解释全在注释里。复制代码挂上运行查看效果,可根据自己的需求修改。
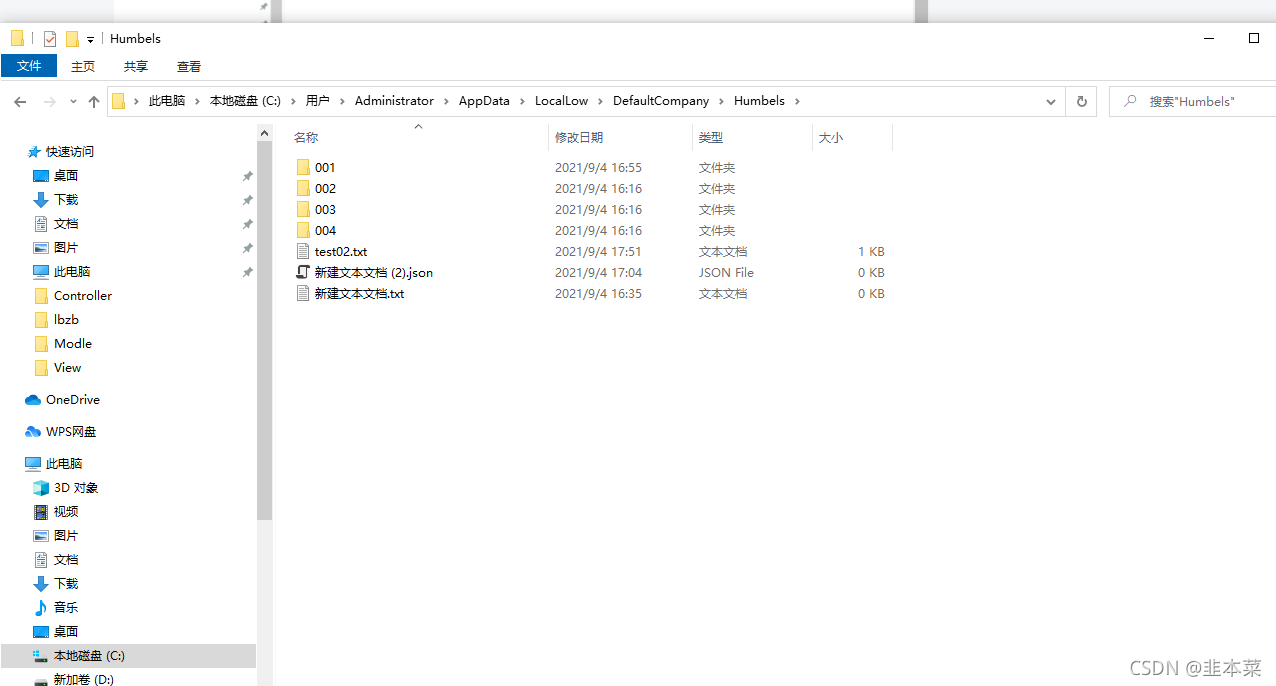
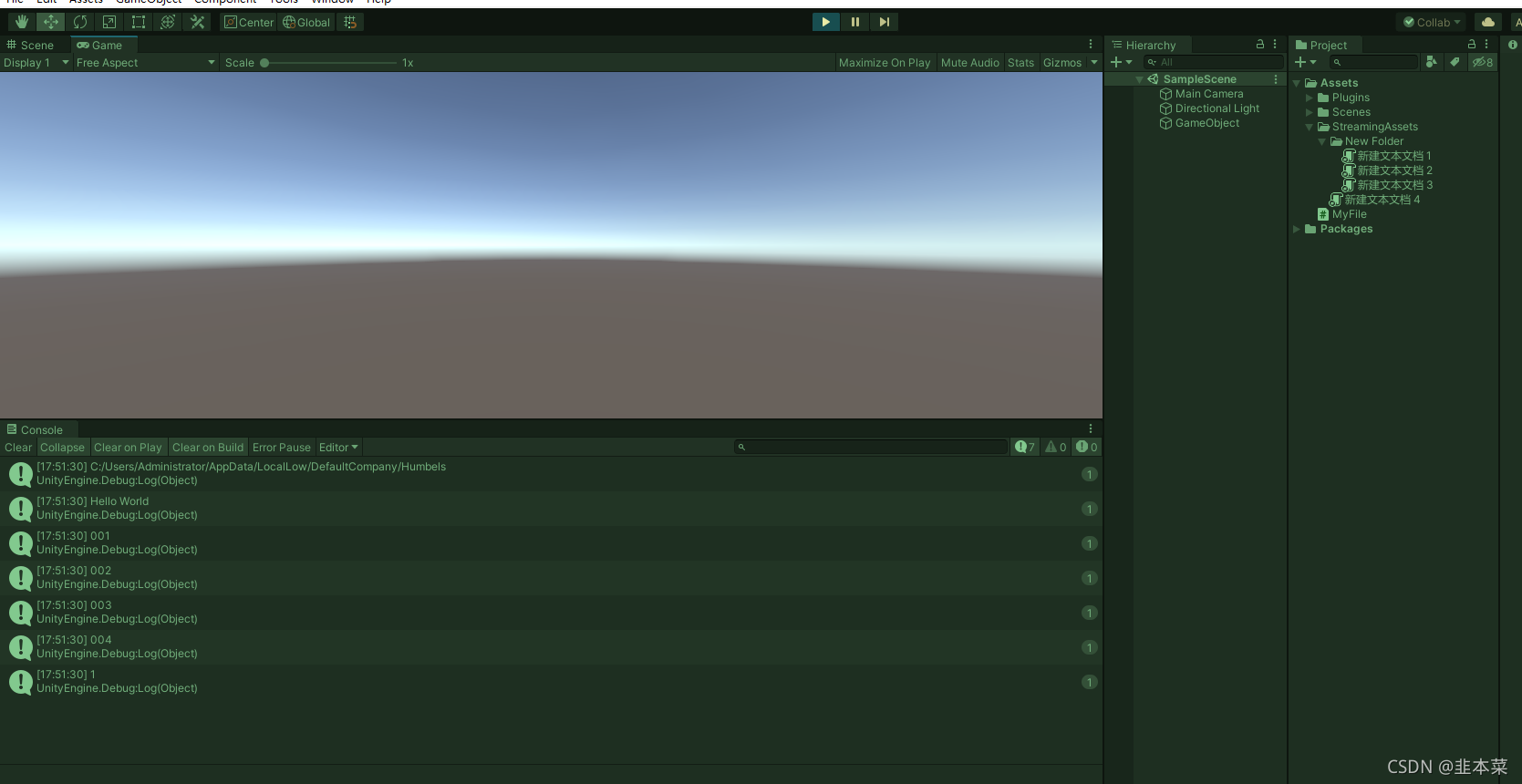
using System;
using System.Collections;
using System.Collections.Generic;
using System.IO;
using System.Text;
using UnityEngine;
public class MyFile : MonoBehaviour
{
void Start()
{
Debug.Log(Application.persistentDataPath);
//建个文件夹留着删除测试
if (!Directory.Exists(Application.persistentDataPath+"/test"))
{
Directory.CreateDirectory(Application.persistentDataPath + "/test");
}
//创建两个txt文件,并且写入Hello World
FileWrite(Application.persistentDataPath, "/test01.txt", "Hello World");
FileWrite(Application.persistentDataPath, "/test02.txt", "Hello World");
//读出txt中的数据
string str= FileRead(Application.persistentDataPath+"/test01.txt");
Debug.Log(str);
//删除文件测试
FileDelete(Application.persistentDataPath, "test01.txt");
//删除文件夹测试
FileDelete(Application.persistentDataPath+ "/test");
//获取所有的文件夹
string [] strs= GetFilePath(Application.persistentDataPath);
for (int i = 0; i < strs.Length; i++)
{
Debug.Log(strs[i]);
}
//获取指定类型的文件
Debug.Log(getFile(Application.streamingAssetsPath, ".json").Count);
}
/// <summary>
/// 获取路径下的所有文件夹
/// </summary>
/// <param name="path"></param>
/// <returns></returns>
public static string[] GetFilePath(string path)
{
if (Directory.Exists(path))
{
//文件路径
string[] dir = Directory.GetDirectories(path);
//文件名
string[] names = new string[dir.Length];
for (int i = 0; i < dir.Length; i++)
{
//赋值文件命名
names[i] = Path.GetFileName(dir[i]);
// print(names[i]);
}
// print(dir.Length);
return names;
}
else
{
Debug.LogError("未找到路径");
return null;
}
}
/// <summary>
/// 私有变量
/// </summary>
private static List<FileInfo> lst = new List<FileInfo>();
/// <summary>
/// 获得目录下所有文件或指定文件类型文件
/// </summary>
