吃掉肉色方块可以加分加个数(方块会在3秒后变换位置),触碰炸弹会减分(当分数小于0时,会结束游戏),按下空格可以发射子弹销毁炸弹?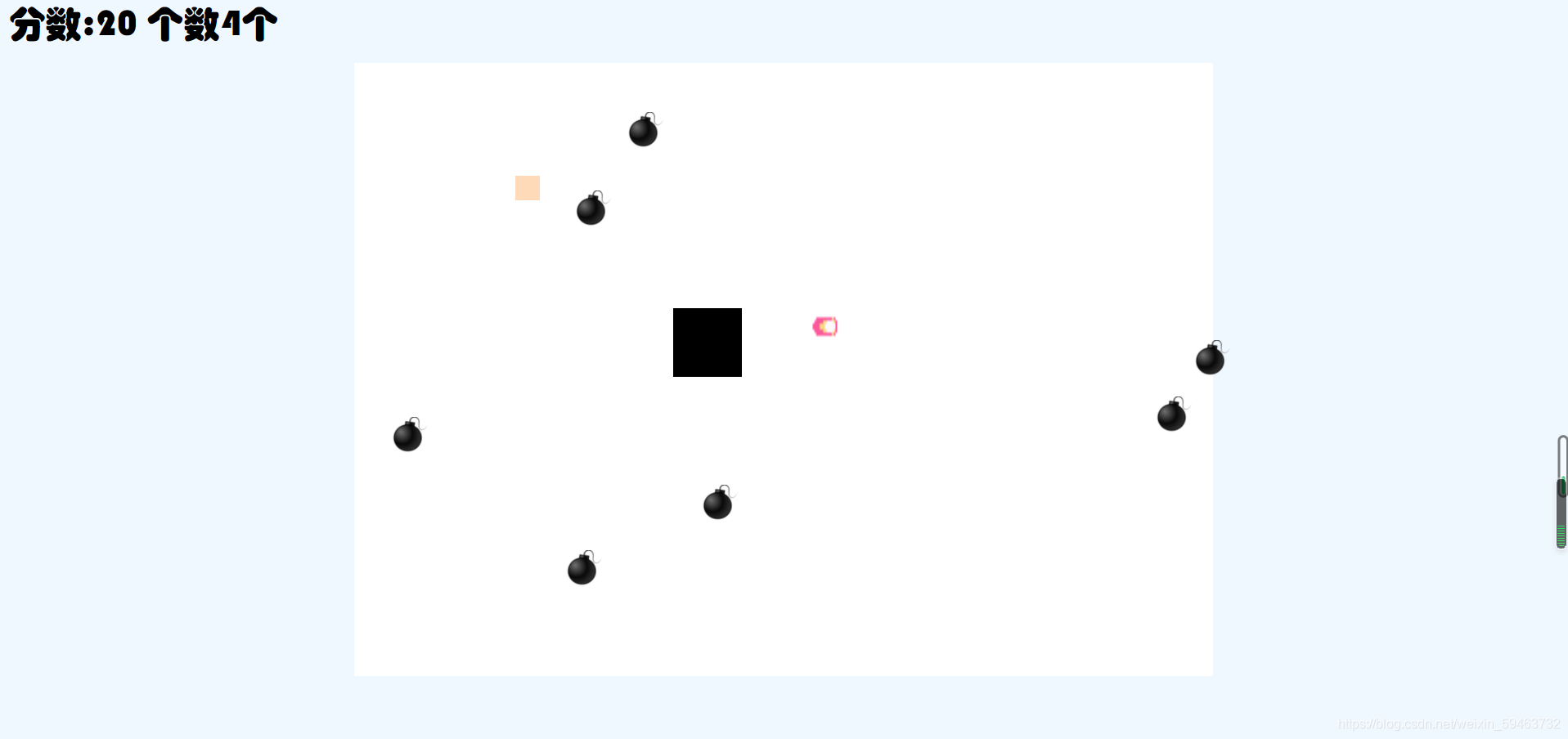
?2.注册登录
注册登录这里用的是localStorage在存储时,应该注意有没有将存储的对象用JSON.stringify()转换
//点击注册
$("[name=register]").click(function() {
var uname = $("#uname").val(); //用户名
var upwd = $("#upwd").val(); //密码
var user = { //用户对象
uname: uname,
upwd: upwd,
uscore: 0
}
//存
localStorage.setItem(uname, JSON.stringify(user));
alert("注册成功");
$("#loginbox").hide();
$("#startbox").show();
})
在登陆获取数据时,也需要转换,用的是JSON.parse()。?
