学习目标:入手unity并制作第一款独立游戏
一、建模及基本组件
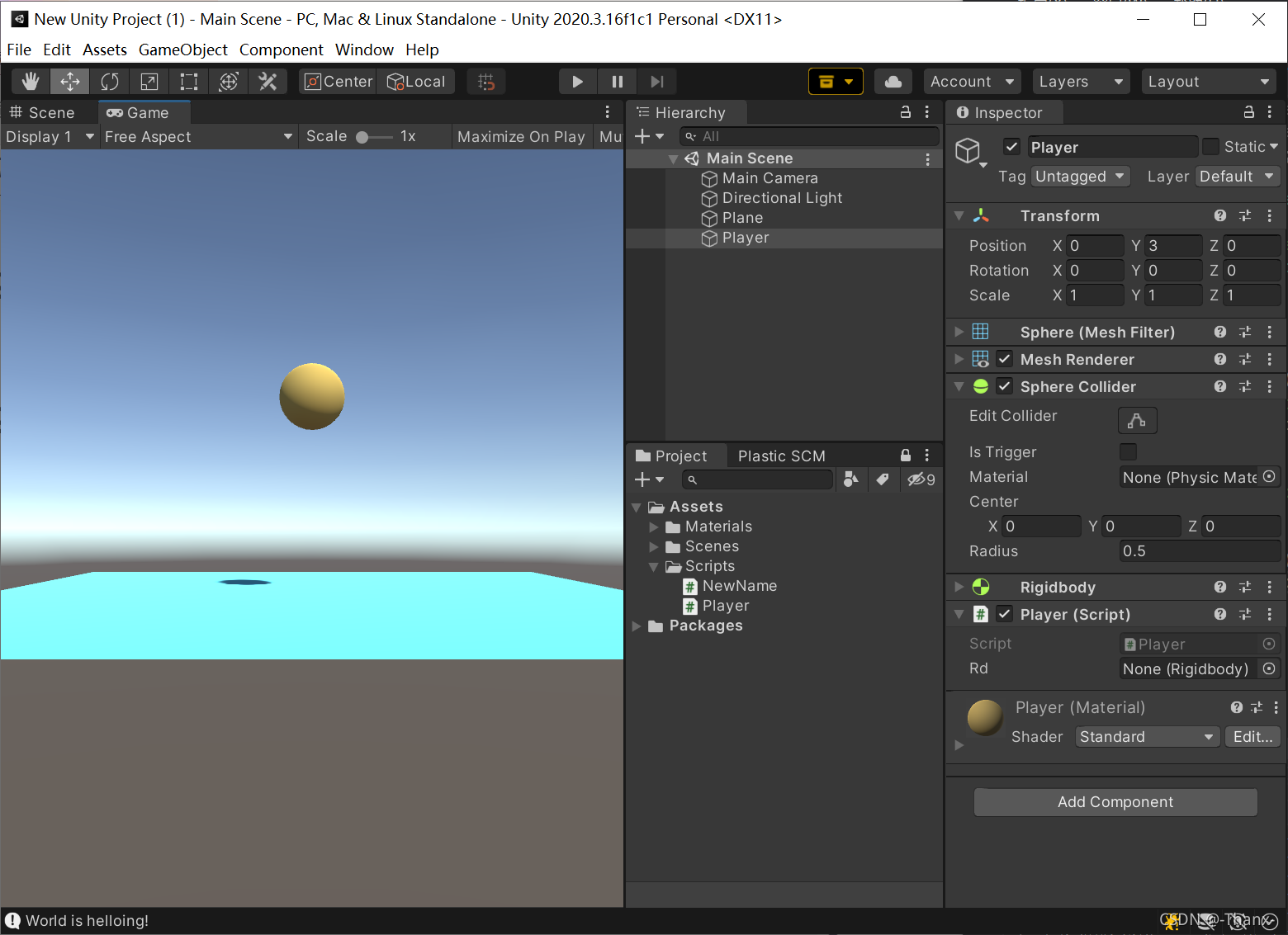
- Materials:存放材质Material的文件夹,将材质拖动到模型上即可附加,其中添加了Rigidbody刚体材质到小球Player上
- Scenes:场景
- Scripts:存放c#代码的文件夹
- Packages:自带的组件包
- Local/Global切换可以切换控制实体坐标轴
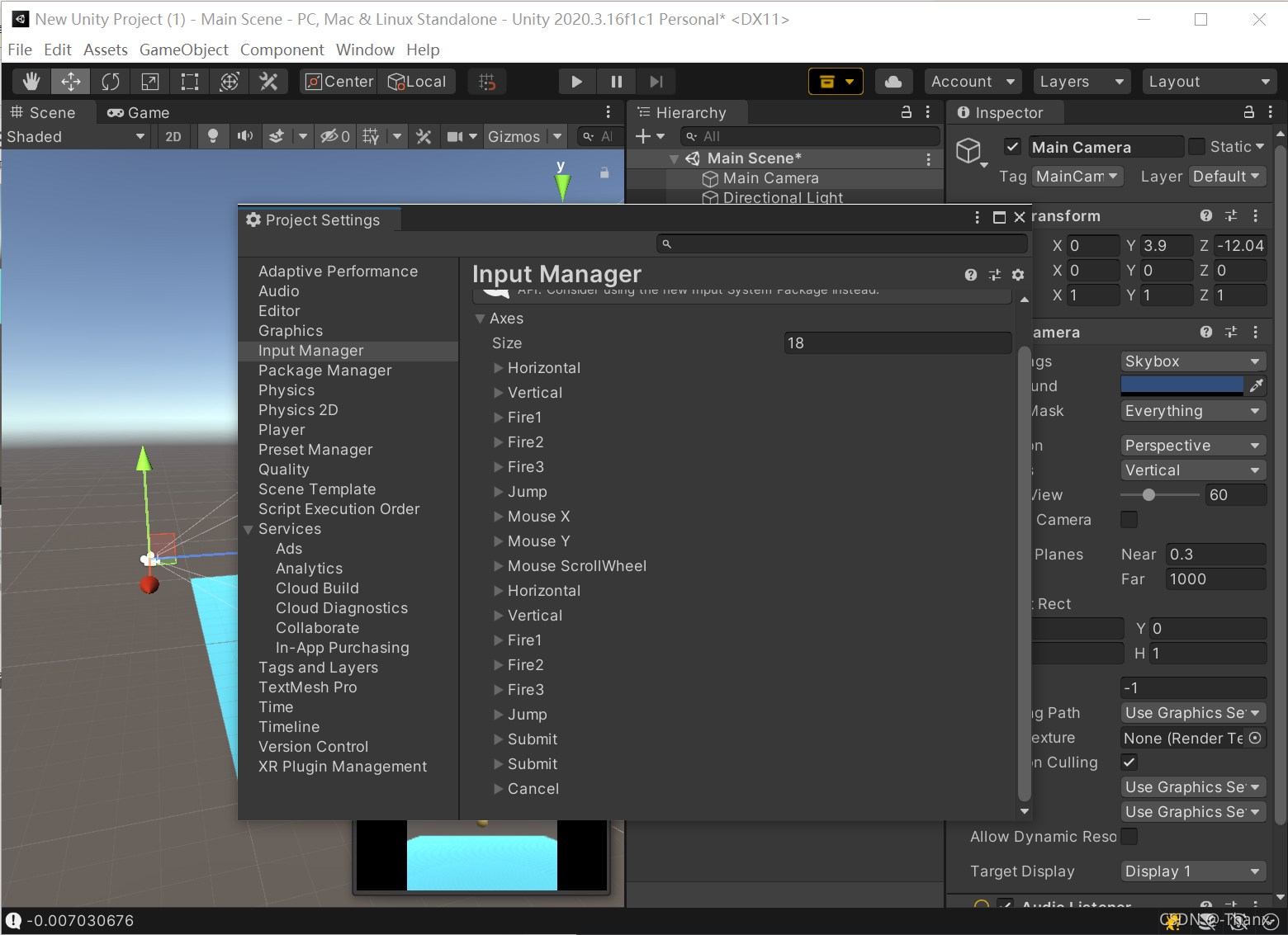 - Project Settings->Input Manager 包含键盘及鼠标输入相应的行为
二、学习代码记录
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
public Rigidbody rd;
void Start()
{
Debug.Log("Hello world!");
rd = GetComponent<Rigidbody>();
}
void Update()
{
Debug.Log("World is helloing!");
rd.AddForce(Vector3.right);
rd.AddForce(Vector3.left);
