Excel 转 unity 配置脚本
.net core 3.1
介绍
支持excel 公式,且只支持 .xlsx 格式文档
仓库地址
支持的数据类型
数据类型 | 表格 |
---|
String | string | Double | double | Float | float | Int | int | Vector2 | v2 | Vector3 | v3 | Bool | bool |
excel 配置
类型为空 则自动引用前一个类型 相同变量名称为同一数组
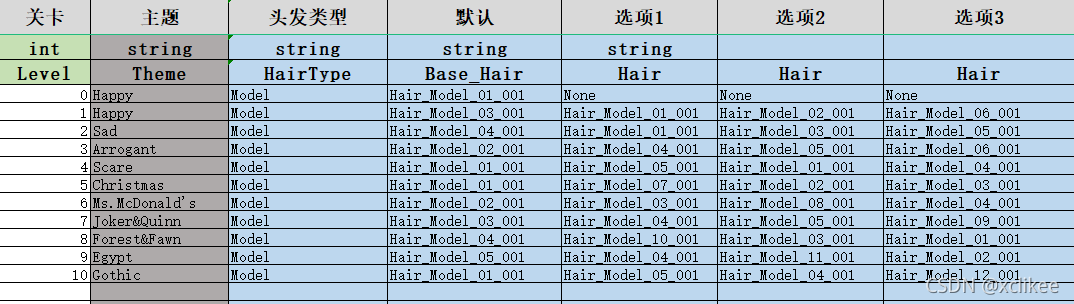
public class LevelFaceCfg : CSVBaseConfig<LevelFaceCfg,LevelFaceCfg.LevelFaceCfgData>
{
public class LevelFaceCfgData
{
public int Level;
public string Theme;
public string HairType;
public string Base_Hair;
public string[] Hair;
}
protected override void Init()
{
Data.Add(new LevelFaceCfgData()
{
Level = 0,
Theme = "Happy",
HairType = "Model",
Base_Hair = "Hair_Model_01_001",
Hair = new string[3]{
"None",
"None",
"None"
}
});
Data.Add(new LevelFaceCfgData()
{
Level = 1,
Theme = "Happy",
HairType = "Model",
Base_Hair = "Hair_Model_03_001",
