这次我会开一个系列,持续更新(也不排除放弃的可能)。
先进行基本的框架搭建
import pygame,sys
canvas=pygame.display.set_mode((1350,680))
pygame.display.set_caption('卡牌')
event=[]
def handleEvent():
global events
for e in pygame.event.get():
if e.type==pygame.QUIT:
pygame.quit()
sys.exit()
while True:
handleEvent()
pygame.display.update()
接下来,我要创建一个地图,大体参照了最近游戏《邪恶冥刻》的形式:
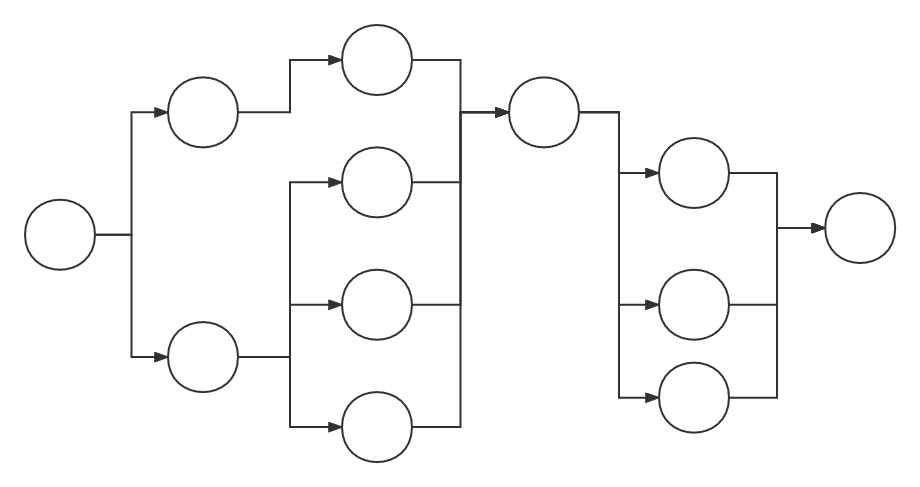
?我总结了一下它的逻辑:
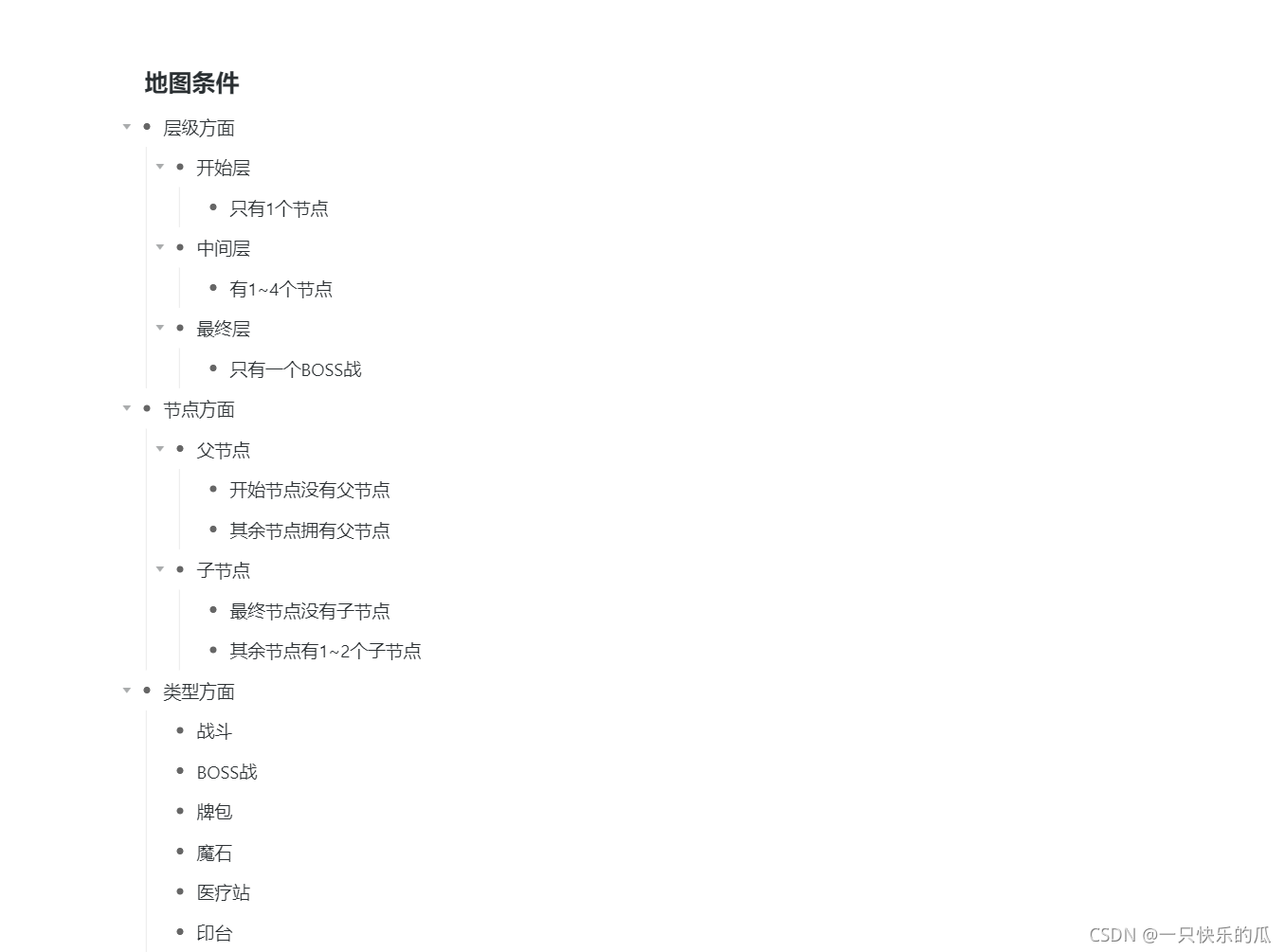
?既然它有这么多方面。那我们肯定会按其中一方面生成并在同时满足其他方面:这里我选择依照层级方面生成:
稍等,我实在是对我的代码水平不自信,咱们还是先做出显示函数吧。
def mapDisplay():
while True:
handleEvent()
canvas.blit(mapImg[0],(0,0))
canvas.blit(mapImg[1],(0,0))
pygame.display.update()
mapDisplay()
现在可以开始一边编写算法一边完善显示了:
#记得引入random库并以r使用
def create_map(mapLength):
global mapNotes
notes_events=['card','printer','hospital','magic_stone','fight']
mapNotes=[]
for i in range(mapLength):
if i==0:
mapNotes.append(['card'])
elif i==mapLength-1:
mapNotes.append(['boss_fight'])
else:
tempL=[]
for i in range(r.randint(1,4)):
tempL.append(notes_events[r.randint(0,len(notes_events)-1)])
mapNotes.append(tempL)
def mapDisplay():
print(mapNotes)
while True:
handleEvent()
canvas.blit(mapImg[0],(0,0))
canvas.blit(mapImg[1],(0,0))
pygame.display.update()
我们现在能生成符合要求的节点了,来开始生成连线吧!
先说一下逻辑:看看我们的示意图:
