【千锋合集】史上最全Unity3D全套教程|匠心之作_哔哩哔哩_bilibili
UI搭建
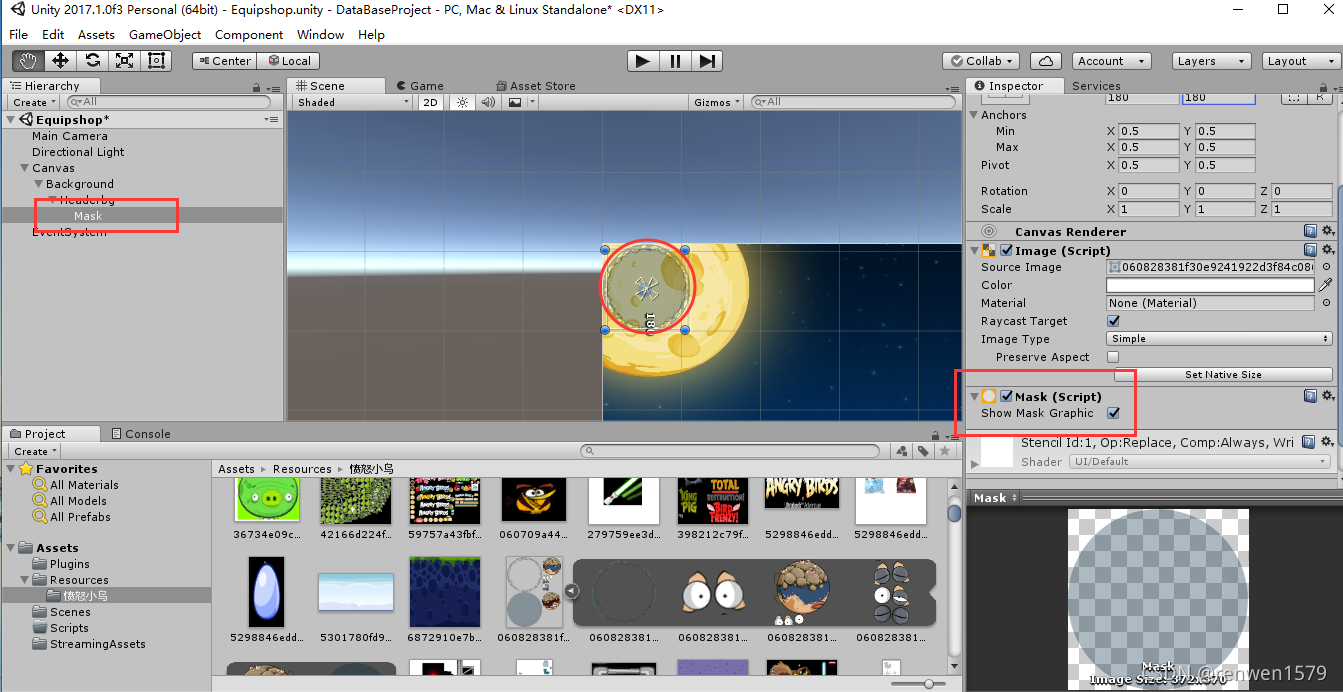
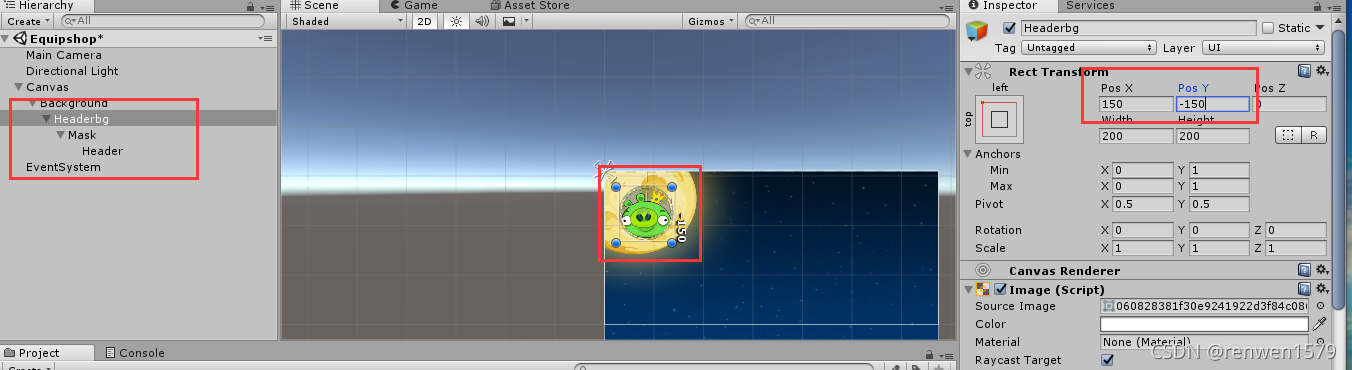
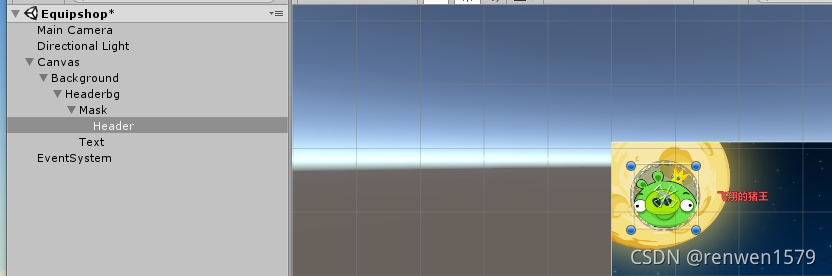
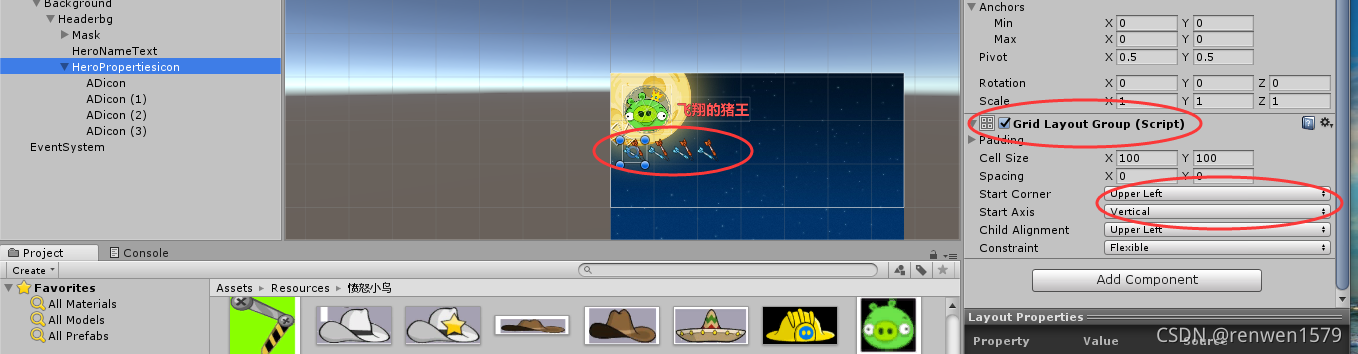
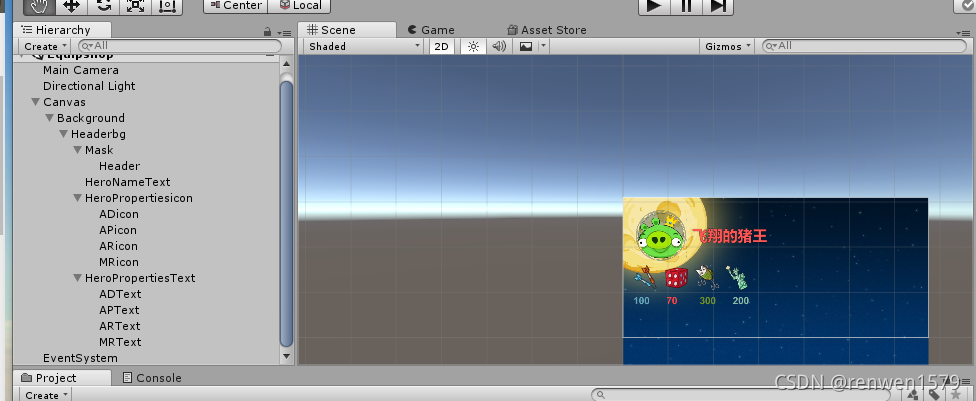
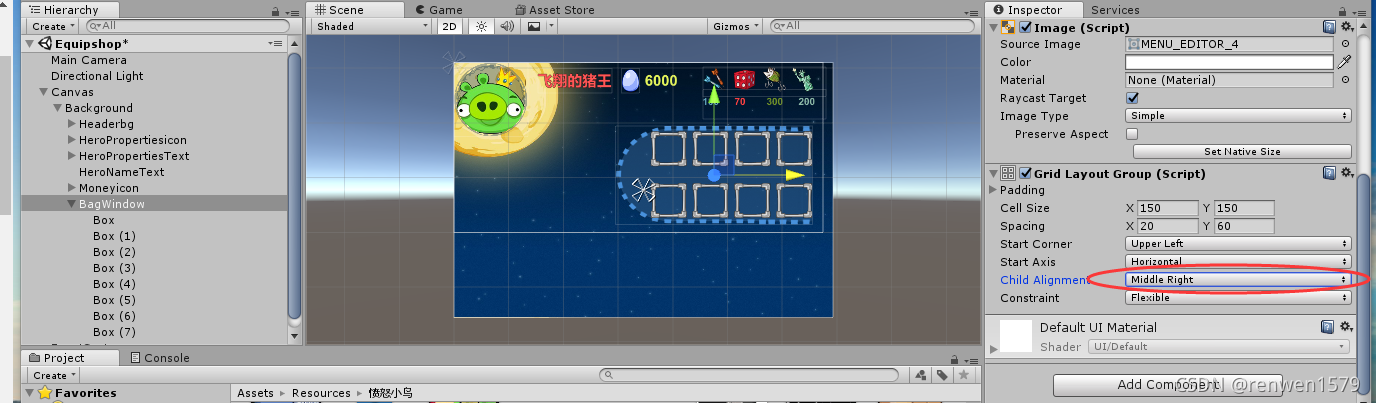
?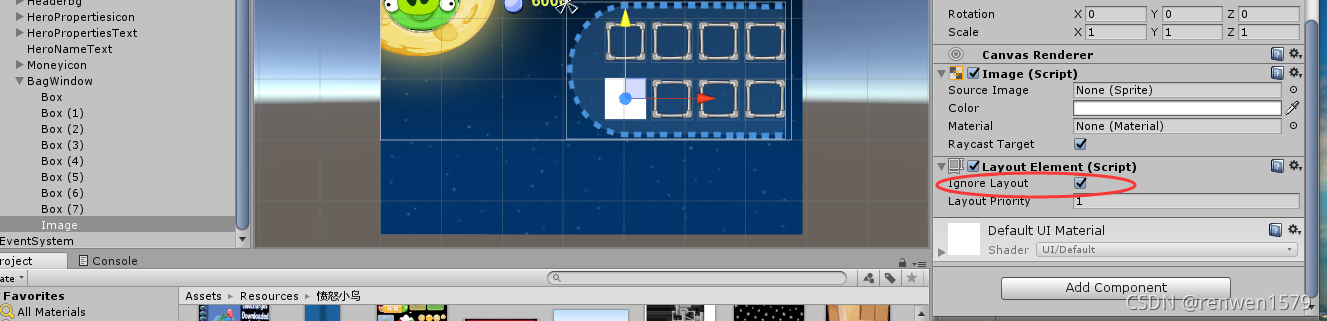
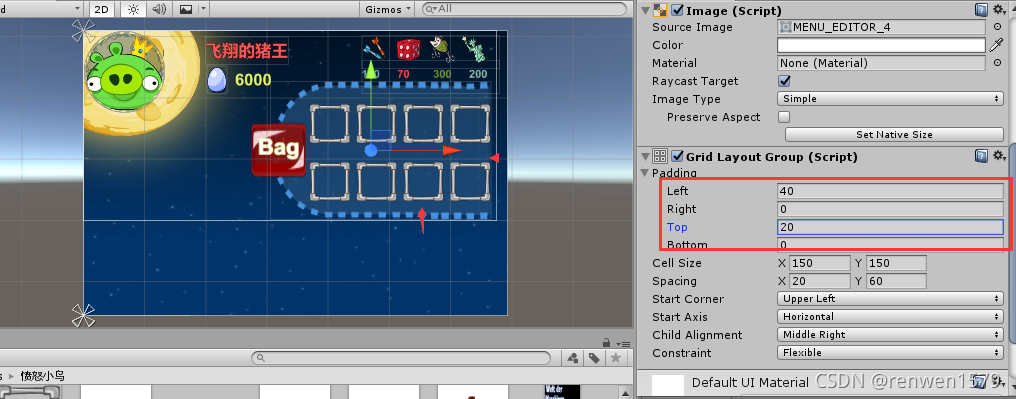
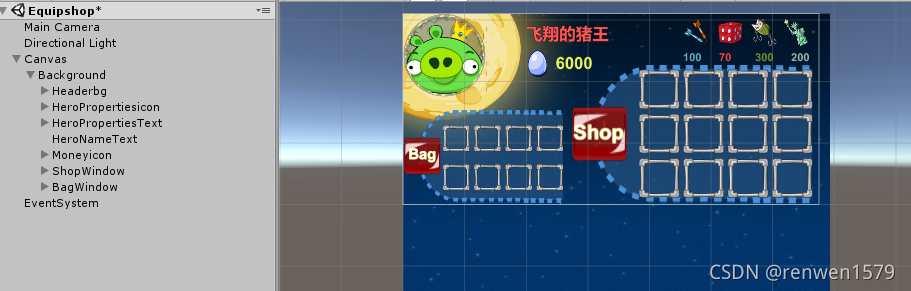
数据库配置
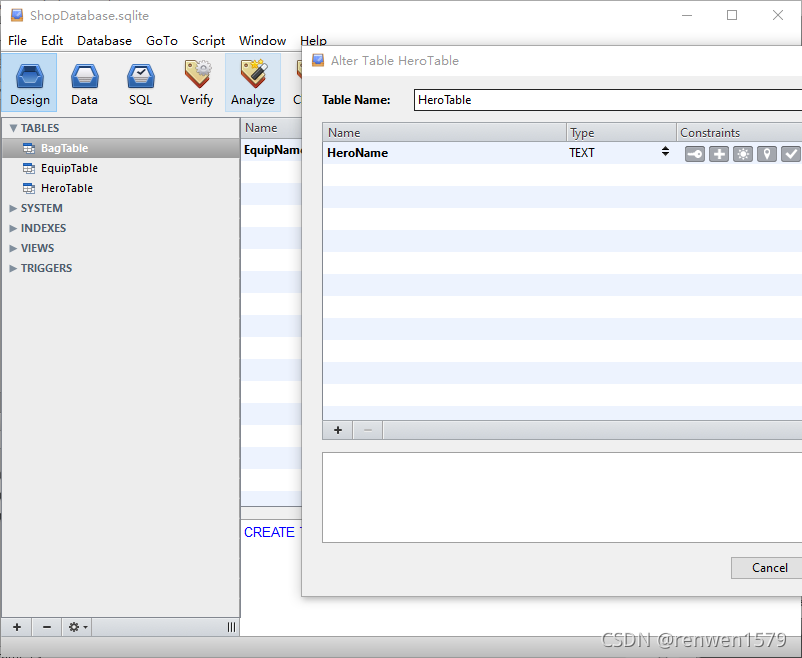
using System.Collections; using System.Collections.Generic; using UnityEngine; using Mono.Data.Sqlite;
public class SQLFrame{ ? ? #region SingleTon ? ? private static SQLFrame instance; ? ? public static SQLFrame GetInstance() { ? ? ? ? if (instance == null) {? ? ? ? ? instance = new SQLFrame(); ? ? ? ? } ? ? ? ? return instance; ? ? } ? ? private SQLFrame() {} //防止外界去new一个SQLFrame ? ? #endregion ? ? //连接字符串 ? ? private string conStr; ? ? private SqliteConnection con; ? ? private SqliteCommand command; ? ? private SqliteDataReader reader; ? ? /// <summary> ? ? /// 打开数据库 ? ? /// </summary> ? ? /// <param name="databaseName"></param> ? ? public void OpenDatabase(string databaseName) { ? ? ? ? //如果名称中不包含后缀,自动添加 ? ? ? ? if (!databaseName.EndsWith(".sqlite")) { ? ? ? ? ? ? databaseName += ".sqlite"; ? ? ? ? } #if UNITY_EDITOR ? ? ? ? conStr = "Data Source =" + Application.streamingAssetsPath +"/" +databaseName; #endif ? ? ? ? con = new SqliteConnection(conStr); ? ? ? ? //打开连接 ? ? ? ? con.Open(); ? ? ? ? //创建指令对象 ? ? ? ? command = con.CreateCommand(); ? ? } ? ? /// <summary> ? ? /// 关闭数据库 ? ? /// </summary> ? ? public void CloseDatabase() { ? ? ? ? if (reader != null) ? ? ? ? { ? ? ? ? ? ? reader.Close(); ? ? ? ? ? ? reader = null; ? ? ? ? } ? ? ? ? if (command != null) ? ? ? ? { ? ? ? ? ? ? command.Dispose(); ? ? ? ? ? ? command = null; ? ? ? ? } ? ? ? ? if (con != null) ? ? ? ? { ? ? ? ? ? ? con.Close(); ? ? ? ? ? ? con = null; ? ? ? ? } ? ? } ? ? /// <summary> ? ? /// 执行非查询类SQL语句 ? ? /// </summary> ? ? /// <param name="query"></param> ? ? public int DontSelect(string query) { ? ? ? ? //赋值SQL语句 ? ? ? ? command.CommandText = query; ? ? ? ? //执行SQL语句 ? ? ? ? return command.ExecuteNonQuery(); ? ? } ? ? public int Insert(string query) { ? ? ? ? return DontSelect(query); ? ? } ? ? public int Update(string query) ? ? { ? ? ? ? return DontSelect(query); ? ? } ? ? public int Delete(string query) ? ? { ? ? ? ? return DontSelect(query); ? ? } ? ? /// <summary> ? ? /// 查询单个数据 ? ? /// </summary> ? ? /// <param name="query"></param> ? ? /// <returns></returns> ? ? public object SelectSingleData(string query) { ? ? ? ? //赋值SQL语句 ? ? ? ? command.CommandText = query; ? ? ? ? //执行SQL语句,返回结果 ? ? ? ? return command.ExecuteScalar(); ? ? } ? ? /// <summary> ? ? /// 查询多个数据 ? ? /// </summary> ? ? /// <param name="query"></param> ? ? public List<ArrayList> SelectMultipleData(string query) { ? ? ? ? //赋值SQL语句 ? ? ? ? command.CommandText = query; ? ? ? ? //执行 ? ? ? ? reader = command.ExecuteReader(); ? ? ? ? //一行多列:ArrayList ? ? ? ? //多行多列:List<Arraylist> ? ? ? ? //实例化结果对象 ? ? ? ? List<ArrayList> result = new List<ArrayList>(); ? ? ? ? while (reader.Read()) { ? ? ? ? ? ? //声明一个ArrayList存储改行的所有数据 ? ? ? ? ? ? ArrayList currentRow = new ArrayList(); ? ? ? ? ? ? //遍历改行所有的列 ? ? ? ? ? ? for (int i = 0; i < reader.FieldCount; i++) { ? ? ? ? ? ? ? ? //将当前列的数据添加到集合 ? ? ? ? ? ? ? ? currentRow.Add(reader.GetValue(i)); ? ? ? ? ? ? } ? ? ? ? ? ? //将当前行的数据放到List里面 ? ? ? ? ? ? result.Add(currentRow); ? ? ? ? } ? ? ? ? //关闭读取器 ? ? ? ? reader.Close(); ? ? ? ? //返回结果 ? ? ? ? return result; ? ? } }
数据库代码
using System.Collections; using System.Collections.Generic; using UnityEngine; using System;
public class EquipShopFrame : SQLFrame { ? ? #region SingleTon ? ? private static EquipShopFrame instance; ? ? public static new EquipShopFrame GetInstance() //盖掉父类方法 ? ? { ? ? ? ? if (instance == null) ? ? ? ? { ? ? ? ? ? ? instance = new EquipShopFrame(); ? ? ? ? } ? ? ? ? return instance; ? ? } ? ? private EquipShopFrame() { } //防止外界去new一个SQLFrame ? ? #endregion ? ? //SQl语句 ? ? //private string sqlQuery; ? ? private string query;