Hair = new string[3]{
"Hair_Model_01_001",
"Hair_Model_02_001",
"Hair_Model_06_001"
}
});
}
}
生成的配置文件
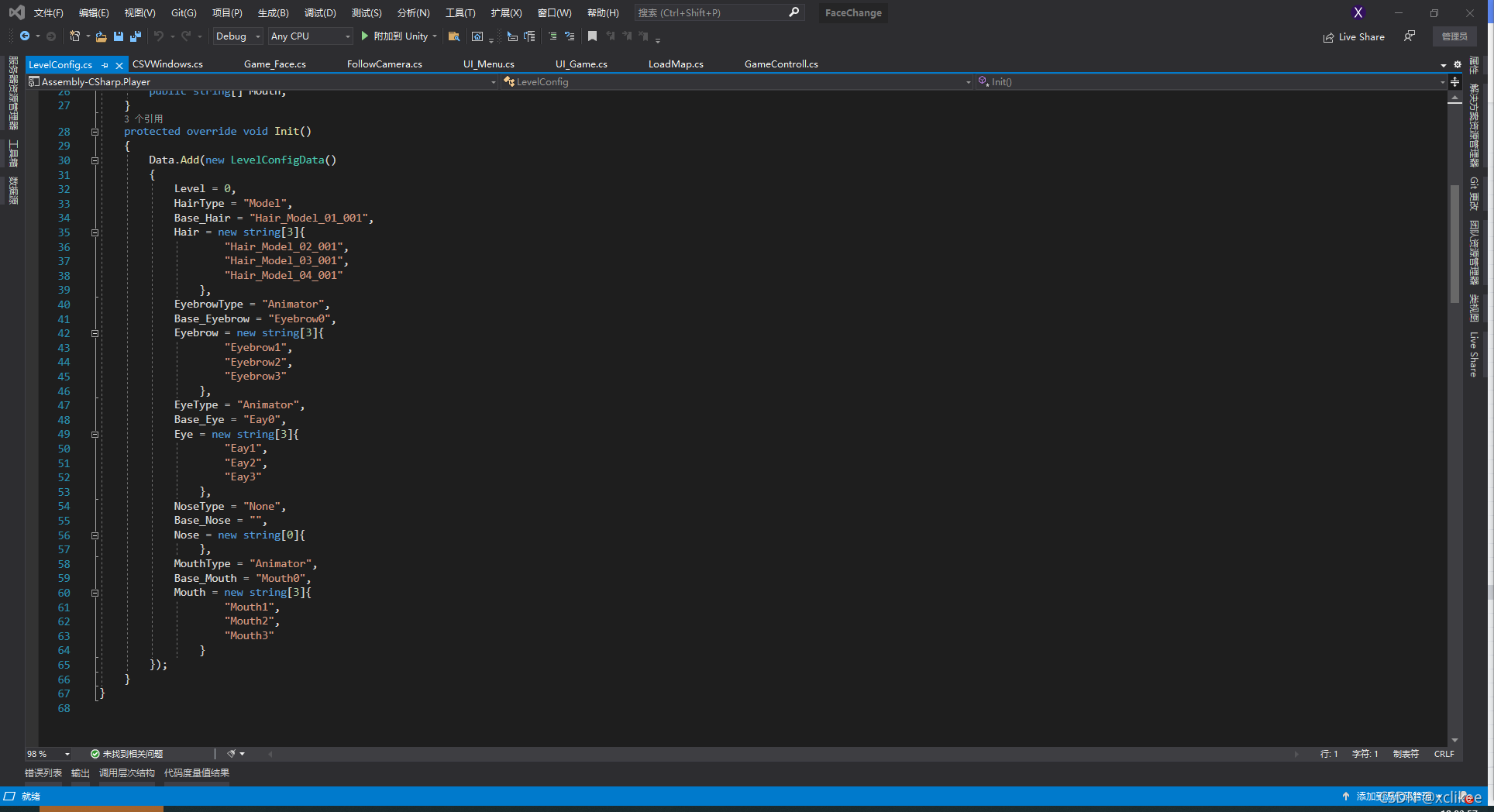 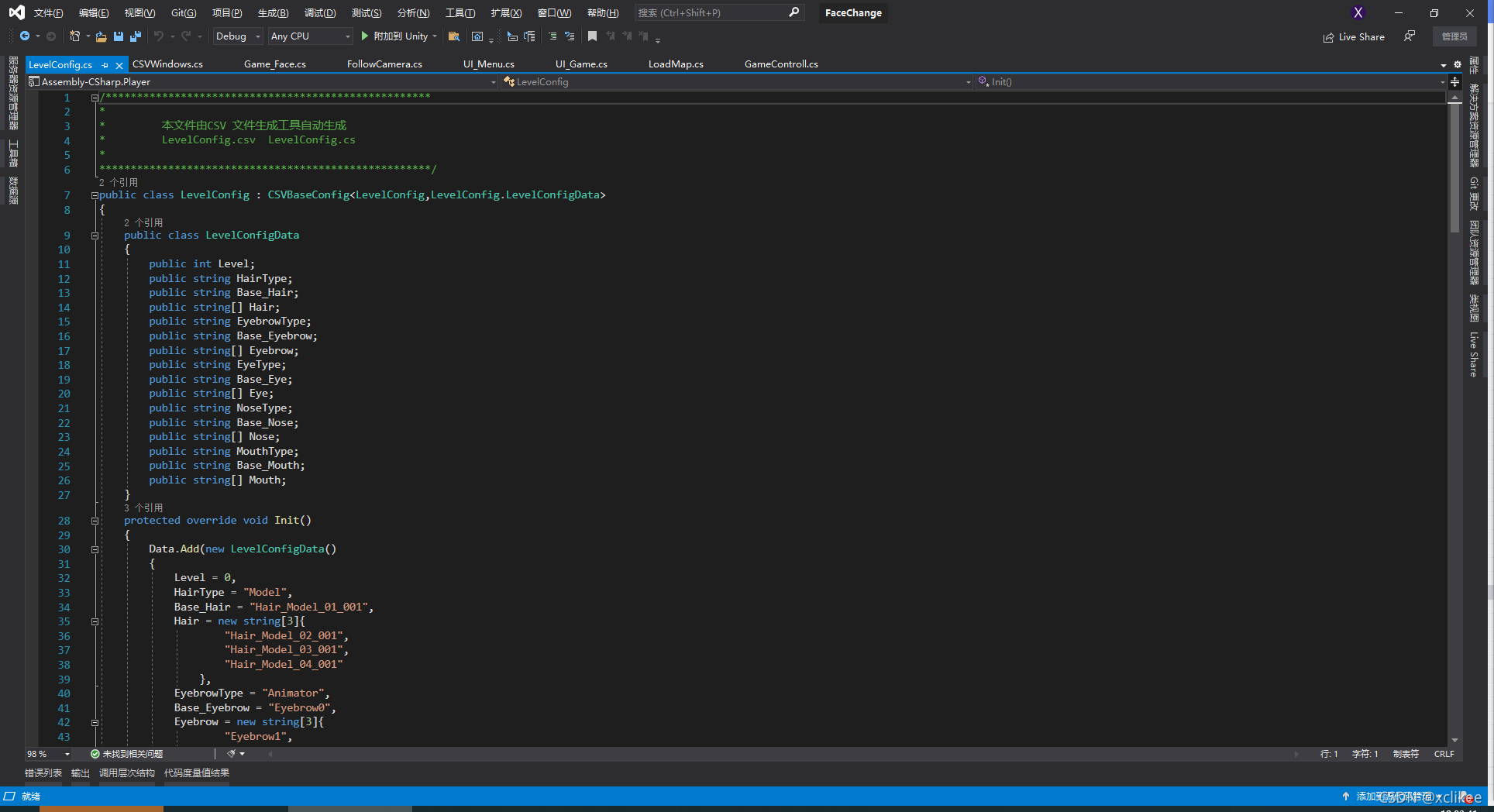
命令行参数
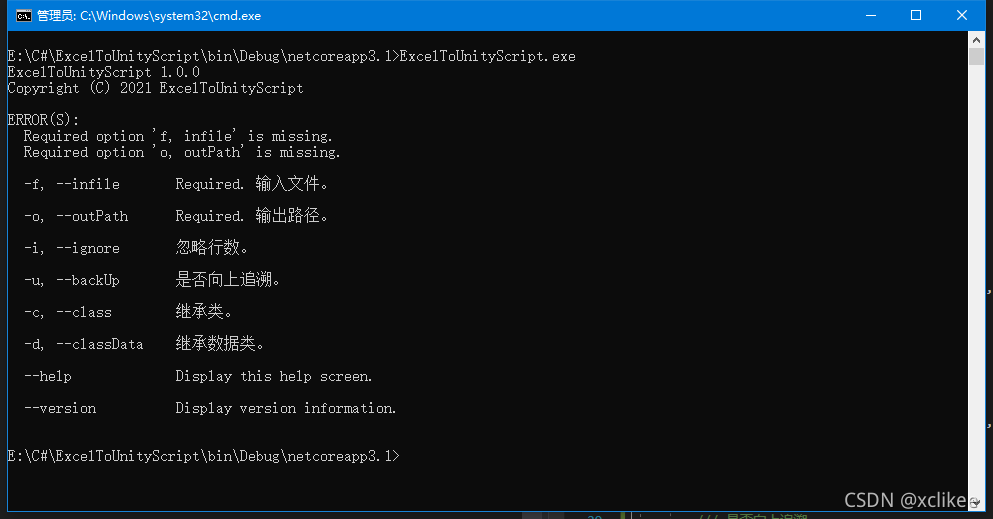
CSVBaseConfig.cs
using System;
using System.Collections.Generic;
using UnityEngine;
public class CSVBaseConfig<T, C> : Single<T> where T : CSVBaseConfig<T, C>, new()
where C : new()
{
protected List<C> Data = new List<C>();
public List<C> GetData { get => Data; }
protected override void Init() { }
public C Find(Predicate<C> func)
{
return Data.Find(func);
}
protected bool ToBool(string[] str, int index)
{
if (str.Length > index)
{
if (bool.TryParse(str[index], out bool res))
return res;
}
return false;
}
protected string ToString(string[] str, int index)
{
if (str.Length > index)
return str[index];
return string.Empty;
}
protected Color ToColor(string[] str, int index)
{
if (str.Length > index)
{
if (ColorUtility.TryParseHtmlString("#" + str[index], out Color color))
return color;
}
return Color.white;
}
protected int ToInt(string[] str, int index)
{
if (str.Length > index)
if (int.TryParse(str[index], out int f))
return f;
return 0;
}
protected float ToFloat(string[] str, int index)
{
if (str.Length > index)
if (float.TryParse(str[index], out float f))
return f;
return 0;
}
}
Single.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Single<T> where T : Single<T>, new()
{
private static T _instance;
public static T Ins
{
get
{
if (null == _instance)
{
_instance = new T();
_instance.Init();
}
return _instance;
}
}
protected virtual void Init()
{
}
}
|