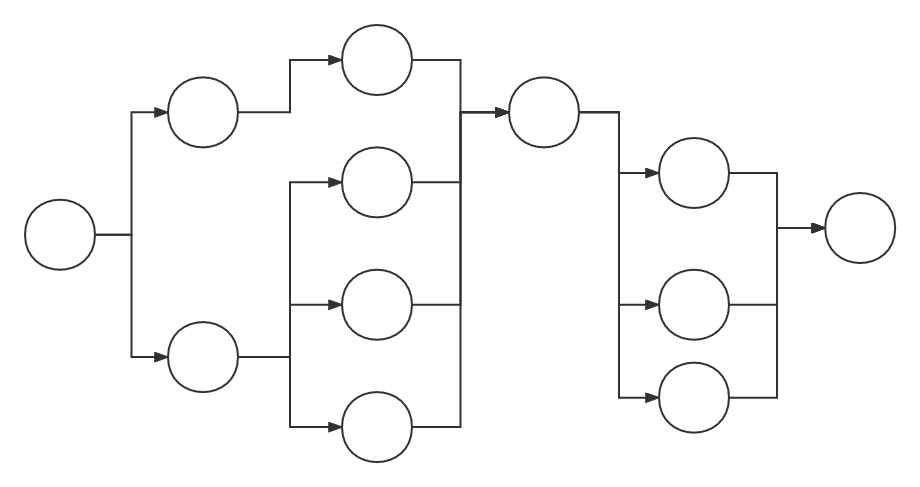
?是不是可以以一个 起点*终点 大小,1表示连接,0表示断开的矩阵表示?例如第二排到第三排
? ? ?1? ? 2? ? 3? ? 4? ? ? ? 第三至第四? ? 1? ? 1? ? 1? ? 1
1? ?1? ? 0? ? 0? ? 0
2? ?0? ? 1? ? 1? ? 1
我们发现了规律:每一行每一列至少但不限于1条连接,现在我们可以开始了:
#注意导入numpy库并以np调用
def create_map(mapLength):
global mapNotes,mapWires
#节点生成
notes_events=['card','printer','hospital','magic_stone','fight']
mapNotes=[]
for i in range(mapLength):
if i==0:
mapNotes.append(['card'])
elif i==mapLength-1:
mapNotes.append(['boss_fight'])
else:
tempL=[]
for i in range(r.randint(1,4)):
tempL.append(notes_events[r.randint(0,len(notes_events)-1)])
mapNotes.append(tempL)
#连线生成
mapWires=[]
for i in range(mapLength-1):
tempArray=np.zeros((len(mapNotes[i]),len(mapNotes[i+1])))
for j in range(len(mapNotes[i])):
tempArray[j,r.randint(0,len(mapNotes[i+1])-1)]=1
for k in range(len(mapNotes[i+1])):
tempArray[r.randint(0,len(mapNotes[i])-1),k]=1
mapWires.append(tempArray)
def mapDisplay():
print(mapNotes)
for i in range(4):
print(mapWires[i])
while True:
handleEvent()
canvas.blit(mapImg[0],(0,0))
canvas.blit(mapImg[1],(0,0))
pygame.display.update()
这是我的其中一次运行结果:
[['card'], ['printer', 'hospital', 'card', 'card']
, ['magic_stone', 'hospital', 'printer'], ['hospital', 'hospital'], ['boss_fight']]