rd.AddForce(Vector3.forward);
rd.AddForce(Vector3.back);
rd.AddForce(Vector3.up*10);
rd.AddForce(Vector3.down);
rd.AddForce(new Vector3(0, 0, -1));
float h = Input.GetAxis("Horizontal");
float h = Input.GetAxis("Vertical");
Debug.Log(h);
rd.AddForce(new Vector3(h, 0, v));
}
}
三、控制相机跟随移动
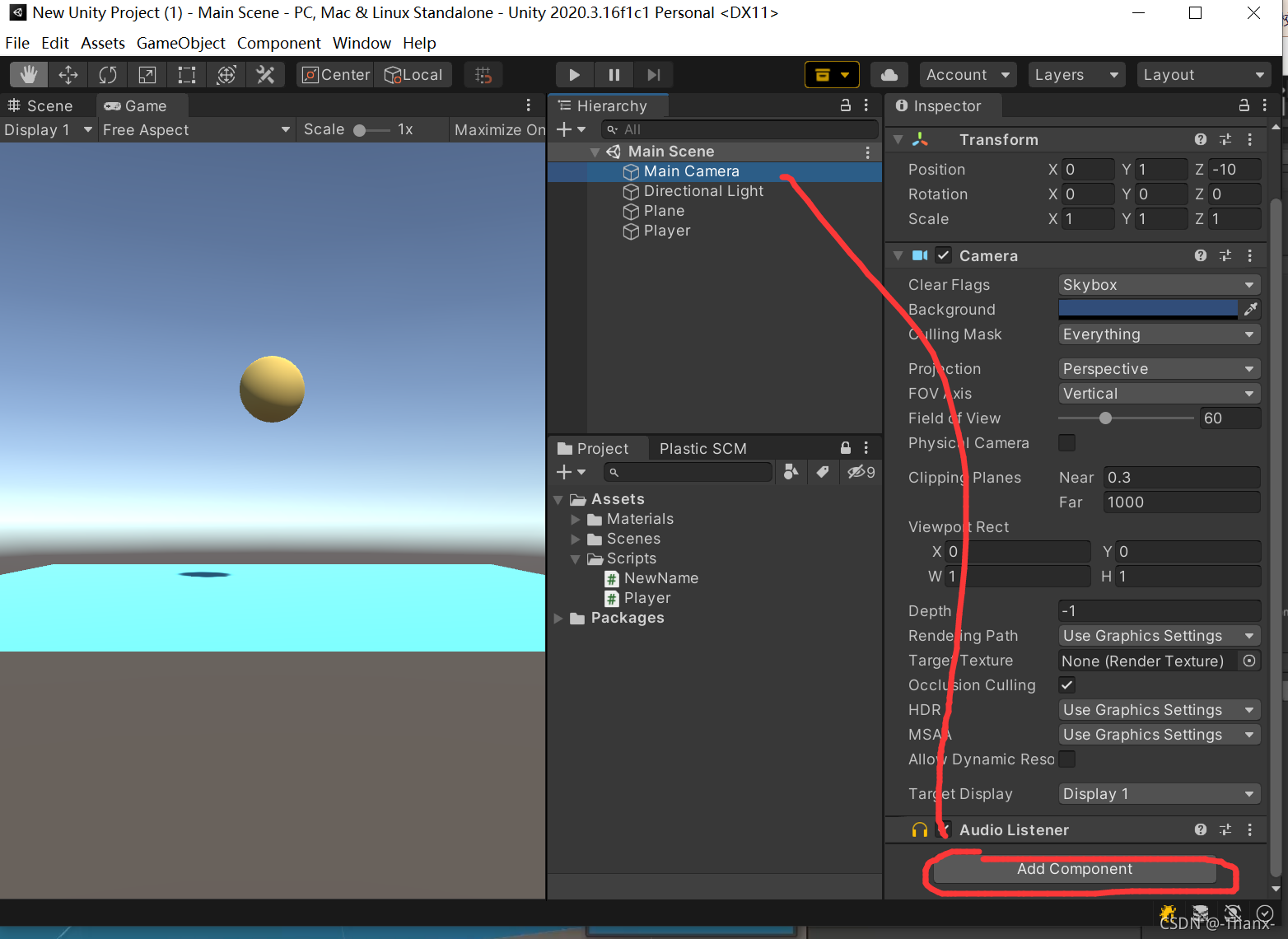
- 给Main Camera添加FollowTarget脚本,用来跟随物体移动
- 在代码中Vector3创建一个距离差对象offset,设置为私有全局变量
- Transform创建一个playertransform对象获取player坐标位置
- transfrom可以直接得到此脚本对应的组件位置
- 代码如下:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FollowTarget : MonoBehaviour
{
public Transform playertransform;
