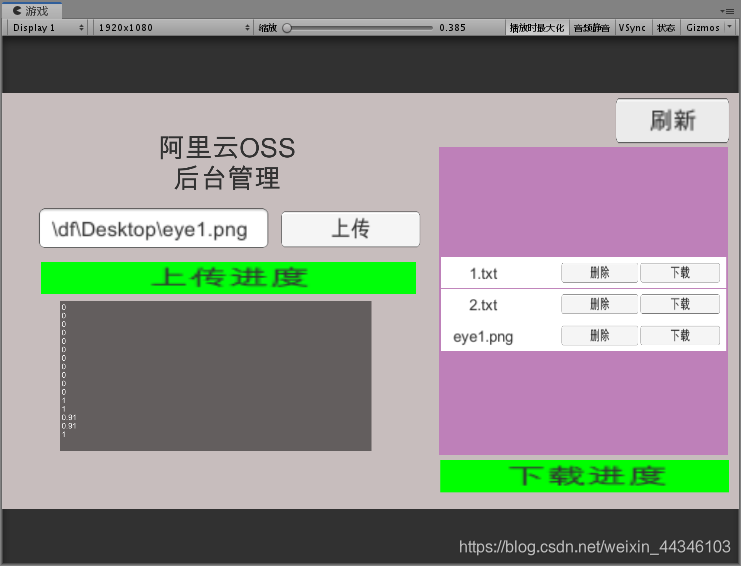
? ? ? ?可以实现上传下载文件,带有进度条显示进度,能显示阿里云端所存的文件,可以删除文件,并且可以打印错误信息和上传下载成功信息。
? ? ?首先需要下载好阿里云的OSS的SDK:https://help.aliyun.com/document_detail/32086.html?spm=a2c4g.11186623.6.1241.3c3b46a1BwiPVc,需要在unity中链接它的Aliyun.OSS.dll这个文件。然后需要申请阿里云的账号,并申请AccessKeyId,AccessKeySecret,和获得Endpoint和BucketName,不懂的可以百度一下。好的下面直接上代码。
//基本信息
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Config
{
public const string AccessKeyId = "你的AccessKeyId";
public const string AccessKeySecret = "你的AccessKeySecret";
public const string Endpoint = "你的Endpoint";
public const string BucketName = "你的BucketName";
}
?
//主控文件
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
using UnityEngine.UI;
using System.Threading;
using MyBaseFunc;
public class test : MonoBehaviour
{
public static test Instance;
private InputField input_LocalPath;
private Button btn_upload;
private Image img_process;
private Image download_process;
public Button btn_Refresh;
public GameObject go_Item;
private Transform parent;
// Start is called before the first frame update
private void Awake()
{
Instance = this;
input_LocalPath = transform.Find("OSS/input_LocalPath").GetComponent<InputField>();
btn_upload= transform.Find("OSS/btn_upload").GetComponent<Button>();
btn_upload.onClick.AddListener(OnUploadButtonClick);
img_process = transform.Find("OSS/img_process").GetComponent<Image>();
download_process = transform.Find("OSS/download_process").GetComponent<Image>();
btn_Refresh = transform.Find("btn_Refresh").GetComponent<Button>();
btn_Refresh.onClick.AddListener(OnRefreshButtonClick);
parent = transform.Find("list/ScrollRect/parent");
}
private void OnRefreshButtonClick()
{
for (int i = parent.childCount - 1; i >= 0; i--)
{
Destroy(parent.GetChild(i).gameObject);
}
List<string> fileNameList = GetObject.Instance.GetAllFileName();
for(int i=0;i<fileNameList.Count;i++)
{
GameObject go = Instantiate(go_Item, parent);
go.transform.GetChild(0).GetComponent<Text>().text = fileNameList[i];
}
}
void Start()
{
}
// Update is called once per frame
void Update()
{
if(Input.GetKeyDown(KeyCode.A))
{
GetObject.Instance.CreatEmptyFloder("123");
foreach(var item in GetObject.Instance.GetAllFileName())
{
Debug.Log(item);
}
}
}
private void OnUploadButtonClick()
{
if (input_LocalPath.text == null || input_LocalPath.text == "")
{
return;
}
PutObject.Instance.PutObjectProcessThread(PutWithProcessCallBake, input_LocalPath.text, Path.GetFileName(input_LocalPath.text));
}
private void PutSuccessCallBake()
{
Debug.Log("上传成功");
}
public void PutWithProcessCallBake(float process)
{
img_process.fillAmount = process;
Debug.Log(process);
}
public void GetWithProcessCallBake(float process)
{
download_process.fillAmount = process;
Debug.Log(process);
}
}
//上传
using Aliyun.OSS;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.Text;
using System.IO;
using Aliyun.OSS.Common;
using System.Threading;
using System;
public class PutObject : MonoBehaviour
{
public static PutObject Instance;
private OssClient client;
private Thread thread;
private string LocalPath;
private string FileName;
