代码很简单没有难度,都有注解,随便 康一康 就会了。
菜单编辑 按钮点击 构建 AssetBundle 包集
有一点需要注意:CreateAssetBundle_ZH 脚本需要安置在Asset 下 Editor文件夹下
不然无法正确执行
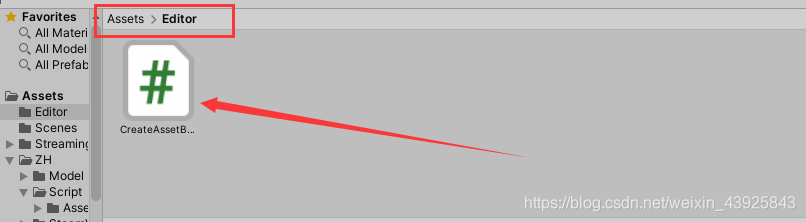
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEditor;
using UnityEngine;
public class CreateAssetBundle_ZH : MonoBehaviour
{
[MenuItem("AssetBundles/Build AssetBundles")]
static void BuildAllAssetBundles()
{
string _AssetBundleDirectory = Application.streamingAssetsPath + "//AssetBundles";
if (!Directory.Exists(_AssetBundleDirectory))
{
Directory.CreateDirectory(_AssetBundleDirectory);
}
BuildPipeline.BuildAssetBundles(_AssetBundleDirectory, BuildAssetBundleOptions.ChunkBasedCompression, BuildTarget.StandaloneWindows64);
#if UNITY_EDITOR
AssetDatabase.Refresh();
#endif
}
}
正确放置之后就需要对你要使用的资源进行分包处理了
比如我这个:我对当前 Cube 把它放在了 model 里面这个AB包集里
AB包集名字 是自己取得 如果你没有就自己 New 一个
注意:如果你要使用的资源没有赋予 AB包集的话 是没有办法生成AssetBundle 文件的
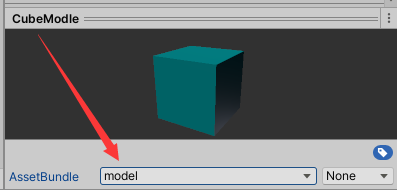
点击菜单栏 AssetBundles按钮下的Build AssetBundles 按钮就可以生成你自己的AssetBundle文件了
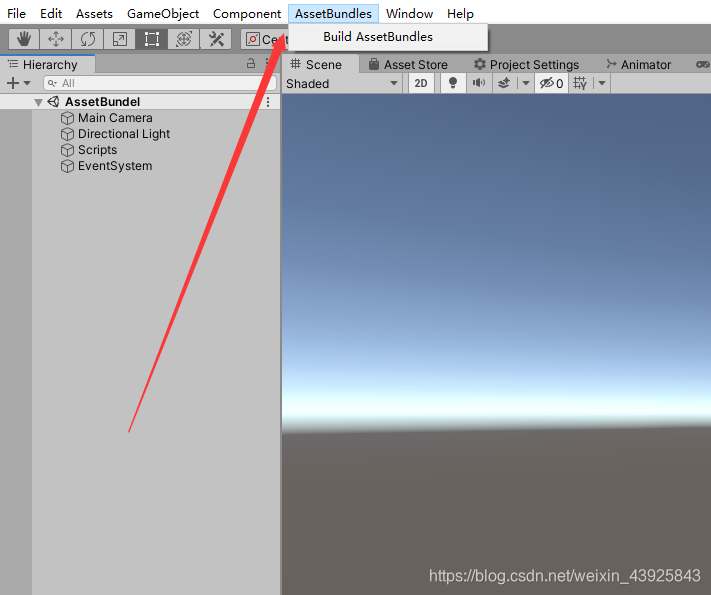
AssetBundle 基础功能
AssetBundle 加载:需要注意AB 包加载需要填写对的路径以及包集名字(小写)
正确加载 AB 包集后可以使用 LoadAsset() 方法加载当前包集中的具体资源
注意拿到具体资源后需要实例化 不然你就只是拿到
当前 AB 包集使用完毕之后注意 卸载 不然会报错
因为 AB 包的特性就是无法重复加载
AssetBundle 包集
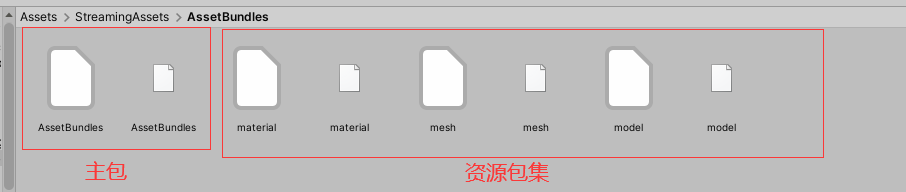
AssetBundle.LoadAsset() 默认返回值是Object 类型

这个就是主包中固定文件里的内容:里面包含了各个 AB 包集 的依赖关系
AssetBundleManifest _AssetBundleManifest = _AssetBundleMain.LoadAsset<AssetBundleManifest>("AssetBundleManifest");
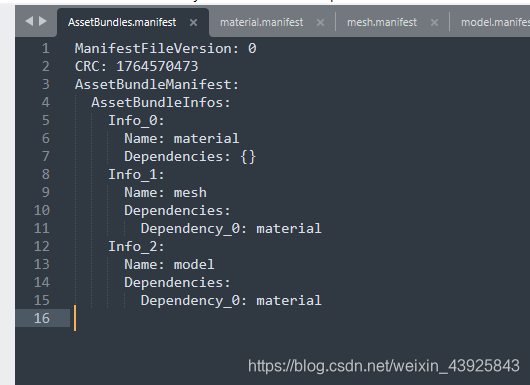
AssetBundle 没有依赖项 加载
public void LoadAssetBundle()
{
AssetBundle _AssetBundle = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/AssetBundles/"+ "model");
GameObject _Gam = _AssetBundle.LoadAsset<GameObject>("SphereModel") ;
GameObject _Gam02 = _AssetBundle.LoadAsset("CubeModle", typeof(GameObject)) as GameObject;
Instantiate(_Gam);
Instantiate(_Gam02);
_AssetBundle.Unload(false);
}