[[1. 1. 1. 1.]]
[[0. 1. 1.]
[0. 1. 1.]
[1. 0. 1.]
[1. 0. 0.]]
[[1. 1.]
[0. 1.]
[1. 1.]]
[[1.]
[1.]]
我手绘了一下以展示:
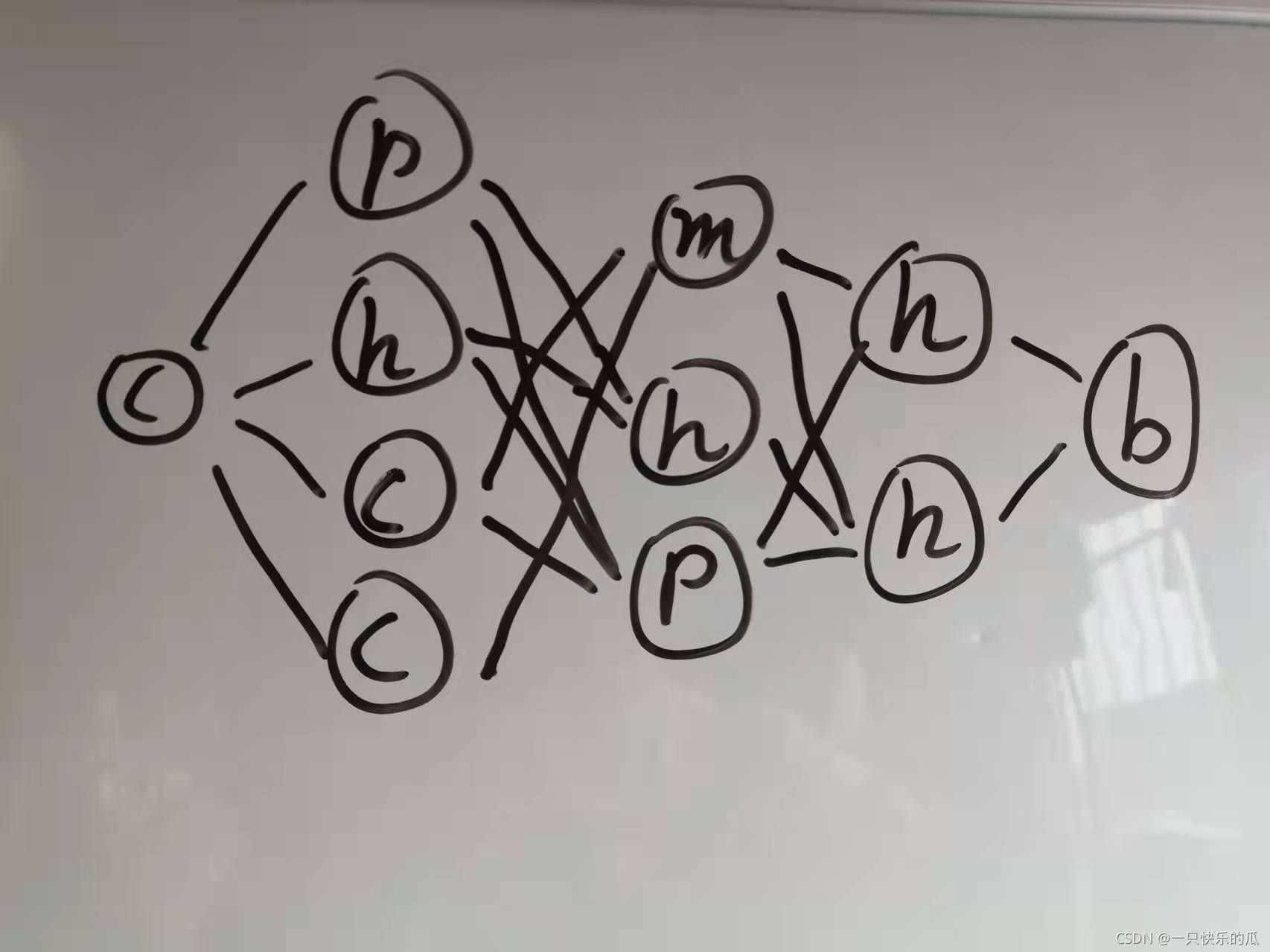
?有点乱,但我满足了。让我们添加显示系统吧。
import pygame,sys,random as r,numpy as np
canvas=pygame.display.set_mode((1350,680))
pygame.display.set_caption('卡牌')
#加载图片
mapImg={0:pygame.image.load('images/map(layer0).png'),\
1:pygame.image.load('images/map(layer1).png')}
noteImg={'card':pygame.image.load('images/notes_events/card.png'),\
'printer':pygame.image.load('images/notes_events/printer.png'),\
'hospital':pygame.image.load('images/notes_events/hospital.png'),\
'magic_stone':pygame.image.load('images/notes_events/magic_stone.png'),\
'fight':pygame.image.load('images/notes_events/fight.png'),\
'boss_fight':pygame.image.load('images/notes_events/boss.png')}
#触发事件
event=[]
def handleEvent():
global events
for e in pygame.event.get():
if e.type==pygame.QUIT:
pygame.quit()
sys.exit()
def create_map(mapLength):
global mapNotes,mapWires
#节点生成
notes_events=['card','printer','hospital','magic_stone','fight']
mapNotes=[]
for i in range(mapLength):
if i==0:
mapNotes.append(['card'])
elif i==mapLength-1:
mapNotes.append(['boss_fight'])
else:
tempL=[]
for i in range(r.randint(1,4)):