/// <param name="path">文件夹路径</param>
/// <param name="extName">扩展名可以多个 例如 .mp3.wma.rm</param>
/// <returns>List<FileInfo></returns>
public static List<FileInfo> getFile(string path, string extName)
{
Getdir(path, extName);
return lst;
}
/// <summary>
/// 获取指定类型文件 注意:Application.persistentDataPath可能获取不到
/// </summary>
/// <param name="path"></param>
/// <param name="extName"></param>
private static void Getdir(string path, string extName)
{
try
{
lst = new List<FileInfo>();
string[] dir = Directory.GetDirectories(path); //文件夹列表
DirectoryInfo fdir = new DirectoryInfo(path);
FileInfo[] file = fdir.GetFiles();
//FileInfo[] file = Directory.GetFiles(path); //文件列表
if (file.Length != 0 || dir.Length != 0) //当前目录文件或文件夹不为空
{
foreach (FileInfo f in file) //显示当前目录所有文件
{
if (extName.ToLower().IndexOf(f.Extension.ToLower()) >= 0)
{
lst.Add(f);
}
}
//foreach (string d in dir)
//{
// Getdir(d, extName);//递归
//}
}
}
catch (Exception ex)
{
Debug.LogError("失败");
}
}
/// <summary>
/// 创建文件并且写入数据
/// </summary>
/// <param name="path">路径</param>
/// <param name="name">名字</param>
/// <param name="data">数据</param>
public static bool FileWrite(string path, string name, string data)
{
try
{
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
FileStream fs = new FileStream(path + name, FileMode.Create, FileAccess.Write);
StreamWriter sw = new StreamWriter(fs, Encoding.UTF8);
sw.Write(data);
sw.Close();
fs.Close();
return true;
}
catch (Exception ex)
{
Debug.LogError(ex);
}
return false;
}
/// <summary>
/// 文件读取数据
/// </summary>
/// <param name="path">路径</param>
/// <returns></returns>
public static string FileRead(string path)
{
string data = string.Empty;
FileInfo t = new FileInfo(path);
if (!t.Exists)
{
return string.Empty;
}
try
{
