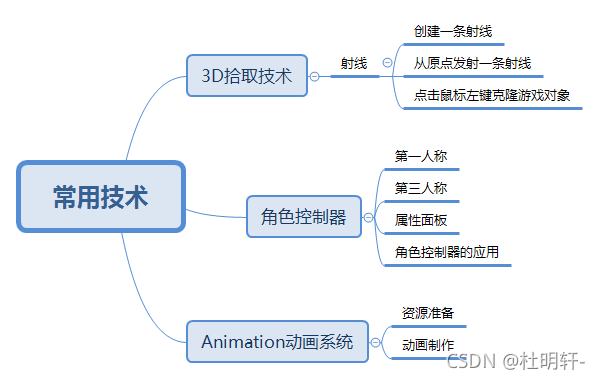
一、射线
要想在游戏中发射一条射线,必须要有两个元素,一个起始点,一个方向。 Ray.origin:射线起点 Ray.direction:射线的方向 创建一条射线的方法: Ray (origin : Vector3, direction : Vector3) Origin是射线的起点,direction是射线的方向
Debug.DrawLine(transform.position, transform.position + transform.forward, Color.red);
Debug.DrawRay(transform.position, transform.forward, Color.green);
从原点发射一条射线
void Update ()
{
Ray ray=new Ray(Vector3.zero,transform.position);
RaycastHit hit;
Physics.Raycast(ray,out hit,100);
Debug.DrawLine(ray.origin,hit.point);
}
点击鼠标左键克隆游戏对象
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
Instantiate(cube,hit.point,Quaternion.identity);
}
}
二、角色控制器
第一人称:在第一人称视角游戏中,整个游戏视图好比主角的眼睛,游戏画面中的一切好像是从自己眼睛看到的一样
第三人称:游戏场景中包含主角对象和摄像机对象,主角移动后,摄像机永远跟着主角移动,所以在Game视图中可以看出主角当前的移动方向
属性面板 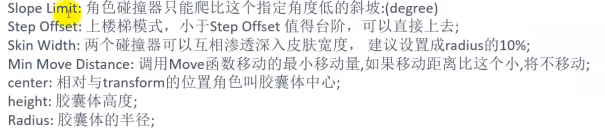 角色控制器的应用
通过键盘控制角色的移动 1.为角色添加CharacterController 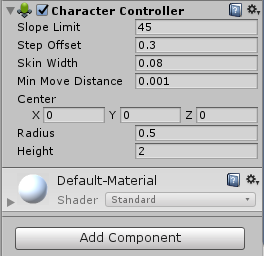
2.通过调用SimpleMove()使角色移动
public class Player : MonoBehaviour {
CharacterController cc;
float speed = 100f;
void Start () {
