一:方法一
适用场景:三维场景中,需要自由、全方位观察模型 描述:通过WASD控制相机的移动,鼠标控制相机的旋转,相机正视的方向即为正前方,按W前进,S后退,A左边平移,D右边平移。滚轮控制视野的放大缩小。 相机需要加一个碰撞器,加碰撞器是为了限制相机在一个范围内移动,然后还需要把相机的可移动范围也用碰撞器包围起来,需要注意的是,不要用一个整的大的碰撞器,这样的话可能会把相机给弹飞,最好还是每一个面都加一个碰撞体,还有就是相机最好加球形碰撞体。 相机使用的事透视模式。 然后将下面脚本挂载在相机上:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MouseLook : MonoBehaviour
{
public Transform _Camera;
public float XSensitivity = 1.5f;
public float YSensitivity = 1.5f;
public bool clampVerticalRotation = false;
public float MinimumX = -45F;
public float MaximumX = 45F;
public bool smooth = true;
public float smoothTime = 8f;
private Quaternion m_CharacterTargetRot;
private Quaternion m_CameraTargetRot;
private bool isMove = true;
private void Start()
{
Init();
}
private void Update()
{
LookRotation(_Camera);
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
Vector3 dir = transform.forward * v + transform.right * h;
transform.GetComponent<Rigidbody>().velocity = dir * 20;
if (Input.GetAxis("Mouse ScrollWheel") != 0)
{
transform.GetComponent<Camera>().fieldOfView = Mathf.Clamp(transform.GetComponent<Camera>().fieldOfView, 10, 75);
transform.GetComponent<Camera>().fieldOfView =
transform.GetComponent<Camera>().fieldOfView - Input.GetAxis
("Mouse ScrollWheel") * 20;
}
}
public void Init()
{
m_CameraTargetRot = _Camera.localRotation;
}
public void LookRotation(Transform _camera)
{
float yRot = Input.GetAxis("Mouse X") * XSensitivity;
float xRot = Input.GetAxis("Mouse Y") * YSensitivity;
m_CameraTargetRot = _camera.localRotation * Quaternion.Euler(-xRot, yRot, 0f);