? ? /// <summary> ? ? /// 买装备流程 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? public void BuyEquip(string equipName) { ? ? ? ? #region 买装备流程 ? ? ? ? //1、查看要买的装备价值多少钱 ? ? ? ? //2、查看当前召唤师有多少钱 ? ? ? ? //3、判断钱够不够买装备 ? ? ? ? //4、如果不够,拒绝购买 ? ? ? ? //5、如果够,购买装备 ? ? ? ? //5.1、获取装备属性加成 ? ? ? ? //5.2、获取英雄当前属性值 ? ? ? ? //5.3、属性加成与当前英雄的属性相加 ? ? ? ? //5.4、更新到英雄表格中 ? ? ? ? //5.5、获取英雄的装备存储字符串 ? ? ? ? //5.6、拼接字符串,形成新的英雄装备存储记录 ? ? ? ? //5.7、将新的英雄装备信息存储到数据库 ? ? ? ? //5.8、将装备的花费扣除,更新英雄的金钱 ? ? ? ? #endregion ? ? } ? ? public void SellEquip(string equipName) {
? ? } ? ? /// <summary> ? ? /// 设置英雄属性信息 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <param name="heroEquips"></param> ? ? public void SetHeroEquips(string heroName, string heroEquips) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroEquips='" + heroEquips + "'Where HeroName='"+heroName+"'"; ? ? ? ? //执行更新操作 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// 获取英雄装备信息 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? public string GetHeroEquips(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select HeroEquips From HeroTable Where HeroName='"+heroName+"'"; ? ? ? ? //执行 ? ? ? ? object result=SelectSingleData(query); ? ? ? ? if (result==null) { ? ? ? ? ? ? return null; ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return result.ToString(); ? ? }
? ? /// <summary> ? ? /// 设置英雄的属性信息 ? ? /// </summary> ? ? /// <param name="properties"></param> ? ? private void SetHeroProperties(string heroName,int[] properties) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroAD="+properties[0] ? ? ? ? ? ? +",HeroAP="+properties[1]+",HeroAR="+properties[2]+",HeroSR="+properties[3]+" Where HeroName'" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// 获取英雄此时的属性值 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <returns></returns> ? ? public int[] GetHeroProperties(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query= "Select * From HeroTable Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? List<ArrayList> result=SelectMultipleData(query); ? ? ? ? //实例化属性数组 ? ? ? ? int[] properties = new int[4]; ? ? ? ? //循环获取值 ? ? ? ? for (int i = 0; i < properties.Length; i++) { ? ? ? ? ? ? //转换数据并存储 ? ? ? ? ? ? properties[i] = Convert.ToInt32(result[0][i + 2]);? ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return properties; ? ? }
