需求:通过类型和名字在某个物体里面找到满足这个条件的物体。 原因:unity原有的transform类提供的查找方法只能查找第一层级的子物体,想要找第二三层级的就比较麻烦。所以就只能自己拓展一个方法出来查找了。
举例:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public static class TransformHelper
{
public static T FindDeepTransform<T>(this Transform trans, string targetName) where T : Component
{
Transform child = trans.Find(targetName);
T t = null;
if (child != null)
{
t = child.GetComponent<T>();
return t;
}
for (int i = 0; i < trans.childCount; i++)
{
Transform parent = FindDeepTransform<Transform>(trans.GetChild(i), targetName);
if (parent != null)
{
t = parent.GetComponent<T>();
return t;
}
}
return t;
}
public static List<T> FindDeepTransforms<T>(this Transform trans, string targetName) where T : Component
{
List<T> t = new List<T>();
Find(trans, targetName, ref t);
return t;
}
private static void Find<T>(Transform trans, string targetName, ref List<T> t) where T : Component
{
int number = trans.childCount;
while (number > 0)
{
for (int i = 0; i < trans.childCount; i++)
{
if (trans.GetChild(i).name == targetName)
{
if (trans.GetComponent<T>())
t.Add(trans.GetChild(i).GetComponent<T>());
}
if (trans.GetChild(i).childCount > 0) Find<T>(trans.GetChild(i), targetName, ref t);
number--;
}
}
}
}
public List<Transform> obj = new List<Transform>();
void Start()
{
obj = transform.FindDeepTransforms<Transform>("Cube");
}
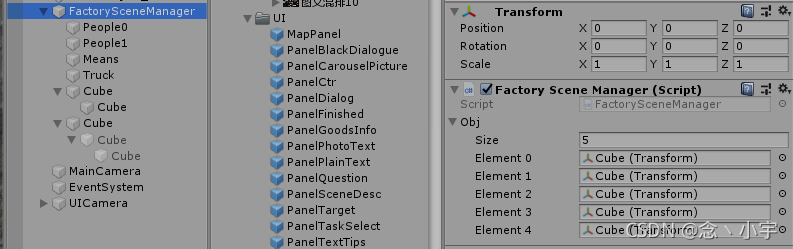
里面提供了两个拓展方法,为了方便查找单个或者多个物体(不管是否激活都能找到)
|