AssetBundle 没有依赖项 异步加载
public IEnumerator LoadAssetBundle(string _AssetBundleName,string _PrefabName)
{
AssetBundleCreateRequest _AssetBundleCreateRequest = AssetBundle.LoadFromFileAsync(Application.streamingAssetsPath + "/AssetBundles/" + _AssetBundleName);
yield return _AssetBundleCreateRequest;
AssetBundleRequest _Abr = _AssetBundleCreateRequest.assetBundle.LoadAssetAsync(_PrefabName,typeof(GameObject));
GameObject _Gam = _Abr.asset as GameObject;
Instantiate(_Gam);
AssetBundle.UnloadAllAssetBundles(false);
yield return null;
}
AssetBundle 有依赖项 加载
public void LoadAssetBundleDepend(string _AssetBundlesPathName, string _AssetBundlesName, string _PrefabName)
{
AssetBundle _AssetBundleMain = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/AssetBundles/" + _AssetBundlesPathName);
AssetBundleManifest _AssetBundleManifest = _AssetBundleMain.LoadAsset<AssetBundleManifest>("AssetBundleManifest");
string[] _DependStr = _AssetBundleManifest.GetAllDependencies(_AssetBundlesName);
if (_DependStr.Length > 0)
{
AssetBundle _NewAssetBundle = null;
for (int j = 0; j < _DependStr.Length; j++)
{
_NewAssetBundle = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/AssetBundles/" + _DependStr[j]);
}
if (_NewAssetBundle != null)
{
AssetBundle _NewAssetBundleSubset;
_NewAssetBundleSubset = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/AssetBundles/" + _AssetBundlesName);
GameObject _Gam = _NewAssetBundleSubset.LoadAsset(_PrefabName, typeof(GameObject)) as GameObject;
Instantiate(_Gam);
}
}
else
{
AssetBundle _NewAssetBundleSubset;
_NewAssetBundleSubset = AssetBundle.LoadFromFile(Application.streamingAssetsPath + "/AssetBundles/" + _AssetBundlesName);
GameObject _Gam = _NewAssetBundleSubset.LoadAsset(_PrefabName, typeof(GameObject)) as GameObject;
Instantiate(_Gam);
}
}
AssetBundle 卸载
_AssetBundle.Unload(false);
AssetBundle.UnloadAllAssetBundles(false);
AssetBundle 包管理器