cc = GetComponent<CharacterController>();
}
void Update () {
float h = Input.GetAxis("Horizontal")*Time.deltaTime*speed;
float v = Input.GetAxis("Vertical") * Time.deltaTime * speed;
cc.SimpleMove(new Vector3(h,0,v));
}
}
cc.SimpleMove(new Vector3(h, 0, v));
三、Animition动画系统 导入角色资源包:Player.unitypackage 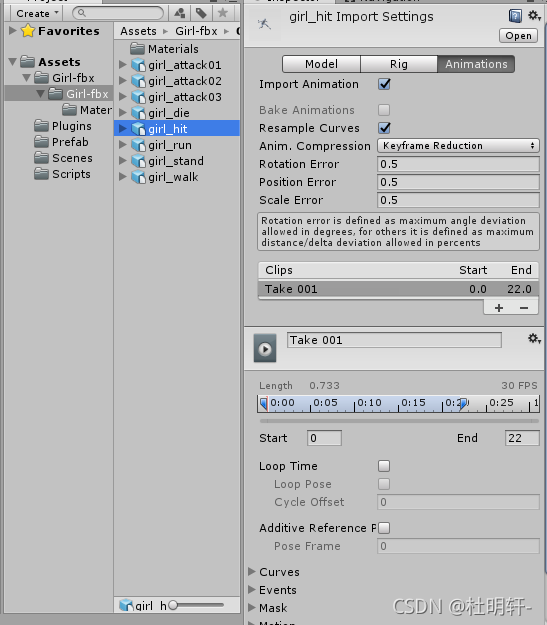
1.2 动画制作
1、更改所有动画类型为Legacy 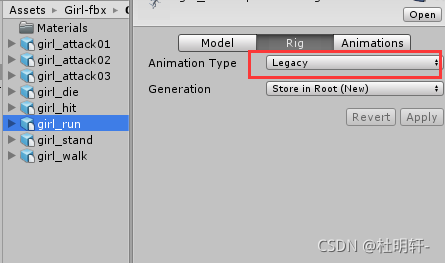
2、把Idle动画设置循环播放 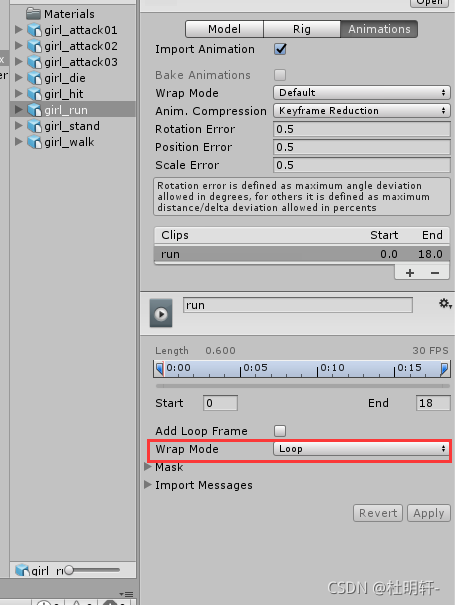
3、为角色添加多个动画 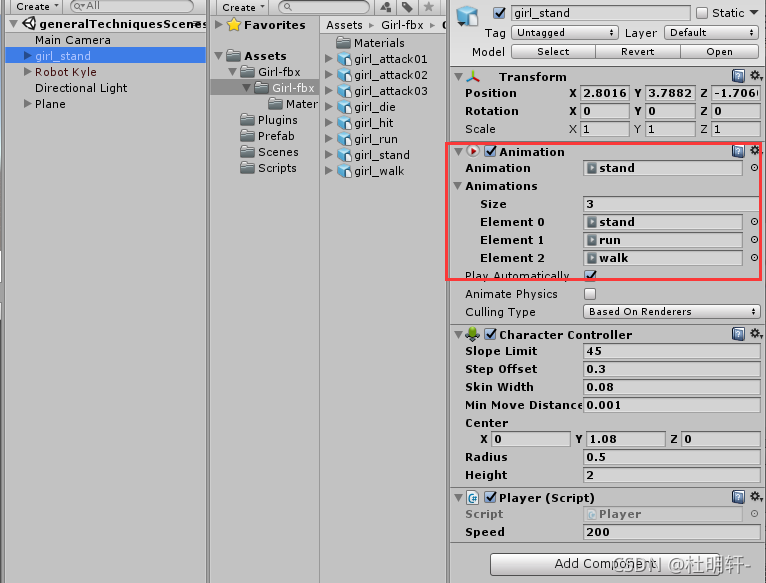
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour {
CharacterController cc;
Animation an;
public float speed;
void Start () {
cc = GetComponent<CharacterController>();
an = GetComponent<Animation>();
}
void Update () {
float h = Input.GetAxis("Horizontal")*Time.deltaTime*speed;
float v =Input.GetAxis("Vertical")*Time.deltaTime*speed;
if (Input.GetKeyDown(KeyCode.Space))
{
transform.position += Vector3.up;
}
if (h!=0||v!=0)
{
cc.SimpleMove(new Vector3(h, 0, v));
an.Play("walk");
}
else
{
an.Play("stand");
}
}
|