private Vector3 offset;
void Start()
{
offset = transform.position - playertransform.position;
}
void Update()
{
transform.position = playertransform.position + offset;
}
}
四、场景布置
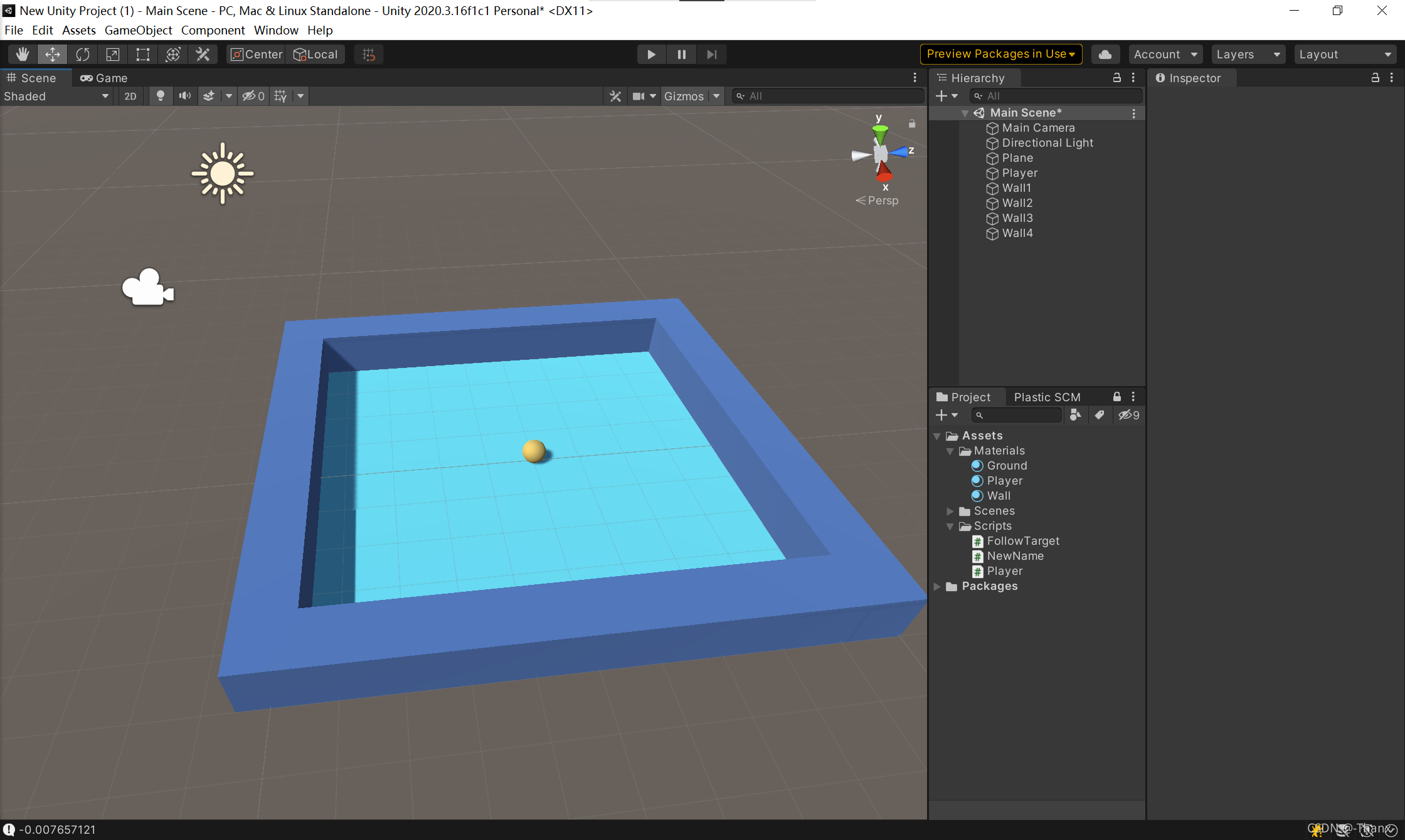
- 创建4个Cube实体作为墙,改变长度和位置使之置于平台四个边上
- 创建食物Food
- 创建预制体文件夹Prefabs,将Food拖入其中,预制体即创建完成
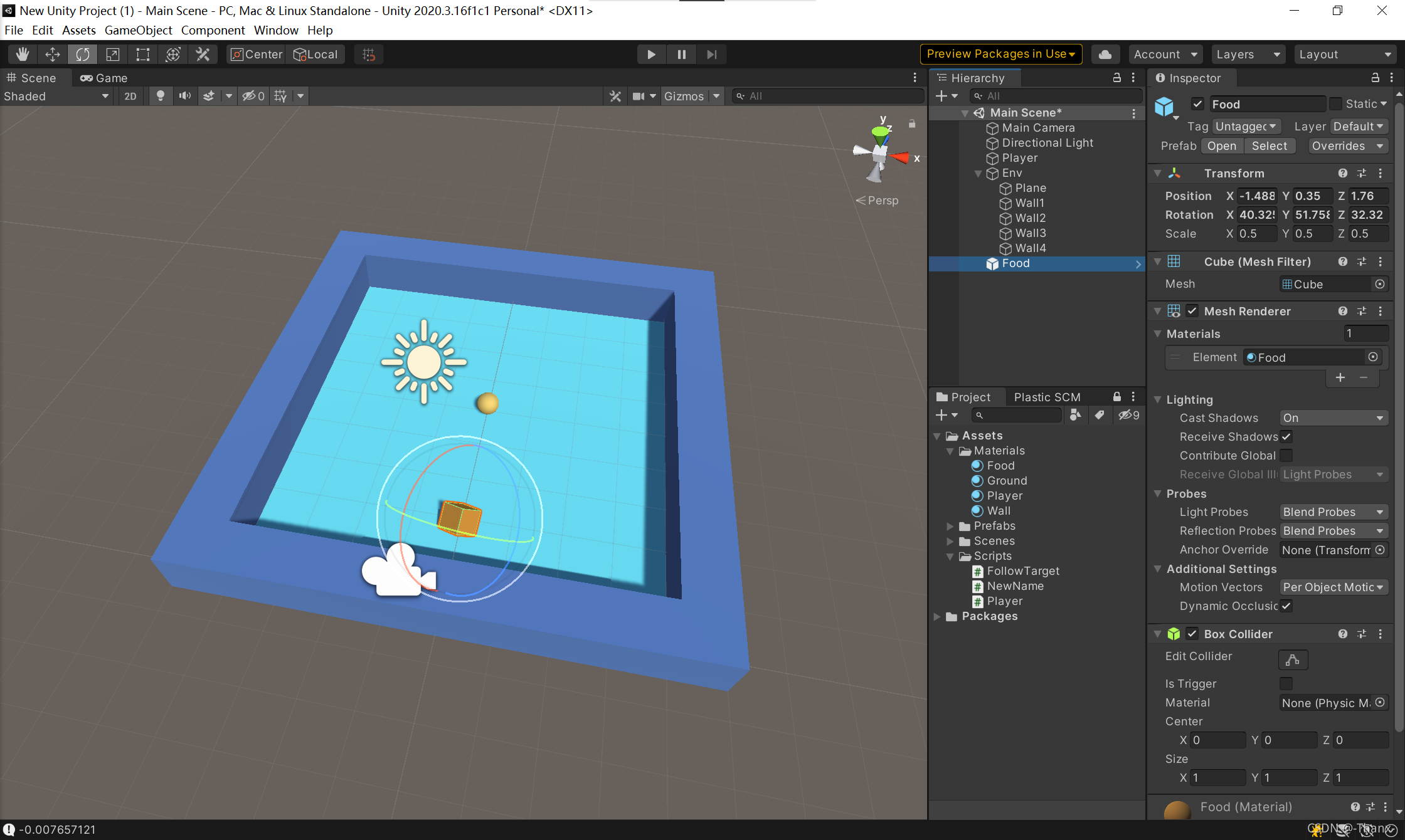
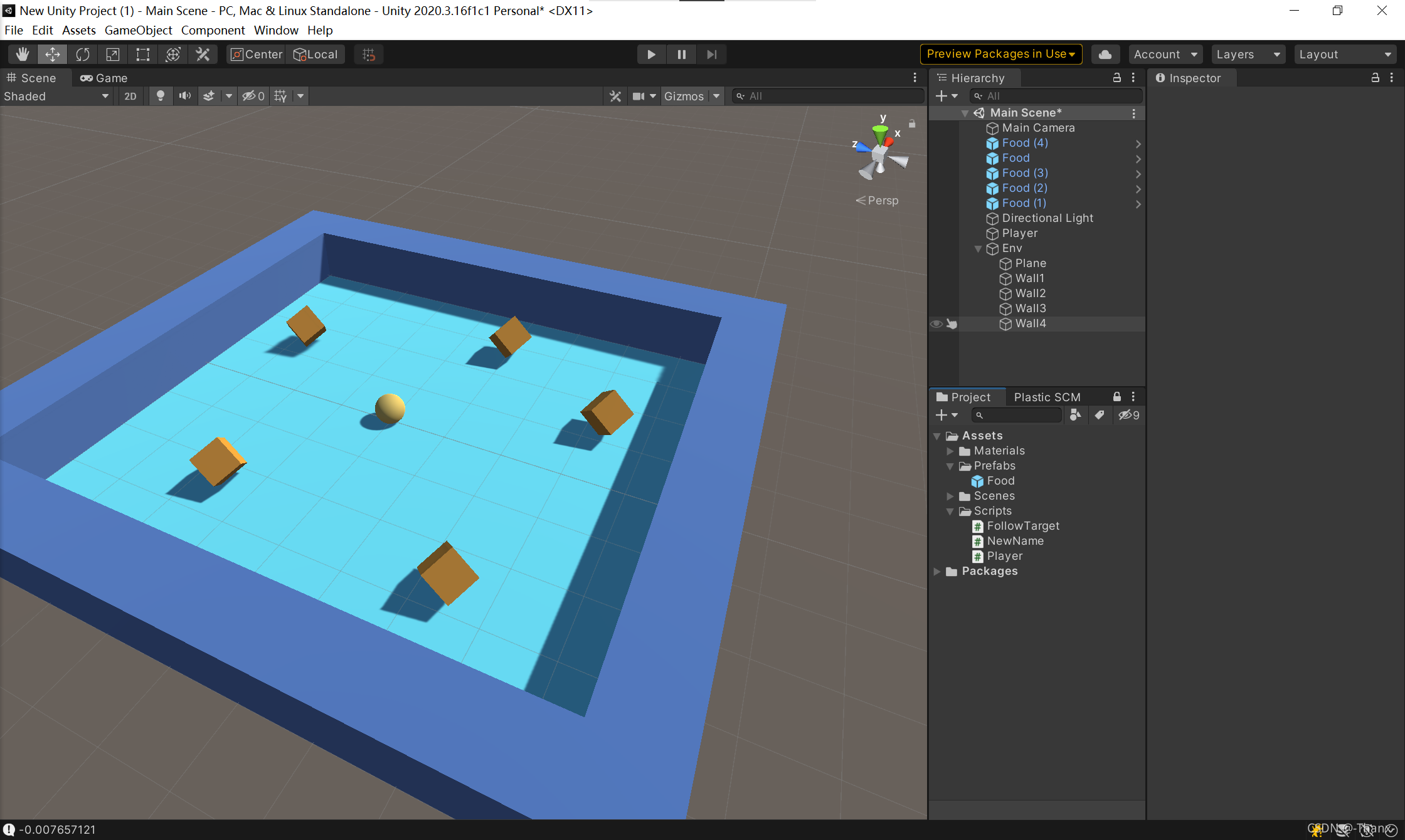
- 控制Food旋转
- 添加脚本Food
transform.Rotate(Vextor.up);//默认一秒执行60次,即一秒旋转60度 - 文档查阅操作如下
也可以登录unity.cn查看中文文档 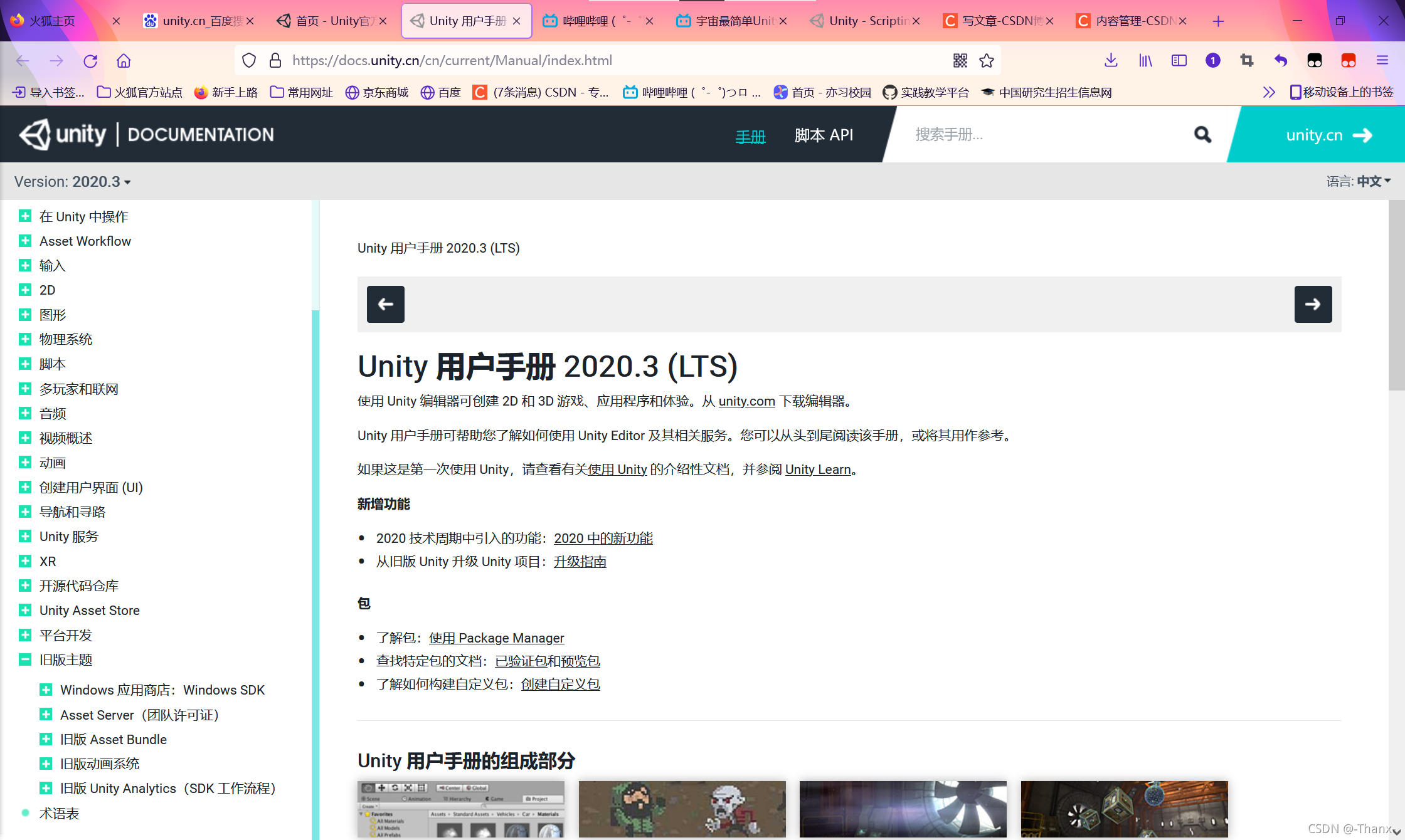 - 旋转脚本代码如下
