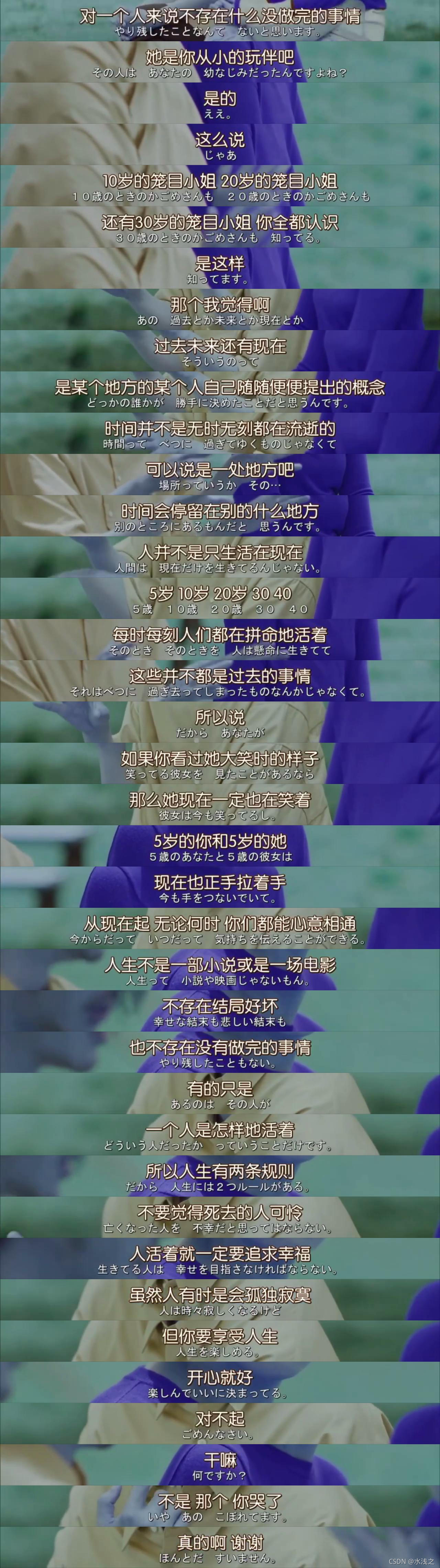
import cv2
from PIL import Image
video_path = "C:/Users/koala/Videos/movie.mp4"
video_capture = cv2.VideoCapture(video_path)
ret,frame = video_capture.read()
subtitle_shape = [frame.shape[0],frame.shape[1],120]
times = [0.5,5,9,10,11,14,17,19,24,26,29,35,37,40,45,49,52,58,60,62,67,71,77,85,90,94,97,99,104,109,115,121,124,127,136,139,141,144]
FPS = video_capture.get(5)
clip_images = []
base_path = "D:/subtitle.jpg"
def get_key_area(video,times,subtitle_shape):
key_frame = 0
current_frame = 0
while True:
ret,frame = video.read()
if ret:
current_frame += 1
if key_frame < len(times) and current_frame == times[key_frame]*FPS:
key_frame += 1
clip = frame[subtitle_shape[0]-subtitle_shape[2]:subtitle_shape[0],0:subtitle_shape[1]]
clip_images.append(clip)
else:
break
print("匹配完成 共计图片 数目为:%d "%len(clip_images))
video.release()
def main():
get_key_area(video_capture,times,subtitle_shape)
subtitle_image = Image.new("RGB",(subtitle_shape[1],subtitle_shape[2]*len(clip_images)))
for index,item in enumerate(clip_images):
img = Image.fromarray(item)
subtitle_image.paste(img,box=(0,index*subtitle_shape[2],subtitle_shape[1],subtitle_shape[2]*(index+1)))
subtitle_image.save(base_path)
subtitle_image.show()
if __name__ == '__main__':
main()
|