//点击登陆
$("[name=login]").click(function() {
var uname = $("#uname").val(); //用户名
var upwd = $("#upwd").val(); //密码
//判断有没有注册
if (JSON.parse(localStorage.getItem(uname)) == null) {
alert("登陆失败!请先注册");
} else {
for (var i = 0; i < localStorage.length; i++) {
var key = localStorage.key(i);
var user = JSON.parse(localStorage.getItem(key));
if (uname == user.uname && upwd == user.upwd) {
alert("登陆成功!");
//console.log(JSON.parse(localStorage.getItem(uname)));
$("#loginbox").hide();
$("#startbox").show();
}
}
}
})
?3.创建方块对象
在这里创建了方块对象,也用到了时间函数生成食物与炸弹,每一个时间函数都需要关闭。
//开始游戏按钮
$("#startGame").click(function() {
if ($(this).text() == "开始游戏") {
$("#startbox").hide();
$(this).text("停止游戏");
//创建方块
var $ball = $("<div></div>");
//设置方块的样式
$ball.addClass("ball");
//将ball添加到div中
$("#smallbox").append($ball);
$("#info").text("分数:" + score + " 个数" + num + "个");
$(".food").remove();
//随机产生食物
timeCreateFood = setInterval("createfood()", 2700);
//随机产生炸弹
timeCreateBomb = setInterval("createbomb()", 100);
//方块碰撞炸弹
timeMoveBomb = setInterval("moveBomb()", 100);
//子弹碰撞炸弹
timeFirecrash = setInterval("firecrash()", 100);
} else {
//关闭
clearInterval(timeCreateFood);
clearInterval(timeMoveFood);
clearInterval(timeCreateBomb);
clearInterval(timeMoveBomb);
clearInterval(timeMoveFire);
clearInterval(timeFirecrash);
$(this).text("开始游戏");
//移除方块和食物
$(".ball").remove();
$(".food").remove();
$(".bomb").remove();
$("#info").text("");
$("#endbox").show();
score = 0;
}
})
这里用到了键盘事件,每一步都需要判断分数是否小于0,小于0结束游戏(需要关闭时间对象,不然会一直产生),在空格处创建子弹对象
向左:需要得到方块的x值,地图的左侧边缘x值为0,使用将0和方块的x值传进gameOver方法中;向上:与向左相似,得到方块的y值,地图的上端边缘y值为0,使用将0和方块的y值传进gameOver方法中;向右:得到方块x值,将方块x值和地图宽与方块宽的差传进gameOver方法中;向下:得到方块的y值,将方块的y值和地图高与方块高的差传进gameOver方法中(gameOver()在本文末尾)
//移动方块
$("body").keydown(function(event) {
switch (event.keyCode) {
case 37: //向左
//碰到边缘
var ball = $(".ball");
var odlLeft = ball.css("left"); //得到方块的y值
gameOver(0, parseInt(odlLeft));
//分数小于0时结束游戏
if (parseInt(score) < 0) {
//关闭
clearInterval(timeCreateFood);
clearInterval(timeMoveFood);
clearInterval(timeCreateBomb);
clearInterval(timeMoveBomb);
clearInterval(timeMoveFire);
clearInterval(timeFirecrash);
/* $("#startGame").text("开始游戏"); */
//移除方块和食物
$(".ball").remove();
$(".food").remove();
$(".bomb").remove();
$(".fire").remove();
$("#info").text("");
score = 0;
$("#endbox").show();
$("#num").text("分数:" + score + "\n吃了" + num + "个");
}
//移动
var left = parseFloat($(".ball").css("left"));
$(".ball").css("left", (left - 20) + "px");
//碰撞食物
if ($(".food").length == 1) {
timeMoveFood = setInterval("moveFood()", 100);
return;
}
break;
case 38: //向上
//碰到边缘
var ball = $(".ball");
var odTop = ball.css("top"); //得到方块的x值
gameOver(0, parseInt(odTop));
//分数小于0是结束游戏
if (parseInt(score) < 0) {
//关闭
clearInterval(timeCreateFood);
clearInterval(timeMoveFood);