? ? /// <summary> ? ? /// 获取当前装备的属性加成 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? /// <returns></returns> ? ? public int[] GetEquipProperties(string equipName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select * From ShopTable Where EquipName='" + equipName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? List<ArrayList> result = SelectMultipleData(query); ? ? ? ? //实例化属性数组 ? ? ? ? int[] properties = new int[4]; ? ? ? ? //遍历赋值 ? ? ? ? for (int i = 0; i < properties.Length; i++) { ? ? ? ? ? ? //转换数据并存储 ? ? ? ? ? ? properties[i] = System.Convert.ToInt32(result[0][i + 2]); ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return properties; ? ? }
? ? /// <summary> ? ? /// 获取英雄剩余金钱 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <returns></returns> ? ? public int GetHeroMoney(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select HeroMoney From HeroTable Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? object money = SelectSingleData(query); ? ? ? ? //返回整型结果 ? ? ? ? return System.Convert.ToInt32(money); ? ? } ? ? /// <summary> ? ? /// 设置英雄金钱 ? ? /// </summary> ? ? public void SetHeroMoney(string heroName,int heroMoney) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroMoney="+ heroMoney.ToString()+ "Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// 获取某个装备值多少钱 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? /// <returns></returns> ? ? public int GetEquipMoney(string equipName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select EquipMoney From ShopTable Where EquipName='"+equipName+"'"; ? ? ? ? //执行SQL语句 ? ? ? ? object money=SelectSingleData(query); ? ? ? ? //返回整型结果 ? ? ? ? return System.Convert.ToInt32(money); ? ? } }
using System.Collections; using System.Collections.Generic; using UnityEngine; using System;