tempL.append(notes_events[r.randint(0,len(notes_events)-1)])
mapNotes.append(tempL)
#连线生成
mapWires=[]
for i in range(mapLength-1):
tempArray=np.zeros((len(mapNotes[i]),len(mapNotes[i+1])))
for j in range(len(mapNotes[i])):
tempArray[j,r.randint(0,len(mapNotes[i+1])-1)]=1
for k in range(len(mapNotes[i+1])):
tempArray[r.randint(0,len(mapNotes[i])-1),k]=1
mapWires.append(tempArray)
noteDisplayPos={1:{0:316},2:{0:188,1:408},3:{0:150,1:280,2:400},4:{0:120,1:270,2:340,3:510}}
def mapDisplay():
print(mapNotes)
for i in range(4):
print(mapWires[i])
playerLayer=0
while True:
handleEvent()
canvas.blit(mapImg[0],(0,0))
for i in range(len(mapNotes)):
for j in range(len(mapNotes[i])):
canvas.blit(noteImg[mapNotes[i][j]],((i-playerLayer)*200+250,noteDisplayPos[len(mapNotes[i])][j]))
for i in range(len(mapWires)):
wires=mapWires[i]
for a in range(wires.shape[0]):
for b in range(wires.shape[1]):
if wires[a,b]==1:
pygame.draw.line(canvas,(10,10,10),\
((i-playerLayer)*200+250+70,noteDisplayPos[len(mapNotes[i])][a]+32),\
((i+1-playerLayer)*200+250-6,noteDisplayPos[len(mapNotes[i+1])][b]+32),4)
canvas.blit(mapImg[1],(0,0))
pygame.display.update()
create_map(7)
mapDisplay()
现在地图系统暂时告一段落,让我们去编写战斗吧。
(15条消息) images.rarrrrrrrrrrrrrrr-其他文档类资源-CSDN文库
|