private Action PutsuccessCallBake;
private Action<float> PutWithProcessCallBake=null;
private float putPocess;
private bool isPutsecess = false;
private void Awake()
{
Instance = this;
client = new OssClient(Config.Endpoint, Config.AccessKeyId, Config.AccessKeySecret);
}
//字符串上传
public void PutObjWithStr(string FileName, string text)
{
try
{
byte[] b = Encoding.UTF8.GetBytes(text);
using (Stream stream = new MemoryStream(b))
{
client.PutObject(Config.BucketName, FileName, stream);
Debug.Log("字符串上传成功:" + text);
}
}
catch (OssException e)
{
Debug.Log("字符串上传错误:" + e);
}
catch (System.Exception e)
{
Debug.Log("字符串上传错误:" + e);
}
}
public void PutObjFromLocalThread(Action action, string LocalPath, string FileName)
{
this.LocalPath = LocalPath;
this.FileName = FileName;
PutsuccessCallBake = action;
thread = new Thread(PutObjFromLocal);
thread.Start();
}
//本地上传
private void PutObjFromLocal()
{
try
{
client.PutObject(Config.BucketName, FileName, LocalPath);
Debug.Log("本地上传成功:" + FileName);
isPutsecess = true;
thread.Abort();//关闭线程
}
catch (OssException e) //捕获oss异常
{
Debug.Log("本地上传错误:" + e.Message);
}
catch (System.Exception e) //捕获异常
{
Debug.Log("本地上传错误:" + e.Message);
}
}
private void FixedUpdate()
{
if (PutWithProcessCallBake != null)
{
PutWithProcessCallBake(putPocess);
if (putPocess == 1)
{
PutWithProcessCallBake = null;
putPocess = 0;
}
}
if (isPutsecess)
{
PutsuccessCallBake();
isPutsecess = false;
}
}
//进度条上传
public void PutObjectProcessThread(Action<float> action, string LocalPath, string FileName)
{
this.LocalPath = LocalPath;
this.FileName = FileName;
PutWithProcessCallBake = action;
thread = new Thread(PutObjectProcess);
thread.Start();
}
private void PutObjectProcess()
{
try
{
using (var fs = File.Open(LocalPath, FileMode.Open))
{
PutObjectRequest putObjectRequest = new PutObjectRequest(Config.BucketName,FileName,fs);
putObjectRequest.StreamTransferProgress += PutStreamProcess;
client.PutObject(putObjectRequest); //调用putObjectRequest
Debug.Log("进度上传成功");
}
}
catch(OssException e)
{
Debug.Log("进度上传有错误:" + e);
}
catch (Exception e)
{
Debug.Log("进度上传有错误:" + e);
}
finally
{
thread.Abort(); //关闭线程
}
}
private void PutStreamProcess(object sender,StreamTransferProgressArgs args)
{
putPocess = (args.TransferredBytes * 100 / args.TotalBytes) / 100.0f; //整数除以浮点型的数得到浮点型的数
}
}
//下载和删除
using Aliyun.OSS;
using Aliyun.OSS.Common;
using System;
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using UnityEngine;
using UnityEngine.Networking;
using System.Linq;
public class GetObject : MonoBehaviour
{
public static GetObject Instance;
private OssClient client;
private Thread getThread;
private Action<float> GetObjectProcessCallBack;
public string FilePath { get; private set; }
public string SavePath { get; private set; }
private float process = 0.0f;
private void Awake()
{
Instance = this;
client = new OssClient(Config.Endpoint, Config.AccessKeyId, Config.AccessKeySecret);
}
private void Update()
{
if(GetObjectProcessCallBack!=null)
{
GetObjectProcessCallBack(process);
}
if(process==1)
{
GetObjectProcessCallBack = null;
process = 0.0f;