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
public class AssetBundleManager_ZH : MonoBehaviour
{
public static AssetBundleManager_ZH _AssetBundleManager;
private AssetBundle _AssetBundleMain = null;
private AssetBundleManifest _AssetBundleManifest = null;
private Dictionary<string, AssetBundle> _AssetBundleDic = new Dictionary<string, AssetBundle>();
public string _AssetBundlePath
{
get
{
return Application.streamingAssetsPath + "/AssetBundles/";
}
set
{
_AssetBundlePath = value;
}
}
public string _AssetBundleMainName
{
get
{
return "AssetBundles";
}
set
{
_AssetBundleMainName = value;
}
}
private void Awake()
{
_AssetBundleManager = this;
}
#region AssetBundle 资源同步加载
public Object LoadAssetBundleSync(string _AssetBundleName, string _ResName)
{
LoadAB(_AssetBundleName);
Object _AssetBundleObject = _AssetBundleDic[_AssetBundleName].LoadAsset(_ResName);
if (_AssetBundleObject as GameObject)
{
return Instantiate(_AssetBundleObject);
}
else
{
return _AssetBundleObject;
}
}
public Object LoadAssetBundleSync(string _AssetBundleName, string _ResName, System.Type _Type)
{
LoadAB(_AssetBundleName);
Object _AssetBundleObject = _AssetBundleDic[_AssetBundleName].LoadAsset(_ResName, _Type);
if (_AssetBundleObject as GameObject)
{
return Instantiate(_AssetBundleObject);
}
else
{
return _AssetBundleObject;
}
}
public T LoadAssetBundleSync<T>(string _AssetBundleName, string _ResName) where T : Object
{
LoadAB(_AssetBundleName);
T _AssetBundleObject = _AssetBundleDic[_AssetBundleName].LoadAsset<T>(_ResName);
if (_AssetBundleObject as GameObject)
{
return Instantiate(_AssetBundleObject);
}
else
{
return _AssetBundleObject;
}
}
#endregion
#region AssetBundle 资源异步加载
public void LoadResAsync(string _AssetBundleName, string _ResName,UnityAction<Object> _CallBack)
{
StartCoroutine(ReallyLoadResAsync(_AssetBundleName, _ResName, _CallBack));
}
