简介
本序列由澳大利亚的一个游戏引擎爱好者整理制作,使用了前瞻的语言技术, 经常多次的修改制作,一个个小的知识点贯通,非常具有引导学习意义!
Bilibili站转载学习,非个人研究,鸣谢!
原作者Github Bilibili直达链接 YouTube直达链接
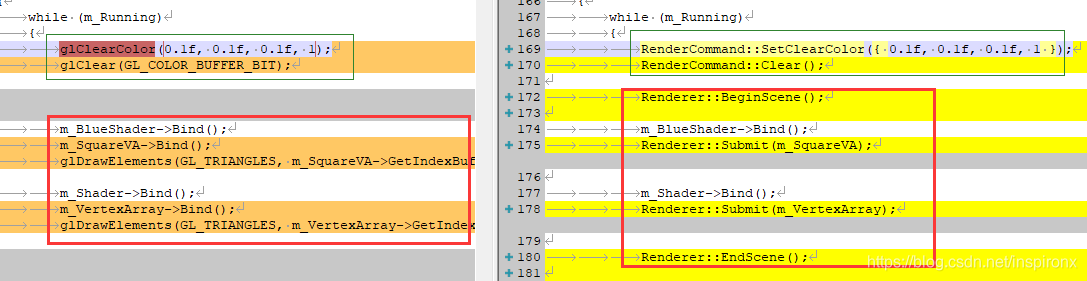 将每次渲染帧的工作统一起来,封装成事务的形式,方便之后对渲染帧的二次处理;
#pragma once
#include <glm/glm.hpp>
#include "VertexArray.h"
namespace Aurora {
class RendererAPI
{
public:
enum class API
{
None = 0, OpenGL = 1
};
public:
virtual void SetClearColor(const glm::vec4& color) = 0;
virtual void Clear() = 0;
virtual void DrawIndexed(const std::shared_ptr<VertexArray>& vertexArray) = 0;
inline static API GetAPI() { return s_API; }
private:
static API s_API;
};
}
#include "aopch.h"
#include "RendererAPI.h"
namespace Aurora {
RendererAPI::API RendererAPI::s_API = RendererAPI::API::OpenGL;
}
#pragma once
#include "Aurora/Renderer/RendererAPI.h"
namespace Aurora {
class OpenGLRendererAPI : public RendererAPI
{
public:
virtual void SetClearColor(const glm::vec4& color) override;
virtual void Clear() override;
virtual void DrawIndexed(const std::shared_ptr<VertexArray>& vertexArray) override;
};
}
#include "aopch.h"
#include "OpenGLRendererAPI.h"
#include <glad/glad.h>
namespace Aurora {
void OpenGLRendererAPI::SetClearColor(const glm::vec4& color)
{
glClearColor(color.r, color.g, color.b, color.a);
}
void OpenGLRendererAPI::Clear()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
}
void OpenGLRendererAPI::DrawIndexed(const std::shared_ptr<VertexArray>& vertexArray)
{
glDrawElements(GL_TRIANGLES, vertexArray->GetIndexBuffer()->GetCount(), GL_UNSIGNED_INT, nullptr);
}
}
#pragma once
#include "RendererAPI.h"
namespace Aurora {
class RenderCommand
{
public:
inline static void SetClearColor(const glm::vec4& color)
{
s_RendererAPI->SetClearColor(color);
}
inline static void Clear()
{
s_RendererAPI->Clear();
}
inline static void DrawIndexed(const std::shared_ptr<VertexArray>& vertexArray)
{
s_RendererAPI->DrawIndexed(vertexArray);
}
private:
static RendererAPI* s_RendererAPI;
};
}
#include "aopch.h"
#include "RenderCommand.h"
#include "Platform/OpenGL/OpenGLRendererAPI.h"
namespace Aurora {
RendererAPI* RenderCommand::s_RendererAPI = new OpenGLRendererAPI;
}
 使用默认的析构函数
|