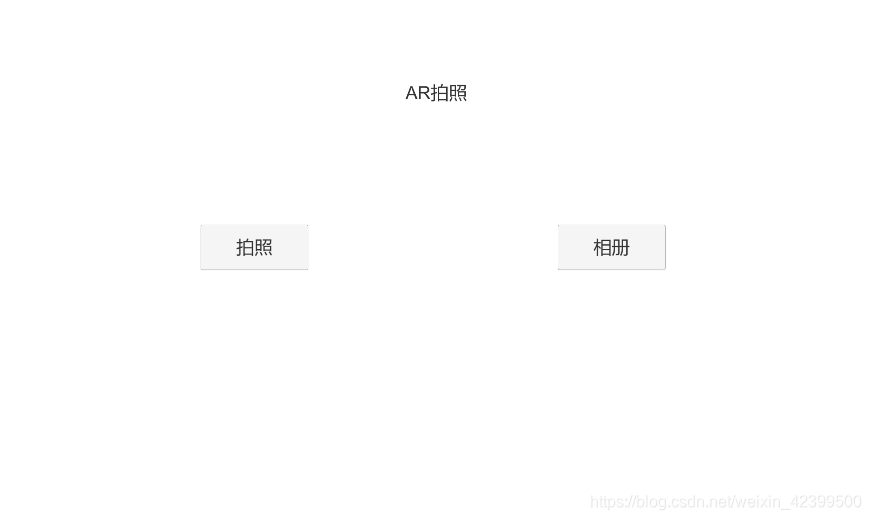
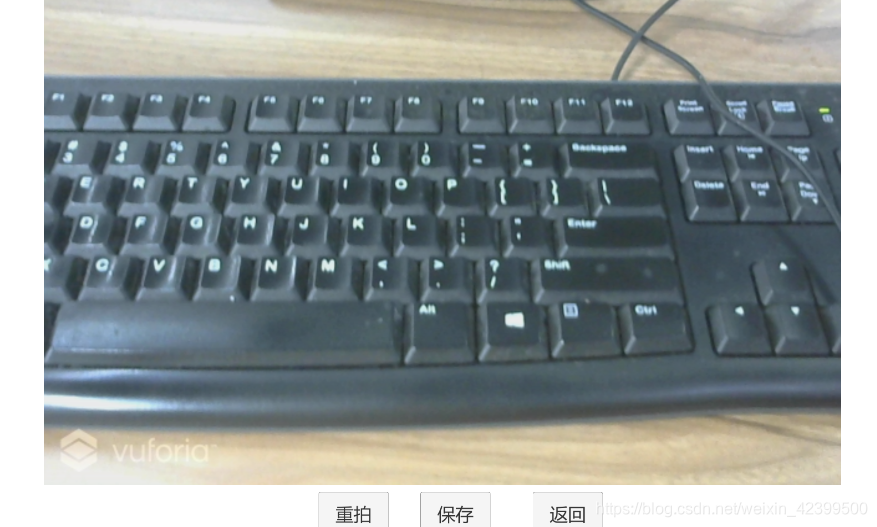
1.主体代码
using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using UnityEditor; using UnityEngine; using UnityEngine.Networking; using UnityEngine.UI;
public class Menu : MonoBehaviour { ??? //单例模式 ??? public static Menu instance; ??? /// <summary> ??? /// 主页界面 ??? /// </summary> ??? public GameObject zhuye; ??? /// <summary> ??? /// 模式界面 ??? /// </summary> ??? public GameObject moshi; ??? /// <summary> ??? /// 识别界面 ??? /// </summary> ??? public GameObject shibie; ??? /// <summary> ??? /// 拍照界面 ??? /// </summary> ??? public GameObject paizhao; ??? /// <summary> ??? ///?? 保存界面 ??? /// </summary> ??? public GameObject baocun; ??? /// <summary> ??? /// 提示文字 ??? /// </summary> ??? public Text tishi; ??? /// <summary> ??? /// 模型系数 ??? /// </summary> ??? public int number; ??? /// <summary> ??? /// 照片展示 ??? /// </summary> ??? public RawImage image; ??? /// <summary> ??? /// 合影动画 ??? /// </summary> ??? public GameObject[] donghua = new GameObject[] { }; ??? /// <summary> ??? /// 图片个数 ??? /// </summary> ??? private int pictrueNumber = 0; ??? /// <summary> ??? /// 截图 ??? /// </summary> ??? private Texture2D screenShot; ??? /// <summary> ??? /// 保存按钮 ??? /// </summary> ??? public Button baocunBtn; ??? /// <summary> ??? /// 保存提示板 ??? /// </summary> ??? public GameObject baocuntishi;
??? /// <summary> ??? /// 照片存储的地址 ??? /// </summary> ??? private string path;
??? /// <summary> ??? /// 照片展示的实例 ??? /// </summary> ??? public GameObject pref; ??? /// <summary> ??? /// 实例化的位置 ??? /// </summary> ??? public Transform content; ??? /// <summary> ??? /// 图片放大界面 ??? /// </summary> ??? public Image da; ??? /// <summary> ??? /// 图片放大展示 ??? /// </summary> ??? public RawImage daPictrue; ??? /// <summary> ??? /// 相册展示界面 ??? /// </summary> ??? public GameObject ImageLibrary;
??? void Awake() ??? { ??????? instance = this;
??? }
??? void Start() ??? { ??????? zhuyeMenu(); ??????? path = ScreenshotPath(); ??????? da.gameObject.SetActive(false); ??????? ImageLibrary.SetActive(false); ??? }
??? /// <summary> ??? /// 主页 ??? /// </summary> ??? public void zhuyeMenu() ??? { ??????? zhuye.SetActive(true); ??????? moshi.SetActive(false); ??????? shibie.SetActive(false); ??????? paizhao.SetActive(false); ??????? baocun.SetActive(false); ??????? number = 0; ??????? Debug.Log("主页!"); ??????? donghuNumber(); ??????? baocuntishi.SetActive(false); ??? }
??? /// <summary> ??? /// 模式 ??? /// </summary> ??? public void moshiMenu() ??? { ??????? zhuye.SetActive(false); ??????? moshi.SetActive(true); ??????? shibie.SetActive(false); ??????? paizhao.SetActive(false); ??????? baocun.SetActive(false); ??????? number = 0; ??????? donghuNumber(); ??? }
