仅个人记录,方便以后忘掉回来看看 服务器买的是腾讯云的,系统是 CentOS。
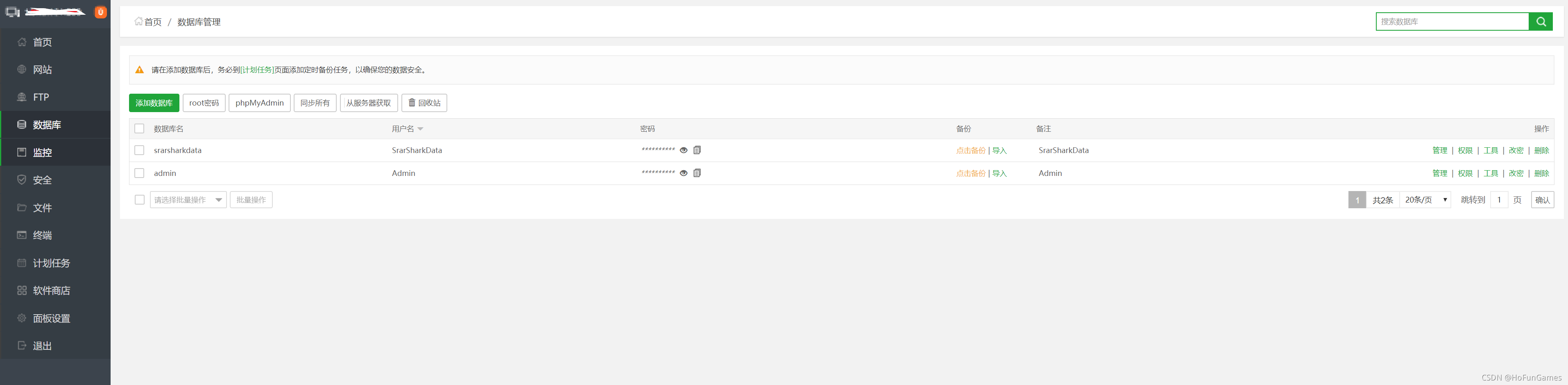
管理工具用的是万胜云面板(好像也叫宝塔),数据库都是在这里面整的,用到的表提前在里面创建好了
using MySql.Data.MySqlClient;
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MySqlManager : Singleton<MySqlManager>
{
protected static string m_databaseIP = "111.50.100.200";
protected static string m_databasePort = "3306";
protected static string m_userID = "SrarSharkDataTest";
protected static string m_password = "StarShark1234";
protected static string m_databaseName = "srarsharkdataall";
protected static string m_connectionString;
public static MySqlConnection connection;
public bool TestConnection()
{
bool isConnected = true;
using (MySqlConnection connection = new MySqlConnection(m_connectionString))
{
try
{
connection.Open();
Debug.Log("打开了数据库");
}
catch (MySqlException E)
{
isConnected = false;
throw new Exception(E.Message);
}
finally
{
connection.Close();
Debug.Log("关闭数据库");
}
}
return isConnected;
}
public static void Init()
{
m_connectionString = string.Format("Server = {0}; port = {1}; Database = {2}; User ID = {3}; Password = {4}; Pooling=true; Charset = utf8;", m_databaseIP, m_databasePort, m_databaseName, m_userID, m_password);
using (connection = new MySqlConnection(m_connectionString))
{
try
{
connection.Open();
Debug.Log("打开了数据库");
}
catch (MySqlException E)
{
Debug.Log("连接失败");
throw new Exception(E.Message);
}
finally
{
connection.Close();
Debug.Log("关闭数据库");
}
}
Debug.Log(m_connectionString);
}
public void Click_login(string username, string password)
{
try
{
connection.Open();
Debug.Log("------输入的用户名---" + username + "-密码-" + password);
string sqlQuary = "select * from StarSharkData where UserName ='" + username + "' and UserPassword = '" + password + "'";