public class EquipShopFrame : SQLFrame { ? ? #region SingleTon ? ? private static EquipShopFrame instance; ? ? public static new EquipShopFrame GetInstance() //盖掉父类方法 ? ? { ? ? ? ? if (instance == null) ? ? ? ? { ? ? ? ? ? ? instance = new EquipShopFrame(); ? ? ? ? } ? ? ? ? return instance; ? ? } ? ? private EquipShopFrame() { } //防止外界去new一个SQLFrame ? ? #endregion ? ? //SQl语句 ? ? //private string sqlQuery; ? ? private string query;
? ? /// <summary> ? ? /// 买装备流程 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? public void BuyEquip(string equipName,string heroName) { ? ? ? ? #region 买装备流程 ? ? ? ? //1、查看要买的装备价值多少钱 ? ? ? ? int equipMoney = GetEquipMoney(equipName); ? ? ? ? //2、查看当前召唤师有多少钱 ? ? ? ? int heroMoney = GetHeroMoney(heroName); ? ? ? ? //3、判断钱够不够买装备 ? ? ? ? if (equipMoney > heroMoney) { ? ? ? ? ? ? //4、如果不够,拒绝购买 ? ? ? ? ? ? Debug.Log("余额不足,无法购买!"); ? ? ? ? ? ? return; ? ? ? ? }
? ? ? ? //5、如果够,购买装备 ? ? ? ? //5.1、获取装备属性加成 ? ? ? ? int[] equipProperties = GetEquipProperties(equipName); ? ? ? ? //5.2、获取英雄当前属性值 ? ? ? ? int[] heroProperties = GetHeroProperties(heroName); ? ? ? ? //5.3、属性加成与当前英雄的属性相加 ? ? ? ? int[] newHeroProperties=PropertiesOpration(heroProperties,equipProperties,1); ? ? ? ? //5.4、更新到英雄表格中 ? ? ? ? SetHeroProperties(heroName,newHeroProperties); ? ? ? ? //5.5、获取英雄的装备存储字符串 ? ? ? ? string equips=GetHeroEquips(heroName); ? ? ? ? //5.6、拼接字符串,形成新的英雄装备存储记录 ? ? ? ? equips += equipName + "|"; ? ? ? ? //5.7、将新的英雄装备信息存储到数据库 ? ? ? ? SetHeroEquips(heroName, equips); ? ? ? ? //5.8、将装备的花费扣除,更新英雄的金钱 ? ? ? ? SetHeroMoney(heroName,heroMoney - equipMoney); ? ? ? ? #endregion ? ? } ? ? public void SellEquip(string equipName, string heroName) { ? ? ? ? //1、查看要买的装备价值多少钱 ? ? ? ? int equipMoney = GetEquipMoney(equipName); ? ? ? ? //2、查看当前召唤师有多少钱 ? ? ? ? int heroMoney = GetHeroMoney(heroName); ? ? ? ? //3、扣钱 ? ? ? ? heroMoney += equipMoney / 2; ? ? ? ? //4、英雄的money更新到数据库 ? ? ? ? SetHeroMoney(heroName, heroMoney); ? ? ? ? //5、获取装备属性信息 ? ? ? ? int[] equipProperties = GetEquipProperties(equipName); ? ? ? ? //6、获取英雄属性信息 ? ? ? ? int[] heroProperties = GetHeroProperties(heroName); ? ? ? ? //7、英雄属性中移除该装备的属性加成 ? ? ? ? int[] newHeroProperties=PropertiesOpration(heroProperties, equipProperties, 2); ? ? ? ? //8、更新英雄属性到数据库 ? ? ? ? SetHeroProperties(heroName, newHeroProperties); ? ? ? ? //9、获取英雄装备字符串 ? ? ? ? string equips = GetHeroEquips(heroName); ? ? ? ? //10、获取装备在字符串中的位置 ? ? ? ? int equipIndex= equips.LastIndexOf(equipName); ? ? ? ? //11、移除装备字符串 ? ? ? ? equips=equips.Remove(equipIndex, equipName.Length+1); ? ? ? ? //12、更新数据库 ? ? ? ? SetHeroEquips(heroName, equips);