??? /// <summary> ??? /// 选择场景动画 ??? /// </summary> ??? /// <param name="index">系数</param> ??? public void xuanxze(int index) ??? { ??????? number = index; ??????? zhuye.SetActive(false); ??????? moshi.SetActive(false); ??????? shibie.SetActive(true); ??????? paizhao.SetActive(false); ??????? baocun.SetActive(false); ??????? paizhaoMenu(); ??? }
??? /// <summary> ??? /// 拍照模式 ??? /// </summary> ??? public void paizhaoMenu() ??? { ??????? zhuye.SetActive(false); ??????? moshi.SetActive(false); ??????? shibie.SetActive(false); ??????? paizhao.SetActive(true); ??????? baocun.SetActive(false); ??????? donghuNumber(); ??? }
??? /// <summary> ??? /// 保存模式 ??? /// </summary> ??? public void baocunMenu(Texture2D tx) ??? { ??????? zhuye.SetActive(false); ??????? moshi.SetActive(false); ??????? shibie.SetActive(false); ??????? paizhao.SetActive(false); ??????? baocun.SetActive(true); ??????? baocunBtn.interactable = true; ??????? image.texture = tx; ??? }
??? /// <summary> ??? /// 出现哪个物体 ??? /// </summary> ??? public void donghuNumber() ??? {
??????? for (int i = 0; i < donghua.Length; i++) ??????? { ??????????? if (donghua[i].activeSelf == true) ??????????? { ??????????????? donghua[i].SetActive(false); ??????????? } ??????? }
??????? if (number > 0) ??????? { ??????????? donghua[number - 1].SetActive(true); ??????? }
??? }
??? /// <summary> ??? /// 获取图片 ??? /// </summary> ??? public void GetPictrue() ??? { ??????? paizhao.SetActive(false); ??????? StartCoroutine(CaptureScreen()); ??? } ??? public IEnumerator CaptureScreen() ??? { ??????? yield return new WaitForSeconds(0.1f); ??????? yield return new WaitForEndOfFrame();
??????? var rect = new Rect(0, 0, Screen.width, Screen.height); ??????? Texture2D tx = new Texture2D((int)rect.width, (int)rect.height, TextureFormat.RGB24, false); ??????? tx.ReadPixels(rect, 0, 0); ??????? tx.Apply(); ??????? screenShot = tx; ??????? baocunMenu(tx); ??? }
??? /// <summary> ??? /// 保存图片 ??? /// </summary> ??? public void SavePictrue() ??? { ??????? baocunBtn.interactable = false;
??????? string dirPath = path;
??????? string names = GetCurTime(); ??????? string filename = path + names + ".png"; ??????? byte[] bytes = screenShot.EncodeToPNG(); ??????? File.WriteAllBytes(filename, bytes);
??????? Debug.Log("保存照片:" + names + ".png"); ??????? baocuntishi.SetActive(true); ??????? StartCoroutine(buxian()); ??? }