if (clampVerticalRotation)
m_CameraTargetRot = ClampRotationAroundXAxis(m_CameraTargetRot);
if (smooth)
{
_camera.localRotation = Quaternion.Slerp(_camera.localRotation, m_CameraTargetRot,
smoothTime * Time.deltaTime);
}
else
{
_camera.localRotation = m_CameraTargetRot;
}
_camera.localRotation = Quaternion.Euler(new Vector3(_camera.eulerAngles.x, _camera.eulerAngles.y, 0f));
}
Quaternion ClampRotationAroundXAxis(Quaternion q)
{
q.x /= q.w;
q.y /= q.w;
q.z /= q.w;
q.w = 1.0f;
float angleX = 2.0f * Mathf.Rad2Deg * Mathf.Atan(q.x);
angleX = Mathf.Clamp(angleX, MinimumX, MaximumX);
q.x = Mathf.Tan(0.5f * Mathf.Deg2Rad * angleX);
return q;
}
}
具体组件、参数设置如下图所示: 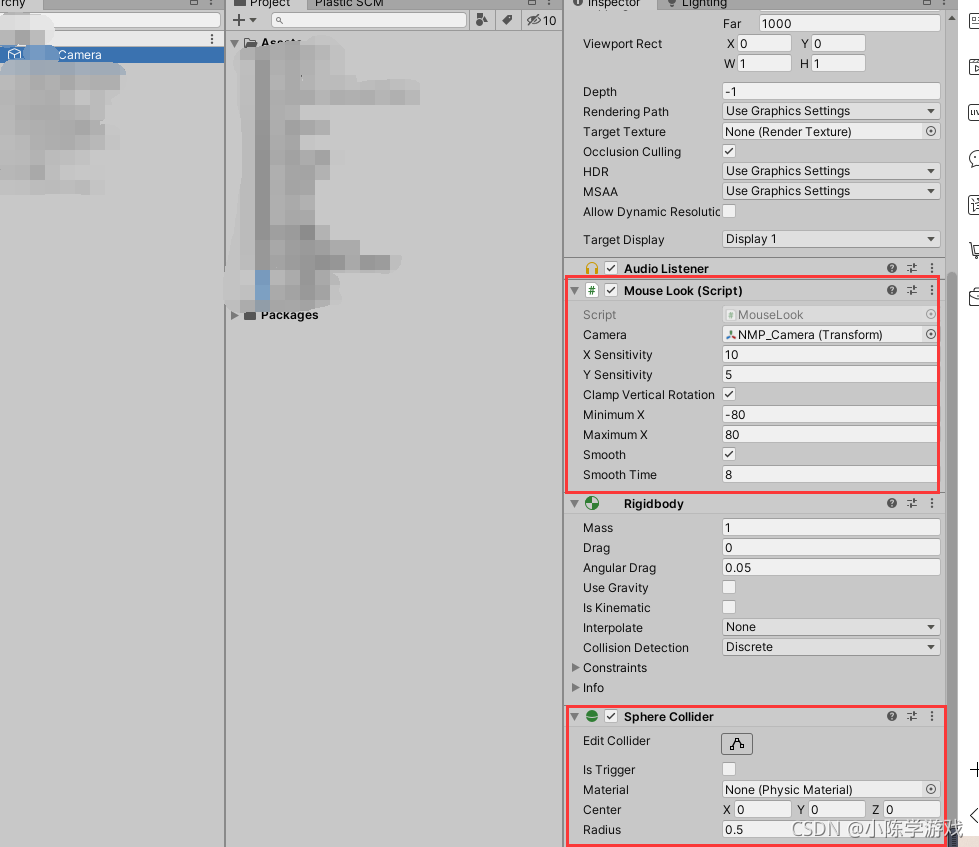
二:方法二
适用场景:二维场景中,需要自由、全方位观察模型 描述:通过WASD控制相机的移动,相机正视的方向即为正前方,按W向上移动,S向下移动,A左边平移,D右边平移。滚轮控制视野的放大缩小。 通过相机的位置坐标来控制相机的移动范围 相机使用的是正交模式。 然后将下面脚本挂载在相机上:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraController : MonoBehaviour
{
private Vector3 dirVector3;
private Vector3 rotaVector3;
[SerializeField]
private float paramater = 1f;
private float dis = 1;
public int MinOrthographicSize=10;
public int MaxOrthographicSize = 120;
public Vector2 MoveX=new Vector2(-215f, 85f);
public Vector2 MoveY = new Vector2(-75f, 92f);
void Awake()
{
}
private void Start()
{
}
private void Update()
{
dirVector3 = Vector3.zero;
if (Input.GetKey(KeyCode.W)||Input.GetKey(KeyCode.UpArrow))
{
dirVector3.y = 1;
}
if (Input.GetKey(KeyCode.S) || Input.GetKey(KeyCode.DownArrow))
{
