Roll A Ball 简单案例记录。以后还有其他不同类型、难易程度不同游戏案例技术点文章也将记录在《Unity游戏案例学习》博文专栏下。
一、主要脚本
1.小球控制脚本(移动及拾取物体得分检测)
using UnityEngine;
using UnityEngine.UI;
public class PlayerControlller : MonoBehaviour
{
private Rigidbody rb;
public float playerSpeed = 5f;
int score = 0;
public Text text;
public GameObject winText;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
PlayerMoveAddForce(h, v);
}
private void PlayerMovePosition(float h,float v)
{
Vector3 dir = new Vector3(h, 0, v);
dir = transform.position + dir.normalized *playerSpeed* Time.deltaTime;
rb.MovePosition(dir);
}
private void PlayerMoveAddForce(float h, float v)
{
Vector3 dir = new Vector3(h, 0, v);
rb.AddForce(dir * playerSpeed);
}
private void OnTriggerEnter(Collider other)
{
if (other.tag == "PickUp")
{
score++;
text.text = score.ToString();
if (score == 10)
{
winText.SetActive(true);
}
Destroy(other.gameObject);
}
}
}
2.摄像机跟随主角移动脚本
using UnityEngine;
public class FollowTarget : MonoBehaviour
{
public Transform playerTranform;
Vector3 offset;
void Start()
{
offset= transform.position - playerTranform.position;
}
void Update()
{
transform.position = playerTranform.position + offset;
}
}
3.拾取物自身旋转脚本
using UnityEngine;
public class PickUp : MonoBehaviour
{
void Update()
{
transform.Rotate(new Vector3(0, 1, 0) * Time.deltaTime * 60);
}
}
二、工程层级
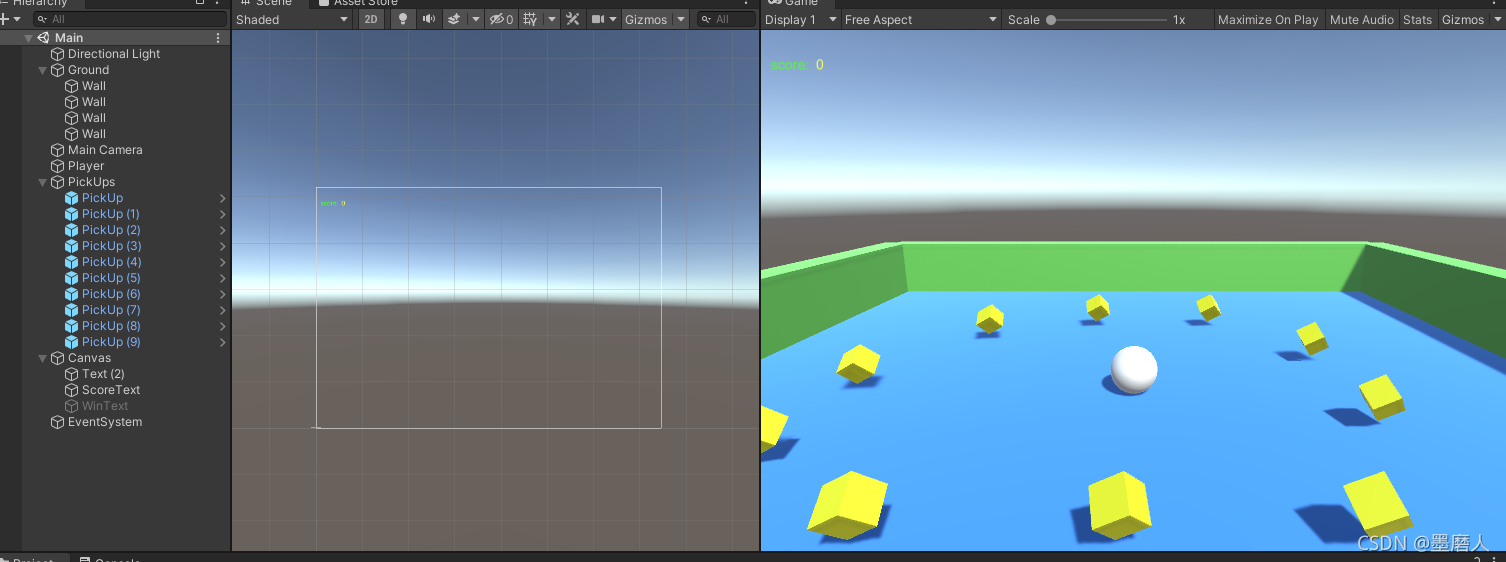
|