private IEnumerator ReallyLoadResAsync(string _AssetBundleName, string _ResName, UnityAction<Object> _CallBack)
{
LoadAB(_AssetBundleName);
AssetBundleRequest _ABR = _AssetBundleDic[_AssetBundleName].LoadAssetAsync(_ResName);
yield return _ABR;
if (_ABR.asset as GameObject)
{
_CallBack(Instantiate(_ABR.asset));
}
else
{
_CallBack(_ABR.asset);
}
}
public void LoadResAsync(string _AssetBundleName, string _ResName, System.Type _Type,UnityAction<Object> _CallBack)
{
StartCoroutine(ReallyLoadResAsync(_AssetBundleName, _ResName, _Type, _CallBack));
}
private IEnumerator ReallyLoadResAsync(string _AssetBundleName, string _ResName, System.Type _Type, UnityAction<Object> _CallBack)
{
LoadAB(_AssetBundleName);
AssetBundleRequest _ABR = _AssetBundleDic[_AssetBundleName].LoadAssetAsync(_ResName, _Type);
yield return _ABR;
if (_ABR.asset as GameObject)
{
_CallBack(Instantiate(_ABR.asset));
}
else
{
_CallBack(_ABR.asset);
}
}
public void LoadResAsync<T>(string _AssetBundleName, string _ResName, UnityAction<T> _CallBack)where T:Object
{
StartCoroutine(ReallyLoadResAsync<T>(_AssetBundleName, _ResName, _CallBack));
}
private IEnumerator ReallyLoadResAsync<T>(string _AssetBundleName, string _ResName, UnityAction<T> _CallBack) where T : Object
{
LoadAB(_AssetBundleName);
AssetBundleRequest _ABR = _AssetBundleDic[_AssetBundleName].LoadAssetAsync(_ResName);
yield return _ABR;
if (_ABR.asset as GameObject)
{
_CallBack(Instantiate(_ABR.asset)as T);
}
else
{
_CallBack(_ABR.asset as T);
}
}
#endregion
public void LoadAB(string _AssetBundleName)
{
if (_AssetBundleMain == null)
{
_AssetBundleMain = AssetBundle.LoadFromFile(_AssetBundlePath + _AssetBundleMainName);
_AssetBundleManifest = _AssetBundleMain.LoadAsset<AssetBundleManifest>("AssetBundleManifest");
}
string[] _DependStr = _AssetBundleManifest.GetAllDependencies(_AssetBundleName);
if (_DependStr.Length > 0)
{
AssetBundle _NewAssetBundleSubset;
for (int i = 0; i < _DependStr.Length; i++)
