创建一个Capsule,在Capsule下面创建一个Camera:
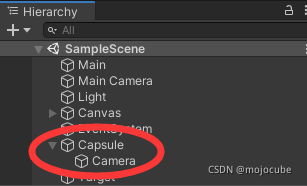
?在Capsule下增加Character Controller,新增FPLook.cs(鼠标控制的第一人称视角)和FPMove.cs(键盘WSAD控制的前后左右移动):
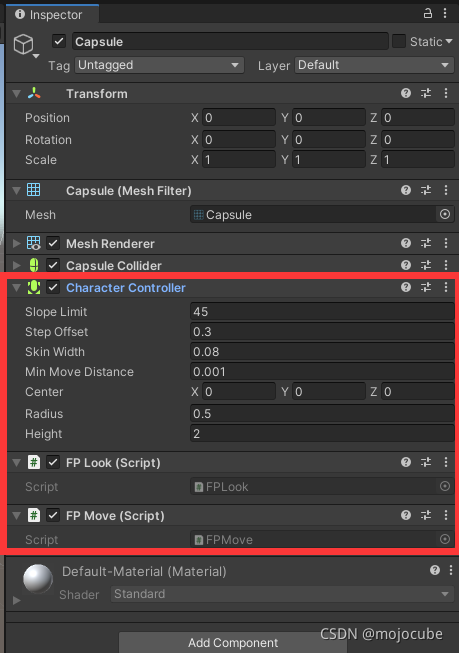
?FPLook.cs:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FPLook : MonoBehaviour
{
//视野转动速度
float speedX = 10f;
float speedY = 10f; //上下观察范围
float minY = -60;
float maxY = 60;
//观察变化量
float rotationX;
float rotationY;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update ()
{
rotationX += Input.GetAxis ("Mouse X")*speedX;
rotationY += Input.GetAxis ("Mouse Y")*speedY;
if (rotationX < 0) {
rotationX += 360;
}
if (rotationX >360) {
rotationX -= 360;
}
rotationY = Mathf.Clamp (rotationY, minY, maxY);
transform.localEulerAngles = new Vector3 (-rotationY, rotationX, 0);
}
}
?FPMove.cs:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FPMove : MonoBehaviour
{
float speed = 5f; //移动速度
private CharacterController characterController;
// Start is called before the first frame update
void Start()
{
characterController = this.GetComponent<CharacterController>();
}
// Update is called once per frame
void Update()
{
//键盘控制前后
float x = Input.GetAxis("Horizontal") * Time.deltaTime * speed;//左右移动
float z = Input.GetAxis("Vertical") * Time.deltaTime * speed;//前后移动
Vector3 movement = new Vector3 (x,0,z);
movement = transform.TransformDirection(movement);//旋转之后的转向
Vector3 newMovement = new Vector3 (movement.x,0,movement.z);
characterController.Move(newMovement);//移动
}
}
起飞降落模式:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FPMove : MonoBehaviour
{
float speed = 5f; //移动速度
private CharacterController characterController;
// Start is called before the first frame update
void Start()
{
characterController = this.GetComponent<CharacterController>();
}
// Update is called once per frame
void Update()
{
//键盘控制前后
float x = Input.GetAxis("Horizontal") * Time.deltaTime * speed;//左右移动
float z = Input.GetAxis("Vertical") * Time.deltaTime * speed;//前后移动
float y = 0;
Vector3 movement = new Vector3 (x,y,z);
movement = transform.TransformDirection(movement);//旋转之后的转向
if(transform.localPosition.y >= 0)
{
y = movement.y;
}
else
{
y = 0.002f;//根据实际情况调整
}
Vector3 newMovement = new Vector3 (movement.x,y,movement.z);
characterController.Move(newMovement);//移动
}
}
|