一:功能点
1、对之前的推箱子代码进行优化。 2、新增使用了easyx图形工具库。
二:最终效果动图
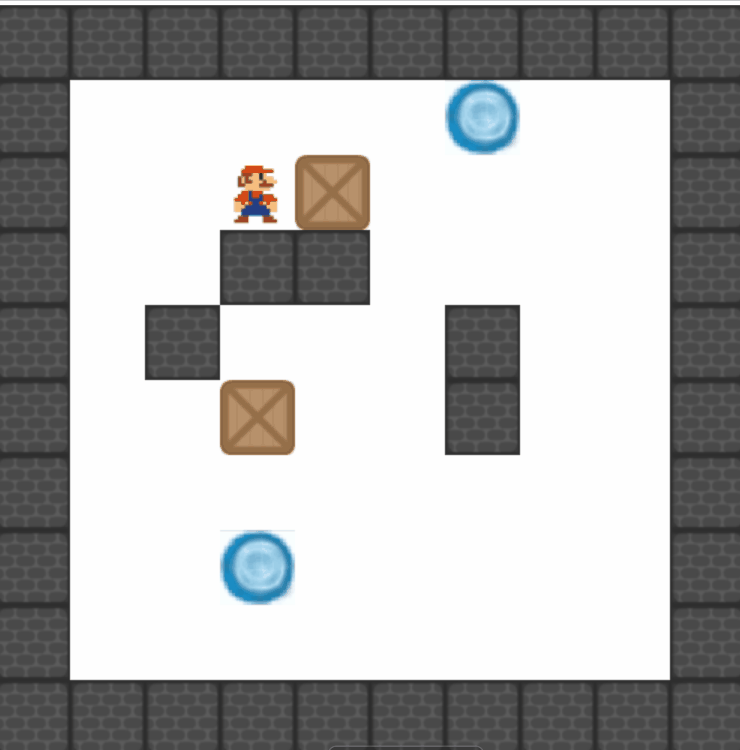
三:代码优化
在网上看到了别人写的代码才发现自己的代码有很多不足的地方。所以参考别人的代码进行了优化。由于代码中都做了注释,所以代码就不进行详细说明了,只说明一点,就是最主要的控制行走部分的代码,只用把一个方向的代码弄清楚了,剩下的三个方向的代码都差不多是一样的。这里直接附上代码。
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <graphics.h>
int map[10][10] = {1,1,1,1,1,1,1,1,1,1,
1,0,0,0,0,0,3,0,0,1,
1,0,0,5,4,0,0,0,0,1,
1,0,0,1,1,0,0,0,0,1,
1,0,1,0,0,0,1,0,0,1,
1,0,0,4,0,0,1,0,0,1,
1,0,0,0,0,0,0,0,0,1,
1,0,0,3,0,0,0,0,0,1,
1,0,0,0,0,0,0,0,0,1,
1,1,1,1,1,1,1,1,1,1,
};
IMAGE img[6];
void loadResource()
{
loadimage(img + 0, "0.bmp", 50, 50);
loadimage(img + 1, "1.bmp", 50, 50);
loadimage(img + 2, "3.bmp", 50, 50);
loadimage(img + 3, "4.bmp", 50, 50);
loadimage(img + 4, "5.bmp", 50, 50);
loadimage(img + 5, "7.bmp", 50, 50);
}
void PrintMap()
{
int i, j;
for (i = 0; i < 10; i++)
{
for (j = 0; j < 10; j++)
{
int x,y;
x = j * 50;
y = i * 50;
switch (map[i][j])
{
case 0: putimage(x, y, img + 0); break;
case 1: putimage(x, y, img + 1); break;
case 3: putimage(x, y, img + 2); break;
case 4: putimage(x, y, img + 3); break;
case 5:
case 8: putimage(x, y, img + 4); break;