? ? } ? ? /// <summary> ? ? /// 设置英雄属性信息 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <param name="heroEquips"></param> ? ? public void SetHeroEquips(string heroName, string heroEquips) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroEquips='" + heroEquips + "'Where HeroName='"+heroName+"'"; ? ? ? ? //执行更新操作 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// Propertieses the opration ? ? /// </summary> ? ? /// <param name="heroPpt"></param> ? ? /// <param name="equipPpt"></param> ? ? /// <param name="oprationID">1 for Add, 2 for Remove.</param> ? ? /// <returns></returns> ? ? public int[] PropertiesOpration(int[] heroPpt, int[] equipPpt,int oprationID) { ? ? ? ? //实例化结果数组 ? ? ? ? int[] result = new int[heroPpt.Length]; ? ? ? ? if (oprationID == 1) { ? ? ? ? ? ? for (int i = 0; i < result.Length; i++) { ? ? ? ? ? ? ? ? //属性相加 ? ? ? ? ? ? ? ? result[i] = heroPpt[i] + equipPpt[i]; ? ? ? ? ? ? } ? ? ? ? } ? ? ? ? else if(oprationID==2) { ? ? ? ? ? ? for (int i = 0; i < result.Length; i++) ? ? ? ? ? ? { ? ? ? ? ? ? ? ? //移除装备属性加成 ? ? ? ? ? ? ? ? result[i] = heroPpt[i] - equipPpt[i]; ? ? ? ? ? ? } ? ? ? ? } ? ? ? ? return result; ? ? }
? ? /// <summary> ? ? /// 获取英雄装备信息 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? public string GetHeroEquips(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select HeroEquips From HeroTable Where HeroName='"+heroName+"'"; ? ? ? ? //执行 ? ? ? ? object result=SelectSingleData(query); ? ? ? ? if (result==null) { ? ? ? ? ? ? return null; ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return result.ToString(); ? ? }
