可以实现记录移动的位置, 撤销和反撤销
先上图:
记录1右 记录2上 记录3上 丢弃3上 记录3左 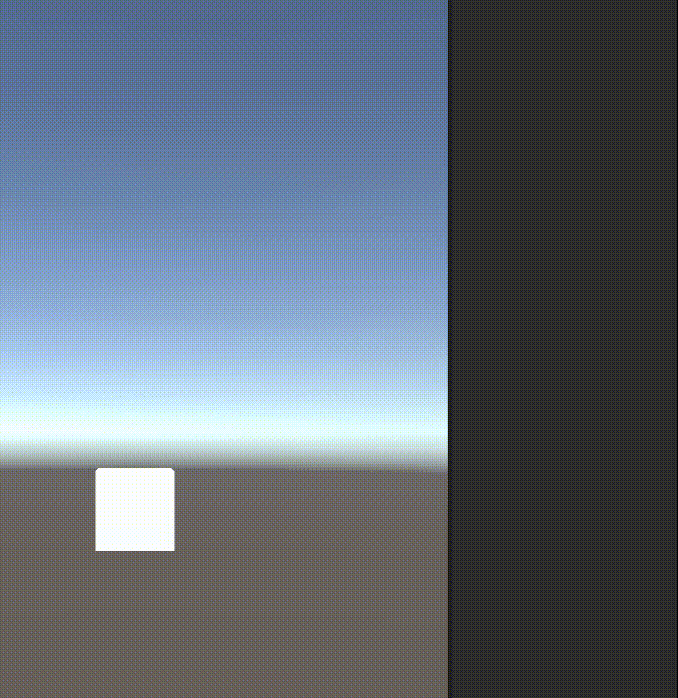
说思路:
每一步操作都是一个"命令" 用列表记录"命令"
"命令"包含新坐标和旧坐标 撤销, 即移动到旧坐标 反撤销, 即移动到新坐标
如果, 撤销后, 执行了新的操作, 则丢弃原始记录
贴代码:
挂在方块上就能运行
using System.Collections.Generic;
using UnityEngine;
public class Command
{
Vector3 _oldPos, _newPos;
Transform _tr;
public Command(Transform tr, Vector3 oldPos, Vector3 newPos)
{
_tr = tr;
_oldPos = oldPos;
_newPos = newPos;
}
public void Do()
{
_tr.position = _newPos;
}
public void UnDo()
{
_tr.position = _oldPos;
}
}
public class CommandPattern : MonoBehaviour
{
int curIndex = -1;
List<Command> commands = new List<Command>();
void AddCommand(Command com)
{
if (curIndex < commands.Count - 1)
{
for (int i = commands.Count - 1; i > curIndex; i--)
{
commands.RemoveAt(i);
}
print("丢弃已记录的操作");
}
com.Do();
commands.Add(com);
curIndex = commands.Count - 1;
}
void Update()
{
if (Input.GetKeyDown(KeyCode.W))
{
Command com = new Command(transform, transform.position, transform.position + Vector3.up);
AddCommand(com);
print("上");
}
else if (Input.GetKeyDown(KeyCode.S))
{
Command com = new Command(transform, transform.position, transform.position + Vector3.down);
AddCommand(com);
print("下");
}
else if (Input.GetKeyDown(KeyCode.A))
{
Command com = new Command(transform, transform.position, transform.position + Vector3.left);
AddCommand(com);
print("左");
}
else if (Input.GetKeyDown(KeyCode.D))
{
Command com = new Command(transform, transform.position, transform.position + Vector3.right);
AddCommand(com);
print("右");
}
else if (Input.GetKeyDown(KeyCode.Z))
{
if (curIndex >= 0)
{
commands[curIndex].UnDo();
curIndex--;
print("撤销");
}
else
{
print("已最旧, 无法再撤销啦");
}
}
else if (Input.GetKeyDown(KeyCode.Y))
{
if (curIndex < commands.Count - 1)
{
curIndex++;
commands[curIndex].Do();
print("反撤销");
}
else
{
print("已最新, 无法再反撤销啦");
}
}
}
}
|