case 7: putimage(x, y, img + 5); break;
default:
break;
}
}
printf("\n");
}
}
void PlayGame()
{
char temp;
int x,y;
int i,j;
for(x=0;x<10;x++)
{
for(y=0;y<10;y++)
{
if(map[x][y] == 5 || map[x][y] == 8)
{
i=x;
j=y;
}
}
}
temp = _getch();
switch (temp)
{
case 'W':
case 'w':
if (map[i - 1][j] == 0 || map[i - 1][j] == 3)
{
map[i][j] -= 5;
map[i - 1][j] += 5;
}
if (map[i - 1][j] == 4 || map[i - 1][j] == 7)
{
if (map[i - 2][j] == 0 || map[i - 2][j] == 3)
{
map[i][j] -= 5;
map[i - 1][j] += 1;
map[i - 2][j] += 4;
}
}
break;
case 'S':
case 's':
if (map[i + 1][j] == 0 || map[i + 1][j] == 3)
{
map[i][j] -= 5;
map[i + 1][j] += 5;
}
if (map[i + 1][j] == 4 || map[i + 1][j] == 7)
{
if (map[i + 2][j] == 0 || map[i + 2][j] == 3)
{
map[i][j] -= 5;
map[i + 1][j] += 1;
map[i + 2][j] += 4;
}
}
break;
case 'A':
case 'a':
if (map[i][j - 1] == 0 || map[i][j - 1] == 3)
{
map[i][j] -= 5;
map[i][j - 1] += 5;
}
if (map[i][j - 1] == 4 || map[i][j - 1] == 7)
{
if (map[i][j - 2] == 0 || map[i][j - 2] == 3)
{
map[i][j] -= 5;
map[i][j - 1] += 1;
map[i][j - 2] += 4;
}
}
break;
case 'D':
case 'd':
if (map[i][j + 1] == 0 || map[i][j + 1] == 3)
