设计模式之观察者模式——猫抓老鼠2:
对猫抓老鼠1再次进行优化,降低代码复杂性,以及使用事件来对方法进行保护
代码优化:
将老鼠代码进行优化,将自己的方法在构造时便注册到猫里面:
using System;
using System.Collections.Generic;
using System.Text;
namespace Unity_Observe_mode
{
class Mouse
{
private string name;
private string color;
public Mouse(string name,string color,Cat cat)
{
this.name = name;
this.color = color;
cat.catCome += this.RunAway;
}
public void RunAway()
{
Console.WriteLine(color + "的老鼠" + name + "说:老猫来了,赶紧跑! ");
}
}
}
猫类代码添加事件:
这里添加事件后,用法与委托一样,主要目的是:防止被外界进行调用,只能在内部触发
using System;
using System.Collections.Generic;
using System.Text;
namespace Unity_Observe_mode
{
class Cat
{
private string name;
private string color;
public Cat(string name,string color)
{
this.name = name;
this.color = color;
}
public void CatComing()
{
Console.WriteLine(color + "的猫" + name + "过来了,喵喵喵!!");
if(catCome!=null)
catCome();
}
public event Action catCome;
}
}
最后Program类:
代码如下:
using System;
using System.Collections.Generic;
using System.Text;
namespace Unity_Observe_mode
{
class Mouse
{
private string name;
private string color;
public Mouse(string name,string color,Cat cat)
{
this.name = name;
this.color = color;
cat.catCome += this.RunAway;
}
public void RunAway()
{
Console.WriteLine(color + "的老鼠" + name + "说:老猫来了,赶紧跑! ");
}
}
}
最后进行测试,得到结果: 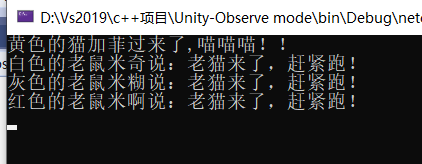 至此设计模式——观察者模式完成。
|