MySqlCommand comd = new MySqlCommand(sqlQuary, connection);
MySqlDataReader reader = comd.ExecuteReader();
if (reader.Read())
{
Debug.Log("------用户存在,登录成功!------");
}
else
{
Debug.Log("------用户不存在,请注册。或请检查用户名或和密码!------");
}
}
catch (System.Exception e)
{
Debug.Log(e.Message);
}
finally
{
connection.Close();
}
}
public void Click_register(string username, string password)
{
if (!(username.Length > 0 && password.Length > 0))
{
Debug.Log("-----用户名和密码必须>0------");
return;
}
try
{
connection.Open();
Debug.Log("-----连接成功!------");
string sqlQuary = "select * from StarSharkData where UserName =@paral1 and UserPassword = @paral2";
MySqlCommand comd = new MySqlCommand(sqlQuary, connection);
comd.Parameters.AddWithValue("paral1", username);
comd.Parameters.AddWithValue("paral2", password);
MySqlDataReader reader = comd.ExecuteReader();
if (reader.Read())
{
Debug.Log("-----用户名已存在,请重新输!------");
connection.Close();
}
else
{
connection.Close();
Insert_User(username, password);
}
}
catch (System.Exception e)
{
Debug.Log(e.Message);
}
finally
{
connection.Close();
}
}
}
数据库管理脚本,提供的连接服务器,以及登录、注册功能
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class MySqlTest : MonoBehaviour
{
public Button SignIn;
public Button Register;
public Button Activation;
public InputField InputUser;
public InputField InputPassword;
void Start()
{
MySqlManager.Init();
SignIn.onClick.AddListener(()=>
{
MySqlManager.Instance.Click_login(InputUser.text, InputPassword.text);
});
Register.onClick.AddListener(()=>
{
MySqlManager.Instance.Click_register(InputUser.text, InputPassword.text);
});
}
测试登录和注册的用户脚本
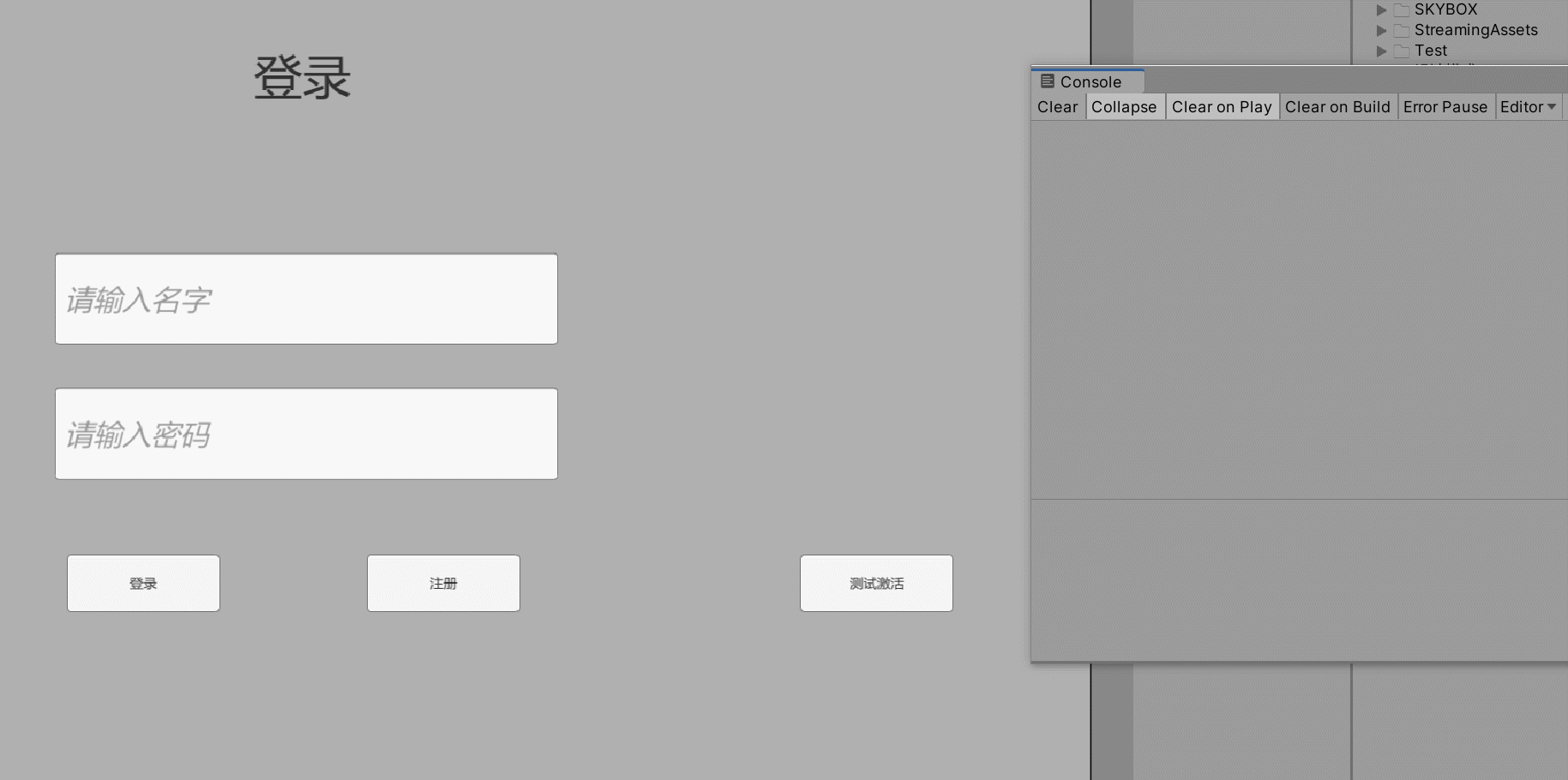 运行后的结果
|