??? /// <summary> ??? /// 重新拍照 ??? /// </summary> ??? public void RefreshPictrue() ??? { ??????? paizhaoMenu(); ??? } ??? /// <summary> ??? /// 保存提示板不显示 ??? /// </summary> ??? /// <returns></returns> ??? IEnumerator buxian() ??? { ??????? yield return new WaitForSeconds(2f); ??????? baocuntishi.SetActive(false); ??? }
??? /// <summary> ??? /// 图片保存路径 ??? /// </summary> ??? /// <returns></returns> ??? public string ScreenshotPath() ??? {
??????? string filePath = ""; ??????? switch (Application.platform) ??????? { ??????????? case RuntimePlatform.WindowsEditor: ??????????????? filePath = Application.streamingAssetsPath + "/Pictrue/"; ??????????????? Debug.Log("模式:" + "RuntimePlatform.WindowsEditor"); ??????????????? break; ??????????? case RuntimePlatform.OSXEditor: ??????????????? Debug.Log("模式:" + "RuntimePlatform.OSXEditor"); ??????????????? filePath = Application.persistentDataPath + "/Pictrue/";
??????????????? break; ??????????? case RuntimePlatform.Android: ??????????????? Debug.Log("模式:" + "RuntimePlatform.Android"); ??????????????? filePath = Application.persistentDataPath + "/Pictrue/"; ??????????????? break; ??????????? case RuntimePlatform.IPhonePlayer: ??????????????? Debug.Log("模式:" + "RuntimePlatform.IPhonePlayer"); ??????????????? filePath = Application.persistentDataPath + "/Pictrue/"; ??????????????? break; ??????? }
??????? if (!Directory.Exists(filePath)) ??????? { ??????????? Directory.CreateDirectory(filePath); ??????? } ??????? return filePath;
??? } ? ? ??? /// <summary> ??? /// 获取当前年月日时分秒,如20181001444 ??? /// </summary> ??? /// <returns></returns> ??? string GetCurTime() ??? { ??????? return DateTime.Now.Year.ToString() + DateTime.Now.Month.ToString() + DateTime.Now.Day.ToString() ??????????? + DateTime.Now.Hour.ToString() + DateTime.Now.Minute.ToString() + DateTime.Now.Second.ToString(); ??? }
??? /// <summary> ??? /// 打开文件夹 ??? /// </summary> ??? public void OpenFile() ??? { ??????? string output = ScreenshotPath(); ??????? if (!Directory.Exists(output)) ??????? { ??????????? Directory.CreateDirectory(output); ??????? } ??????? output = output.Replace("/", "\\"); ??????? System.Diagnostics.Process.Start("explorer.exe", output); ??? }
??? /// <summary> ??? /// 点击调出相册 ??? /// </summary> ??? public void Xiangce() ??? { ??????? ImageLibrary.SetActive(true); ??????? string[] dirs = Directory.GetFiles(path, "*.png"); ??????? int i = 0; ??????? while (i < dirs.Length) ??????? { ??????????? Debug.Log(dirs[i]); ??????????? //一定要加"file:///",否则安卓端找不到位置,加载不出来图片 ??????????? StartCoroutine(GetTexture("file:///" + dirs[i])); ??????????? i++; ??????? } ??? } ??? /// <summary> ??? /// 异步加载图片 ??? /// </summary> ??? /// <param name="file">地址</param> ??? /// <returns></returns> ??? IEnumerator GetTexture(string