C#光速学习
1、.net
.net一般指.net平台和.net framework 框架。
框架包含于平台。
.net framework 框架包含CLR和.NET类库。
2、C#
用C#开发基于.net平台的应用。
3.net的作用
桌面应用程序(WinForm) 、ASP.net(Internet应用程序)、手机开发、Unity3D游戏开发和虚拟现实。
3.两种交互模式
C/S(WinForm)、B/S(Internet应用程序)。
4.VS的使用
写.net 注意先安装.net组件。
解决方案 包含 项目 包含 类(.cs)
类比公司-部门-员工
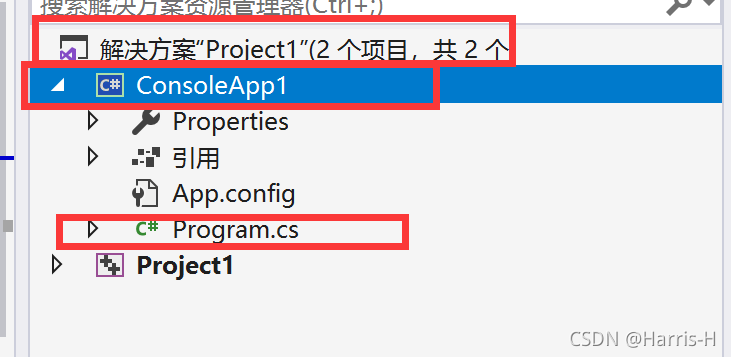
项目的目录结构
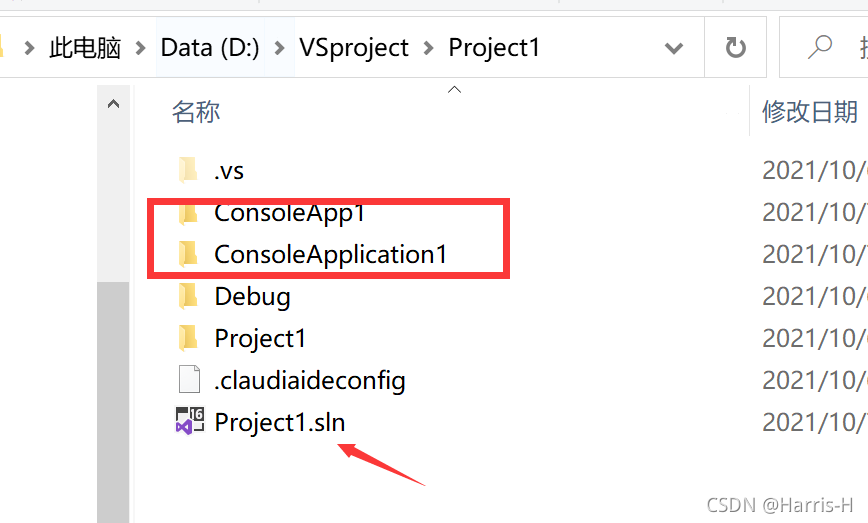
.sln 解决方案文件。
每个项目里面有对应的项目文件
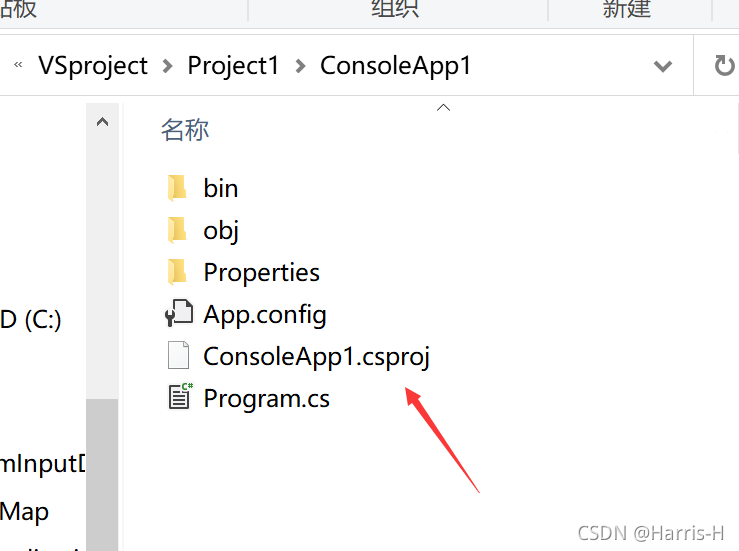
5.HelloWorld
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
Console.ReadKey();
}
}
}
输入cw+两次tab 即可快速打印。
F5 快速调试运行。
6.VS基本设置
多项目怎么启动当前的项目
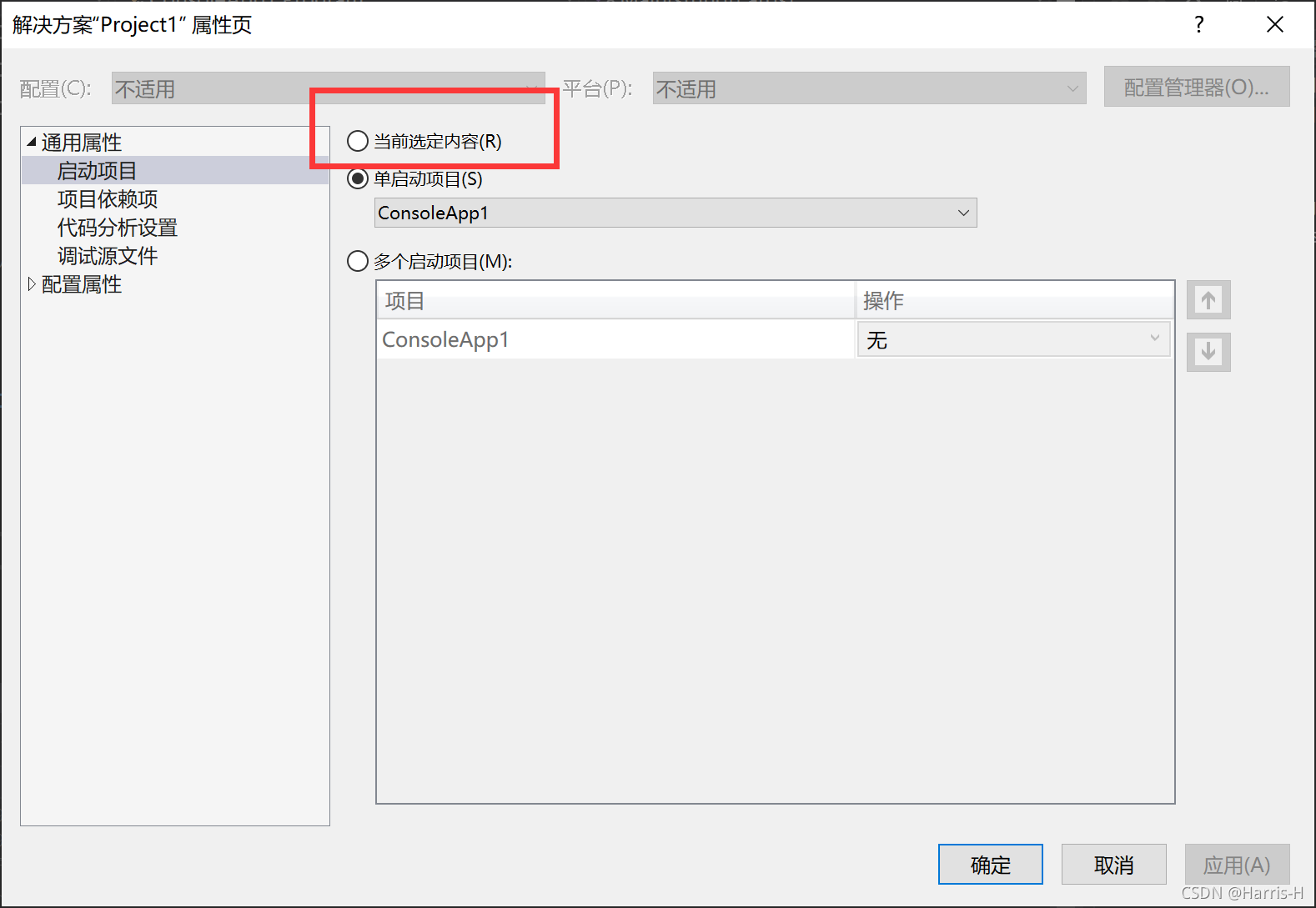
选择当前选定内容即可;
7.C#的注释
- 单行注释 //
- 多行注释 /* */
- 文档注释,一般用于类方法 ///