{
map[i][j] -= 5;
map[i][j + 1] += 5;
}
if (map[i][j + 1] == 4 || map[i][j + 1] == 7)
{
if (map[i][j + 2] == 0 || map[i][j + 2] == 3)
{
map[i][j] -= 5;
map[i][j + 1] += 1;
map[i][j + 2] += 4;
}
}
break;
default:
break;
}
}
int gamePass()
{
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
if (map[i][j] == 4)
{
return 0;
}
}
}
return 1;
}
int main()
{
loadResource();
initgraph(500, 500);
while (!gamePass())
{
PrintMap();
PlayGame();
system("cls");
}
closegraph();
printf("恭喜通关\r\n");
return 0;
}
代码参考文章:https://blog.csdn.net/qq_40655454/article/details/108046333
四:easyx图形工具库
这个图形工具库的作用请直接看下图: 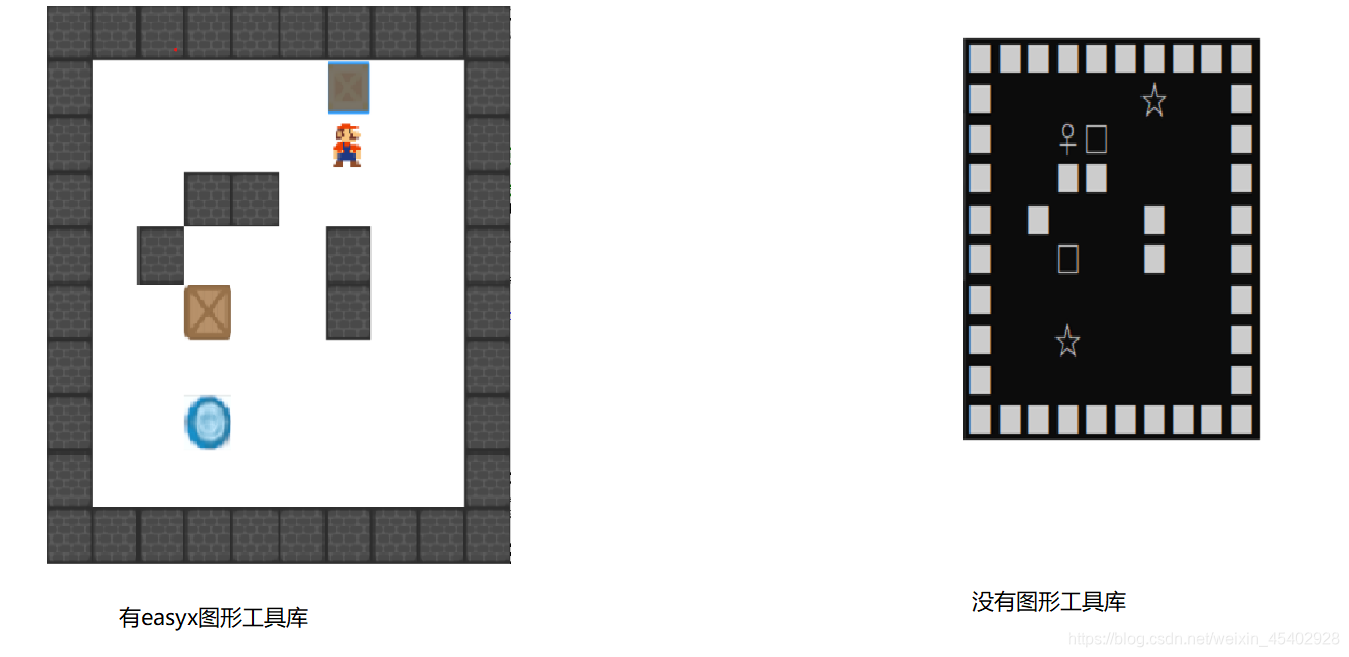
easyx的下载链接:https://easyx.cn/ 点击这里圈起来的下载EasyX就可以下载了。 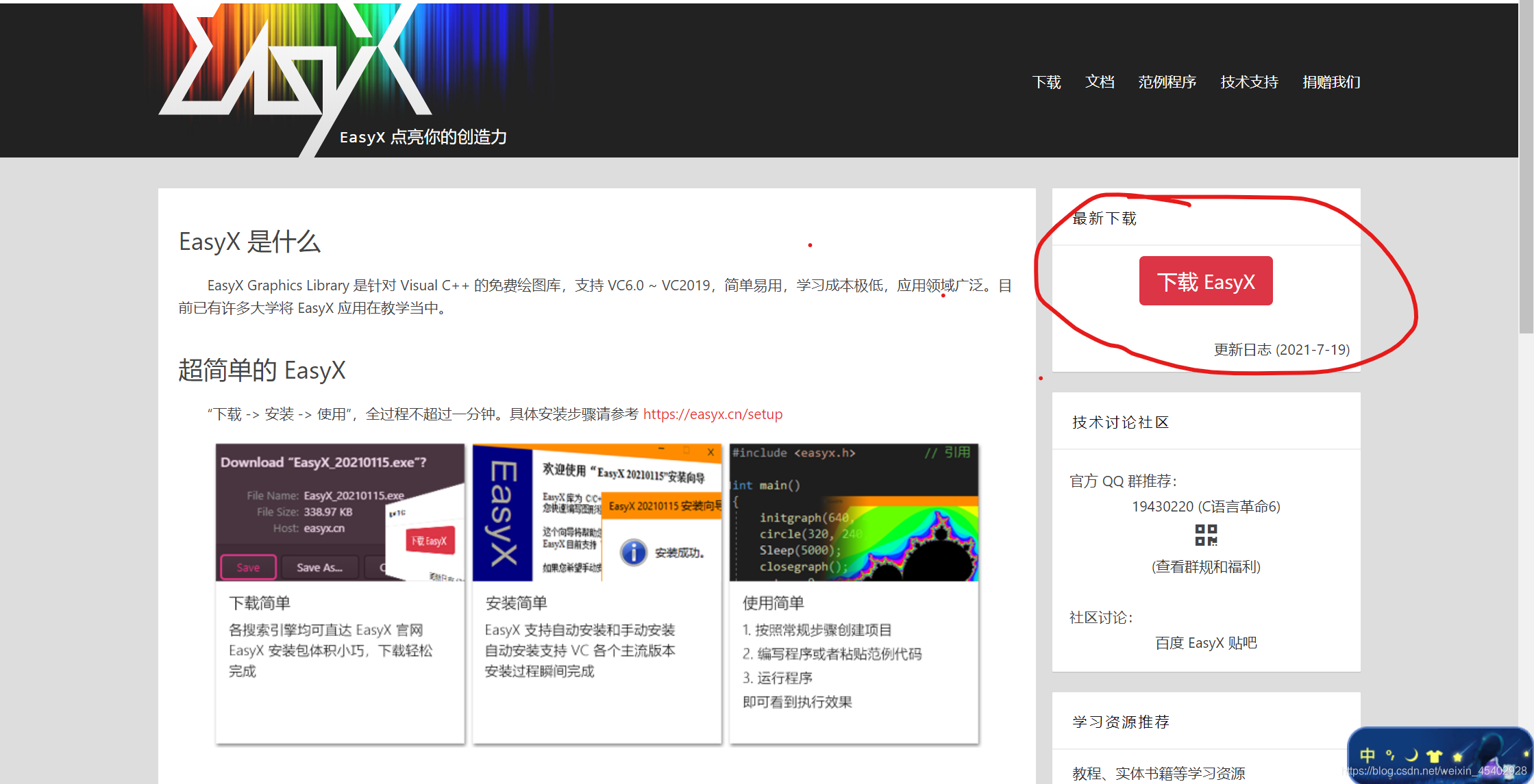
适用编译环境:Visual C++ 6.0,Visual Studio 2008 至 Visual Studio 2019
安装流程:点击下一步,只带最后到这个画面,点击安装即可。我电脑上装了vc++6.0,所以才能安装,如果没有的vc++6.0或者Visual Studio话是不能安装的。 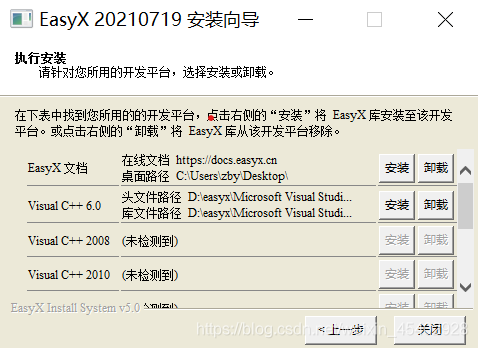 如何使用这个图形工具库: 第一:可在程序中申明#include <graphics.h> 头文件,从而来使用有关easyx的功能函数。可以参考文章https://blog.csdn.net/ych9527/article/details/111666487 第二:图片素材需要与c程序文件放在同一个目录下。
ps:如果有需要代码和图片素材,可以在评论区留下邮箱,我看到了就会回复。
|