}
}
//获取所有文件名
public List<string> GetAllFileName()
{
ObjectListing list = client.ListObjects(Config.BucketName);
List<string> nameList = new List<string>();
foreach(var item in list.ObjectSummaries)
{
nameList.Add(item.Key);
}
return nameList;
}
//创建文件夹
public void CreatEmptyFloder(string floderName)
{
try
{
using (var stream = new MemoryStream())
{
client.PutObject(Config.BucketName, floderName + "/", stream);
}
}
catch(OssException e)
{
Debug.Log("创建文件夹错误" + e.Message);
}
catch(System.Exception e)
{
Debug.Log("创建文件夹错误" + e.Message);
}
}
public void GetObjectProcessThread(Action<float> action, string filePath, string savePath)
{
GetObjectProcessCallBack = action;
FilePath = filePath;
SavePath = savePath;
getThread = new Thread(GetObjectProcess);
getThread.Start();
}
//下载文件
private void GetObjectProcess()
{
try //try{}catch{}捕获错误信息
{
GetObjectRequest getObjectRequest = new GetObjectRequest(Config.BucketName, FilePath);
getObjectRequest.StreamTransferProgress += StreamProcess;
OssObject result = client.GetObject(getObjectRequest);
using (var resultStream = result.Content) //var定义局部变量,必须赋值
{
using (var fs = File.Open(SavePath, FileMode.OpenOrCreate))
{
int length = (int)resultStream.Length;
byte[] bytes = new byte[length];
do
{
length = resultStream.Read(bytes, 0, length);
fs.Write(bytes, 0, length);
}
while (length != 0);
}
}
Debug.Log("进度下载成功");
}
catch(OssException e)
{
Debug.Log("带有进度条下载失败"+e.Message);
}
catch(Exception e)
{
Debug.Log("带有进度条下载失败"+e.Message);
}
finally
{
getThread.Abort();//关闭线程
}
}
private void StreamProcess(object sender, StreamTransferProgressArgs args)
{
process = (args.TransferredBytes * 100 / args.TotalBytes) / 100.0f; //整数除以浮点型的数得到浮点型的数
}
//删除单个文件
public void DeleteObject(string fliePath)
{
try
{
client.DeleteObject(Config.BucketName, fliePath);
Debug.Log("删除文件成功");
}
catch(OssException e)
{
Debug.Log("删除文件错误:" + e.Message);
}
catch(Exception e)
{
Debug.Log("删除文件错误:" + e.Message);
}
}
//删除多个文件
public void DeleteObjects(params string[] filePathArr)
{
try
{
List<string> filePathList = filePathArr.ToList();
DeleteObjectsRequest deleteObjectsRequest = new DeleteObjectsRequest(Config.BucketName, filePathList);
client.DeleteObjects(deleteObjectsRequest);
}
catch (OssException e)
{
Debug.Log("删除文件错误:" + e.Message);
}
catch (Exception e)
{
Debug.Log("删除文件错误:" + e.Message);
}
}
}
//云端文件显示
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class GetObjectButtonClick : MonoBehaviour
{
public Button btn_delete;
private void Awake()
{
GetComponentInChildren<Button>().onClick.AddListener(() =>
{
string filePath = transform.GetChild(0).GetComponent<Text>().text;
GetObject.Instance.GetObjectProcessThread(test.Instance.GetWithProcessCallBake, transform.GetChild(0).GetComponent<Text>().text, @"C:\Users\df\Desktop\" + filePath);
});
btn_delete = transform.Find("btn_delete").GetComponent<Button>();
btn_delete.onClick.AddListener(onDeleteButtonClick);
}
private void onDeleteButtonClick()
{
string filePath = transform.GetChild(0).GetComponent<Text>().text;
GetObject.Instance.DeleteObject(filePath);
}
}
未经允许,禁止转载。
|