这个项目分为头文件和CPP文件和一个.txt文件
实现功能如图所示 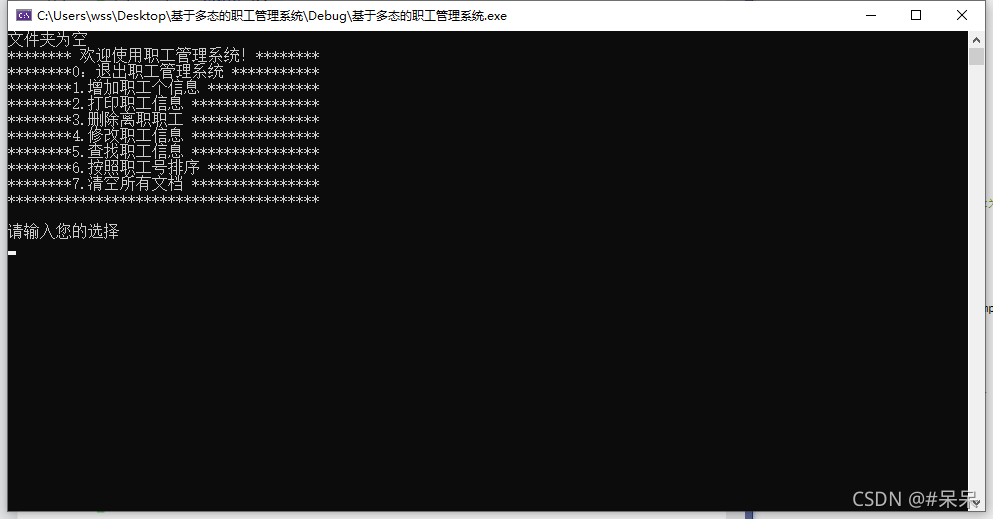
我一一列出来(头文件,cpp文件,txt文件) 按着这三个复制粘贴就可以用
boss.h
#pragma once
#include"worker.h"
#include<iostream>
#include<string>
using namespace std;
class boss :public worker {
public:
boss(int id, string name, int did);
virtual void showinfo() ;
virtual string getdepainfo() ;
};
employee.h
#pragma once
#include"worker.h"
#include<iostream>
#include<string>
using namespace std;
class Employee:public worker
{
public:
Employee(int id, string name,int did);
void showinfo();
string getdepainfo();
};
manager.h
#pragma once
#include<iostream>
#include<string>
#include"worker.h"
using namespace std;
class manager :public worker {
public:
manager(int id, string name, int did);
void showinfo() ;
string getdepainfo() ;
};
worker.h
#pragma once
#include<iostream>
#include<string>
using namespace std;
class worker {
public:
virtual void showinfo() = 0;
virtual string getdepainfo() = 0;
int m_id;
string m_name;
int m_depaid;
};
workerManager.h
#pragma once
#include<iostream>
#include"worker.h"
using namespace std;
class workermanager {
public:
workermanager();
void showmenu();
void exitsystem();
int m_empnum;
worker ** m_emparray;
void addemp();
void save();
void show();
bool m_fileisemp;
int getnum();
void init();
void delemp();
int isexist(int id);
void modify();
void search();
void sort();
void clean();
~workermanager();
};
接下来是.cpp文件
boss.h
#pragma once
#include"worker.h"
#include<iostream>
#include<string>
using namespace std;
#include"boss.h"
boss::boss(int id, string name, int did)
{
this->m_id = id;
this->m_name = name;
this->m_depaid = did;
}
void boss::showinfo()
{
cout << "职工编号为:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t职工岗位为:" << this->getdepainfo()
<< "\t岗位职责:管理公司" << endl;
}
string boss::getdepainfo()
{
return "老板";
}
employee.cpp
#pragma once;
#include<iostream>
#include<string>
using namespace std;
#include"employee.h"
Employee::Employee(int id, string name,int did)
{
this->m_id = id;
this->m_name = name;
this->m_depaid = did;
}
void Employee::showinfo()
{
cout << "职工编号为:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t职工岗位为:" << this->getdepainfo()
<< "\t岗位职责:完成经理分配的任务" << endl;
}
string Employee::getdepainfo()
{
return "员工";
}
manager.cpp
#include<iostream>
#pragma once
#include<string>
#include"worker.h"
#include"manager.h"
manager::manager(int id,string name,int did)
{
this->m_id = id;
this->m_name = name;
this->m_depaid = did;
}
void manager::showinfo()
{
cout << "职工编号为:" << this->m_id
<< "\t职工姓名:" << this->m_name
<< "\t职工岗位为:" << this->getdepainfo()
<< "\t岗位职责:完成老板分配的任务" << endl;
}
string manager::getdepainfo()
{
return "经理";
}
workerManager.cpp
#include"workerManger.h"
#include"boss.h"
#include"employee.h"
#include"manager.h"
#include<fstream>
#define FILENAME "EMPFILE.txt"
workermanager::workermanager()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
if (!ifs.is_open())
{
cout << "文件不存在" << endl;
this->m_empnum = 0;
this->m_emparray = NULL;
this->m_fileisemp = true;
ifs.close();
return;
}
char ch;
ifs >> ch;
if (ifs.eof())
{
cout << "文件夹为空" << endl;
this->m_empnum = 0;
this->m_emparray = NULL;
this->m_fileisemp = true;
ifs.close();
return;
}
int num = this->getnum();
cout << "职工人数为:" << num << endl;
this->m_empnum = num;
this->m_emparray = new worker * [this->m_empnum];