clearInterval(timeCreateBomb);
clearInterval(timeMoveBomb);
clearInterval(timeMoveFire);
clearInterval(timeFirecrash);
/* $("#startGame").text("开始游戏"); */
//移除方块和食物
$(".ball").remove();
$(".food").remove();
$(".bomb").remove();
$(".fire").remove();
$("#info").text("");
score = 0;
$("#endbox").show();
$("#num").text("分数:" + score + "\n吃了" + num + "个");
}
//移动
var top = parseFloat($(".ball").css("top"));
$(".ball").css("top", (top - 20) + "px");
//碰撞食物
if ($(".food").length == 1) {
timeMoveFood = setInterval("moveFood()", 100);
return;
}
break;
case 39: //向右
//碰到边缘
var ball = $(".ball");
var odlLeft = ball.css("left");
var max = smallbox.width() - ball.width() - 50;
gameOver(parseInt(odlLeft), max);
//分数小于0是结束游戏
if (parseInt(score) < 0) {
//关闭
clearInterval(timeCreateFood);
clearInterval(timeMoveFood);
clearInterval(timeCreateBomb);
clearInterval(timeMoveBomb);
clearInterval(timeMoveFire);
clearInterval(timeFirecrash);
/* $("#startGame").text("开始游戏"); */
//移除方块和食物
$(".ball").remove();
$(".food").remove();
$(".bomb").remove();
$(".fire").remove();
$("#info").text("");
score = 0;
$("#endbox").show();
$("#num").text("分数:" + score + "\n吃了" + num + "个");
}
//移动
var left = parseFloat($(".ball").css("left"));
$(".ball").css("left", (left + 20) + "px");
//碰撞食物
if ($(".food").length == 1) {
timeMoveFood = setInterval("moveFood()", 100);
return;
}
break;
case 40: //向下
//碰到边缘
var ball = $(".ball");
var odBottom = ball.css("top");
var bottomMax = smallbox.outerHeight() - (ball.height() + 50);
gameOver(parseInt(odBottom), bottomMax);
//分数小于0是结束游戏
if (parseInt(score) < 0) {
//关闭
clearInterval(timeCreateFood);
clearInterval(timeMoveFood);
clearInterval(timeCreateBomb);
clearInterval(timeMoveBomb);
clearInterval(timeMoveFire);
clearInterval(timeFirecrash);
/* $("#startGame").text("开始游戏"); */
//移除方块和食物
$(".ball").remove();
$(".food").remove();
$(".bomb").remove();
$(".fire").remove()
$("#info").text("");
score = 0;
$("#endbox").show();
$("#num").text("分数:" + score + "\n吃了" + num + "个");
}
//移动
var top = parseFloat($(".ball").css("top"));
$(".ball").css("top", (top + 20) + "px");
//碰撞食物
if ($(".food").length == 1) {
timeMoveFood = setInterval("moveFood()", 100);
return;
}
break;
case 32: //空格
//发射子弹
var $fire = $("<img/>");
$fire.attr("src", "img/子弹.gif");
$fire.addClass("fire");
var top = $(".ball").css("top");
var left = $(".ball").css("left");
$fire.css("top", top);
$fire.css("left", left);
$("#smallbox").append($fire);
timeMoveFire = setInterval("MoveFire()", 100)
break;
}
})
4.创建食物和炸弹对象
食物与炸弹产生的范围:设置了top与left,随机数*地图的宽和高
食物只能有一个
//随机产生食物
function createfood() {
$(".food").remove();
var $food = $("<div></div>");
$food.addClass("food");
$("#smallbox").append($food);
$food.css({
"top": Math.random() * 490,
"left": Math.random() * 690
});
}
炸弹不允许超过7个。?