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Food : MonoBehaviour
{
void Start()
{
}
void Update()
{
transform.Rotate(Vector3.up);
}
}
五、碰撞检测
- player.cs中创建系统事件
private void OnCollisionEnter(Collision collision)
{
Debug.Log("发生碰撞 OnCollisionEnter");
}
private void OnCollisionExit(Collision collision)
{
Debug.Log("发生碰撞 OnCollisionExit");
}
private void OnCollisionStay(Collision collision)
{
Debug.Log("发生碰撞 OnCollisionStay");
}
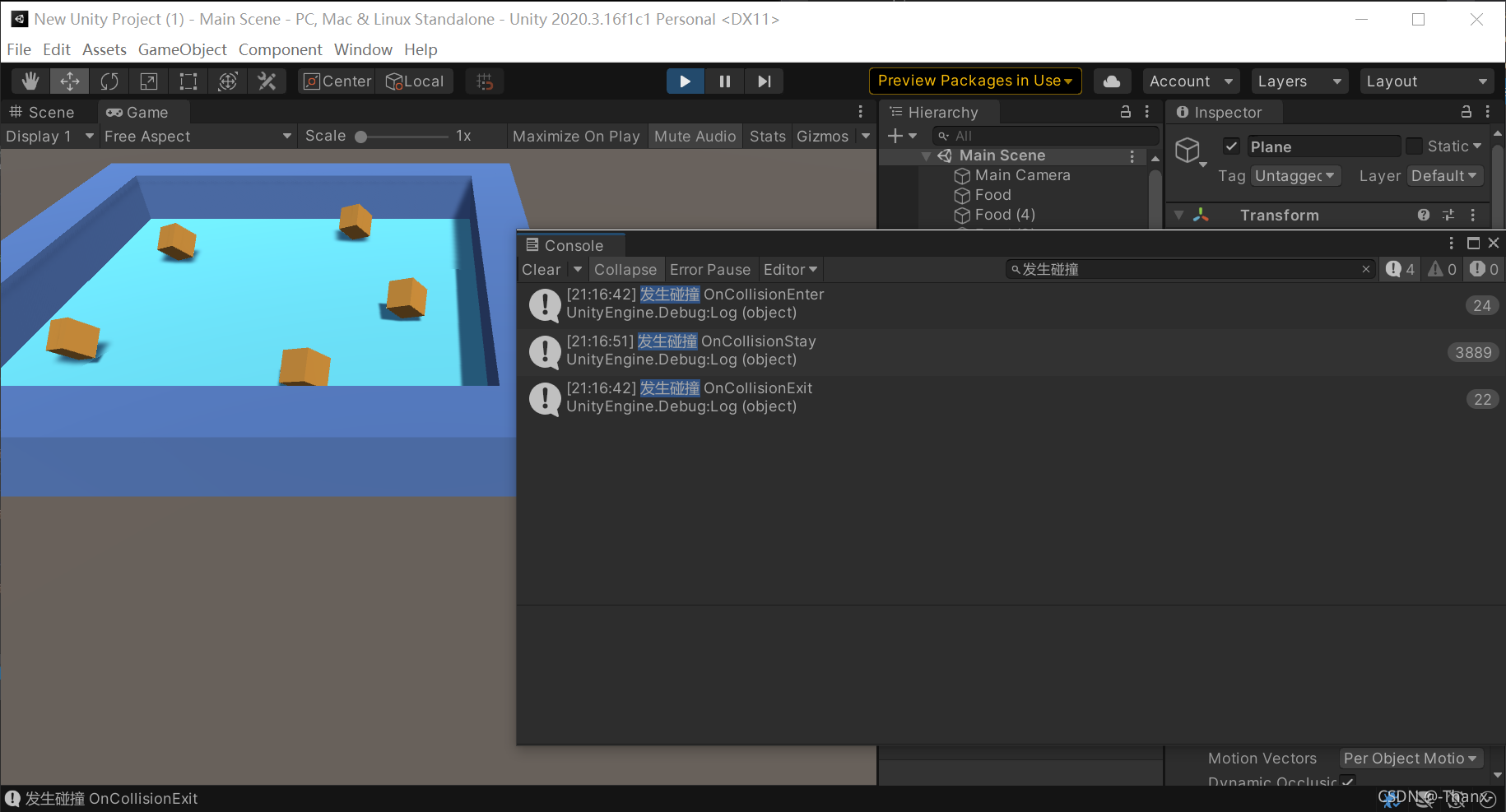
- Food添加 Food 标签,便于区分物体
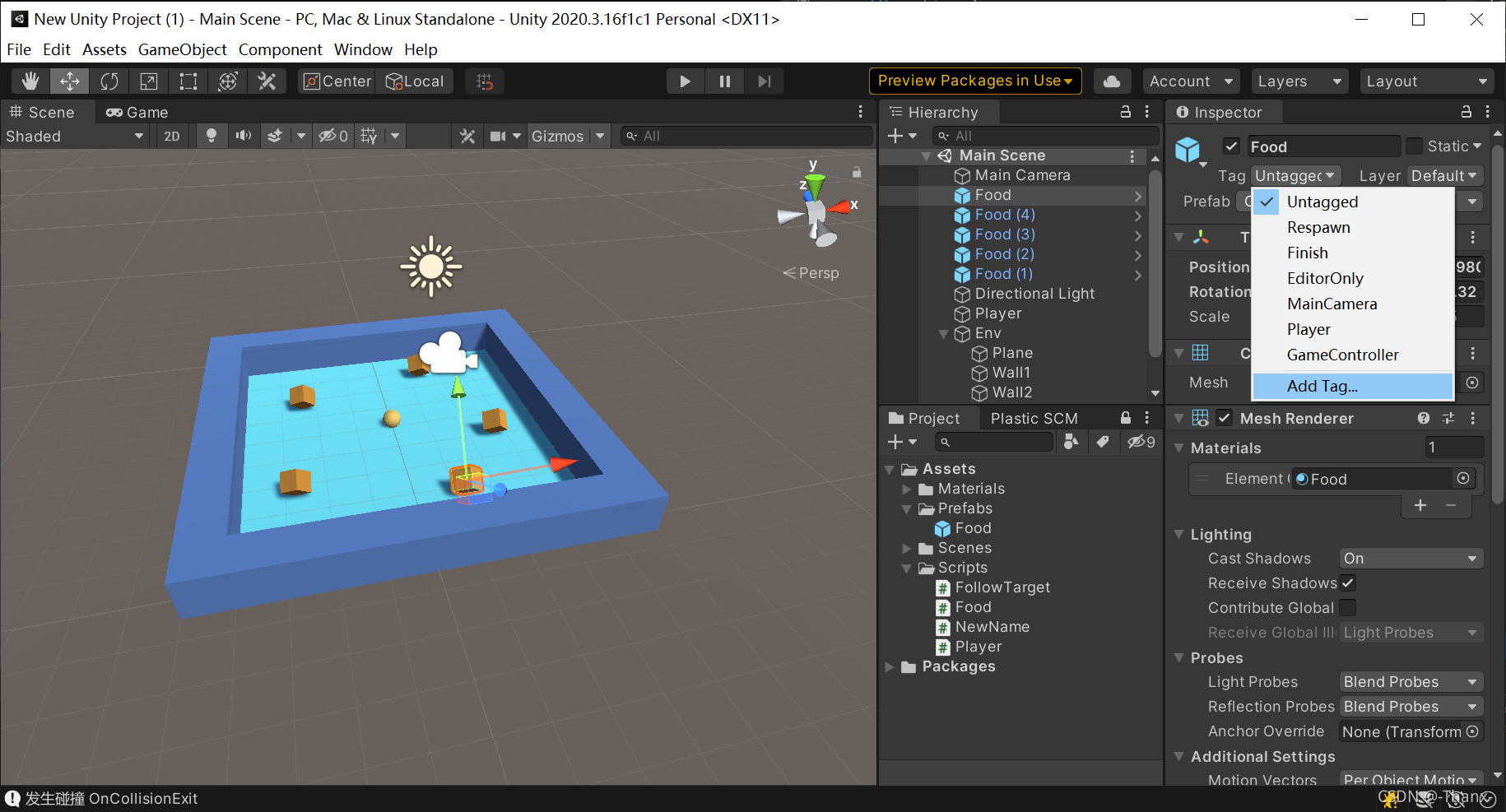 - 碰撞判断语句,碰撞器检测到碰撞物体后判断其tag,若为真,物体销毁
if(collision.gameObject.tag=="Food")
{