this->init();
for (int i = 0; i < this->m_empnum; i++)
{
cout << "职工编号为" << this->m_emparray[i]->m_id
<< "姓名为:" << this->m_emparray[i]->m_name
<< "部门编号:" << this->m_emparray[i]->m_depaid << endl;
}
}
void workermanager::delemp()
{
if (this->m_fileisemp)
{
cout << "文件不存在或者为空" << endl;
}
else {
cout << "请输入要删除的职工编号" << endl;
int id = 0;
cin >> id;
int index = this->isexist(id);
if (index != -1)
{
for (int i = index; i < this->m_empnum-1; i++)
{
this->m_emparray[i] = this->m_emparray[i + 1];
}
this->m_empnum--;
this->save();
cout << "删除成功" << endl;
}
else
cout << "未找到该职工" << endl;
}
system("pause");
system("cls");
}
void workermanager::modify()
{
if (this->m_fileisemp)
{
cout << "文件不存在或者为空" << endl;
}
cout << "请输入要修改职工的编号" << endl;
int id;
cin >> id;
int t = this->isexist(id);
if (t != -1)
{
delete this->m_emparray[t];
int newid = 0;
string newname = "";
int ds = 0;
cout << "查询到id为" << t << "号职工,请输入新职工号:" << endl;
cin >> newid;
cout << "请输入新姓名" << endl;
cin >> newname;
cout << "请输入岗位:" << endl << "1,普通员工\n2.经理\n3.老板\n";
cin >> ds;
worker* worker = NULL;
switch (ds)
{
case 1:worker = new Employee(newid, newname, ds);
break;
case 2:
worker = new manager(newid, newname, ds);
break;
case 3:worker = new boss(newid, newname, ds);
break;
default:
break;
}
this->m_emparray[t] = worker;
cout << "修改成功" << endl;
this->save();
}
else
cout << "查无此人" << endl;
system("pause");
system("cls");
}
int workermanager::isexist(int id)
{
int index = -1;
for (int i = 0; i < this->m_empnum; i++)
{
if (this->m_emparray[i]->m_id == id)
{
index = i;
break;
}
}
return index;
}
void workermanager::show()
{
if (this->m_fileisemp)
{
cout << "文件不存在或者记录为空" << endl;
}
else
{
for (int i = 0; i < m_empnum; i++)
{
this->m_emparray[i]->showinfo();
}
}
system("pause");
system("cls");
}
int workermanager::getnum()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id;
string name;
int did;
int num = 0;
while (ifs >> id && ifs >> name && ifs >> did)
{
num++;
}
return num;
}
void workermanager::showmenu()
{
cout << "******** 欢迎使用职工管理系统!********" << endl;
cout << "********0:退出职工管理系统 ***********" << endl;
cout << "********1.增加职工个信息 **************" << endl;
cout << "********2.打印职工信息 ****************" << endl;
cout << "********3.删除离职职工 ****************" << endl;
cout << "********4.修改职工信息 ****************" << endl;
cout << "********5.查找职工信息 ****************" << endl;
cout << "********6.按照职工号排序 **************" << endl;
cout << "********7.清空所有文档 ****************" << endl;
cout << "***************************************" << endl;
cout << endl;
}
void workermanager::exitsystem()
{
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);
}
void workermanager::addemp()
{
cout << "请输入添加职工人数:\n";
int num;
cin >> num;
if (num > 0)
{
int newsize = this->m_empnum + num;
worker **newspace= new worker* [newsize];
if (this->m_emparray != NULL)
{
for (int i = 0; i < this->m_empnum; i++)
{
newspace[i] = this->m_emparray[i];
}
}
for (int i = 0; i < num; i++)
{
int id;
string name;
int ds;
cout << "请输入第" << i + 1 << "个新职工编号:\n";
cin >> id;
cout << "请输入第" << i + 1 << "个新职工姓名:\n";
cin >> name;
cout << "请选择该职工岗位:\n";
cout << "1,普通员工" << endl;
cout << "2,经理" << endl;
cout << "3,老板" << endl;
cin >> ds;
worker* worker = NULL;
switch (ds)
{
case 1:
worker = new Employee(id, name, 1);
break;
case 2:
worker = new manager(id, name, 2);
break;