{
if (!_AssetBundleDic.ContainsKey(_DependStr[i]))
{
_NewAssetBundleSubset = AssetBundle.LoadFromFile(_AssetBundlePath + _DependStr[i]);
_AssetBundleDic.Add(_DependStr[i], _NewAssetBundleSubset);
}
}
if (!_AssetBundleDic.ContainsKey(_AssetBundleName))
{
_NewAssetBundleSubset = AssetBundle.LoadFromFile(_AssetBundlePath + _AssetBundleName);
_AssetBundleDic.Add(_AssetBundleName, _NewAssetBundleSubset);
}
}
}
public void UnLoadAssetBuindle(string _AssetBundleName)
{
if (_AssetBundleDic.ContainsKey(_AssetBundleName))
{
_AssetBundleDic[_AssetBundleName].Unload(false);
_AssetBundleDic.Remove(_AssetBundleName);
}
}
public void UnLoadAllAssetBuindles()
{
AssetBundle.UnloadAllAssetBundles(false);
_AssetBundleDic.Clear();
_AssetBundleMain = null;
_AssetBundleManifest = null;
_AssetBundlePath = null;
_AssetBundleMainName = null;
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Q))
{
LoadAssetBundleSync("model", "CubeModle");
LoadAssetBundleSync("model", "SphereModel",typeof(GameObject));
LoadAssetBundleSync<GameObject>("model", "CylinderModle");
}
if (Input.GetKeyDown(KeyCode.E))
{
LoadResAsync("model", "CubeModle",(_Obj=>
{
(_Obj as GameObject).transform.position = new Vector3((_Obj as GameObject).transform.position.x, 3, (_Obj as GameObject).transform.position.z);
}));
LoadResAsync("model", "SphereModel",typeof(GameObject), (_Obj =>
{
(_Obj as GameObject).transform.position = new Vector3((_Obj as GameObject).transform.position.x, 3, (_Obj as GameObject).transform.position.z);
}));
LoadResAsync<GameObject>("model", "CylinderModle", (_Obj =>
{
_Obj .transform.position = new Vector3(_Obj.transform.position.x, 3, _Obj.transform.position.z);
}));
}
}
}
搭载运行
脚本搭载
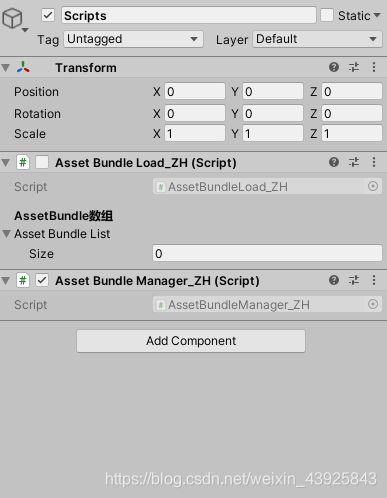
基础运行
点击两次也不会报错说明运行良好可以直接使用
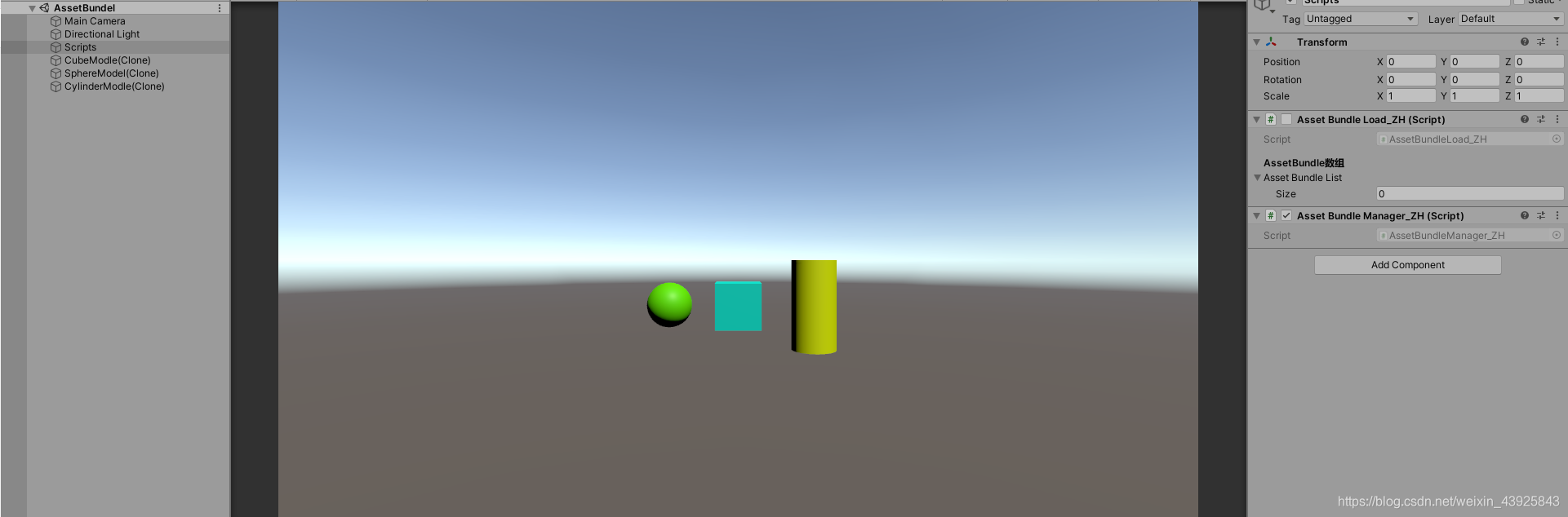
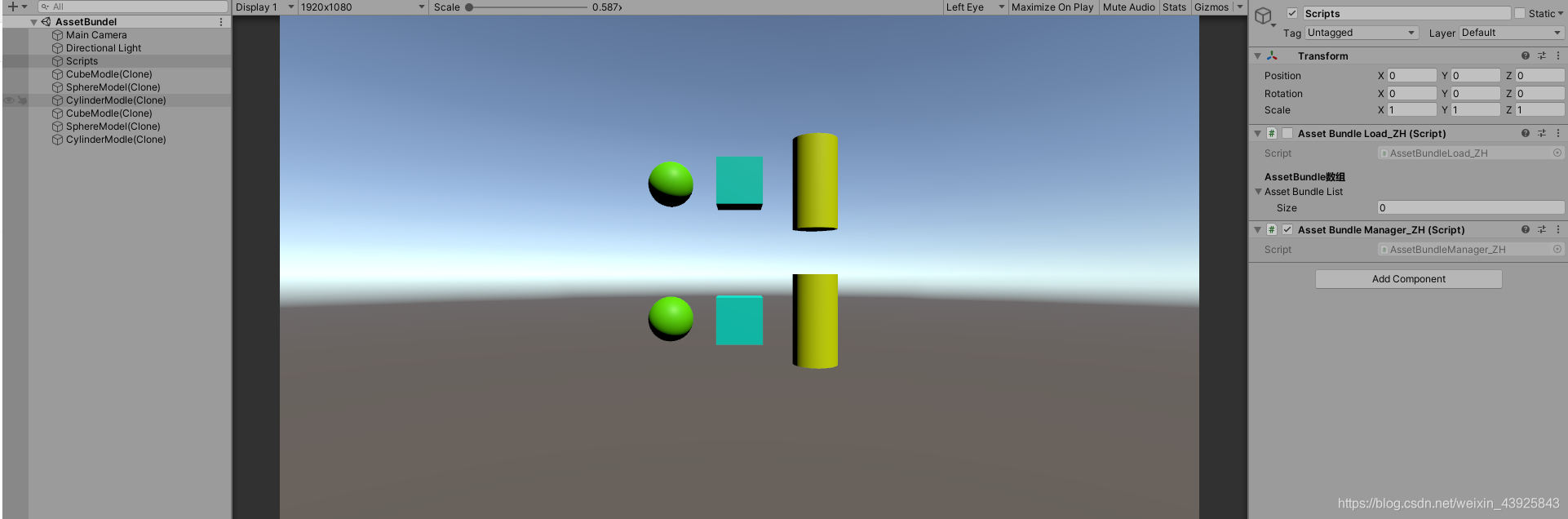
暂时先这样吧,如果有时间的话就会更新,实在看不明白就留言,看到我会回复的。 路漫漫其修远兮,与君共勉。
|