Destroy(collision.gameObject);
}
- 碰撞检测弊端:画面会产生碰撞的效果后物体才会消失,吞并食物效果不连贯
六、触发检测
-
将物体的碰撞器部分中的"Is Trigger"勾选上,表示物体拥有触发器属性 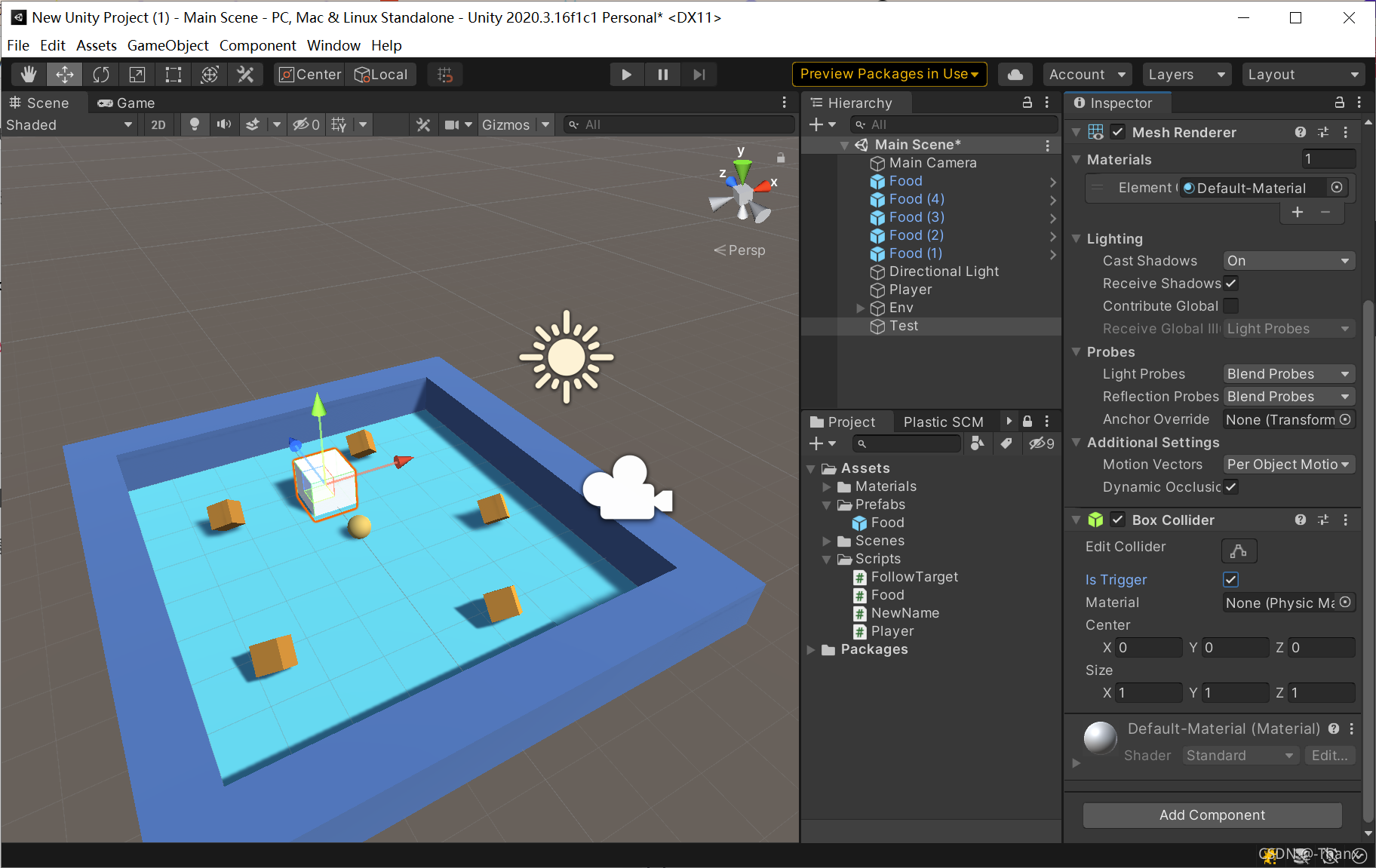 -
勾选后player能对Test物体穿模 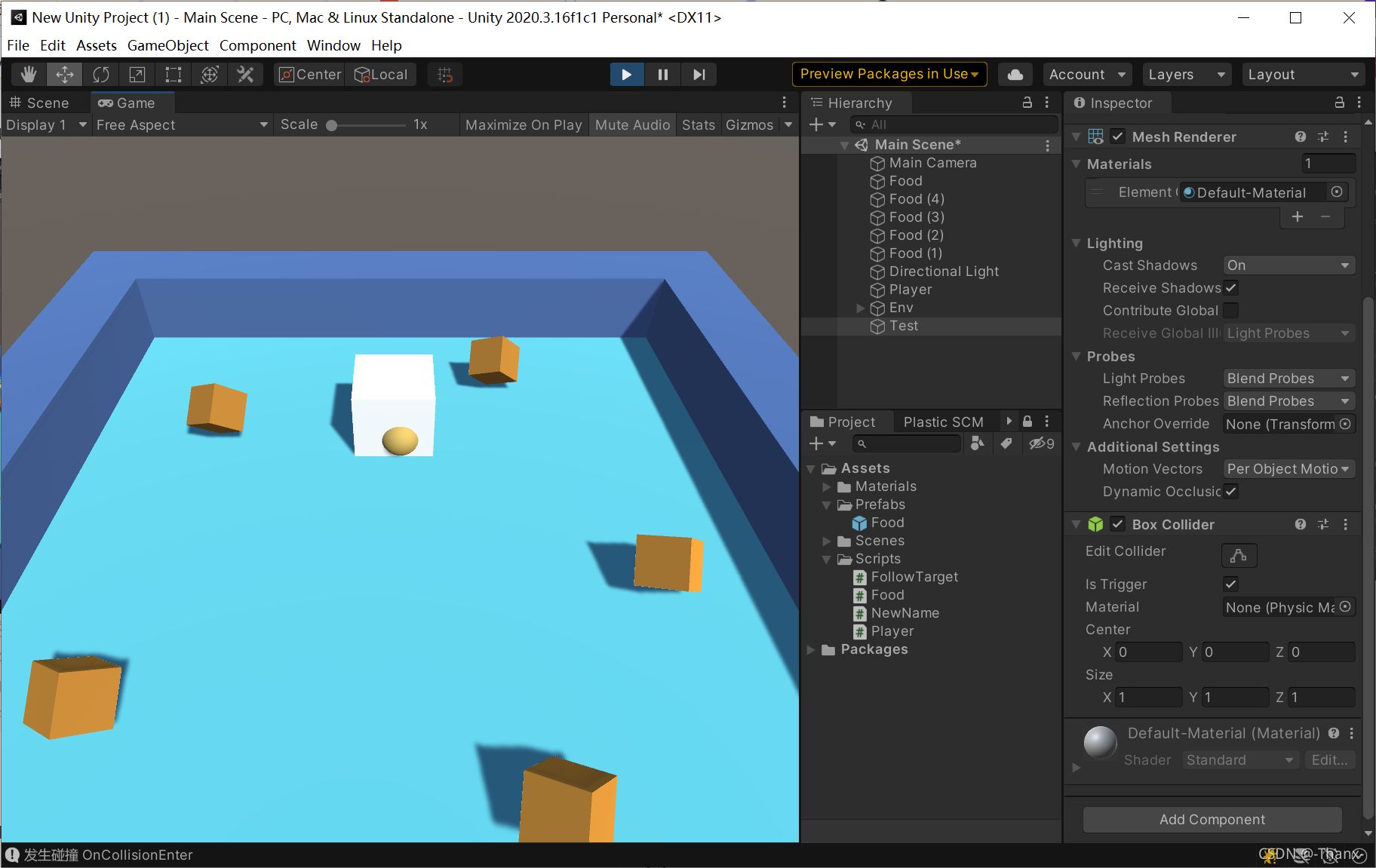 -
触发器出发状态代码如下:
private void OnTriggerEnter(Collider other)
{
Debug.Log("OnTriggerEnter");
}
private void OnTriggerExit(Collider other)
{
Debug.Log("OnTriggerExit");
}
private void OnTriggerStay(Collider other)
{
Debug.Log("OnTriggerStay");
}
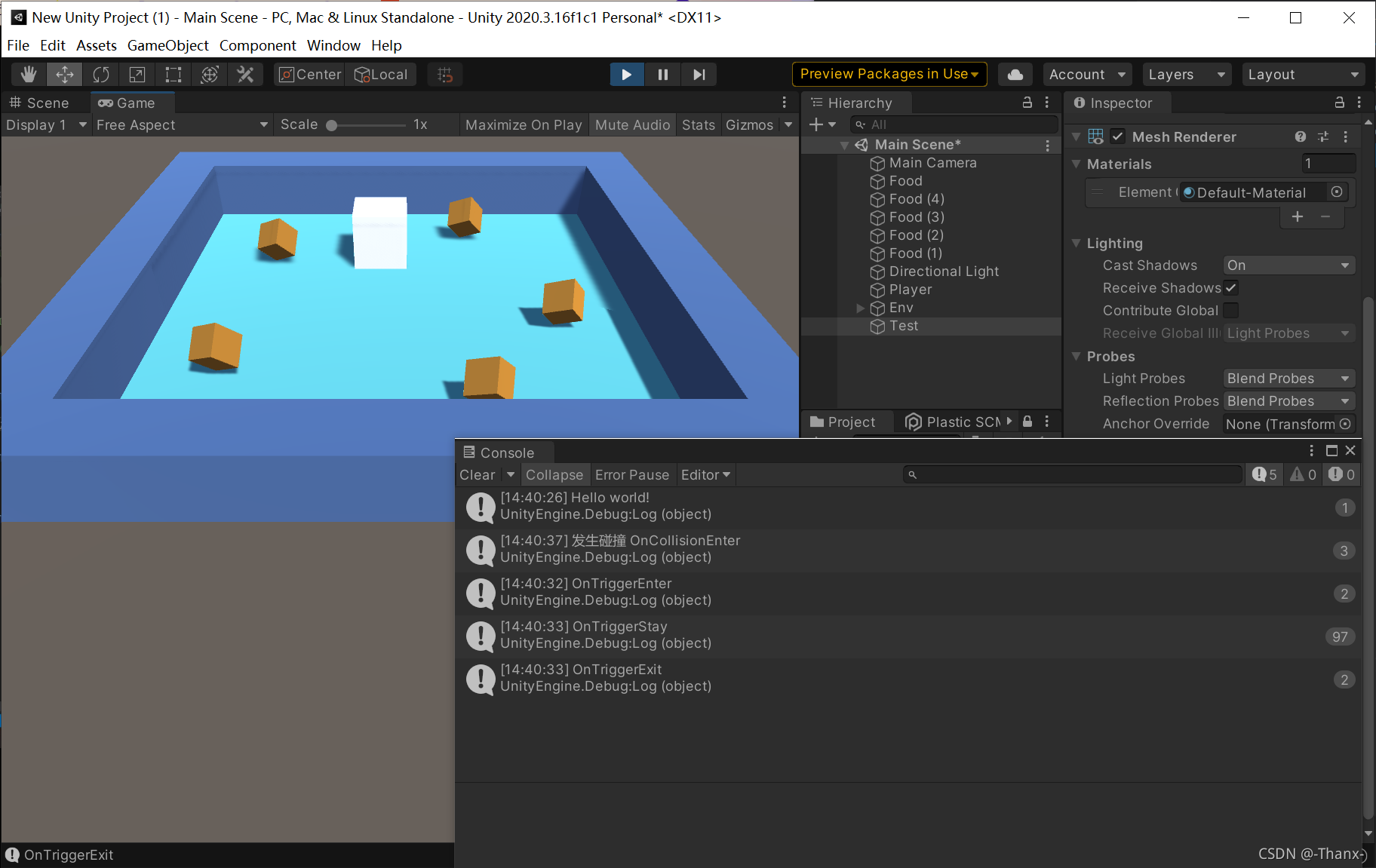
- 触发器代码:直接other.tag,和collision.gameObject.tag不一样,当勾选触发器属性后,碰撞方法内就不会执行