? ? /// <summary> ? ? /// 设置英雄的属性信息 ? ? /// </summary> ? ? /// <param name="properties"></param> ? ? private void SetHeroProperties(string heroName,int[] properties) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroAD="+properties[0] ? ? ? ? ? ? +",HeroAP="+properties[1]+",HeroAR="+properties[2]+",HeroSR="+properties[3]+" Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// 获取英雄此时的属性值 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <returns></returns> ? ? public int[] GetHeroProperties(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query= "Select * From HeroTable Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? List<ArrayList> result=SelectMultipleData(query); ? ? ? ? //实例化属性数组 ? ? ? ? int[] properties = new int[4]; ? ? ? ? //循环获取值 ? ? ? ? for (int i = 0; i < properties.Length; i++) { ? ? ? ? ? ? //转换数据并存储 ? ? ? ? ? ? properties[i] = Convert.ToInt32(result[0][i + 2]);? ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return properties; ? ? }
? ? /// <summary> ? ? /// 获取当前装备的属性加成 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? /// <returns></returns> ? ? public int[] GetEquipProperties(string equipName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select * From ShopTable Where EquipName='" + equipName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? List<ArrayList> result = SelectMultipleData(query); ? ? ? ? //实例化属性数组 ? ? ? ? int[] properties = new int[4]; ? ? ? ? //遍历赋值 ? ? ? ? for (int i = 0; i < properties.Length; i++) { ? ? ? ? ? ? //转换数据并存储 ? ? ? ? ? ? properties[i] = System.Convert.ToInt32(result[0][i + 2]); ? ? ? ? } ? ? ? ? //返回结果 ? ? ? ? return properties; ? ? }
? ? /// <summary> ? ? /// 获取英雄剩余金钱 ? ? /// </summary> ? ? /// <param name="heroName"></param> ? ? /// <returns></returns> ? ? public int GetHeroMoney(string heroName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select HeroMoney From HeroTable Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? object money = SelectSingleData(query); ? ? ? ? //返回整型结果 ? ? ? ? return System.Convert.ToInt32(money); ? ? } ? ? /// <summary> ? ? /// 设置英雄金钱 ? ? /// </summary> ? ? public void SetHeroMoney(string heroName,int heroMoney) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Update HeroTable Set HeroMoney="+ heroMoney.ToString()+ " Where HeroName='" + heroName + "'"; ? ? ? ? //执行SQL语句 ? ? ? ? Update(query); ? ? } ? ? /// <summary> ? ? /// 获取某个装备值多少钱 ? ? /// </summary> ? ? /// <param name="equipName"></param> ? ? /// <returns></returns> ? ? public int GetEquipMoney(string equipName) { ? ? ? ? //编写SQL语句 ? ? ? ? query = "Select EquipMoney From ShopTable Where EquipName='"+equipName+"'"; ? ? ? ? //执行SQL语句 ? ? ? ? object money=SelectSingleData(query); ? ? ? ? //返回整型结果 ? ? ? ? return System.Convert.ToInt32(money); ? ? } }
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI;
public class ShopView : MonoBehaviour { ? ? #region UI Component ? ? //商店装备的预设体 ? ? private GameObject shopEquipPrefab; ? ? //商店窗口 ? ? private Transform shopWindow;
? ? #endregion
? ? //装备商店单例 ? ? EquipShopFrame eFrame; ? ? private void Awake() ? ? { ? ? ? ? eFrame = EquipShopFrame.GetInstance(); ? ? ? ? ? ? ? ? UIComponentInit(); ? ? } ? ? private void Start() ? ? { ? ? ? ? //打开数据库 ? ? ? ? eFrame.OpenDatabase("ShopDatabase"); ? ? ? ? //初始化商店 ? ? ? ? ShopInit(); ? ? } ? ? /// <summary> ? ? /// UI组件初始化 ? ? /// </summary> ? ? private void UIComponentInit() { ? ? ? ? shopWindow = transform.Find("ShopWindow"); ? ? ? ? shopEquipPrefab = Resources.Load<GameObject>("Prefabs/ShopEquip"); ? ? }
? ? /// <summary> ? ? /// 商品初始化 ? ? /// </summary> ? ? private void ShopInit() { ? ? ? ? //获取商店所有装备的名称 ? ? ? ? ?string[] equips=eFrame.GetShopEquips(); ? ? ? ? //遍历生成所有商店装备 ? ? ? ? for (int i=0;i<equips.Length;i++) { ? ? ? ? ? ? //生成装备对象 ? ? ? ? ? ? GameObject crtEquip=Instantiate(shopEquipPrefab); ? ? ? ? ? ? //找到存放装备图片的格子 ? ? ? ? ? ? Transform box=shopWindow.GetChild(i); ? ? ? ? ? ? //设置为格子的子对象 ? ? ? ? ? ? crtEquip.transform.SetParent(box); ? ? ? ? ? ? //设置本地坐标 ? ? ? ? ? ? crtEquip.transform.localPosition = Vector3.zero; ? ? ? ? ? ? //设置缩放 ? ? ? ? ? ? crtEquip.transform.localScale = Vector3.one; ? ? ? ? ? ? //获取装备图片【Sprite】 ? ? ? ? ? ? Sprite equipSpr=Resources.Load<Sprite>("Textures/"+equips[i]); ? ? ? ? ? ? //更改装备图片 ? ? ? ? ? ? crtEquip.GetComponent<Image>().sprite = equipSpr; ? ? ? ? }
? ? } ? ? private void OnApplicationQuit() ? ? { ? ? ? ? //关闭数据库 ? ? ? ? eFrame.CloseDatabase(); ? ? } }
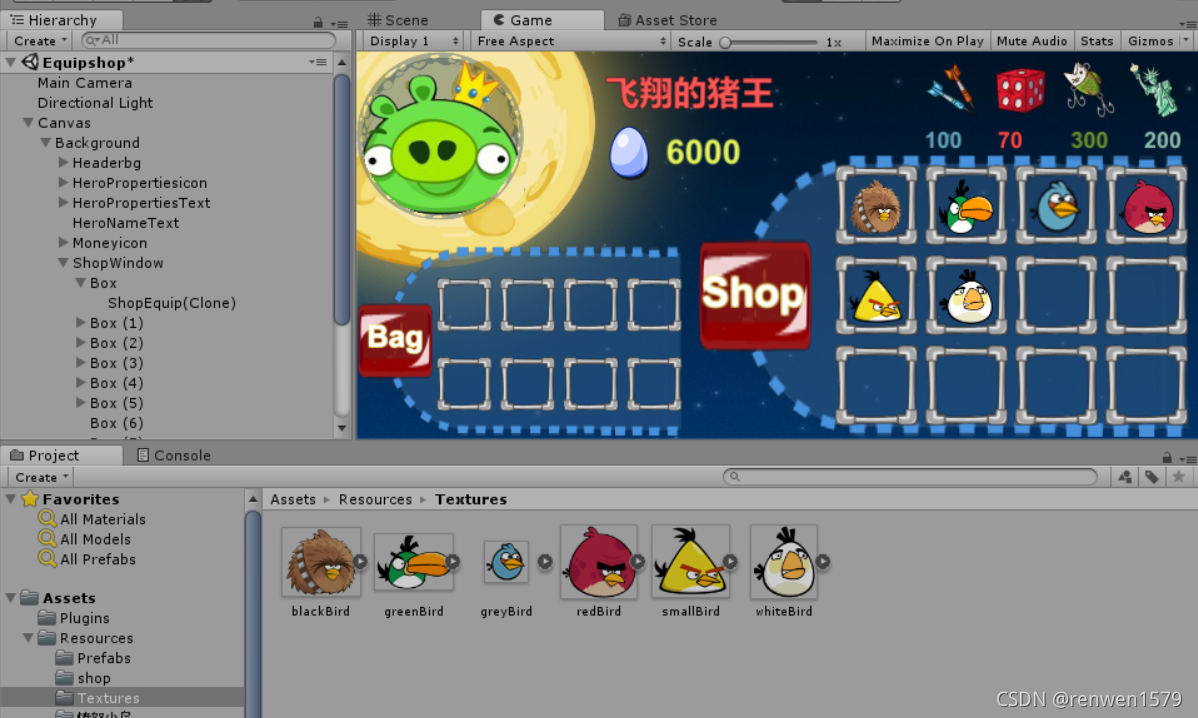
?
|