//产生炸弹
var bomb = $(".bomb");
function createbomb() {
if ($(".bomb").length > 6) {
return;
}
var $bomb = $("<img />");
$bomb.attr("src", "img/炸弹.png");
$bomb.addClass("bomb");
$("#smallbox").append($bomb);
$bomb.css({
"top": Math.random() * 490,
"left": Math.random() * 690
});
}
5.各种碰撞
碰撞统一都用到了碰撞公式:
x轴判断:|(x1+w1/2)-(x2+w2/2)|<|(w1+w2)/2|
y轴判断:|(y1+h1/2)-(y2+h2/2)|<|(h1+h2)/2|
//碰撞食物
function moveFood() {
$(".food").each(function(index, item) {
//食物的xy的位置
var x1 = parseFloat($(item).css("left")); //食物的x值
var y1 = parseFloat($(item).css("top")); //食物的y值
//食物的宽和高
var w1 = parseFloat($(item).css("width"));
var h1 = parseFloat($(item).css("height"));
$(".ball").each(function(index2, item2) {
//方块左边的xy的位置
var bl = parseFloat($(item2).css("left"));
var bt = parseFloat($(item2).css("top"));
//方块的宽和高
var w2 = parseFloat($(item2).css("padding"));
var h2 = parseFloat($(item2).css("height"));
if (Math.abs((x1 + w1 / 2) - (bl + w2 / 2)) < Math.abs((w1 + w2) / 2) &&
Math.abs((y1 + h1 / 2) - (bt + w2 / 2)) < Math.abs((h1 + w2) / 2)) {
$(item).remove();
num = num + 1; //吃到食物的个数
score = score + 5; //分数
$("#info").text("分数:" + score + "\n\n个数" + num + "个")
ballpadding = parseFloat($(".ball").css("padding")) + 2; //方块的padding
$(".ball").css("padding", ballpadding + "px");
}
})
})
}
//碰撞炸弹
function moveBomb() {
$(".bomb").each(function(index, item) {
//炸弹的xy的位置
var x1 = parseFloat($(item).css("left")); //炸弹的x值
var y1 = parseFloat($(item).css("top")); //炸弹的y值
//炸弹的宽和高
var w1 = parseFloat($(item).css("width"));
var h1 = parseFloat($(item).css("height"));
$(".ball").each(function(index2, item2) {
//方块左边的xy的位置
var bl = parseFloat($(item2).css("left"));
var bt = parseFloat($(item2).css("top"));
//方块的宽和高
var w2 = parseFloat($(item2).css("padding"));
var h2 = parseFloat($(item2).css("height"));
if (Math.abs((x1 + w1 / 2) - (bl + w2 / 2)) < Math.abs((w1 + w2) / 2) &&
Math.abs((y1 + h1 / 2) - (bt + w2 / 2)) < Math.abs((h1 + w2) / 2)) {
//$(item).arrt("src","img/爆炸.png");
$(item).remove();
score = score - 5;
$("#info").text("分数:" + score + "\n\n个数" + num + "个")
ballpadding = parseFloat($(".ball").css("padding")) - 2; //方块的padding
$(".ball").css("padding", ballpadding + "px");
}
})
})
}
//子弹碰撞炸弹
function firecrash() {
$(".bomb").each(function(index, item) {
//炸弹的xy的位置
var x1 = parseFloat($(item).css("left")); //炸弹的x值
var y1 = parseFloat($(item).css("top")); //炸弹的y值
//炸弹的宽和高
var w1 = parseFloat($(item).css("width"));
var h1 = parseFloat($(item).css("height"));
$(".fire").each(function(index2, item2) {
//子弹左边的xy的位置
var bl = parseFloat($(item2).css("left"));
var bt = parseFloat($(item2).css("top"));
//子弹的宽和高
var w2 = parseFloat($(item2).css("padding"));
var h2 = parseFloat($(item2).css("height"));
if (Math.abs((x1 + w1 / 2) - (bl + w2 / 2)) < Math.abs((w1 + w2) / 2) &&
Math.abs((y1 + h1 / 2) - (bt + w2 / 2)) < Math.abs((h1 + w2) / 2)) {
/* $(item).arrt("src","img/爆炸.png"); */
$(item).remove();
}
})
})
}
6.发射子弹
得到子弹的left,如果子弹的left大于地图宽与方块宽的差,子弹就消失
//移动子弹
function MoveFire() {
$(".fire").each(function(index, item) {
var left = parseFloat($(item).css("left"));
var max = smallbox.width() - ball.width();
if (left > max) {
$(item).remove();
} else {
$(item).css("left", (left + 3) + "px");
}
})
}
7.碰到边缘结束游戏
从键盘事件中判断,传参见键盘事件。
//游戏结束
function gameOver(pos, max) {
if (pos >= max) {
$("#num").text("分数:" + score + "\n吃了" + num + "个");
$("#endbox").show();
}
}
项目下载地址:https://download.csdn.net/download/weixin_59463732/20402846
|