【Unity扩展】- Button UIButtonSuper
前言
unity UIGUI 系统原本自带Button 只有点击功能,由于现在项目需要双击,长按等效果,原本的button不支持,然后扩展了UGUI Button,增加了双击,长按功能,修改的代码也很少。
先看图 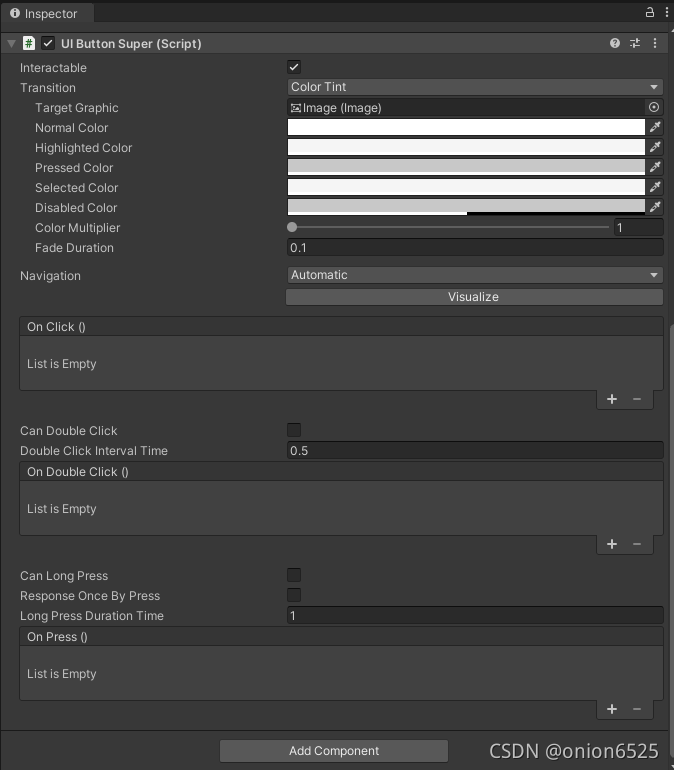
下边是源码
1.UIButtonSuper
代码如下(示例):
using System.Collections;
using LuaInterface;
using UnityEngine;
using UnityEngine.Events;
using UnityEngine.EventSystems;
using UnityEngine.Serialization;
using UnityEngine.UI;
#if UNITY_EDITOR
using UnityEditor;
using UnityEditor.UI;
[NoToLua]
[CustomEditor(typeof(UIButtonSuper),true)]
[CanEditMultipleObjects]
public class UIButtonSuperEditor : ButtonEditor
{
private SerializedProperty m_CanDoubleClick;
private SerializedProperty m_DoubleClickIntervalTime;
private SerializedProperty onDoubleClick;
private SerializedProperty m_CanLongPress;
private SerializedProperty m_ResponseOnceByPress;
private SerializedProperty m_LongPressDurationTime;
private SerializedProperty onPress;
protected override void OnEnable()
{
base.OnEnable();
m_CanDoubleClick = serializedObject.FindProperty("m_CanDoubleClick");
m_DoubleClickIntervalTime = serializedObject.FindProperty("m_DoubleClickIntervalTime");
onDoubleClick = serializedObject.FindProperty("onDoubleClick");
m_CanLongPress = serializedObject.FindProperty("m_CanLongPress");
m_ResponseOnceByPress = serializedObject.FindProperty("m_ResponseOnceByPress");
m_LongPressDurationTime = serializedObject.FindProperty("m_LongPressDurationTime");
onPress = serializedObject.FindProperty("onPress");
}
[NoToLua]
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
serializedObject.Update();
EditorGUILayout.Space();
EditorGUILayout.PropertyField(m_CanDoubleClick);
EditorGUILayout.PropertyField(m_DoubleClickIntervalTime);
EditorGUILayout.PropertyField(onDoubleClick);
EditorGUILayout.Space();
EditorGUILayout.PropertyField(m_CanLongPress);
EditorGUILayout.PropertyField(m_ResponseOnceByPress);
EditorGUILayout.PropertyField(m_LongPressDurationTime);
EditorGUILayout.PropertyField(onPress);
serializedObject.ApplyModifiedProperties();
}
}
#endif
public class UIButtonSuper : Button
{
[Tooltip("是否可以双击")]
public bool m_CanDoubleClick = false;
[Tooltip("双击间隔时长")]
public float m_DoubleClickIntervalTime = 0.5f;
[Tooltip("双击事件")]
public ButtonClickedEvent onDoubleClick;
[Tooltip("是否可以长按")]
public bool m_CanLongPress = false;
[Tooltip("长按是否只响应一次")]
public bool m_ResponseOnceByPress = false;
[Tooltip("长按满足间隔")]
public float m_LongPressDurationTime = 1;
[Tooltip("长按事件")]
public ButtonClickedEvent onPress;
private bool isDown = false;
private bool isPress = false;
private float downTime = 0;
private float clickIntervalTime = 0;
private int clickTimes = 0;
void Update() {
if (isDown) {
if (!m_CanLongPress)
{
return;
}
if (m_ResponseOnceByPress && isPress) {
return;
}
downTime += Time.deltaTime;
if (downTime > m_LongPressDurationTime) {
isPress = true;
onPress.Invoke();
}
}
if (clickTimes >= 1) {
clickIntervalTime += Time.deltaTime;
if (clickIntervalTime >= m_DoubleClickIntervalTime) {
if (clickTimes >= 2) {
if (m_CanDoubleClick)
{
onDoubleClick.Invoke();
}
}
else {
onClick.Invoke();
}
clickTimes = 0;
clickIntervalTime = 0;
}
}
}
public override void OnPointerDown(PointerEventData eventData) {
base.OnPointerDown(eventData);
isDown = true;
downTime = 0;
}
public override void OnPointerUp(PointerEventData eventData) {
base.OnPointerUp(eventData);
isDown = false;
}
public override void OnPointerExit(PointerEventData eventData) {
base.OnPointerExit(eventData);
isDown = false;
isPress = false;
}
public override void OnPointerClick(PointerEventData eventData) {
base.OnPointerClick(eventData);
if (!isPress ) {
clickTimes += 1;
}
else
isPress = false;
}
}
2.示例
和我们之前用Button一样 代码如下(示例):
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.EventSystems;
using UnityEngine.UI;
public class TestButton : MonoBehaviour
{
public UIButtonSuper testBtn;
void Start()
{
testBtn.onClick.AddListener(Click);
testBtn.onPress.AddListener(Press);
testBtn.onDoubleClick.AddListener(DoubleClick);
}
void Update()
{
}
void Click() {
Debug.Log("click");
}
void Press() {
Debug.Log("press");
}
void DoubleClick() {
Debug.Log("double click");
}
}
总结
修改代码少,原来功能全部持有,增加双击,长按功能,使用习惯还是之前UGUI Button习惯
|