1、常用同步加载AB包方式
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ABTest : MonoBehaviour
{
void Start()
{
AssetBundle ab = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/" + "mat");
GameObject obj = ab.LoadAsset("Sphere", typeof(GameObject)) as GameObject;
Instantiate(obj);
}
}
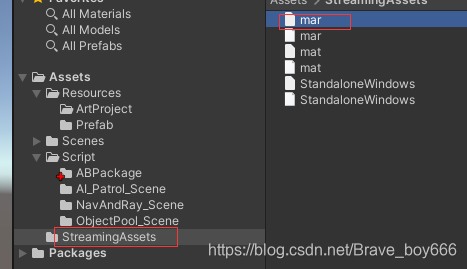
2、异步加载
用协程来达到延迟帧数加载资源
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ABTest : MonoBehaviour
{
public Image ima;
void Start()
{
StartCoroutine(LoadABRes("mat", "first"));
}
IEnumerator LoadABRes(string ABName, string resName)
{
AssetBundleCreateRequest abcr = AssetBundle.LoadFromFileAsync(Application.streamingAssetsPath + "/" + "mat");
yield return abcr;
AssetBundleRequest abq = abcr.assetBundle.LoadAssetAsync(resName,typeof(Sprite));
yield return abq;
ima.sprite = abq.asset as Sprite;
}
}
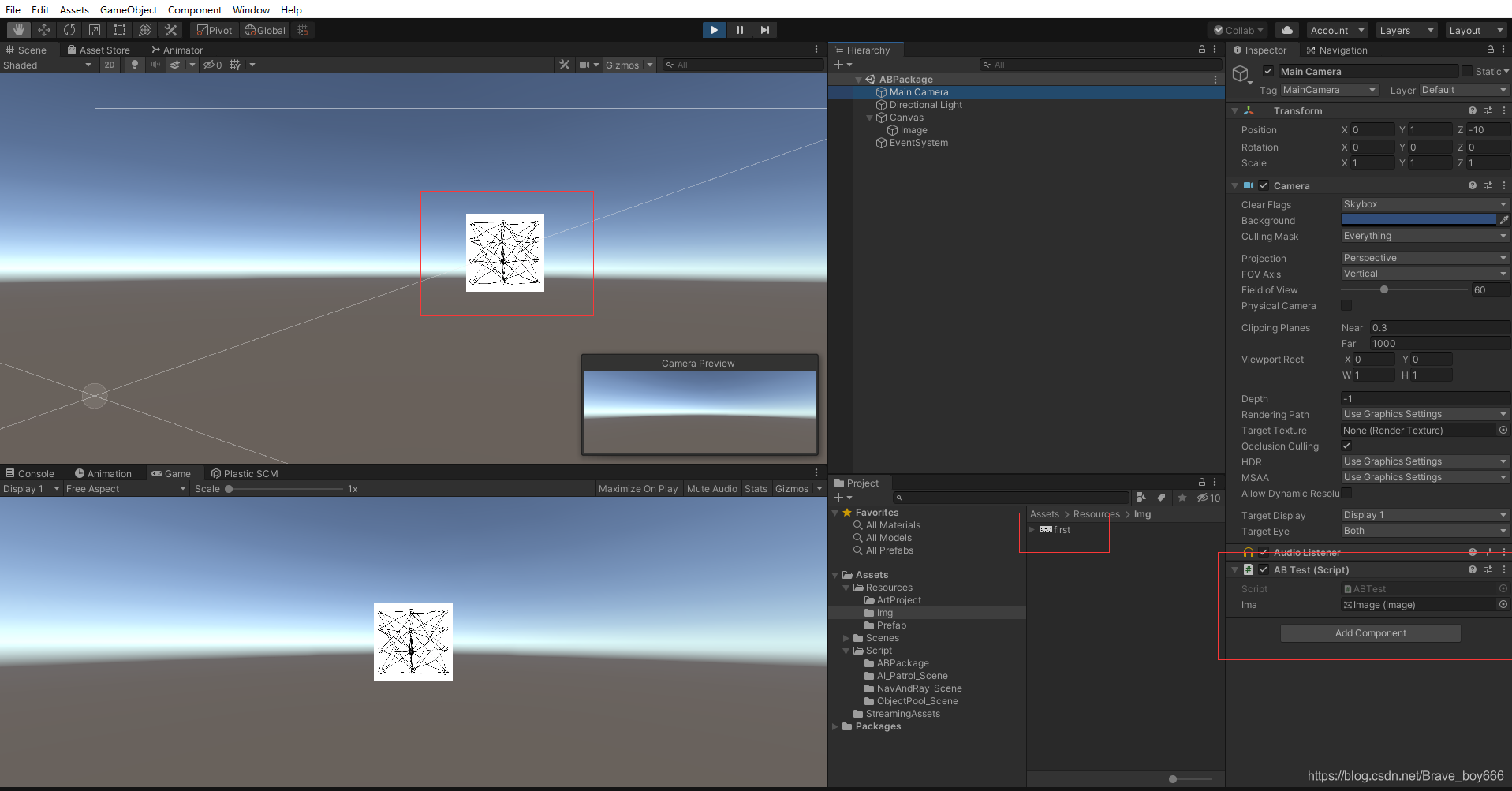
3、卸载包
卸载所有包
AssetBundle.UnloadAllAssetBundles(false);
卸载单个包
AssetBundle ab = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/" + "mat");
ab.Unload(false);
4、AB包依赖
比如我们给一个预制体上附一个材质球。然后预制体打包了,但材质球没放进去,我们AB包打完生成预制体就会发现材质丢失了,如下图 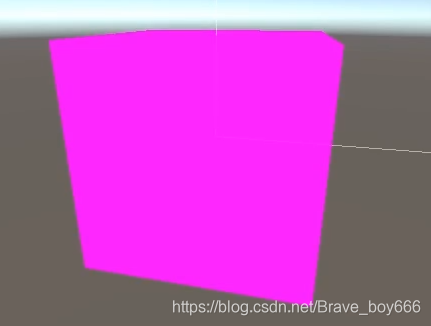 出现这种情况,要么把材质球跟预制体放在同一个包里,要么把材质球的那个包也加载进来  利用主包 获取依赖信息(依赖包关键信息)
|