private void OnTriggerEnter(Collider other)
{
Debug.Log("OnTriggerEnter");
if(other.tag=="Food")
{
Destroy(other.gameObject);
}
}
七、通过UI显示得分
-
player.cs内定义全局变量score -
新建UI->text,切换2D模式 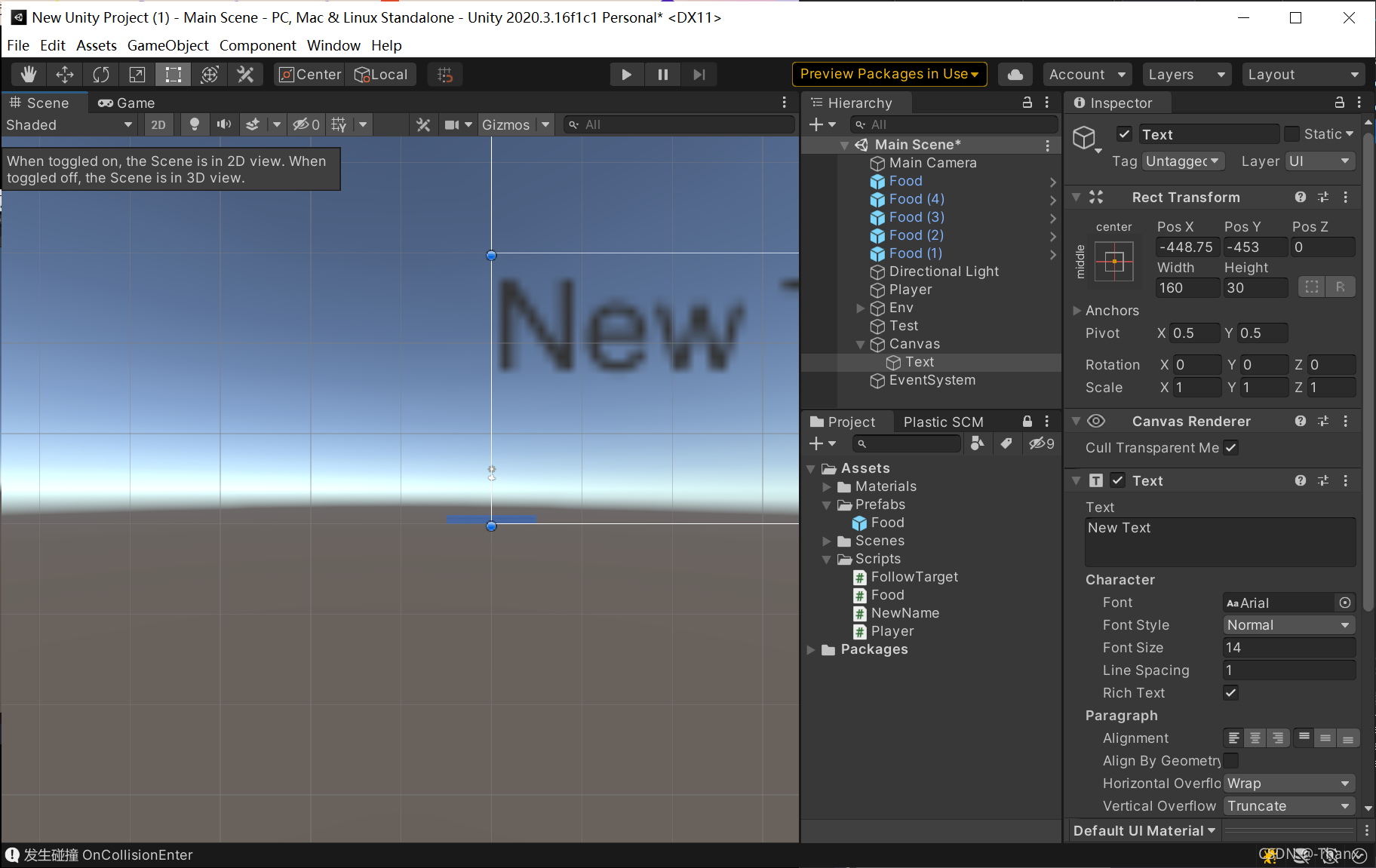 -
Canvas表示屏幕对应的视角,也是画布  -
EventSystem表示事件 -
修改文本位置 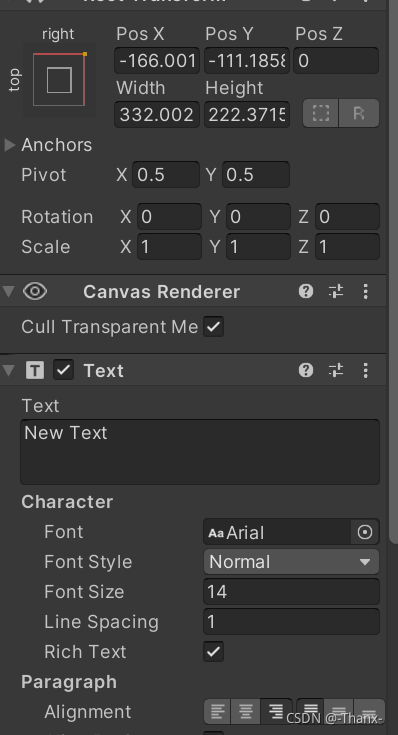 -
引入UI包using UnityEngine.UI; -
定义全局变量Text型scoreTextpublic Text scoreText; 将UI的Text拖入player的Score Text内 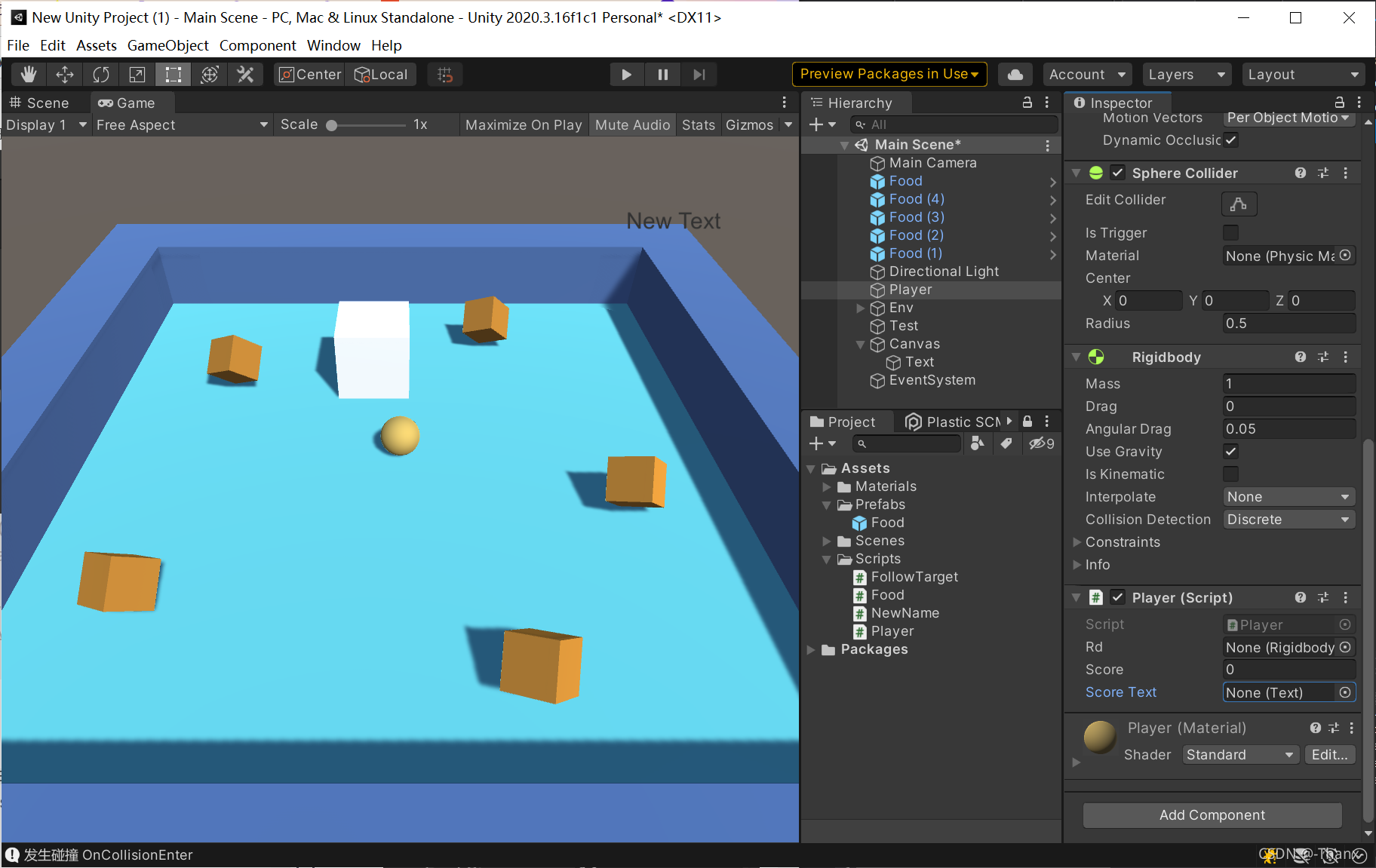 -
之后更新UI显示内容ScoreText.text = "得分:"+score; -
显示游戏胜利 教程中提到的方法: 1、Canvas中新建Text文件WinText,取消勾选Text属性,即false 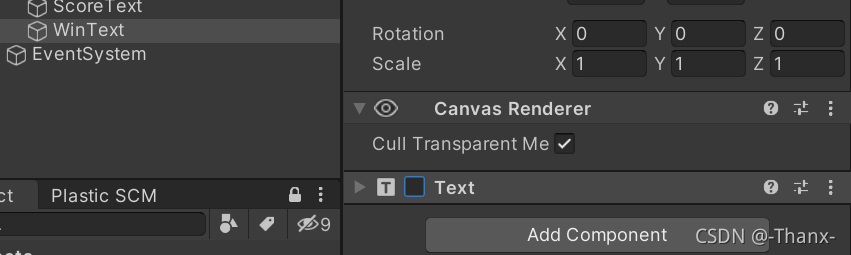 