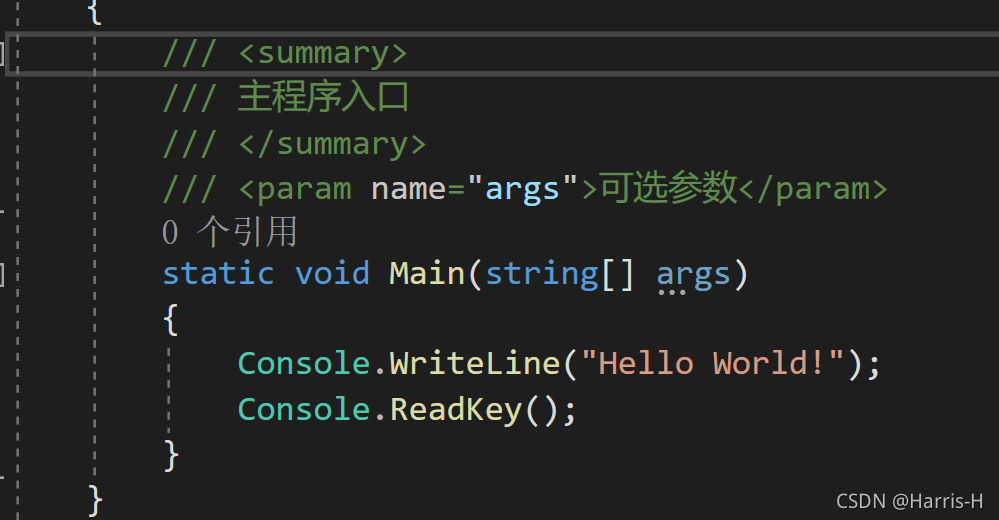
7.VS快捷键
ctrl+s 保存
ctrl+z 撤销
shitf+home shift+end 快速选中 home句首,end句尾。 可以配合上下左右。
折叠代码 #region #endreigon
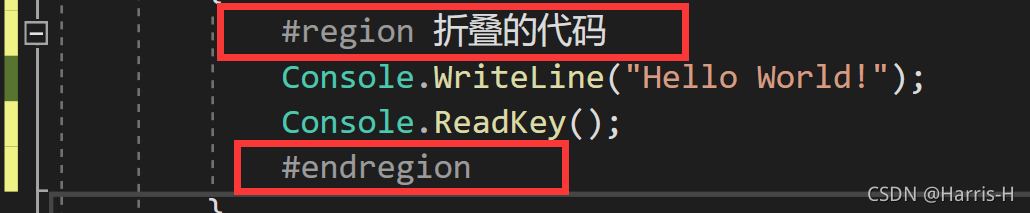 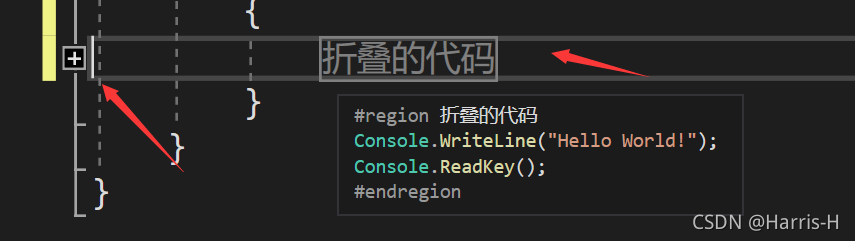
7.基础语法
a.类型
int整数,char字符,string字符串,double浮点数,decimal。
String 也可以用,是所有语言通用,小写string是cs独有。
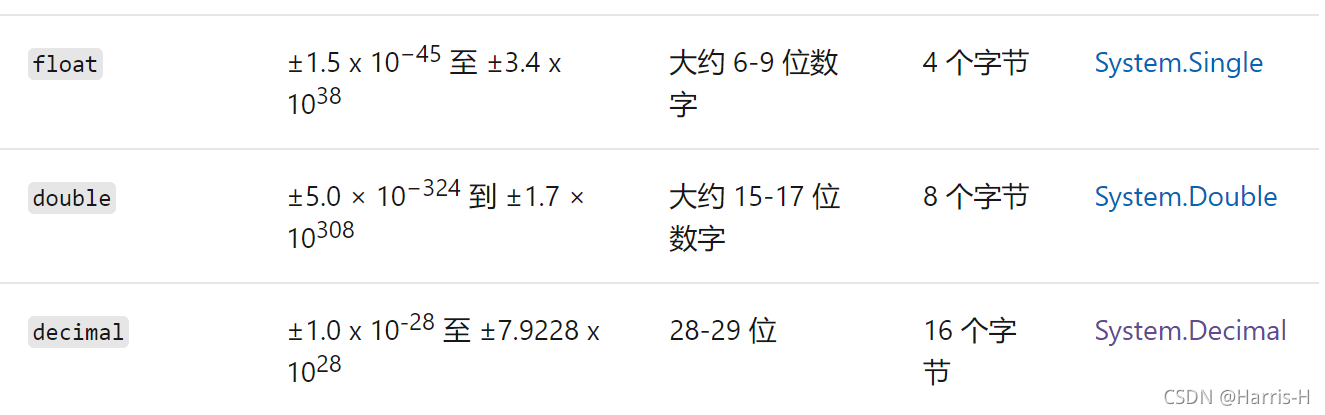
b.+号的使用
当字符串和整数用+连接时,整数会自动转为字符串。
static void Main(string[] args)
{
String s = "123";
Console.WriteLine(5+s);
Console.ReadKey();
}
c.字符串的占位
int a = 10;
int b = 20;
int c = 30;
Console.WriteLine("{0} {2} {1}",a,b,c);
Console.ReadKey();
d.输入
string s = Console.ReadLine();
e.转义符和原生字符串
与C语言类似。
字符串用@表示
string s = @"www.adada\csadasdasd\dsadsad";
Console.WriteLine(s);
