libGDX游戏开发之Box2D(十四) libGDX系列,游戏开发有unity3D巴拉巴拉的,为啥还用java开发?因为我是Java程序员emm…国内用libgdx比较少,多数情况需要去官网和google找资料,相互学习的可以加我联系方式。 libgdx官方描述:
Box2D 是一个二维物理库。它是最流行的 2D 游戏物理库之一,并已移植到多种语言和许多不同的引擎,包括 libGDX。libGDX 中的 Box2D 实现是一个围绕 C++ 引擎的瘦 Java 包装器。
由此知道libgdx的Box2d底层是调用Box2d C++的,即时对它的封装,官网也描述了使用Box2D基本能与C++文档描述基本一致:https://box2d.org/ Box2d在libgdx的使用文档:https://github.com/libgdx/libgdx/wiki/Box2d 但是,实际用起来也有诸多描述不清不楚的,特别是在正常像素下物体重力现象不明显,需要对纹理进行缩小100++倍才有比较明显的物理效果;使用起来为以下步骤:
1、创建一个世界,有重力,里面放物体 2、使用Box2DDebugRenderer 这个渲染器能帮我们把Box2d的物体轮廓描绘出来,方便观察物体在哪里、多大等等,正式游戏中需要注释它。 3、使用:创建身体(Body):静态物体(地面、墙壁)、动态物体(玩家、怪物)、运动物体()
Box2DDebugRenderer
值得一提的是Box2DDebugRenderer 的效果如下: 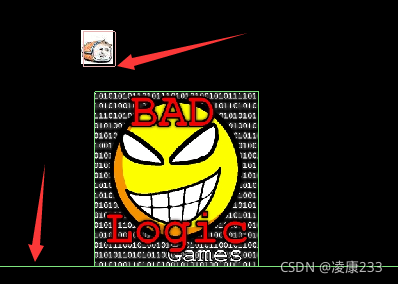 观察到线条与框框就是它的效果了。
1、编写一个简单demo
还是要提一下:在正常像素下物体重力现象不明显,需要对纹理进行缩小100++倍才有比较明显的物理效果 当然你也可以自己摸索,下面是我的demo效果: 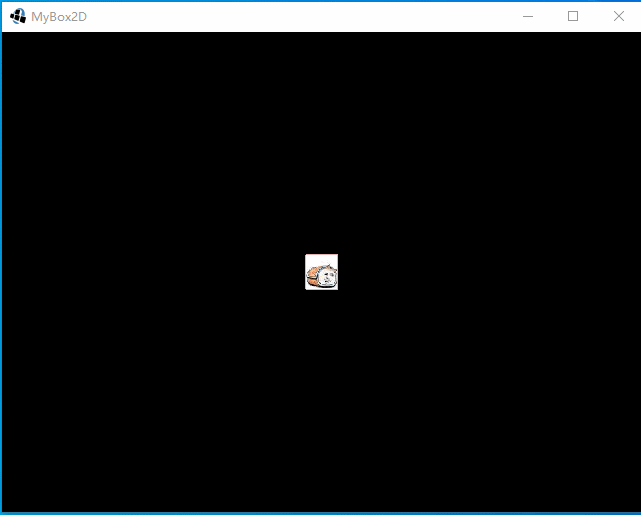 代码如下
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.Input;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureRegion;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.physics.box2d.*;
public class MyBox2D extends ApplicationAdapter {
private SpriteBatch batch;
private TextureRegion img, dog;
private OrthographicCamera camera;
private World world;
private Box2DDebugRenderer debugRenderer;
private Body dogBody;
private float reduce = 100;
private boolean isJump;
@Override
public void create() {
batch = new SpriteBatch();
camera = new OrthographicCamera();
camera.setToOrtho(false, Gdx.graphics.getWidth() / 64, Gdx.graphics.getHeight() / 64);
dog = new TextureRegion(new Texture("dog.jpg"), 50, 50);
img = new TextureRegion(new Texture("badlogic.jpg"), 256, 256);
world = new World(new Vector2(0, -10), true);
debugRenderer = new Box2DDebugRenderer();
BodyDef groundBodyDef = new BodyDef();
groundBodyDef.type = BodyDef.BodyType.StaticBody;
groundBodyDef.position.x = 0;
groundBodyDef.position.y = 0;
Body groundBody = world.createBody(groundBodyDef);
PolygonShape groundBox = new PolygonShape();
groundBox.setAsBox(Gdx.graphics.getWidth(), 10);
groundBody.createFixture(groundBox, 0);
BodyDef badlogicBodyDef = new BodyDef();
badlogicBodyDef.type = BodyDef.BodyType.StaticBody;
badlogicBodyDef.position.x = 10;
badlogicBodyDef.position.y = 10 + 1.28f;
Body badlogicBody = world.createBody(badlogicBodyDef);
PolygonShape badlogicBox = new PolygonShape();
badlogicBox.setAsBox(256 / 2 / reduce, 256 / 2 / reduce);
badlogicBody.createFixture(badlogicBox, 0);
BodyDef dogBodyDef = new BodyDef();
dogBodyDef.type = BodyDef.BodyType.DynamicBody;
dogBodyDef.position.x = 10;
dogBodyDef.position.y = 20;
dogBody = world.createBody(dogBodyDef);
PolygonShape dynamicBox = new PolygonShape();
dynamicBox.setAsBox(50 / 2 / reduce, 50 / 2 / reduce);
FixtureDef fixtureDef = new FixtureDef();
fixtureDef.shape = dynamicBox;
fixtureDef.restitution = 0.2f;
dogBody.createFixture(fixtureDef).setUserData(this);
groundBox.dispose();
dynamicBox.dispose();
}
@Override
public void render() {
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
Vector2 position = dogBody.getPosition();
camera.position.set(position.x, position.y, 0);
camera.update();
batch.setProjectionMatrix(camera.combined);
batch.begin();
batch.draw(img, 10 - 1.28f, 10, 0, 0, 256, 256, 0.01f, 0.01f, 0);
batch.draw(dog, position.x - 50 / 2 / reduce, position.y - 50 / 2 / reduce,
0, 0, 50, 50,
1 / reduce, 1 / reduce,
0
);
batch.end();
Vector2 linearVelocity = dogBody.getLinearVelocity();
if (Gdx.input.isKeyPressed(Input.Keys.D) && linearVelocity.x <= 2) {
dogBody.applyLinearImpulse(new Vector2(0.1f, 0), dogBody.getWorldCenter(), true);
}
if (Gdx.input.isKeyPressed(Input.Keys.A) && linearVelocity.x >= -2) {
dogBody.applyLinearImpulse(new Vector2(-0.1f, 0), dogBody.getWorldCenter(), true);
}
if (!isJump && Gdx.input.isKeyPressed(Input.Keys.W) && linearVelocity.y <= 4) {
dogBody.applyLinearImpulse(new Vector2(0, 4), dogBody.getWorldCenter(), true);
isJump = true;
}
if (linearVelocity.y == 0) {
isJump = false;
}
debugRenderer.render(world, camera.combined);
world.step(1 / 60f, 6, 2);
}
}
public class MyBox2DApplication {
public static void main(String[] args) {
LwjglApplicationConfiguration config = new LwjglApplicationConfiguration();
new LwjglApplication(new MyBox2D(), config);
}
}
|