FileStream fs = new FileStream(path, FileMode.Open, FileAccess.Read);
StreamReader sr = new StreamReader(fs, Encoding.UTF8);
data = sr.ReadToEnd();
sr.Close();
fs.Close();
}
catch (IOException e)
{
Debug.LogError("FileRead: " + e.Message);
}
return data;
}
/// <summary>
/// 删除指定路径下的指定文件
/// </summary>
/// <param name="fullPath">路径</param>
/// <param name="fileName">文件名称</param>
/// <returns></returns>
public static bool FileDelete(string fullPath, string fileName)
{
//获取指定路径下面的所有资源文件 然后进行删除
if (Directory.Exists(fullPath))
{
DirectoryInfo direction = new DirectoryInfo(fullPath);
FileInfo[] files = direction.GetFiles("*", SearchOption.AllDirectories);
for (int i = 0; i < files.Length; i++)
{
if (files[i].Name.EndsWith(".meta"))
{
continue;
}
string FilePath = fullPath + "/" + files[i].Name;
// Debug.Log(files[i].Name);
if (files[i].Name == fileName)//如果不指定文件名称将删除这个文件夹下的所有文件
File.Delete(FilePath);
}
return true;
}
return false;
}
/// <summary>
/// 删除指定的文件夹
/// </summary>
/// <param name="path">路径</param>
public static void FileDelete(string path)
{
directoryInfo = new DirectoryInfo(path);
DeleteDirs(directoryInfo);
}
static DirectoryInfo directoryInfo;
static void DeleteDirs(DirectoryInfo dirs)
{
if (dirs == null || (!dirs.Exists))
{
Debug.LogError("没有指定文件");
return;
}
DirectoryInfo[] subDir = dirs.GetDirectories();
if (subDir != null)
{
for (int i = 0; i < subDir.Length; i++)
{
if (subDir[i] != null)
{
DeleteDirs(subDir[i]);
}
}
subDir = null;
}
FileInfo[] files = dirs.GetFiles();
if (files != null)
{
for (int i = 0; i < files.Length; i++)
{
if (files[i] != null)
{
// Debug.Log("删除文件:" + files[i].FullName + "__over");
files[i].Delete();
files[i] = null;
}
}
files = null;
}
// Debug.Log("删除文件夹:" + dirs.FullName + "__over");
dirs.Delete();
}
}
|