更新日期:2021年10月16日。 Github源码:[点我获取源码] Gitee源码:[点我获取源码]
TableView表格视图
使用TableView 可以很方便的绘制表格视图,当然,实际上它就是使用了TreeView进行绘制而已,所以关于TreeView的一些信息我便不再讲解了,如有什么不明白的地方可以参考官方脚本手册。
使用
定义表格行
TableView 的使用非常简单,首先,表格的数据对应了一个List集合,其中每一行对应集合中的每一个元素,所以,我们先自定义一个表格数据集合:
public class Student
{
public string name;
public Sex sex;
public int age;
public string grade;
public string address;
public GameObject entity;
public Color col;
public Texture headImage;
}
public enum Sex
{
Man,
Woman
}
List<Student> _students = new List<Student>();
定义表格列
然后,我们需要定义表格列对象TableColumn 的集合,他告诉了TableView 我们的表格需要绘制多少列,每列如何绘制数据等,如下,定义一个列,用于绘制数据类Student 的name 字段:
new TableColumn<Student>()
{
headerContent = new GUIContent("姓名"),
width = 100,
canSort = true,
Compare = (a, b) =>
{
return a.name.CompareTo(b.name);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.name = EditorGUI.TextField(cellRect, data.name);
},
}
如此,便定义了表格中某一列的规则,我们依葫芦画瓢的加入Student 的其他字段的列绘制规则:
List<TableColumn<Student>> _tableColumns = new List<TableColumn<Student>>();
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("姓名"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.name.CompareTo(b.name);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.name = EditorGUI.TextField(cellRect, data.name);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("性别"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.sex.CompareTo(b.sex);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.sex = (Sex)EditorGUI.EnumPopup(cellRect, data.sex);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("年龄"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.age.CompareTo(b.age);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.age = EditorGUI.IntField(cellRect, data.age);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("班级"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.grade.CompareTo(b.grade);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.grade = EditorGUI.TextField(cellRect, data.grade);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("家庭住址"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.address.CompareTo(b.address);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.address = EditorGUI.TextField(cellRect, data.address);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("实体"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.entity = (GameObject)EditorGUI.ObjectField(cellRect, data.entity, typeof(GameObject), true);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("颜色"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.col = EditorGUI.ColorField(cellRect, data.col);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("头像"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.headImage = (Texture)EditorGUI.ObjectField(cellRect, data.headImage, typeof(Texture), true);
},
});
表格视图
然后通过上面的表格行 和表格列 便可以生成一个表格视图 :
TableView<Student> _tableView = new TableView<Student>(_students, _tableColumns);
此时,我们调用他的OnGUI 方法便可以直接绘制出表格:
_tableView.OnGUI(new Rect(0, 0, width, height));
如下图,由于_students 的默认数据长度为0,所以现在一条数据也没有: 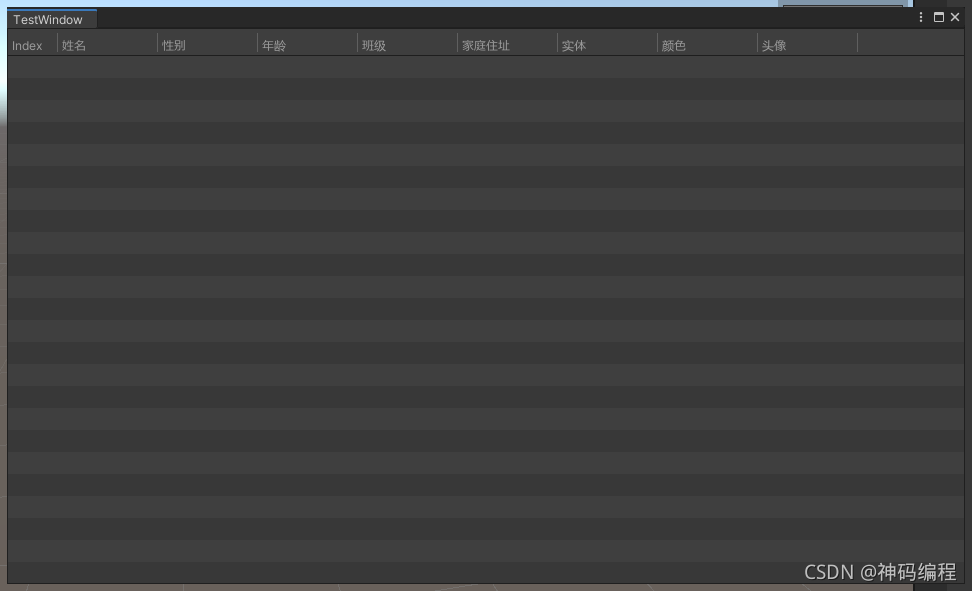 我们点击右键,可以添加数据 ,或删除已选数据 :
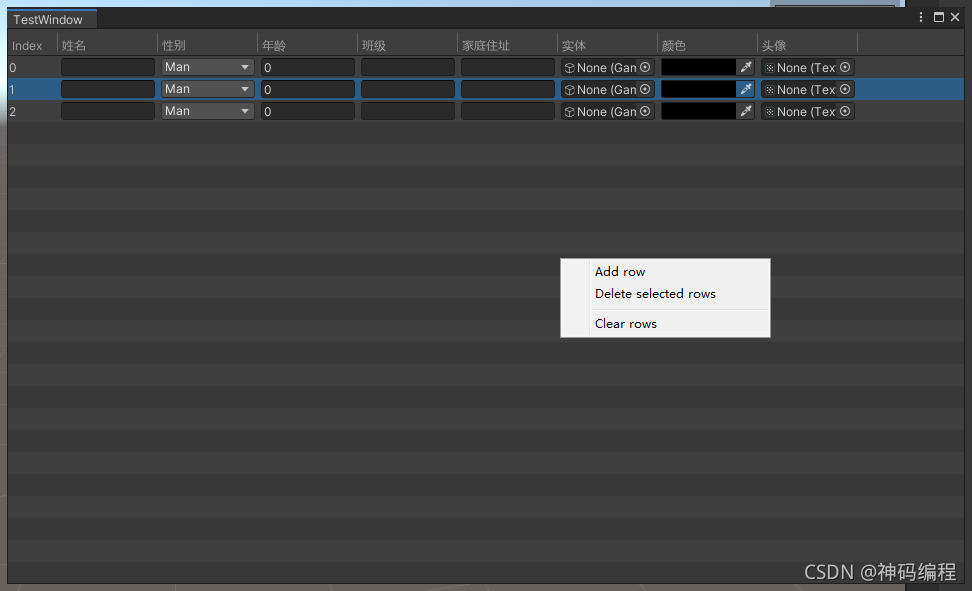 表格视图的基本使用就这么简单,如下是demo源码:
public class TestWindow : HTFEditorWindow
{
[MenuItem("Test/Table Window")]
private static void OpenWindow()
{
GetWindow<TestWindow>();
}
private List<Student> _students;
private List<TableColumn<Student>> _tableColumns;
private TableView<Student> _tableView;
protected override void OnEnable()
{
base.OnEnable();
_students = new List<Student>();
_tableColumns = new List<TableColumn<Student>>();
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("姓名"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.name.CompareTo(b.name);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.name = EditorGUI.TextField(cellRect, data.name);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("性别"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.sex.CompareTo(b.sex);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.sex = (Sex)EditorGUI.EnumPopup(cellRect, data.sex);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("年龄"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.age.CompareTo(b.age);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.age = EditorGUI.IntField(cellRect, data.age);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("班级"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.grade.CompareTo(b.grade);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.grade = EditorGUI.TextField(cellRect, data.grade);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("家庭住址"),
width = 100,
canSort = true,
autoResize = false,
Compare = (a, b) =>
{
return a.address.CompareTo(b.address);
},
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.address = EditorGUI.TextField(cellRect, data.address);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("实体"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.entity = (GameObject)EditorGUI.ObjectField(cellRect, data.entity, typeof(GameObject), true);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("颜色"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.col = EditorGUI.ColorField(cellRect, data.col);
},
});
_tableColumns.Add(new TableColumn<Student>()
{
headerContent = new GUIContent("头像"),
width = 100,
canSort = false,
autoResize = false,
Compare = null,
DrawCell = (cellRect, data, rowIndex, isSelected, isFocused) =>
{
data.headImage = (Texture)EditorGUI.ObjectField(cellRect, data.headImage, typeof(Texture), true);
},
});
_tableView = new TableView<Student>(_students, _tableColumns);
}
protected override void OnBodyGUI()
{
base.OnBodyGUI();
_tableView.OnGUI(new Rect(0, 0, position.width, position.height));
}
public class Student
{
public string name;
public Sex sex;
public int age;
public string grade;
public string address;
public GameObject entity;
public Color col;
public Texture headImage;
}
public enum Sex
{
Man,
Woman
}
}
通用表格检视器
针对挂到物体上的组件,可以对其的自定义类型的数组、集合 字段使用通用表格检视器,他将会自动将其的可序列化字段展开到一个表格视图中,可以很便捷的进行预览和编辑数据:
检视器面板数据: 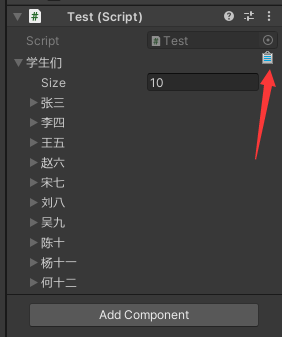 展开至通用表格窗口: 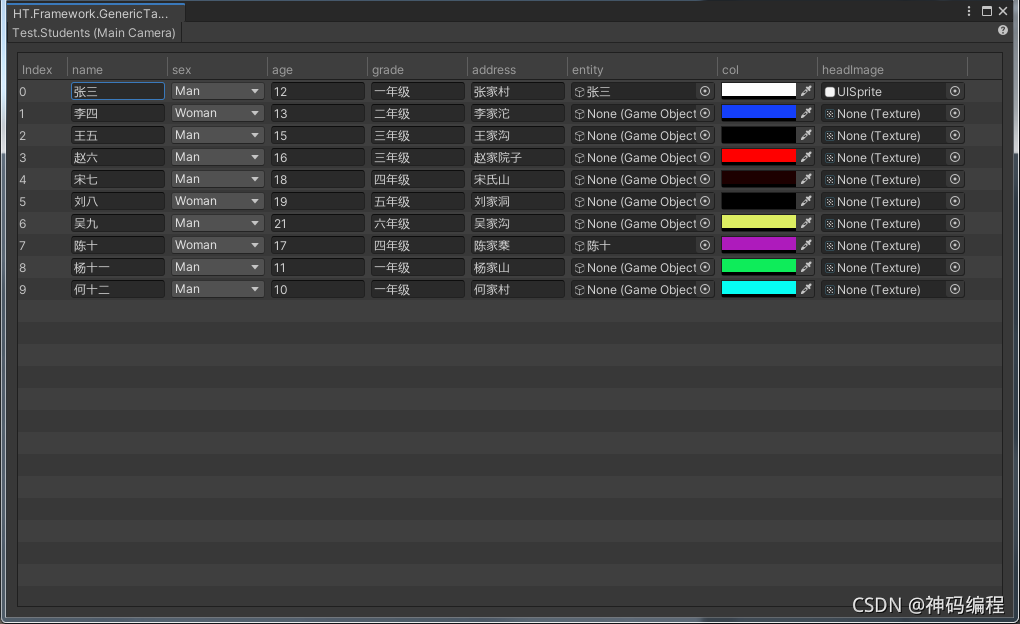
|