2、创建全局变量GameObject类WinText(注:每一个UI组件本质都是GameObject) 3、游戏中激活WinText的Text属性:WinText.SetActive(true); 使Text为真 4、代码如下:
public GameObject winText;
判定部分:
if (other.tag == "Food")
{
Destroy(other.gameObject);
score++;
scoreText.text = "分数:" + score ;
if(score == 12)
{
winText.SetActive(true);
}
}
public GameObject WinText;
void Start()
{
WinText = GameObject.Find("WinText");
WinText.SetActive(false);
}
判定部分:
if (other.tag == "Food")
{
Destroy(other.gameObject);
score++;
ScoreText.text = "得分:" + score;
if (score == 5)
{
WinText.SetActive(true);
}
}
- 结果展示:(好耶!)
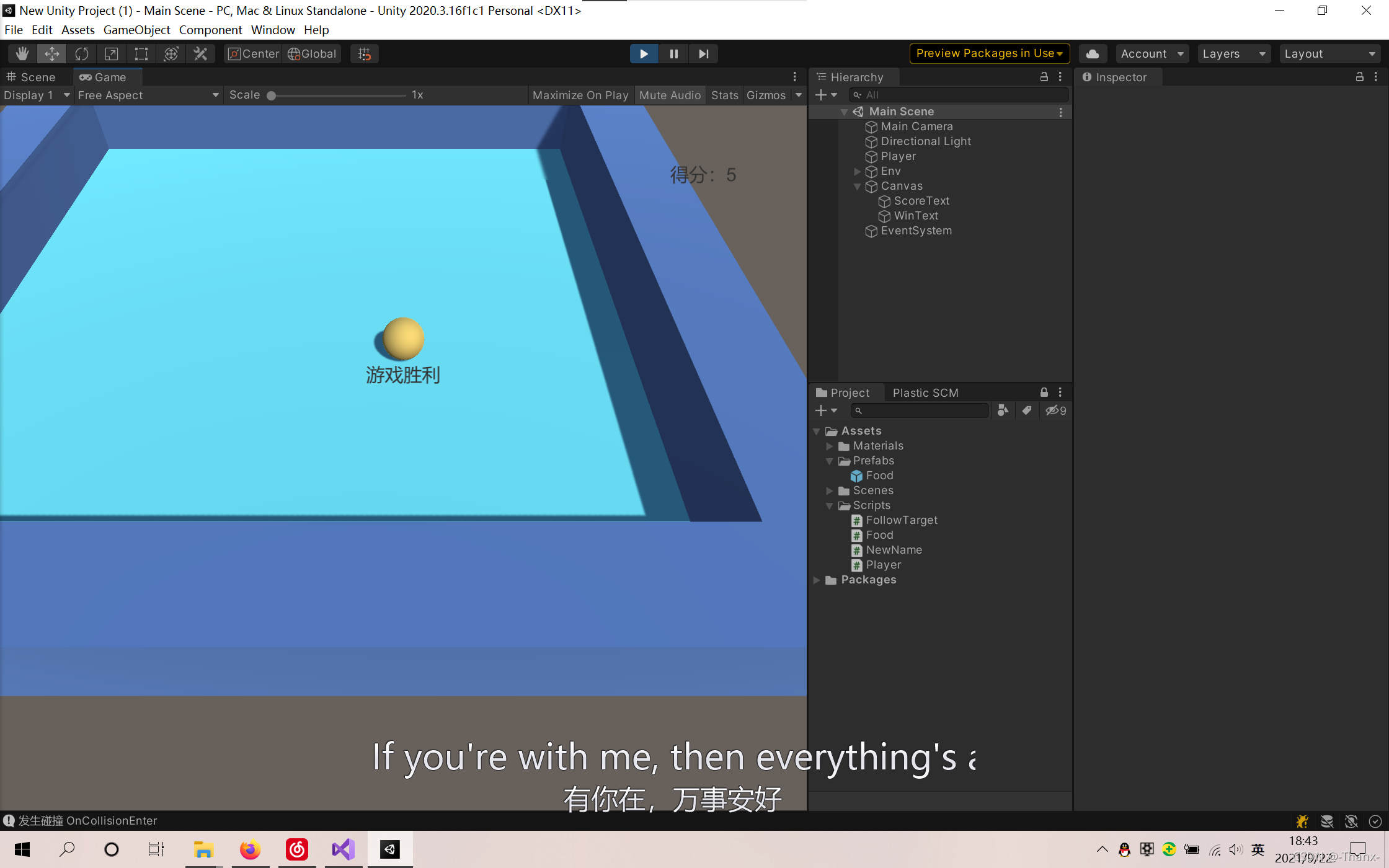
八、游戏打包
- File->Build Settings,直接将scenes拖进去并选择打包平台后即可打包。打包平台可以在UnityHub中下载
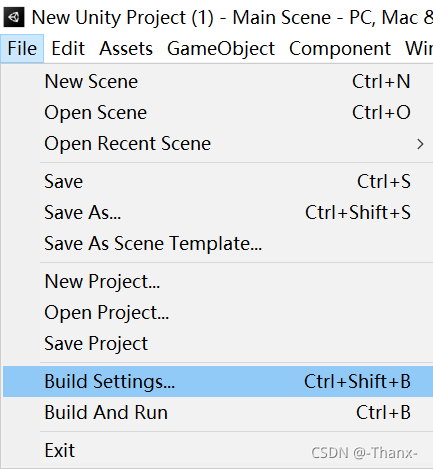 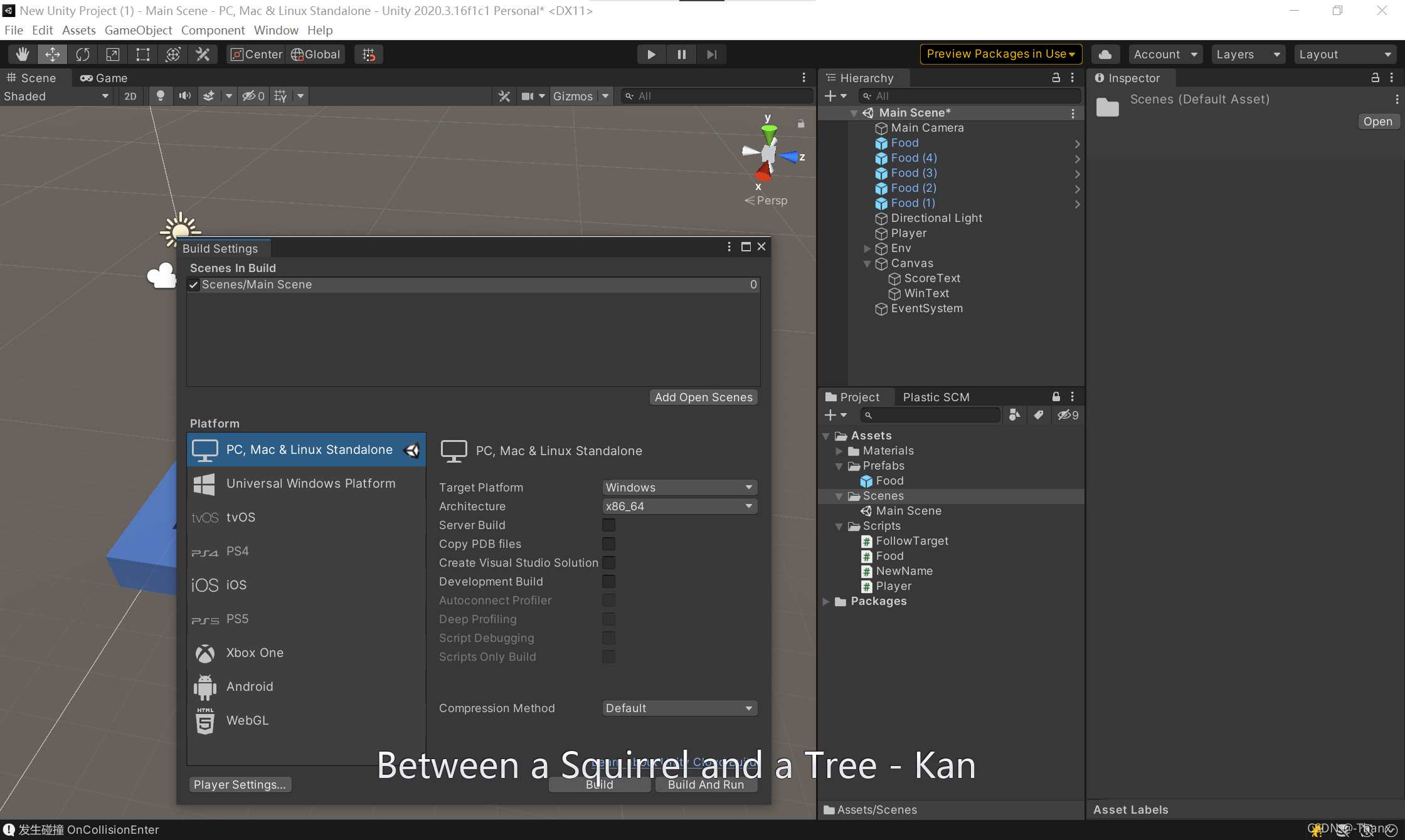
- 修改游戏屏幕设置:打包前选择player settings
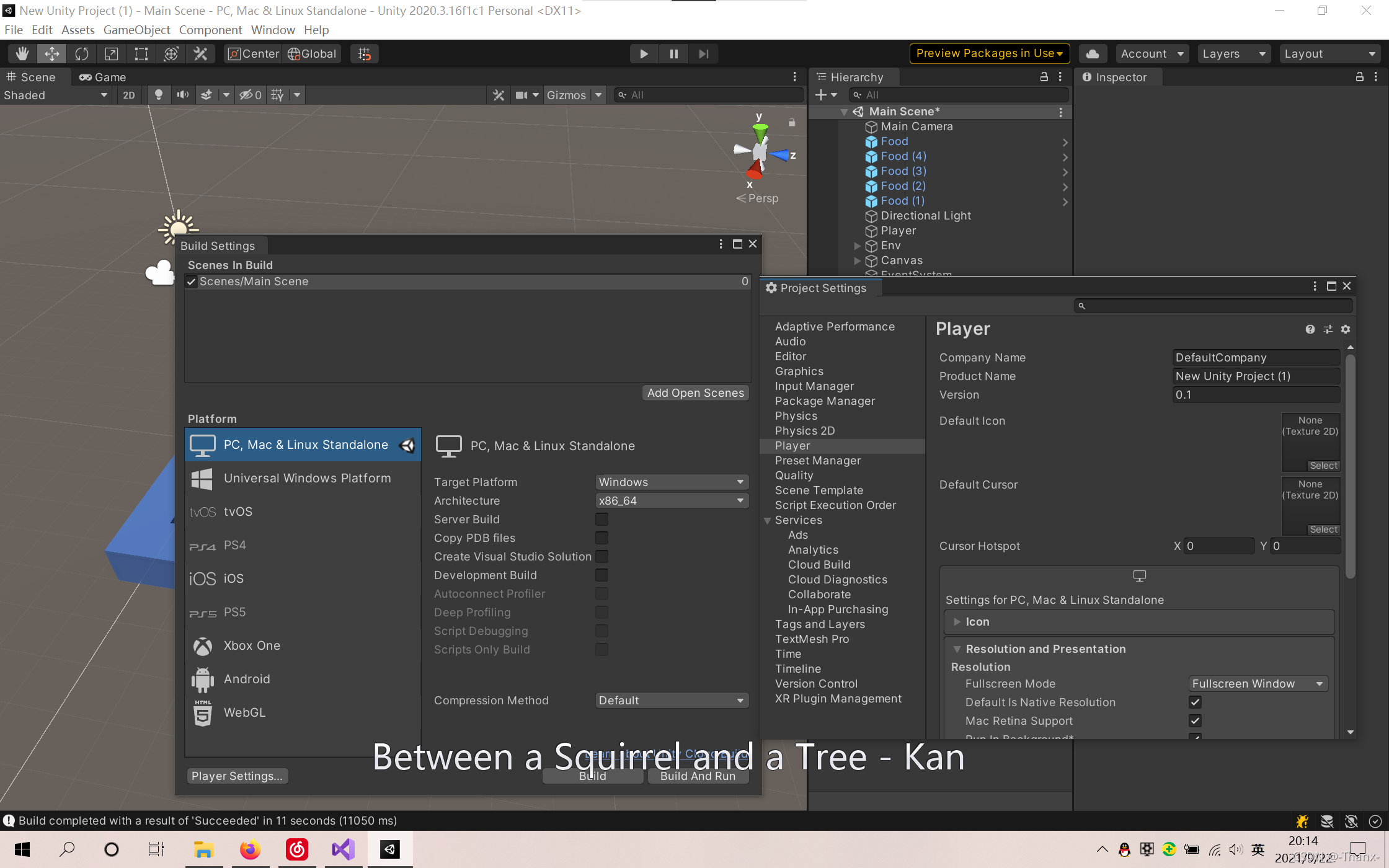 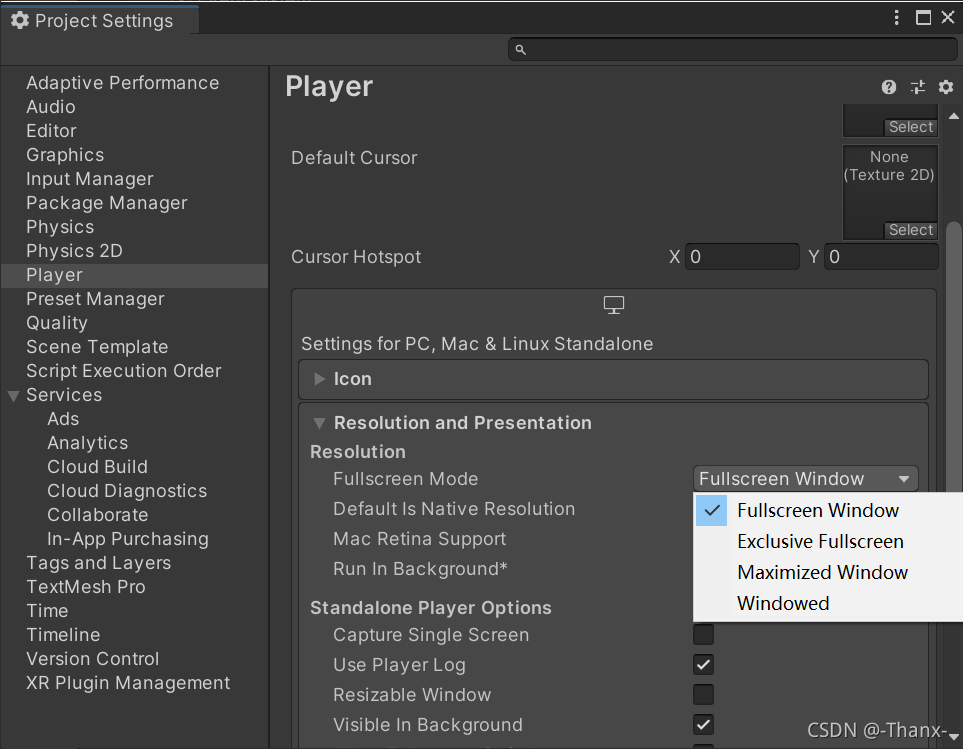
九、加点元素
float times = 3f;
public Food food;
void Update()
{
times -= Time.deltaTime;
if (times < 0)
{
Food obj = (Food)Instantiate(food);
int x = Random.Range(-4, 4);
int z = Random.Range(1, 9);
obj.transform.position = new Vector3(x, 4247512 / 10000000, z);
times = Random.Range(0, 5);
}
}
|