dirVector3.y = -1;
}
if (Input.GetKey(KeyCode.A) || Input.GetKey(KeyCode.LeftArrow))
{
dirVector3.x = -1;
}
if (Input.GetKey(KeyCode.D) || Input.GetKey(KeyCode.RightArrow))
{
dirVector3.x = 1;
}
if (Input.GetAxis("Mouse ScrollWheel") != 0)
{
transform.GetComponent<Camera>().orthographicSize = Mathf.Clamp(transform.GetComponent<Camera>().orthographicSize, MinOrthographicSize, MaxOrthographicSize);
transform.GetComponent<Camera>().orthographicSize =
transform.GetComponent<Camera>().orthographicSize - Input.GetAxis
("Mouse ScrollWheel") * 20;
}
if (80< transform.GetComponent<Camera>().orthographicSize&&transform.GetComponent<Camera>().orthographicSize<100)
{
paramater = 0.8f;
}
if (50 < transform.GetComponent<Camera>().orthographicSize && transform.GetComponent<Camera>().orthographicSize < 80)
{
paramater = 0.5f;
}
if (30 < transform.GetComponent<Camera>().orthographicSize && transform.GetComponent<Camera>().orthographicSize < 50)
{
paramater = 0.25f;
}
if (10 < transform.GetComponent<Camera>().orthographicSize && transform.GetComponent<Camera>().orthographicSize < 30)
{
paramater = 0.15f;
}
if ( transform.GetComponent<Camera>().orthographicSize < 10)
{
paramater = 0.05f;
}
transform.Translate(dirVector3 * paramater, Space.Self);
float TempX = Mathf.Clamp(transform.position.x, MoveX.x, MoveX.y);
float TempY = Mathf.Clamp(transform.position.y, MoveY.x,MoveY.y);
transform.position = new Vector3(TempX, TempY, 30);
}
void FixedUpdate()
{
}
}
具体组件、参数设置如下图所示: 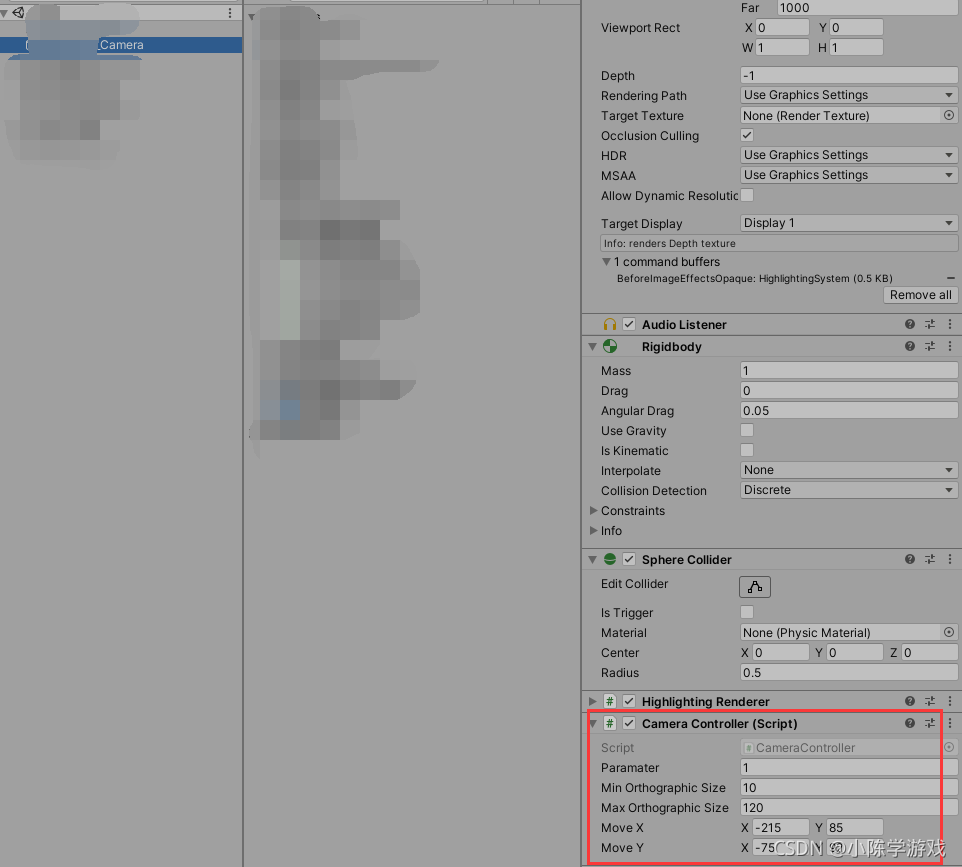
|