case 3:
worker = new boss(id, name, 3);
break;
default:
break;
}
newspace[this->m_empnum + i] = worker;
delete[]this->m_emparray;
this->m_emparray = newspace;
this->m_empnum = newsize;
this->m_fileisemp = false;
cout << "成功添加" << num << "个新职工" << endl;
this->save();
}
}
else
cout << "输入数据有误\n";
system("pause");
system("cls");
}
void workermanager::search()
{
if (this->m_fileisemp)
cout << "文件为空或者不存在" << endl;
int id;
int did;
cout << "请输入查询者的职工号\n";
cin >> id;
int t = this->isexist(id);
if (t != -1)
{
cout << "职工号为" << id << "的信息\n";
cout << "职工号为:" << this->m_emparray[t]->m_id << "职工名为:" << this->m_emparray[t]->m_name << "部门编号为:" << this->m_emparray[t]->m_depaid << endl;
}
else
cout << "查无此人\n";
}
void workermanager::save()
{
ofstream ofs;
ofs.open(FILENAME, ios::out);
for (int i = 0; i < this->m_empnum; i++)
{
ofs << this->m_emparray[i]->m_id << " "
<< this->m_emparray[i]->m_name << " "
<< this->m_emparray[i]->m_depaid << endl;
}
ofs.close();
}
void workermanager::sort()
{
if (this->m_fileisemp)
{
cout << "文件不存在或者为空,无法排序" << endl;
system("pause");
system("cls");
}
int id;
string name;
int did;
for (int i = 0; i < this->m_empnum; i++)
{
for (int j = 0; j < i; j++)
{
if (this->m_emparray[i]->m_id > this->m_emparray[j]->m_id)
{
id = this->m_emparray[j]->m_id;
name = this->m_emparray[j]->m_name;
did = this->m_emparray[j]->m_depaid;
this->m_emparray[j]->m_id = this->m_emparray[i]->m_id;
this->m_emparray[j]->m_name = this->m_emparray[i]->m_name;
this->m_emparray[j]->m_depaid = this->m_emparray[i]->m_depaid;
this->m_emparray[i]->m_id = id;
this->m_emparray[i]->m_name = name;
this->m_emparray[i]->m_depaid =did;
}
}
}
system("pause");
system("cls");
}
void workermanager::clean()
{
ofstream ofs(FILENAME, ios::trunc);
ofs.close();
if (this->m_emparray != NULL)
{
for (int i = 0; i < this->m_empnum; i++)
{
delete this->m_emparray[i];
this->m_emparray[i] = NULL;
}
delete[]this->m_emparray;
this->m_empnum = 0;
this->m_fileisemp = 1;
}
cout << "清空成功\n";
system("pause");
system("cls");
}
void workermanager::init()
{
ifstream ifs;
ifs.open(FILENAME, ios::in);
int id;
string name;
int did;
int index = 0;
while (ifs >> id && ifs >> name && ifs >> did)
{
worker* worker = NULL;
switch (did)
{
case 1:
worker = new Employee(id, name, did);
break;
case 2:
worker = new manager(id, name, did);
break;
case 3:
worker = new boss(id, name, did);
break;
default:
break;
}
this->m_emparray[index] = worker;
index++;
}
ifs.close();
}
workermanager::~workermanager()
{
if (this->m_emparray != NULL)
{
delete[] this->m_emparray;
this->m_emparray = NULL;
}
}
职工管理系统.cpp
#pragma once
#include<iostream>
#include"workerManger.h"
#include"worker.h"
#include"employee.h"
#include"manager.h"
#include"boss.h"
#include<string>
#include<fstream>
#define FILENAME "EMPFILE.txt"
using namespace std;
int main()
{
workermanager wss;
int choice = 0;
while (true)
{
wss.showmenu();
cout << "请输入您的选择" << endl;
cin >> choice;
switch (choice)
{
case 0:wss.exitsystem();
break;
case 1:
wss.addemp();
break;
case 2:
wss.show();
break;
case 3:
wss.delemp();
break;
case 4:
wss.modify();
break;
case 5:
wss.search();
break;
case 6:
wss.sort();
break;
case 7:
wss.clean();
break;
default:
system("cls");
break;
}
}
system("pause");
return 0;
}
.txt文件:
1 张三 2 2 李四 3 4 王五 1 把这三行数据复制粘贴到一个txt文件中(保存这个文件的时候用ANSI编码格式) 以上的文件都粘贴到一个项目中,就可以运行了。
|