file) ??? { ??????? //这里的地址可以填本地文件地址? file://[文件路径] ??????? using (UnityWebRequest www = UnityWebRequestTexture.GetTexture(file)) ??????? { ??????????? yield return www.SendWebRequest(); ??????????? if (www.result == UnityWebRequest.Result.ConnectionError) ??????????? { ??????????????? Debug.Log(www.error); ??????????? } ??????????? else ??????????? { ??????????????? /*这里把贴图转换的过程放到了后台线程来完成, ??????????????? 并且相比于直接脚本加载图片做了优化,减少了内存分配*/ ??????????????? Texture2D tx = DownloadHandlerTexture.GetContent(www); ??????????????? RectTransform xin = (Instantiate(pref) as GameObject).GetComponent<RectTransform>(); ??????????????? xin.transform.SetParent(content); ??????????????? xin.transform.localScale = new Vector3(1, 1, 1); ??????????????? xin.transform.GetComponent<Pictrue>().xianshi(tx); ??????????????? ContentSize(); ??????????? } ??????? }
??? } ??? /// <summary> ??? /// 滑动条的大小设置 ??? /// </summary> ??? public void ContentSize() ??? { ??????? int number = content.childCount; ??????? if (number > 12) ??????? { ??????????? int index = (number - 12) / 4;
??????????? if (number % 4 == 0) ??????????? { ??????????????? content.GetComponent<RectTransform>().sizeDelta = new Vector2(1900, 960 + index * 360); ??????????? } ??????????? else ??????????? { ??????????????? content.GetComponent<RectTransform>().sizeDelta = new Vector2(1900, 960 + (index + 1) * 360); ??????????? }
??????? } ??????? else ??????? { ??????????? content.GetComponent<RectTransform>().sizeDelta = new Vector2(1900, 960); ??????? } ??? }
??? /// <summary> ??? /// 图片放大 ??? /// </summary> ??? public void FangDa(Texture2D tx) ??? { ??????? daPictrue.texture = tx; ??????? da.gameObject.SetActive(true); ??? } ??? /// <summary> ??? /// 关闭放大 ??? /// </summary> ??? public void DaQuit() ??? { ??????? da.gameObject.SetActive(false); ??? }
??? /// <summary> ??? /// 关闭相册 ??? /// </summary> ??? public void XiangceClearn() ??? { ??????? ImageLibrary.SetActive(false); ??????? foreach (Transform go in content) ??????? { ??????????? Destroy(go.gameObject); ??????? } ??? }
}
2.实例代码
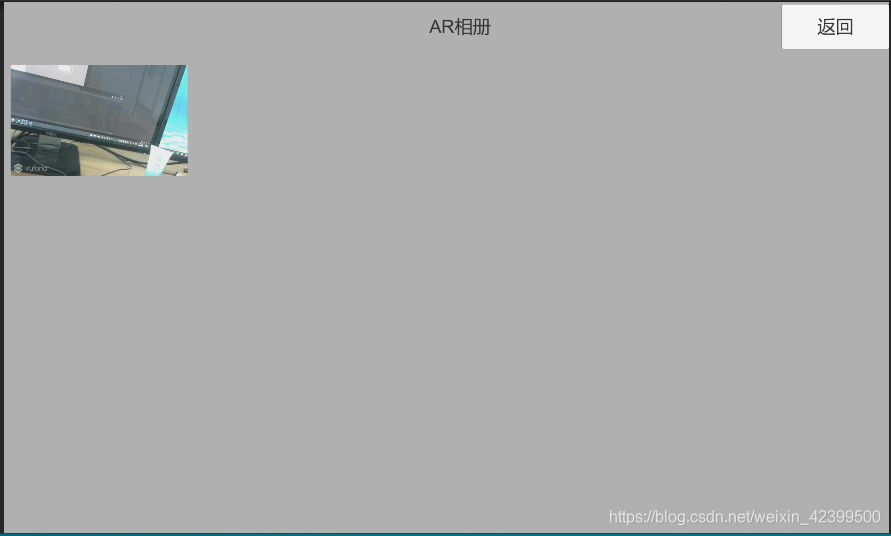
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI;
public class Pictrue : MonoBehaviour {
??? Texture2D myTextrue2d;
??? public RawImage raw;
??? void Start() ??? { ??????? //raw = transform.GetComponent<RawImage>(); ??? }
??? public void xianshi(Texture2D tx) ??? { ??????? myTextrue2d = tx; ??????? raw.texture = myTextrue2d; ??? }
??? public void OnDisable() ??? { ??????? Destroy(myTextrue2d); ??? }
??? public void Click() ??? { ??????? Menu.instance.FangDa(myTextrue2d); ??? }
}

?PLAYER SETTING :这个设置一定别忘记了
备注:无论是pc端还是安卓端都好使,亲测!
|