Console.ReadKey();
f.类型转换
string s = "12313";
double v = Convert.ToDouble(s);
int a = Convert.ToInt32(s);
Console.WriteLine(v);
Console.WriteLine(a);
Console.ReadKey();
使用TryParse方法:
string s1=Console.ReadLine();
int res = 0;
bool ok = int.TryParse(s1,out res);
Console.WriteLine("ok: "+ok);
Console.WriteLine("res: "+res);
Console.ReadKey();
8.流程控制
a.异常捕获
string s1=Console.ReadLine();
string s2= Console.ReadLine();
double v = 0;
int a = 0;
try
{
v = Convert.ToDouble(s1);
a = Convert.ToInt32(s2);
}
catch
{
Console.WriteLine("格式错误");
}
Console.WriteLine(v);
Console.WriteLine(a);
Console.ReadKey();
b.断点调试
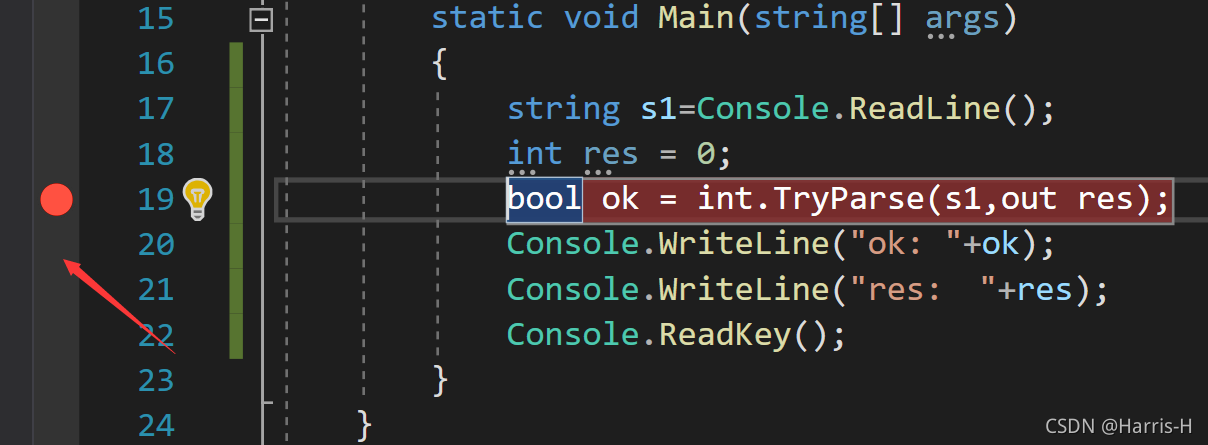
灰色区域左键即可添加端点,F11分步调试。
c.枚举
public enum gender
{
男,
女
}
public enum name
{
herio,
harris
}
gender g1 = gender.男;
name n1 = name.herio;
Console.WriteLine("name: {0} gender: {1}",n1,g1);
Console.ReadKey();
枚举能与int类型相互转换。
所有类型都能转换为string类型,使用toString()。
int number = 2;
name n1 = (name)number;
Console.WriteLine(n1);
int new_number = (int)n1;
Console.WriteLine("new_number: "+new_number);
string s = n1.ToString();
Console.WriteLine("name: "+s);
Console.ReadKey();
string 转换为enum 使用parse
string s1 = "0";
string s2 = "男";
gender g1 = (gender) Enum.Parse(typeof(gender), s1);
gender g2 = (gender)Enum.Parse(typeof(gender), s2);
Console.WriteLine("g1:" +g1);
Console.WriteLine("g2: " + g2);
Console.ReadKey();
9.复杂数据类型
学习视频
https://www.bilibili.com/video/BV1FJ411W7e5?p=67
|